
Initial publish
Dependencies: mbed
Fork of el17dg by
game.h
00001 #ifndef GAME_H 00002 #define GAME_H 00003 00004 /** 00005 * GameGlobals Class 00006 * @brief Globals that different places in code can modify 00007 * @author Dmitrijs Griskovs 00008 * @date 22/04/2019 00009 */ 00010 class GameGlobals { 00011 public: 00012 static int game_score; 00013 static int score_count_for_difficulty; 00014 static int score_count_for_boss_mode; 00015 static int player_lifes; 00016 static int high_score; 00017 static bool is_shield_on; 00018 }; 00019 00020 /** 00021 * Game Class 00022 * @brief Stores general game logic. 00023 * @author Dmitrijs Griskovs 00024 * @date 15/04/2019 00025 */ 00026 class Game{ 00027 public: 00028 /** 00029 * Constructor. 00030 * @brief It is crucial to init game_state to GameState_newgame, so it calls 00031 * startNewGame() on the first update. 00032 */ 00033 Game() : game_state(GameState_newgame) { } 00034 00035 /** 00036 * @brief The main game function where all the gameplay and rendering happens. 00037 * @details This is the main function of game.cpp, where the actual gameplay happens. 00038 * Here all other functions are activeated, and when the player dies, it 00039 * returns back to main menu "main.cpp" it also draws all sprites in the game. 00040 * @returns bool want_to_pause, when "START" button is pressed. 00041 */ 00042 bool updateAndDraw(); 00043 00044 /** 00045 * @brief Resets all the in game variable when new game begins. 00046 * @details This function resets all the values to their intial states when 00047 * the game is first began when the player dies and wants to restart the game. 00048 * It does not reset the values when the game is paused. 00049 */ 00050 void startNewGame(); 00051 00052 /** 00053 * @brief Check whether the button R was pressed or not to turn ON/OFF the shield. 00054 * @details When the button R is pressed it sets bool "is_shield_active" to its opposite 00055 * value. If the shield is active then, a ship with force shield sprite is drawn and 00056 * the player's ability to shoot deactivates. 00057 */ 00058 bool forceShildActivate(); 00059 00060 private: 00061 00062 void checkButtonToShoot(); 00063 bool checkForGameOver(); 00064 void collideEnemiesAndBlasts(); 00065 void collideEnemiesBlastsAndPlayer(); 00066 void collideEnemiesAndPlayer(); 00067 void collideBossAndPlayerBlasts(); 00068 void collideBossBlastsAndPlayer(); 00069 void starsSpawnDelay(); 00070 void increaseGameDifficultyAndEnemySpawnDelay(); 00071 void printMusicCountersTest(); 00072 bool checkIfNeedsToReturnToMenu(); 00073 void updateAndDrawGameplay(); 00074 void updateAndDrawGameover(); 00075 void updateAndDrawBossCutscene(); 00076 void updateAndDrawBossGameplay(); 00077 00078 int enemy_ship_delay_counter; 00079 int enemy_ship_delay_max; 00080 bool is_boss_active; 00081 00082 00083 enum GameState { 00084 GameState_newgame, 00085 GameState_gameplay, 00086 GameState_boss_cutscene, 00087 GameState_boss_gameplay, 00088 GameState_gameover 00089 }; 00090 GameState game_state; 00091 }; 00092 00093 00094 #endif
Generated on Thu Jul 14 2022 14:15:02 by
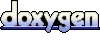