
Ikenna Adrian Ozoemena 201157039
Dependencies: mbed
Menu.h
00001 #ifndef MENU_H 00002 #define MENU_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 #include "Ship.h" 00008 00009 /** Menu Class 00010 @brief Library for displaying menu screen 00011 @author Ozoemena Adrian Ikenna 00012 @date 8th May 2019 00013 */ 00014 class Menu 00015 { 00016 public: 00017 /** constructor */ 00018 Menu(); 00019 /** deconstructor */ 00020 ~Menu(); 00021 00022 /** A mutator method that initializes the cursor used to select options in the menu 00023 *@param ycursor the yposition of the rectangle used as a cursor 00024 */ 00025 void init(int ycursor); 00026 /** Displays the title screen and changes the displayed ship based on ship selected 00027 *@param &lcd address of the N5110 library used for the lcd display 00028 *@param shipUsed a variable of enum SHIP which contains the ship currently being used 00029 */ 00030 void title(N5110 &lcd, SHIP shipUsed); 00031 /** A mutator method that updates the x and y cursor values based on joystick movemnt 00032 *@param d the current direction of the joystick 00033 *@param joystick a 2D vector of the x and y joystick value 00034 */ 00035 void update(Direction d,Vector2D joystick); 00036 /** Accessor method to get tthe current ycursor position used to select options 00037 *from the menu 00038 *@returns the ycursor value 00039 */ 00040 int get_ycursor(); 00041 /** Accessor method to get the current xcursor position used to select a ship 00042 *in the ship section of the menu 00043 *@returns the xcursor value 00044 */ 00045 int get_xcursor(); 00046 /** Displays the ship in the menu home screen 00047 *@param &lcd address of the N5110 library used for the lcd display 00048 */ 00049 void disp_ships(N5110 &lcd); 00050 //void Menu::scroll(N5110 &lcd, Direction d); 00051 00052 private: 00053 //_______________Private-Variables__________________________________________ 00054 int _xcursor; // the x_cursor used in ship select 00055 int _ycursor; // the y cursor used in main menu 00056 00057 00058 }; 00059 #endif
Generated on Wed Jul 13 2022 17:45:42 by
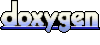