
Ikenna Adrian Ozoemena 201157039
Dependencies: mbed
Ship.h
00001 #ifndef SHIP_H 00002 #define SHIP_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 /** A enum to store the three playable ships*/ 00008 enum SHIP{kestrel, /** The first one */ 00009 imperion, /** The best one */ 00010 orion,}; /** The one for people who can't aim */ 00011 00012 /** Ship Class 00013 @brief Library for drawing and updating the ship used in game 00014 @author Ozoemena Adrian Ikenna 00015 @date 8th May 2019 00016 */ 00017 class Ship 00018 { 00019 00020 public: 00021 /** constructor */ 00022 Ship(); 00023 /** destructor */ 00024 ~Ship(); 00025 /** A mutator method that initializes the value of the ship size, speed and position 00026 *@c(The ships width, height and speed differ from ship to ship) 00027 *@param ship_width the width of the ship 00028 *@param ship_height the height of the ship 00029 *@param ship_speed the speed of the ship 00030 *@param ship_xpos the y position of the ship 00031 *@param ship_ypos the x position of the ship 00032 */ 00033 void init(int ship_width,int ship_height,int ship_speed,int _ship_xpos,int _ship_ypos); 00034 /** Draws the ship based on the ship being used 00035 *@param &lcd address of the N5110 library used for the lcd display 00036 *@param shipUsed a variable of enum SHIP which contains the ship currently being used 00037 */ 00038 void draw_ship(N5110 &lcd, SHIP shipUsed); 00039 /** Mutator method that changes the ships position based on joystick input 00040 *@param x_joystick the xjoystick value 00041 *@param y_joystick the yjoystick value 00042 */ 00043 void update_ship(float x_joystick,float y_joystick); 00044 /** An accesor method used to get the ships position 00045 *@returns the ships position 00046 */ 00047 Vector2D get_pos(); 00048 /** A mutator method set the ships speed and dimensions when ship being used is changed 00049 *@param ship_width the width of the ship 00050 *@param ship_height the height of the ship 00051 *@param ship_speed the speed of the ship 00052 */ 00053 void set_parameters(int ship_width, int ship_height,int ship_speed); 00054 00055 private: 00056 //_______________Private-Variables__________________________________________ 00057 int _ship_width; // the ships width along the x-axis 00058 int _ship_height; // the ships height along the y-axis 00059 int _ship_speed; // the ships speed 00060 int _ship_xpos; // the ships x position 00061 int _ship_ypos; // the ships y position 00062 00063 }; 00064 00065 #endif
Generated on Wed Jul 13 2022 17:45:42 by
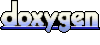