Project Submission (late)
Dependencies: mbed
Maze.h
00001 #ifndef MAZE_H 00002 #define MAZE_H 00003 00004 /** Maze Class 00005 * @brief This class is responsible for selecting, storing and manipulating the current maze. 00006 * @brief It also makes it easier to pass the 2D array of the matrix to other classes 00007 * @brief that need access to the maze's data, such as Drawer and Player. 00008 * 00009 * @brief All pre-defined matrices are 20x20 in size, even if their actual size is smaller 00010 * @brief The extra space is padded with 1's, this is to make the function calling significantly 00011 * @brief simpler. 00012 * 00013 * @brief Version 1.0 00014 * @author Thomas Caine 00015 * @date May 2019 00016 */ 00017 class Maze { 00018 00019 public: 00020 /** Create a Maze object to manage the maze matrix. 00021 */ 00022 Maze(); 00023 /** Fill the maze matrix with a specific value in every cell 00024 * @param val - the value to put in every cell 00025 * Used to reset the maze by setting it to all 1's (solid cells) 00026 * every time the game restarts. 00027 */ 00028 void fillMaze(int val); 00029 /** Copies the contents of a maze to the object's version 00030 * @param maze - a predefined maze to be copied into the object's matrix field. 00031 * @details the mazes passed to this function are all pre-defined in MazeMatrices.h 00032 */ 00033 void copyMaze(int maze[20][20]); 00034 /** Randomly selects a maze of a specified size 00035 * @param size - can be 12, 16 or 20, all mazes are square. 00036 * @details this function will randomly select one from 3 pre-defined mazes. 00037 */ 00038 void selectMaze(int size); 00039 00040 public: 00041 int mazeMatrix[20][20]; 00042 int startX; 00043 int startY; 00044 int timeToFinish; 00045 00046 }; 00047 00048 00049 00050 #endif // MAZE_H
Generated on Thu Jul 14 2022 20:06:28 by
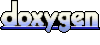