
Initial publish
Dependencies: mbed
Fork of el17dg by
stars.h
00001 #ifndef STARS_H 00002 #define STARS_H 00003 00004 /** 00005 * Stars Class 00006 * @brief Manages, updates and draws the background stars. 00007 * @author Dmitrijs Griskovs 00008 * @date 15/04/2019 00009 */ 00010 class Stars { 00011 public: 00012 /** 00013 * @var static const int max_small_stars variable; 00014 * @brief Sets the limit of maximum small stars allowed on the screen. 00015 */ 00016 static const int max_small_stars = 10; 00017 /** 00018 * @var static const int max_medium_stars variable; 00019 * @brief Sets the limit of maximum medium stars allowed on the screen. 00020 */ 00021 static const int max_medium_stars = 5; 00022 00023 GameObject small_stars[max_small_stars]; 00024 GameObject medium_stars[max_medium_stars]; 00025 00026 /** 00027 * @brief draws small stars on the screen. 00028 * @details when a small star is active, this function updates the position and 00029 * draws it on the screen. Also, When the star leaves the screen limits it 00030 * deactivates the star for a new star to fly. 00031 */ 00032 void updateAndDrawSmallStars(){ 00033 for (int i = 0; i < max_small_stars; ++i) { 00034 if (small_stars[i].active) { 00035 small_stars[i].pos.x -= small_star_speed; 00036 if (small_stars[i].pos.x <= 0){ 00037 small_stars[i].active = false; 00038 } 00039 drawSpriteOnTop(small_stars[i].pos, small_star_sprite); 00040 } 00041 } 00042 } 00043 00044 /** 00045 * @brief draws medium stars on the screen. 00046 * @details when a medium star is active, this function updates the position and 00047 * draws it on the screen. Also, When the star leaves the screen limits it 00048 * deactivates the star for a new star to fly. 00049 */ 00050 void updateAndDrawMediumStars(){ 00051 for (int i = 0; i < max_medium_stars; ++i) { 00052 if (medium_stars[i].active) { 00053 medium_stars[i].pos.x -= medium_star_speed; 00054 if (medium_stars[i].pos.x <= -2){ 00055 medium_stars[i].active = false; 00056 } 00057 drawSpriteOnTop(medium_stars[i].pos, medium_star_sprite); 00058 } 00059 } 00060 } 00061 00062 /** @brief A separate function for delaying the stars spawn time.*/ 00063 void starsSpawnDelay(){ 00064 if (stars_delay == stars_delay_max){ 00065 //This is dealy between stars generation. 00066 newSmallStarFlies(); 00067 newMediumStarFlies(); 00068 stars_delay = 0; 00069 } 00070 else { 00071 stars_delay += 1; 00072 } 00073 } 00074 /** 00075 * @var int stars delay; 00076 * @brief The value is incremented every time until it reaches stars_delay_max 00077 * value, which triggers the stars spawn and then is reseted to zero. . 00078 */ 00079 int stars_delay; 00080 00081 00082 00083 private: 00084 /** 00085 * @var static const int small_star_speed variable; 00086 * @brief Sets the speed of the small stars. 00087 */ 00088 static const int small_star_speed = 2; 00089 /** 00090 * @var static const int medium_star_speed variable; 00091 * @brief Sets the speed of the medium stars. 00092 */ 00093 static const int medium_star_speed = 4; 00094 /** 00095 * @var static const int stars_delay_max variable; 00096 * @brief Sets the limit of the stars delay spawn. 00097 */ 00098 static const int stars_delay_max = 5; 00099 00100 /** 00101 * @brief Makes a small star active and gives it the start positions. 00102 * @details Searches through the small stars array and if a star is not active, 00103 * it becomes active and is given the position of x (which is 0) and. 00104 * and random position of y. 00105 */ 00106 void newSmallStarFlies() { 00107 // Search the array of stars if inactive we can use it. - the same as with blasts 00108 int found = -1; 00109 for (int i = 0; i < max_small_stars; ++i) { 00110 if (!small_stars[i].active) { 00111 found = i; 00112 break; 00113 } 00114 } 00115 00116 if (found != -1) { 00117 small_stars[found].active = true; 00118 small_stars[found].pos.x = screen_width; 00119 small_stars[found].pos.y = rand() % screen_height + game_area_y; 00120 } 00121 } 00122 00123 /** 00124 * @brief Makes a medium star active and gives it the start positions. 00125 * @details Searches through the medium stars array and if a star is not active, 00126 * it becomes active and is given the position of x (which is 0) and. 00127 * and random position of y. 00128 */ 00129 void newMediumStarFlies() { 00130 // Search the array of stars if inactive we can use it. - the same as with blasts 00131 int found = -1; 00132 for (int i = 0; i < max_medium_stars; ++i) { 00133 if (!medium_stars[i].active) { 00134 found = i; 00135 break; 00136 } 00137 } 00138 if (found != -1) { 00139 medium_stars[found].active = true; 00140 medium_stars[found].pos.x = screen_width; 00141 medium_stars[found].pos.y = rand() % screen_height + game_area_y; 00142 } 00143 } 00144 }; 00145 #endif
Generated on Thu Jul 14 2022 14:15:02 by
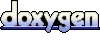