
Ikenna Adrian Ozoemena 201157039
Dependencies: mbed
Health.cpp
00001 #include "Health.h" 00002 Health::Health() 00003 { 00004 00005 } 00006 00007 Health::~Health() 00008 { 00009 00010 } 00011 // ship health bar sprites 00012 const int kestrel_h[45][3] = { 00013 {1,1,1}, 00014 {1,0,1}, 00015 {1,0,1}, 00016 {1,0,1}, 00017 {1,1,1}, 00018 {1,0,1}, 00019 {1,0,1}, 00020 {1,0,1}, 00021 {1,1,1}, 00022 {1,0,1}, 00023 {1,0,1}, 00024 {1,0,1}, 00025 {1,1,1}, 00026 {1,0,1}, 00027 {1,0,1}, 00028 {1,0,1}, 00029 {1,1,1}, 00030 {1,0,1}, 00031 {1,0,1}, 00032 {1,0,1}, 00033 {1,1,1}, 00034 {1,0,1}, 00035 {1,0,1}, 00036 {1,0,1}, 00037 {1,1,1}, 00038 {1,0,1}, 00039 {1,0,1}, 00040 {1,0,1}, 00041 {1,1,1}, 00042 {1,0,1}, 00043 {1,0,1}, 00044 {1,0,1}, 00045 {1,1,1}, 00046 {1,0,1}, 00047 {1,0,1}, 00048 {1,0,1}, 00049 {1,1,1}, 00050 {1,0,1}, 00051 {1,0,1}, 00052 {1,0,1}, 00053 {1,1,1}, 00054 {1,0,1}, 00055 {1,0,1}, 00056 {1,0,1}, 00057 {1,1,1}, 00058 }; 00059 00060 const int imperion_h[45][3] = { 00061 {1,1,1}, 00062 {1,0,1}, 00063 {1,0,1}, 00064 {1,0,1}, 00065 {1,0,1}, 00066 {1,0,1}, 00067 {1,1,1}, 00068 {1,0,1}, 00069 {1,0,1}, 00070 {1,0,1}, 00071 {1,0,1}, 00072 {1,0,1}, 00073 {1,1,1}, 00074 {1,0,1}, 00075 {1,0,1}, 00076 {1,0,1}, 00077 {1,0,1}, 00078 {1,0,1}, 00079 {1,1,1}, 00080 {1,0,1}, 00081 {1,0,1}, 00082 {1,0,1}, 00083 {1,0,1}, 00084 {1,0,1}, 00085 {1,1,1}, 00086 {1,0,1}, 00087 {1,0,1}, 00088 {1,0,1}, 00089 {1,0,1}, 00090 {1,0,1}, 00091 {1,1,1}, 00092 {1,0,1}, 00093 {1,0,1}, 00094 {1,0,1}, 00095 {1,0,1}, 00096 {1,0,1}, 00097 {1,0,1}, 00098 {1,1,1}, 00099 {1,0,1}, 00100 {1,0,1}, 00101 {1,0,1}, 00102 {1,0,1}, 00103 {1,0,1}, 00104 {1,0,1}, 00105 {1,1,1}, 00106 00107 }; 00108 const int orion_h[45][3] = { 00109 {1,1,1}, 00110 {1,0,1}, 00111 {1,0,1}, 00112 {1,0,1}, 00113 {1,0,1}, 00114 {1,0,1}, 00115 {1,0,1}, 00116 {1,0,1}, 00117 {1,0,1}, 00118 {1,0,1}, 00119 {1,0,1}, 00120 {1,0,1}, 00121 {1,0,1}, 00122 {1,0,1}, 00123 {1,0,1}, 00124 {1,0,1}, 00125 {1,0,1}, 00126 {1,0,1}, 00127 {1,0,1}, 00128 {1,0,1}, 00129 {1,0,1}, 00130 {1,0,1}, 00131 {1,1,1}, 00132 {1,0,1}, 00133 {1,0,1}, 00134 {1,0,1}, 00135 {1,0,1}, 00136 {1,0,1}, 00137 {1,0,1}, 00138 {1,0,1}, 00139 {1,0,1}, 00140 {1,0,1}, 00141 {1,0,1}, 00142 {1,0,1}, 00143 {1,0,1}, 00144 {1,0,1}, 00145 {1,0,1}, 00146 {1,0,1}, 00147 {1,0,1}, 00148 {1,0,1}, 00149 {1,0,1}, 00150 {1,0,1}, 00151 {1,0,1}, 00152 {1,0,1}, 00153 {1,1,1}, 00154 }; 00155 // Note when only refering to health or shields functions will be named after them, 00156 // if a function affects both it will be named with HP 00157 void Health::init(SHIP shipUsed) 00158 { 00159 // assign health and shield values based on the ship 00160 switch (shipUsed) { 00161 case kestrel: 00162 _ship_shields = 45; 00163 _ship_health = 11; 00164 _health_unit = 4; 00165 break; 00166 case imperion: 00167 _ship_shields = 30; 00168 _ship_health = 6; 00169 _health_unit = 7; 00170 break; 00171 case orion: 00172 _ship_shields = 70; 00173 _ship_health = 2; 00174 _health_unit = 22; 00175 break; 00176 } 00177 _seekerH[0] = 10; 00178 _seekerH[1] = 10; 00179 _seekerH[2] = 10; 00180 _shooterH[0] = 30; 00181 _shooterH[1] = 30; 00182 _shooterH[2] = 30; 00183 } 00184 void Health::draw_health(N5110 &lcd,SHIP shipUsed) 00185 { 00186 // Draw shield and sealth bars based on ship 00187 switch (shipUsed) { 00188 case kestrel: 00189 lcd.drawSprite(81,2,45,3,(int *)kestrel_h); 00190 lcd.drawLine(82,45,82,46 - (_ship_health * _health_unit),FILL_BLACK); 00191 break; 00192 case imperion: 00193 lcd.drawSprite(81,2,45,3,(int *)imperion_h); 00194 lcd.drawLine(82,45,82,46 - (_ship_health * _health_unit),FILL_BLACK); 00195 break; 00196 case orion: 00197 lcd.drawSprite(81,2,45,3,(int *)orion_h); 00198 lcd.drawLine(82,45,82,46 - (_ship_health * _health_unit),FILL_BLACK); 00199 break; 00200 } 00201 00202 } 00203 void Health::draw_shields(N5110 &lcd) 00204 { 00205 // draw the sheields as a line next to the health bar 00206 lcd.drawRect(79,2,1,_ship_shields,FILL_BLACK); 00207 } 00208 void Health::update(int bars,Gamepad &pad) 00209 { 00210 // only take damage in shields if shields are up 00211 if(_ship_shields > 0) { 00212 _ship_shields = _ship_shields - (bars * 10); 00213 // dont let ship shields drop below zero 00214 if(_ship_shields < 0) { 00215 _ship_shields = 0; 00216 pad.tone(100,0.1); // add noise to indicate if shields are brocken 00217 wait(0.1); 00218 } 00219 } else { 00220 // once shields are down start losing health 00221 _ship_health = _ship_health - bars; 00222 if(_ship_health < 0) { 00223 _ship_health = 0; 00224 } 00225 } 00226 } 00227 void Health::seekerh_update(int seno,int dmg) 00228 { 00229 // seno determines what seeker took damage 00230 switch (seno) { 00231 case 1: 00232 _seekerH[0] = _seekerH[0] - dmg; 00233 break; 00234 case 2: 00235 _seekerH[1] = _seekerH[1] - dmg; 00236 break; 00237 case 3: 00238 _seekerH[2] = _seekerH[2] - dmg; 00239 break; 00240 } 00241 // dont let seeker health fall below zero 00242 if(_seekerH[0] < 0) { 00243 _seekerH[0] = 0; 00244 } 00245 if(_seekerH[1] < 0) { 00246 _seekerH[1] = 0; 00247 } 00248 if(_seekerH[2] < 0) { 00249 _seekerH[2] = 0; 00250 } 00251 } 00252 void Health::shooterh_update(int shno, int dmg) 00253 { 00254 // shno determines what shooter took damage 00255 switch (shno) { 00256 case 1: 00257 _shooterH[0] = _shooterH[0] - dmg; 00258 break; 00259 case 2: 00260 _shooterH[1] = _shooterH[1] - dmg; 00261 break; 00262 case 3: 00263 _shooterH[2] = _shooterH[2] - dmg;; 00264 break; 00265 } 00266 // dont let shooter health fall below zero 00267 if(_shooterH[0] < 0) { 00268 _shooterH[0] = 0; 00269 } 00270 if(_shooterH[1] < 0) { 00271 _shooterH[1]= 0; 00272 } 00273 if(_shooterH[2] < 0) { 00274 _shooterH[2] = 0; 00275 } 00276 } 00277 Vector2D Health::get_hp() 00278 { 00279 return{_ship_health, _ship_shields}; 00280 } 00281 int Health::get_seekerh(int seno) 00282 { 00283 // return appropriate health based on seeker number 00284 if(seno == 1) { 00285 return _seekerH[0]; 00286 } 00287 if(seno == 2) { 00288 return _seekerH[1]; 00289 } 00290 if(seno == 3) { 00291 return _seekerH[2]; 00292 } 00293 } 00294 int Health::get_shooterh(int shno) 00295 { 00296 // return appropriate health based on shooter number 00297 if(shno == 1) { 00298 return _shooterH[0]; 00299 } 00300 if(shno == 2) { 00301 return _shooterH[1]; 00302 } 00303 if(shno == 3) { 00304 return _shooterH[2]; 00305 } 00306 } 00307 void Health::reset_seekerh(int seno) 00308 { 00309 // reset any seeker health to default based on seeker number 00310 if(seno == 1) { 00311 _seekerH[0] = 10; 00312 } 00313 if(seno == 2) { 00314 _seekerH[1] = 10; 00315 } 00316 if(seno == 3) { 00317 _seekerH[2] = 10; 00318 } 00319 } 00320 void Health::reset_shooterh(int shno) 00321 { 00322 // reset any shooter health to default based on shooter number 00323 if(shno == 1) { 00324 _shooterH[0] = 30; 00325 } 00326 if(shno == 2) { 00327 _shooterH[1] = 30; 00328 } 00329 if(shno == 3) { 00330 _shooterH[2] = 30; 00331 } 00332 } 00333
Generated on Wed Jul 13 2022 17:45:42 by
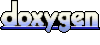