
Control a solenoid using a power MOSFET circuit
Dependencies: mbed
main.cpp
00001 #include "mbed.h" 00002 //Solenoid Hello World 00003 DigitalOut myled(LED1); 00004 //Non blocking with auto off delay using timer interrupt setup by class 00005 class Solenoid 00006 { 00007 public: 00008 Solenoid (PinName pin, float delay=0.5); 00009 void write(bool state); 00010 Solenoid& operator= (bool value); 00011 00012 private: 00013 void Solenoid_Off_Int(); 00014 DigitalOut _pin; 00015 Timeout tint; 00016 float ontime; 00017 }; 00018 Solenoid::Solenoid(PinName pin, float delay) : _pin(pin), ontime(delay) 00019 { 00020 _pin=0; 00021 } 00022 void Solenoid::Solenoid_Off_Int() 00023 { 00024 _pin=0;//timer interrupt routine to auto turn off solenoid 00025 } 00026 void Solenoid::write(bool value) 00027 { 00028 _pin = value; 00029 if (value!=0) //do auto off with timer interrupt 00030 tint.attach(this,&Solenoid::Solenoid_Off_Int,ontime);//setup a timer interrupt 00031 } 00032 Solenoid& Solenoid::operator= (bool value) 00033 { 00034 write(value); 00035 return *this; 00036 } 00037 00038 Solenoid mySolenoid(p21); 00039 int main() 00040 { 00041 while(1) { 00042 mySolenoid = 1; //ON with timer auto off 00043 myled = 1; 00044 wait(.5); //just for LEDs - solenoid turns off with timer interrupt 00045 myled = 0; 00046 wait(2.0); 00047 } 00048 }
Generated on Tue Jul 19 2022 20:38:05 by
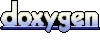