Project Submission (late)
Dependencies: mbed
Gamepad.h
00001 #ifndef GAMEPAD_H 00002 #define GAMEPAD_H 00003 00004 #include <bitset> 00005 00006 // Forward declaration of the classes that we use from the mbed library 00007 // This avoids the need for us to include the huge mbed.h header inside our 00008 // own library API 00009 00010 namespace mbed 00011 { 00012 class AnalogIn; 00013 class InterruptIn; 00014 class PwmOut; 00015 class Timeout; 00016 } 00017 00018 #define TOL 0.1f 00019 #define RAD2DEG 57.2957795131f 00020 00021 /** Enum for direction */ 00022 enum Direction { 00023 CENTRE, /**< joystick centred */ 00024 N, /**< pushed North (0)*/ 00025 NE, /**< pushed North-East (45) */ 00026 E, /**< pushed East (90) */ 00027 SE, /**< pushed South-East (135) */ 00028 S, /**< pushed South (180) */ 00029 SW, /**< pushed South-West (225) */ 00030 W, /**< pushed West (270) */ 00031 NW /**< pushed North-West (315) */ 00032 }; 00033 00034 /** Vector 2D struct */ 00035 struct Vector2D { 00036 float x; /**< float for x value */ 00037 float y; /**< float for y value */ 00038 }; 00039 00040 /** Polar coordinate struct */ 00041 struct Polar { 00042 float mag; /**< float for magnitude */ 00043 float angle; /**< float for angle (in degrees) */ 00044 }; 00045 00046 /** Gamepad Class 00047 * @brief Library for interfacing with ELEC2645 Gamepad PCB, University of Leeds 00048 * @author Dr Craig A. Evans 00049 * @author Dr Alex Valavanis 00050 */ 00051 class Gamepad 00052 { 00053 public: 00054 /** Gamepad events 00055 * @brief List of events that can be registered on the gamepad 00056 */ 00057 enum GamepadEvent { 00058 A_PRESSED, ///< Button A has been pressed 00059 B_PRESSED, ///< Button B has been pressed 00060 X_PRESSED, ///< Button X has been pressed 00061 Y_PRESSED, ///< Button Y has been pressed 00062 L_PRESSED, ///< Button L has been pressed 00063 R_PRESSED, ///< Button R has been pressed 00064 BACK_PRESSED, ///< Button "Back" has been pressed 00065 START_PRESSED, ///< Button "Start" has been pressed 00066 JOY_PRESSED, ///< Joystick button has been pressed 00067 N_EVENTS ///< A dummy flag that marks the end of the list 00068 }; 00069 00070 private: 00071 mbed::PwmOut *_led1; 00072 mbed::PwmOut *_led2; 00073 mbed::PwmOut *_led3; 00074 mbed::PwmOut *_led4; 00075 mbed::PwmOut *_led5; 00076 mbed::PwmOut *_led6; 00077 00078 mbed::InterruptIn *_button_A; 00079 mbed::InterruptIn *_button_B; 00080 mbed::InterruptIn *_button_X; 00081 mbed::InterruptIn *_button_Y; 00082 mbed::InterruptIn *_button_L; 00083 mbed::InterruptIn *_button_R; 00084 mbed::InterruptIn *_button_back; 00085 mbed::InterruptIn *_button_start; 00086 mbed::InterruptIn *_button_joystick; 00087 00088 mbed::AnalogIn *_vert; 00089 mbed::AnalogIn *_horiz; 00090 00091 mbed::PwmOut *_buzzer; 00092 mbed::AnalogIn *_pot; 00093 00094 mbed::Timeout *_timeout; 00095 00096 std::bitset<N_EVENTS> _event_state; ///< A binary list of buttons that has been pressed 00097 00098 // centred x,y values 00099 float _x0; 00100 float _y0; 00101 00102 public: 00103 /** Constructor */ 00104 Gamepad(); 00105 00106 /** Destructor */ 00107 ~Gamepad(); 00108 00109 /** Initialise all peripherals and configure interrupts */ 00110 void init(); 00111 00112 /** Turn all LEDs on */ 00113 void leds_on(); 00114 00115 /** Turn all LEDs off */ 00116 void leds_off(); 00117 00118 /** Set all LEDs to duty-cycle 00119 *@param value in range 0.0 to 1.0 00120 */ 00121 void leds(float val) const; 00122 00123 /** Set LED to duty-cycle 00124 *@param led number (0 to 5) 00125 *@param value in range 0.0 to 1.0 00126 */ 00127 void led(int n,float val) const; 00128 00129 /** Read potentiometer 00130 *@returns potentiometer value in range 0.0 to 1.0 00131 */ 00132 float read_pot() const; 00133 00134 /** Play tone on piezo 00135 * @param frequency in Hz 00136 * @param duration of tone in seconds 00137 */ 00138 void tone(float frequency, float duration); 00139 00140 /** 00141 * @brief Check whether an event flag has been set and clear it 00142 * @param id[in] The ID of the event to test 00143 * @return true if the event occurred 00144 */ 00145 bool check_event(GamepadEvent const id); 00146 00147 /** 00148 * @brief Get the raw binary event state 00149 * @return The event state as a binary code 00150 * @details The check_event() function is likely to be more useful than 00151 * this, for testing whether a given event has occurred. It can be 00152 * useful for debugging via the terminal, however, for example: 00153 * @code 00154 * std::cout << gamepad.get_raw_event_state() << std::endl; 00155 * @endcode 00156 */ 00157 inline std::bitset<N_EVENTS> get_raw_event_state() const { 00158 return _event_state; 00159 } 00160 00161 /** Get magnitude of joystick movement 00162 * @returns value in range 0.0 to 1.0 00163 */ 00164 float get_mag(); 00165 00166 /** Get angle of joystick movement 00167 * @returns value in range 0.0 to 359.9. 0.0 corresponds to N, 180.0 to S. -1.0 is central 00168 */ 00169 float get_angle(); 00170 00171 /** Gets joystick direction 00172 * @returns an enum: CENTRE, N, NE, E, SE, S, SW, W, NW, 00173 */ 00174 Direction get_direction(); // N,NE,E,SE etc. 00175 00176 /** Gets raw cartesian co-ordinates of joystick 00177 * @returns a struct with x,y members, each in the range 0.0 to 1.0 00178 */ 00179 Vector2D get_coord(); // cartesian co-ordinates x,y 00180 00181 /** Gets cartesian coordinates mapped to circular grid 00182 * @returns a struct with x,y members, each in the range 0.0 to 1.0 00183 */ 00184 Vector2D get_mapped_coord(); // x,y mapped to circle 00185 00186 /** Gets polar coordinates of the joystick 00187 * @returns a struct contains mag and angle 00188 */ 00189 Polar get_polar(); // mag and angle in struct form 00190 00191 private: 00192 void init_buttons(); 00193 void tone_off(); 00194 00195 void a_isr(); 00196 void b_isr(); 00197 void x_isr(); 00198 void y_isr(); 00199 void l_isr(); 00200 void r_isr(); 00201 void back_isr(); 00202 void start_isr(); 00203 void joy_isr(); 00204 }; 00205 00206 #endif
Generated on Thu Jul 14 2022 20:06:28 by
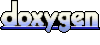