
Initial publish
Dependencies: mbed
Fork of el17dg by
Gamepad.h
00001 #ifndef GAMEPAD_H 00002 #define GAMEPAD_H 00003 00004 #include <bitset> 00005 00006 // Forward declaration of the classes that we use from the mbed library 00007 // This avoids the need for us to include the huge mbed.h header inside our 00008 // own library API 00009 namespace mbed 00010 { 00011 class AnalogIn; 00012 class InterruptIn; 00013 class PwmOut; 00014 class Timeout; 00015 } 00016 00017 #define TOL 0.1f 00018 #define RAD2DEG 57.2957795131f 00019 00020 /** Enum for direction */ 00021 enum Direction { 00022 CENTRE, /**< joystick centred */ 00023 N, /**< pushed North (0)*/ 00024 NE, /**< pushed North-East (45) */ 00025 E, /**< pushed East (90) */ 00026 SE, /**< pushed South-East (135) */ 00027 S, /**< pushed South (180) */ 00028 SW, /**< pushed South-West (225) */ 00029 W, /**< pushed West (270) */ 00030 NW /**< pushed North-West (315) */ 00031 }; 00032 00033 /** Vector 2D struct */ 00034 struct Vector2D { 00035 float x; /**< float for x value */ 00036 float y; /**< float for y value */ 00037 }; 00038 00039 /** Polar coordinate struct */ 00040 struct Polar { 00041 float mag; /**< float for magnitude */ 00042 float angle; /**< float for angle (in degrees) */ 00043 }; 00044 00045 /** Gamepad Class 00046 * @brief Library for interfacing with ELEC2645 Gamepad PCB, University of Leeds 00047 * @author Dr Craig A. Evans 00048 * @author Dr Alex Valavanis 00049 */ 00050 class Gamepad 00051 { 00052 public: 00053 /** Gamepad events 00054 * @brief List of events that can be registered on the gamepad 00055 */ 00056 enum GamepadEvent { 00057 A_PRESSED, ///< Button A has been pressed 00058 B_PRESSED, ///< Button B has been pressed 00059 X_PRESSED, ///< Button X has been pressed 00060 Y_PRESSED, ///< Button Y has been pressed 00061 L_PRESSED, ///< Button L has been pressed 00062 R_PRESSED, ///< Button R has been pressed 00063 BACK_PRESSED, ///< Button "Back" has been pressed 00064 START_PRESSED, ///< Button "Start" has been pressed 00065 JOY_PRESSED, ///< Joystick button has been pressed 00066 N_EVENTS ///< A dummy flag that marks the end of the list 00067 }; 00068 00069 private: 00070 mbed::PwmOut *_led1; 00071 mbed::PwmOut *_led2; 00072 mbed::PwmOut *_led3; 00073 mbed::PwmOut *_led4; 00074 mbed::PwmOut *_led5; 00075 mbed::PwmOut *_led6; 00076 00077 mbed::InterruptIn *_button_A; 00078 mbed::InterruptIn *_button_B; 00079 mbed::InterruptIn *_button_X; 00080 mbed::InterruptIn *_button_Y; 00081 mbed::InterruptIn *_button_L; 00082 mbed::InterruptIn *_button_R; 00083 mbed::InterruptIn *_button_back; 00084 mbed::InterruptIn *_button_start; 00085 mbed::InterruptIn *_button_joystick; 00086 00087 mbed::AnalogIn *_vert; 00088 mbed::AnalogIn *_horiz; 00089 00090 mbed::PwmOut *_buzzer; 00091 mbed::AnalogIn *_pot; 00092 00093 mbed::Timeout *_timeout; 00094 00095 std::bitset<N_EVENTS> _event_state; ///< A binary list of buttons that has been pressed 00096 00097 // centred x,y values 00098 float _x0; 00099 float _y0; 00100 00101 public: 00102 /** Constructor */ 00103 Gamepad(); 00104 00105 /** Destructor */ 00106 ~Gamepad(); 00107 00108 /** Initialise all peripherals and configure interrupts */ 00109 void init(); 00110 00111 /** Turn all LEDs on */ 00112 void leds_on(); 00113 00114 /** Turn all LEDs off */ 00115 void leds_off(); 00116 00117 /** Set all LEDs to duty-cycle 00118 *@param value in range 0.0 to 1.0 00119 */ 00120 void leds(float val) const; 00121 00122 /** Set LED to duty-cycle 00123 *@param led number (0 to 5) 00124 *@param value in range 0.0 to 1.0 00125 */ 00126 void led(int n,float val) const; 00127 00128 /** Read potentiometer 00129 *@returns potentiometer value in range 0.0 to 1.0 00130 */ 00131 float read_pot() const; 00132 00133 /** Play tone on piezo 00134 * @param frequency in Hz 00135 * @param duration of tone in seconds 00136 */ 00137 void tone(float frequency, float duration); 00138 00139 /** 00140 * @brief Check whether an event flag has been set and clear it 00141 * @param id[in] The ID of the event to test 00142 * @return true if the event occurred 00143 */ 00144 bool check_event(GamepadEvent const id); 00145 00146 /** 00147 * @brief Get the raw binary event state 00148 * @return The event state as a binary code 00149 * @details The check_event() function is likely to be more useful than 00150 * this, for testing whether a given event has occurred. It can be 00151 * useful for debugging via the terminal, however, for example: 00152 * @code 00153 * std::cout << gamepad.get_raw_event_state() << std::endl; 00154 * @endcode 00155 */ 00156 inline std::bitset<N_EVENTS> get_raw_event_state() const { 00157 return _event_state; 00158 } 00159 00160 /** Get magnitude of joystick movement 00161 * @returns value in range 0.0 to 1.0 00162 */ 00163 float get_mag(); 00164 00165 /** Get angle of joystick movement 00166 * @returns value in range 0.0 to 359.9. 0.0 corresponds to N, 180.0 to S. -1.0 is central 00167 */ 00168 float get_angle(); 00169 00170 /** Gets joystick direction 00171 * @returns an enum: CENTRE, N, NE, E, SE, S, SW, W, NW, 00172 */ 00173 Direction get_direction(); // N,NE,E,SE etc. 00174 00175 /** Gets raw cartesian co-ordinates of joystick 00176 * @returns a struct with x,y members, each in the range 0.0 to 1.0 00177 */ 00178 Vector2D get_coord(); // cartesian co-ordinates x,y 00179 00180 /** Gets cartesian coordinates mapped to circular grid 00181 * @returns a struct with x,y members, each in the range 0.0 to 1.0 00182 */ 00183 Vector2D get_mapped_coord(); // x,y mapped to circle 00184 00185 /** Gets polar coordinates of the joystick 00186 * @returns a struct contains mag and angle 00187 */ 00188 Polar get_polar(); // mag and angle in struct form 00189 00190 private: 00191 void init_buttons(); 00192 void tone_off(); 00193 00194 void a_isr(); 00195 void b_isr(); 00196 void x_isr(); 00197 void y_isr(); 00198 void l_isr(); 00199 void r_isr(); 00200 void back_isr(); 00201 void start_isr(); 00202 void joy_isr(); 00203 }; 00204 00205 #endif
Generated on Thu Jul 14 2022 14:15:02 by
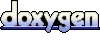