Project Submission (late)
Dependencies: mbed
MainMenu.h
00001 #ifndef MAINMENU_H 00002 #define MAINMENU_H 00003 00004 #include "StartMenu.h" 00005 #include "OptionsMenu.h" 00006 #include "MenuGraphics.h" 00007 // StartButton takes you to the start menu screen. 00008 /** StartButton Class 00009 * @brief Derived from Button. Takes you to the StartMenu screen where game params are set. 00010 */ 00011 class StartButton : public Button { 00012 public: 00013 N5110* lcd; 00014 StartButton(N5110* screenPtr) : lcd(screenPtr) { 00015 printf("StartButton constructor\n"); 00016 x = 5; 00017 y = 16; 00018 } 00019 void virtual run() { 00020 printf("StartButton\n"); 00021 delete currentMenu; // free the previous memory assigned to the pointer 00022 currentMenu = new StartMenu(lcd); // assign the new (next) menu to it 00023 } 00024 }; 00025 00026 // OptionsButton takes you to the game's options. 00027 /** OptionsButton Class 00028 * @brief Derived from Button. Takes you to the options menu. 00029 */ 00030 class OptionsButton : public Button { 00031 public: 00032 N5110* lcd; 00033 OptionsButton(N5110* screenPtr) : lcd(screenPtr) { 00034 printf("OptionsButton constructor\n"); 00035 x = 5; 00036 y = 24; 00037 } 00038 void virtual run() { 00039 printf("OptionsButton\n"); 00040 delete currentMenu; // free the previous memory assigned to the pointer 00041 currentMenu = new OptionsMenu(lcd); // assign the new (next) menu to it 00042 } 00043 }; 00044 00045 // The MainMenu is the first menu displayed. 00046 // It has only two buttons because I did not have time for a leaderboard 00047 /** MainMenu Class 00048 * @brief Derived from Menu. MainMenu is the first menu displayed and serves as the start of the program loop. 00049 */ 00050 class MainMenu : public Menu { 00051 public: 00052 //Button* buttons[2]; 00053 MainMenu(N5110* screenPtr) : Menu(screenPtr) { 00054 buttons[0] = new StartButton(lcd); 00055 buttons[1] = new OptionsButton(lcd); 00056 currentButton = buttons[0]; 00057 numOfButtons = 2; 00058 buttonIndex = 0; 00059 } 00060 void virtual draw() { 00061 lcd->drawSprite(20,1,12,44,(int *)menuGraphic); 00062 lcd->printString("Start",10,2); 00063 lcd->printString("Options",10,3); 00064 } 00065 /** Destructor 00066 */ 00067 ~MainMenu() { 00068 delete buttons[0]; 00069 delete buttons[1]; 00070 } 00071 }; 00072 00073 #endif // MAINMENU_H
Generated on Thu Jul 14 2022 20:06:28 by
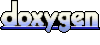