
Ikenna Adrian Ozoemena 201157039
Dependencies: mbed
Weapons.h
00001 #ifndef WEAPONS_H 00002 #define WEAPONS_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 #include "Ship.h" 00008 #include "Enemy.h" 00009 00010 00011 /** Weapon Class 00012 @brief Draws the weapons used by all ships in the game 00013 @author Ozoemena Adrian Ikrnna 00014 @date 8th May 2019 00015 */ 00016 00017 class Weapons 00018 { 00019 public: 00020 /** constructor */ 00021 Weapons(); 00022 /** destructor */ 00023 ~Weapons(); 00024 00025 00026 /** A mutator method that initializes the value of the ship position and ship width 00027 *@param ship_xpos the x position of the ship 00028 *@param ship_ypos the y position of the ship 00029 *@param ship_width the width of the ship 00030 */ 00031 void init(int ship_xpos, int ship_ypos, int ship_width); 00032 /** Draws the appropriate weapon depending on the ship selected 00033 *@param &lcd address of the N5110 library used for the lcd display 00034 *@param &pad address of the gamepad library used to read inputs and send outputs to the gamepad 00035 *@param shipUsed a variable of enum SHIP which contains the ship currently being used 00036 *@param closest the position vector of the nearest enemy 00037 */ 00038 void draw(N5110 &lcd,Gamepad &pad,SHIP shipUsed,Vector2D closest); 00039 /** Accessor method that gets the position of the projectile where applicable(depends on ship) 00040 *@param shipUsed a variable of enum SHIP which contains the ship currently being used 00041 @returns the 2D vector of the projectile fired from ship 00042 */ 00043 Vector2D get_pos(SHIP _shipUsed); 00044 /** Updates the missle position based on the missle velocity 00045 */ 00046 void update(); 00047 /** Mutator function used to set the position of the projectile to a specific coordinate on screen. 00048 *used to reset the projectile after a collision with an enemy 00049 *@param xpos the ships x co-ordinate 00050 *@param ypos the ships y co-ordinate 00051 */ 00052 void set_pos(int xpos, int ypos); 00053 00054 private: 00055 //_______________Private-Variables__________________________________________ 00056 Enemy _enemy; 00057 int _ship_xpos; // ship's x co-ordinate 00058 int _ship_ypos; // ship's y co-ordinate 00059 int reset; // variable to reset the kestrels missle 00060 Vector2D _velocity; // vector to contain the velocity of the kestrels missle 00061 int _x; // missle x co-ordinate 00062 int _y; // missle y co-ordinate 00063 00064 }; 00065 #endif
Generated on Wed Jul 13 2022 17:45:42 by
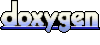