
Ikenna Adrian Ozoemena 201157039
Dependencies: mbed
main.cpp
00001 /* 00002 ELEC2645 Embedded Systems Project 00003 School of Electronic & Electrical Engineering 00004 University of Leeds 00005 Name: Ikenna Adrian Ozoemena 00006 Username: el17aio 00007 Student ID Number: 201157039 00008 Date: 20/02/2019 00009 */ 00010 00011 #include "main.h" 00012 /** @file main.cpp 00013 * @brief Main file used to run the game using the game engine RosenEngine 00014 */ 00015 00016 00017 //_______________functions______________________________________________________ 00018 int main() 00019 { 00020 // adds testing to the main file if it is declared in macros 00021 #ifdef WITH_TESTING 00022 int number_of_failures = run_all_tests(); 00023 00024 if(number_of_failures > 0) return number_of_failures; 00025 #endif 00026 00027 // initialize game objects and engine 00028 init(); 00029 while(1) { 00030 welcome(); 00031 rosen.reset(); 00032 if(rosen.get_ycursor() == 16) { 00033 while ( pad.check_event(Gamepad::BACK_PRESSED) == false && rosen.dead() == false) { 00034 rosen.read_input(pad); 00035 rosen.update(pad); 00036 render(); 00037 wait(1.0f/fps); 00038 rosen.timer(fps); 00039 } 00040 } 00041 if(rosen.get_ycursor() == 24) { 00042 while ( pad.check_event(Gamepad::BACK_PRESSED) == false) { 00043 rosen.read_input(pad); 00044 rosen.update(pad); 00045 ship_select(); 00046 wait(1.0f/fps); 00047 } 00048 } 00049 if(rosen.get_ycursor() == 32) { 00050 while ( pad.check_event(Gamepad::BACK_PRESSED) == false) { 00051 rosen.read_input(pad); 00052 rosen.update(pad); 00053 display_help(); 00054 wait(1.0f/fps); 00055 } 00056 } 00057 } 00058 } 00059 00060 void init() 00061 { 00062 // need to initialise LCD and Gamepad 00063 lcd.init(); 00064 pad.init(); 00065 rosen.init(9,6,3,42,41); 00066 printf("Initializing...\n"); 00067 00068 } 00069 void render() 00070 { 00071 // clear screen, re-draw and refresh 00072 lcd.clear(); 00073 rosen.draw(lcd, pad); 00074 rosen.get_pos(); 00075 lcd.refresh(); 00076 // printf("Rendering...\n"); 00077 } 00078 void ship_select() 00079 { 00080 lcd.clear(); 00081 rosen.ship_select(lcd); 00082 rosen.get_pos(); 00083 lcd.refresh(); 00084 // printf("Ship Select...\n"); 00085 } 00086 void display_help() 00087 { 00088 lcd.clear(); 00089 rosen.get_pos(); 00090 rosen.help(lcd); 00091 lcd.refresh(); 00092 // printf("Ship Select...\n"); 00093 } 00094 00095 void welcome() 00096 { 00097 while( pad.check_event(Gamepad::START_PRESSED) == false) { 00098 lcd.clear(); 00099 rosen.title(lcd); 00100 rosen.read_input(pad); 00101 lcd.refresh(); 00102 } 00103 // printf("Welcome Function completed...\n"); 00104 }
Generated on Wed Jul 13 2022 17:45:42 by
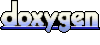