
Initial publish
Dependencies: mbed
Fork of el17dg by
gameovermanager.h
00001 #ifndef GAMEOVERMANAGER_H 00002 #define GAMEOVERMANAGER_H 00003 00004 /** 00005 * GameOverManager Class 00006 * @brief A class for updating and drawing GameOver state. 00007 * @author Dmitrijs Griskovs 00008 * @date 30/04/2019 00009 */ 00010 class GameOverManager { 00011 public: 00012 /** 00013 * Constructor. 00014 * @brief It init the GameOver with reseting 00015 * the statrting sequence of the gameOver state. 00016 */ 00017 GameOverManager() { reset(); } 00018 00019 /** 00020 * @brief Resets the gameOver to the animiation to appear again 00021 * @returns bool started, when GameOver begins. 00022 */ 00023 void reset() { started = false; } 00024 /** 00025 * @brief Updates and draws the game over animation. 00026 * @details It draws "GameOver" sprite from the leftside of the screen to the center 00027 * and "youDied" sprite from the right side to the center. 00028 */ 00029 void updateAndDraw() { 00030 if (!started) { startAnimation();} 00031 if (animation_counter < animation_length) { 00032 if (gamepad.check_event(gamepad.START_PRESSED)) { 00033 gameOverLogo.pos.x = game_area_x - 29 + 42; 00034 youDied.pos.x = game_area_width - 42; 00035 animation_counter = animation_length; 00036 } else { 00037 animation_counter++; 00038 gameOverLogo.pos.x += 1; 00039 youDied.pos.x -= 1; 00040 } 00041 } 00042 drawSprite(gameOverLogo.pos, game_over_sprite); 00043 drawSprite(youDied.pos, you_died_sprite); 00044 gameOverPostAnimation(); 00045 musicGameOver(); 00046 ledsGameOver(); 00047 } 00048 00049 /** 00050 * @brief Checks for whether the animation of GameOver has stoped drawing or not 00051 * @details It check the value of the counter against the total length of the 00052 * animation play. When the counter equals to the total length, it indicates that 00053 * the animation has stopped 00054 */ 00055 bool isPlayingAnimation() { 00056 return animation_counter < animation_length; 00057 }; 00058 00059 private: 00060 void startAnimation() { 00061 started = true; 00062 gameOverLogo.pos.x = game_area_x - 29; // 0 - the sprite length 00063 gameOverLogo.pos.y = game_area_y; 00064 youDied.pos.x = game_area_width; 00065 youDied.pos.y = game_area_y; 00066 animation_counter = 0; 00067 led_state = false; 00068 low_frequency_music_counter = 0; 00069 high_frequency_music_counter = 0; 00070 } 00071 00072 void ledsGameOver(){ 00073 gamepad.led(1,(float)led_state); 00074 gamepad.led(2,(float)!led_state); 00075 gamepad.led(3,(float)led_state); 00076 gamepad.led(4,(float)!led_state); 00077 gamepad.led(5,(float)led_state); 00078 gamepad.led(6,(float)!led_state); 00079 led_state = !led_state; 00080 } 00081 00082 void musicGameOver(){ 00083 lowFrequencyPartMusic(); 00084 highFrequencyPartMusic(); 00085 high_frequency_music_counter++; 00086 low_frequency_music_counter++;//comment out this for epic game over beat. 00087 DG_PRINTF("Low frequency counter value:: %i\n", low_frequency_music_counter); 00088 DG_PRINTF("high frequency counter value:: %i\n", high_frequency_music_counter); 00089 } 00090 00091 void lowFrequencyPartMusic(){ 00092 // Low frequency 00093 if (low_frequency_music_counter == 0){ gamepad.tone(60,3);} 00094 else if (low_frequency_music_counter == 3){gamepad.tone(90,3);} 00095 else if (low_frequency_music_counter == 6){gamepad.tone(60,3);} 00096 else if (low_frequency_music_counter == 9){gamepad.tone(80,3);} 00097 else if (low_frequency_music_counter == 12){gamepad.tone(70,2);} 00098 else if (low_frequency_music_counter == 14){gamepad.tone(60,2);} 00099 else if (low_frequency_music_counter == 16){gamepad.tone(70,3);} 00100 else if (low_frequency_music_counter == 19){gamepad.tone(50,1);} 00101 else if (low_frequency_music_counter == 20){gamepad.tone(40,3);} 00102 else if (low_frequency_music_counter== 23){ 00103 gamepad.tone(60,2); 00104 low_frequency_music_counter = 0; 00105 } 00106 } 00107 00108 void highFrequencyPartMusic(){ 00109 // High frequency 00110 if ( high_frequency_music_counter == 0){ gamepad.tone(300,0.1);} 00111 else if (high_frequency_music_counter == 3){gamepad.tone(250,0.1);} 00112 else if (high_frequency_music_counter == 6){gamepad.tone(230,0.2);} 00113 else if (high_frequency_music_counter == 9){gamepad.tone(250,0.1);} 00114 else if ( high_frequency_music_counter == 12){gamepad.tone(250,0.2);} 00115 else if ( high_frequency_music_counter == 14){gamepad.tone(220,0.1);} 00116 else if ( high_frequency_music_counter == 16){gamepad.tone(210,0.3);} 00117 else if ( high_frequency_music_counter == 19){gamepad.tone(200,1);} 00118 else if ( high_frequency_music_counter == 21){gamepad.tone(250,1);} 00119 else if ( high_frequency_music_counter == 22){ 00120 gamepad.tone(200,1); 00121 high_frequency_music_counter = 0; 00122 } 00123 } 00124 void updateHighScore(){ 00125 if (GameGlobals::high_score < GameGlobals::game_score){ 00126 GameGlobals::high_score = GameGlobals::game_score; 00127 } 00128 } 00129 00130 void gameOverPostAnimation(){ 00131 char buffer[32]; 00132 sprintf(buffer,"Score: %i", GameGlobals::game_score); 00133 updateHighScore(); 00134 lcd.printString(buffer,0,3); 00135 lcd.printString("Press Y",0,4); 00136 lcd.printString("to restart",0,5); 00137 gamepad.check_event(gamepad.START_PRESSED); 00138 } 00139 00140 bool started; 00141 static const int animation_length = 42; 00142 int animation_counter; 00143 GameObject gameOverLogo; 00144 GameObject youDied; 00145 bool led_state; 00146 int low_frequency_music_counter; 00147 int high_frequency_music_counter; 00148 }; 00149 00150 #endif
Generated on Thu Jul 14 2022 14:15:02 by
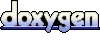