Project Submission (late)
Dependencies: mbed
Maze.cpp
00001 #include <ctime> // for seeding the random number generator 00002 #include <cstdlib> // for rand() 00003 #include "Maze.h" 00004 #include "mbed.h" 00005 #include "MazeMatrices.h" 00006 00007 // Constructor generates a maze of solid cells 00008 Maze::Maze() { 00009 fillMaze(1); 00010 } 00011 00012 // Fills the maze with a specific value 00013 void Maze::fillMaze(int val) { 00014 for (int i = 0; i < 20; i++) { 00015 for (int j = 0; j < 20; j++) { 00016 mazeMatrix[i][j] = val; 00017 } 00018 } 00019 } 00020 00021 // cycles through each index to copy a maze to the 00022 // the maze as stored in the Maze object 00023 void Maze::copyMaze(int maze[20][20]) { 00024 fillMaze(1); 00025 for (int i = 0; i < 20; i++) { 00026 for (int j = 0; j < 20; j++) { 00027 mazeMatrix[i][j] = maze[i][j]; 00028 } 00029 } 00030 } 00031 00032 // randomly selects between 3 variants for one of the 3 available sizes 00033 // there are 9 mazes in total, the size is user specified but which variant is random 00034 void Maze::selectMaze(int size) { 00035 printf("selecting maze size\n"); 00036 srand(time(0)); 00037 int variant = rand() % 3 + 1; 00038 printf("maze %i was randomly chosen\n", variant); 00039 switch (size) { 00040 case 12: 00041 printf("mazeSize is 12\n"); 00042 switch (variant) { 00043 case 1: 00044 copyMaze(maze12one); 00045 startX = 10; 00046 startY = 10; 00047 break; 00048 case 2: 00049 copyMaze(maze12two); 00050 startX = 5; 00051 startY = 10; 00052 break; 00053 case 3: 00054 copyMaze(maze12three); 00055 startX = 1; 00056 startY = 10; 00057 break; 00058 } 00059 timeToFinish = 120; 00060 break; 00061 case 16: 00062 printf("mazeSize is 16\n"); 00063 switch (variant) { 00064 case 1: 00065 copyMaze(maze16one); 00066 startX = 7; 00067 startY = 14; 00068 break; 00069 case 2: 00070 copyMaze(maze16two); 00071 startX = 4; 00072 startY = 9; 00073 break; 00074 case 3: 00075 copyMaze(maze16three); 00076 startX = 12; 00077 startY = 14; 00078 break; 00079 } 00080 timeToFinish = 180; 00081 break; 00082 case 20: 00083 printf("mazeSize is 20\n"); 00084 switch (variant) { 00085 case 1: 00086 copyMaze(maze20one); 00087 startX = 16; 00088 startY = 18; 00089 break; 00090 case 2: 00091 copyMaze(maze20one); 00092 startX = 10; 00093 startY = 10; 00094 break; 00095 case 3: 00096 copyMaze(maze20one); 00097 startX = 3; 00098 startY = 18; 00099 break; 00100 } 00101 timeToFinish = 240; 00102 break; 00103 } 00104 }
Generated on Thu Jul 14 2022 20:06:28 by
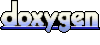