
Lab3_Gui code
Dependencies: mbed
mbed_beat_new.py
00001 #*****************************************************************# 00002 # # 00003 # File: beatfactorygui.py # 00004 # Author: Ethan Takla # 00005 # Date Created: 10/6/14 # 00006 # Description: A python GUI for graphically creating songs on # 00007 # the FDRM-KL46z # 00008 # # 00009 #*****************************************************************# 00010 # Last edit: Matthew Sessions 00011 00012 #System Imports 00013 import sys 00014 00015 #Serial Imports 00016 import serial 00017 from serial.tools import list_ports 00018 00019 #Signal Imports 00020 import signal 00021 00022 #Sound Imports 00023 00024 # Uncomment for sound 00025 #import winsound 00026 00027 #Threading imports 00028 import thread 00029 00030 #PyQt Imports 00031 from PyQt4.QtGui import QApplication, QDialog, QMainWindow, QFileDialog 00032 from PyQt4 import QtCore, QtGui 00033 00034 00035 try: 00036 _fromUtf8 = QtCore.QString.fromUtf8 00037 except AttributeError: 00038 def _fromUtf8(s): 00039 return s 00040 00041 #Setup text encoding 00042 try: 00043 _encoding = QtGui.QApplication.UnicodeUTF8 00044 def _translate(context, text, disambig): 00045 return QtGui.QApplication.translate(context, text, disambig, _encoding) 00046 except AttributeError: 00047 def _translate(context, text, disambig): 00048 return QtGui.QApplication.translate(context, text, disambig) 00049 00050 #Create Note Classs 00051 class Note: 00052 def __init__( self, note ): 00053 self.note = note 00054 00055 #Main Window Class 00056 class Ui_MainWindow(QtGui.QMainWindow): 00057 00058 def initializeUI(self, MainWindow): 00059 00060 #Initialize main window properties 00061 MainWindow.setObjectName(_fromUtf8("FDRM-KL46z Beat Factory")) 00062 MainWindow.resize(1200, 848) 00063 MainWindow.setMaximumSize(QtCore.QSize(16777215, 848)) 00064 00065 #Create central widget 00066 self.centralwidget = QtGui.QWidget(MainWindow) 00067 self.centralwidget.setObjectName(_fromUtf8("centralwidget")) 00068 00069 #Create gridLayout_2 00070 self.gridLayout_2 = QtGui.QGridLayout(self.centralwidget) 00071 self.gridLayout_2.setObjectName(_fromUtf8("gridLayout_2")) 00072 00073 #Create and format a graphics view widget 00074 self.graphicsView = QtGui.QGraphicsView(self.centralwidget) 00075 self.graphicsView.setMinimumSize(QtCore.QSize(300, 120)) 00076 self.graphicsView.setMaximumSize(QtCore.QSize(300, 120)) 00077 self.graphicsView.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) 00078 self.graphicsView.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) 00079 00080 #Set the graphics view image 00081 self.scene = QtGui.QGraphicsScene(); 00082 self.pixmap = QtGui.QPixmap( "TekBots.png" ) 00083 self.scene.addPixmap(self.pixmap) 00084 self.graphicsView.setScene( self.scene ) 00085 self.graphicsView.setObjectName(_fromUtf8("graphicsView")) 00086 00087 #Add the graphics view to gridLayout_2 00088 self.gridLayout_2.addWidget(self.graphicsView, 0, 1, 1, 1) 00089 00090 #Create and format verticalLayout_2 00091 self.verticalLayout_2 = QtGui.QVBoxLayout() 00092 self.verticalLayout_2.setSpacing(7) 00093 self.verticalLayout_2.setSizeConstraint(QtGui.QLayout.SetNoConstraint) 00094 self.verticalLayout_2.setContentsMargins(-1, -1, -1, 0) 00095 self.verticalLayout_2.setObjectName(_fromUtf8("verticalLayout_2")) 00096 00097 #Create a table view for beat editing 00098 self.tableView = QtGui.QTableWidget(self.centralwidget) 00099 00100 #Add the table view to verticalLayout2 00101 self.verticalLayout_2.addWidget(self.tableView) 00102 00103 #Add verticalLayout2 to gridLayout2 00104 self.gridLayout_2.addLayout(self.verticalLayout_2, 0, 0, 7, 1) 00105 00106 #Create gridLayout 00107 self.gridLayout = QtGui.QGridLayout() 00108 self.gridLayout.setObjectName(_fromUtf8("gridLayout")) 00109 00110 #Create and format pushButton, add them to gridLayout 00111 self.pushButton = QtGui.QPushButton(self.centralwidget) 00112 self.pushButton.setObjectName(_fromUtf8("pushButton")) 00113 self.pushButton.setStyleSheet("color: black; background-color: red;") 00114 self.gridLayout.addWidget(self.pushButton, 0, 2, 1, 1) 00115 self.pushButton_2 = QtGui.QPushButton(self.centralwidget) 00116 self.pushButton_2.setObjectName(_fromUtf8("pushButton_2")) 00117 self.pushButton_2.setEnabled( False ) 00118 self.gridLayout.addWidget(self.pushButton_2, 3, 0, 1, 1) 00119 self.pushButton_3 = QtGui.QPushButton(self.centralwidget) 00120 self.pushButton_3.setObjectName(_fromUtf8("pushButton_3")) 00121 self.pushButton_3.setEnabled( False ) 00122 self.gridLayout.addWidget(self.pushButton_3, 3, 1, 1, 1) 00123 00124 #Create labels and add them to gridLayout 00125 self.label = QtGui.QLabel(self.centralwidget) 00126 self.label.setObjectName(_fromUtf8("label")) 00127 self.gridLayout.addWidget(self.label, 0, 0, 1, 1) 00128 self.label2 = QtGui.QLabel(self.centralwidget) 00129 self.label2.setObjectName(_fromUtf8("label2")) 00130 self.gridLayout.addWidget(self.label2, 1, 0, 1, 1) 00131 self.label3 = QtGui.QLabel(self.centralwidget) 00132 self.label3.setObjectName(_fromUtf8("label3")) 00133 self.gridLayout.addWidget(self.label3, 2, 0, 1, 1) 00134 00135 #Create comboBoxs and add them to gridLayout 00136 self.comboBox = QtGui.QComboBox(self.centralwidget) 00137 self.comboBox.setObjectName(_fromUtf8("comboBox")) 00138 self.gridLayout.addWidget(self.comboBox, 0, 1, 1, 1) 00139 self.comboBox2 = QtGui.QComboBox(self.centralwidget) 00140 self.comboBox2.setObjectName(_fromUtf8("comboBox2")) 00141 self.gridLayout.addWidget(self.comboBox2, 1, 1, 1, 1) 00142 self.comboBox3 = QtGui.QComboBox(self.centralwidget) 00143 self.comboBox3.setObjectName(_fromUtf8("comboBox3")) 00144 self.gridLayout.addWidget(self.comboBox3, 2, 1, 1, 1) 00145 00146 #Add gridLayout to gridLayout_2 and format 00147 self.gridLayout_2.addLayout(self.gridLayout, 1, 1, 1, 1) 00148 MainWindow.setCentralWidget(self.centralwidget) 00149 00150 #Create and configure the menu bar 00151 self.menubar = QtGui.QMenuBar(MainWindow) 00152 self.menubar.setGeometry(QtCore.QRect(0, 0, 972, 26)) 00153 self.menubar.setObjectName(_fromUtf8("menubar")) 00154 self.menuFile = QtGui.QMenu(self.menubar) 00155 self.menuFile.setObjectName(_fromUtf8("menuFile")) 00156 self.menuHelp = QtGui.QMenu(self.menubar) 00157 self.menuHelp.setObjectName(_fromUtf8("menuHelp")) 00158 MainWindow.setMenuBar(self.menubar) 00159 self.statusbar = QtGui.QStatusBar(MainWindow) 00160 self.statusbar.setObjectName(_fromUtf8("statusbar")) 00161 MainWindow.setStatusBar(self.statusbar) 00162 00163 #Create a QAction for the Load and Save songs, and About button 00164 self.actionLoad_Song = QtGui.QAction(MainWindow) 00165 self.actionLoad_Song.setObjectName(_fromUtf8("actionLoad_Song")) 00166 self.actionSave_Song = QtGui.QAction(MainWindow) 00167 self.actionSave_Song.setObjectName(_fromUtf8("actionSave_Song")) 00168 self.actionAbout = QtGui.QAction(MainWindow) 00169 self.actionAbout.setObjectName(_fromUtf8("actionAbout")) 00170 self.menuFile.addAction(self.actionLoad_Song) 00171 self.menuFile.addAction(self.actionSave_Song) 00172 self.menuHelp.addAction(self.actionAbout) 00173 self.menubar.addAction(self.menuFile.menuAction()) 00174 self.menubar.addAction(self.menuHelp.menuAction()) 00175 00176 #Set text of all UI elements 00177 self.retranslateUI(MainWindow) 00178 00179 #Connect Slots 00180 QtCore.QMetaObject.connectSlotsByName(MainWindow) 00181 00182 #Populate comboBox3 and configure tempo 00183 self.comboBox3.clear() 00184 self.comboBox3.addItems(self.tempoValues) 00185 self.comboBox3.setCurrentIndex( 14 ) 00186 self.tempo = 120 00187 00188 #Populate Combobox 2 00189 self.comboBox2.clear() 00190 self.comboBox2.addItems(self.noteNumberValues) 00191 00192 def retranslateUI(self, MainWindow): 00193 00194 #Set the text of the UI elements 00195 MainWindow.setWindowTitle(_translate("FDRM-KL46z Beat Factory", "FDRM-KL46z Beat Factory", None)) 00196 self.pushButton.setText(_translate("FDRM-KL46z Beat Factory", "Scan", None)) 00197 self.pushButton_2.setText(_translate("FDRM-KL46z Beat Factory", "Play", None)) 00198 self.pushButton_3.setText(_translate("FDRM-KL46z Beat Factory", "Clear", None)) 00199 self.label.setText(_translate("FDRM-KL46z Beat Factory", "COM Port:", None)) 00200 self.label2.setText(_translate("FDRM-KL46z Beat Factory", "Number of Notes:", None)) 00201 self.label3.setText(_translate("FDRM-KL46z Beat Factory", "Tempo:", None)) 00202 self.menuFile.setTitle(_translate("FDRM-KL46z Beat Factory", "File", None)) 00203 self.menuHelp.setTitle(_translate("FDRM-KL46z Beat Factory", "Help", None)) 00204 self.actionLoad_Song.setText(_translate("FDRM-KL46z Beat Factory", "Load Song", None)) 00205 self.actionSave_Song.setText(_translate("FDRM-KL46z Beat Factory", "Save Song", None)) 00206 self.actionAbout.setText(_translate("FDRM-KL46z Beat Factory", "About", None)) 00207 00208 def initializeVariables( self ): 00209 00210 #Define cell selected/unselected variables 00211 self.cellSelected = 1 00212 self.cellUnselected = 0 00213 00214 #Set initial comm. state 00215 self.comButtonState = "Scan" 00216 00217 #Create the song array 00218 self.song_array = [] # delete all note elements 00219 00220 #Set table row and column count variables 00221 self.tableViewRowCount = 24 00222 self.tableViewColumnCount = 16 00223 self.selectedCells = [[0 for x in xrange(self.tableViewColumnCount)] for x in xrange(self.tableViewRowCount)] 00224 self.noteFrequencies = [ 831, 784, 740, 698, 659, 622, 587, 554, 523, 494, 466, 440, 415, 392, 370, 349, 330, 311, 294, 277, 261, 247, 233, 220 ] 00225 00226 #Define tempo values 00227 self.tempoValues = [ "50", "55", "60", "65", "70", "75", "80", "85", "90", "95", "100", "105", "110", "115", "120", "125", "130", 00228 "135", "140", "145", "150", "155", "160", "165", "170", "175", "180", "185", "190", "195", "200", "205", 00229 "210", "215", "220", "225", "230", "235", "240"] 00230 00231 #Define note number values 00232 self.noteNumberValues = [ "16", "32", "48", "64" ] 00233 00234 #Define serial ports that are to be 'detected' 00235 self.ports = [ "Select", 00236 'COM1', 'COM2', 'COM3', 'COM4', 'COM5', 'COM6', 'COM7', 'COM8', 'COM9', 'COM10', 00237 'COM11', 'COM12', 'COM13', 'COM14', 'COM15', 'COM16', 'COM17', 'COM18', 00238 'COM19', 'COM20', 'COM21', '/dev/ttyACM0', '/dev/ttyACM1', '/dev/ttyACM2', '/dev/ttyACM3'] 00239 00240 00241 00242 def initializeTable( self ): 00243 00244 #Initialize beat table columns and rows 00245 self.tableView.setColumnCount(self.tableViewColumnCount) 00246 self.tableView.setRowCount(self.tableViewRowCount) 00247 00248 #Initialize beat table rows for notes 00249 self.verticalHeaderLabels = [ "G#", "G", "F#", "F", "E", "D#", "D", "C#", "C(High)", "B", "A#", "A", "G#", "G", "F#", "F", "E", "D#", "D", "C#", "C (Middle)", "B", "A#", "A" ] 00250 00251 #Label vertical headers on table 00252 self.tableView.setVerticalHeaderLabels( self.verticalHeaderLabels ) 00253 00254 #Make table ready only by creating items widgets in each cell and disabling them 00255 for i in xrange(self.tableView.rowCount()): 00256 for j in xrange(self.tableView.columnCount()): 00257 self.tableView.setItem(i,j, QtGui.QTableWidgetItem()) 00258 self.tableView.item(i,j).setFlags(QtCore.Qt.ItemIsEnabled) 00259 00260 def initializeSerial( self ): 00261 00262 #Configure the serial port for 8-N-1, baudrate doesn't matter since the FDRM-KL46z is running a VCP 00263 self.ser = serial.Serial() 00264 self.ser.timeout = 1000 00265 self.ser.baudrate = 9600 00266 00267 def highlightColumn( self, column ): 00268 00269 #Go through the unselected cells in each row and highlight them 00270 for i in xrange(self.tableView.rowCount()): 00271 if self.selectedCells[i][column] == self.cellUnselected: 00272 self.tableView.item(i, column).setBackground(QtGui.QColor(255,179,0)) 00273 00274 def unhighlightColumn( self, column ): 00275 00276 #Go through the unselected cells in each row and unhighlight them 00277 for i in xrange(self.tableView.rowCount()): 00278 if self.selectedCells[i][column] == self.cellUnselected: 00279 self.tableView.item(i, column).setBackground(QtGui.QColor(255,255,255)) 00280 00281 def handlePlayButton( self ): 00282 00283 #Disable play and clear buttons while song is playing 00284 self.pushButton_2.setEnabled( False ) 00285 self.pushButton_3.setEnabled( False ) 00286 00287 #Empty song array 00288 del self.song_array[:] 00289 emptyCells = 0 00290 00291 00292 for j in xrange(self.tableView.columnCount()): 00293 for i in xrange(self.tableView.rowCount()): 00294 00295 #If a cell is selected, add the corresponding note to the song array 00296 if self.selectedCells[i][j] == self.cellSelected: 00297 00298 #Store the note index 00299 note_name = i # note name is letter A-G 00300 00301 # create Note class and populate 00302 note = Note( note_name ) 00303 self.song_array.append( note ) # add Note to array of Notes 00304 else: 00305 emptyCells += 1 00306 00307 #If an empty column is found, add a rest (index 24) to the song array 00308 if emptyCells == self.tableView.rowCount(): 00309 00310 # store user-inputted note into a Note class 00311 note_name = 24 # note name is letter A-G 00312 00313 # create Note class and populate 00314 note = Note( note_name ) 00315 self.song_array.append( note ) # add Note to array of Notes 00316 00317 emptyCells = 0 00318 00319 00320 try: 00321 self.ser.open() 00322 except: 00323 self.serialScan() 00324 return 00325 self.ser.write( "$NEW\n" ) #Send new song packet 00326 self.ser.write( "$T" ) #Send Tempo Packet 00327 self.ser.write( "%d" % (self.tempo*4) ) 00328 self.ser.write( '\n' ) 00329 self.ser.write( "$L" ) #Send song length packet 00330 self.ser.write( "%d" % (self.tableView.columnCount() ) ) 00331 self.ser.write( '\n' ) 00332 for i in range(self.tableView.columnCount()): 00333 self.ser.write( "$S" ) #Send note data packet 00334 self.ser.write( "%d" % (self.song_array[i].note) ) 00335 self.ser.write( '\n' ) 00336 self.ser.write( "$PLAY\n" ) #Send play song packet 00337 00338 data = 0 00339 00340 while 1: 00341 00342 #Wait for serial data to be available 00343 if self.ser.inWaiting() != 0: 00344 00345 #Read a line of data 00346 data = int(self.ser.readline()) 00347 00348 #Exit the while loop if a termination character (0xFF) is received 00349 if data == 255: 00350 break; 00351 00352 #Highlight the column specified by the data 00353 self.highlightColumn( data ) 00354 00355 #Scroll to the column so we can see it 00356 self.tableView.scrollToItem( self.tableView.item(0,data) ) 00357 00358 #Unhighlight previous column 00359 if data > 0: 00360 self.unhighlightColumn( data - 1 ) 00361 00362 #Force a GUI uptdate 00363 QApplication.processEvents() 00364 00365 self.unhighlightColumn( self.tableView.columnCount()-1 ) 00366 self.ser.close() 00367 00368 #Enable play and clear buttons after song is song playing 00369 self.pushButton_2.setEnabled( True ) 00370 self.pushButton_3.setEnabled( True ) 00371 00372 def handleClearButton( self ): 00373 00374 #Go through each cell, make it white, and set it as unselected 00375 for i in xrange(self.tableView.rowCount()): 00376 for j in xrange(self.tableView.columnCount()): 00377 self.tableView.item(i, j).setBackground(QtGui.QColor(255,255,255)) 00378 self.selectedCells[i][j] = self.cellUnselected 00379 00380 def serialScan( self ): 00381 00382 #Populate the combobox with ports 00383 self.comboBox.clear() 00384 self.comboBox.addItems( self.ports ) 00385 00386 #Go through each port name to see if it exists 00387 for i in self.ports[1:]: 00388 self.ser.port = i 00389 try: 00390 self.ser.open() 00391 except Exception, e: 00392 00393 #If the port name does not exist, remove it from the combobox 00394 remove_port = self.comboBox.findText( self.ser.port ) 00395 self.comboBox.removeItem( remove_port ) 00396 self.ser.close() 00397 00398 def handleComButton( self ): 00399 00400 #If the comm. button is is Scan mode, scan the serial ports 00401 if self.comButtonState == "Scan": 00402 00403 self.serialScan() 00404 00405 if self.comboBox.count() > 1: 00406 00407 #If we have detected a serial port, change the button name to "connect" and change color 00408 self.pushButton.setText(_translate("MainWindow", "Connect", None)) 00409 self.pushButton.setStyleSheet("color: black; background-color: yellow;") 00410 self.comButtonState = "Connect" 00411 00412 #Enable the play and clear buttons 00413 self.pushButton_2.setEnabled( True ) 00414 self.pushButton_3.setEnabled( True ) 00415 else: 00416 00417 #Create a message box if there was no COM port detected 00418 QtGui.QMessageBox.information(self, 'Error',"A serial device was not detected, please ensure that it is plugged into your computer.") 00419 00420 elif self.comButtonState == "Connect": 00421 00422 #Create a new port 00423 self.ser.close() 00424 new_port = str(self.comboBox.currentText()) 00425 self.ser.port = new_port 00426 00427 try: 00428 if self.comboBox.currentText() != "Select": 00429 self.ser.open() 00430 else: 00431 QtGui.QMessageBox.information(self, 'Error',"Please Select a COM port.") 00432 00433 except Exception ,e: 00434 00435 #If we can't connect, reset the button state to "scan" 00436 self.pushButton.setText(_translate("MainWindow", "Disconnect", None)) 00437 self.pushButton.setStyleSheet("color: black; background-color: red;") 00438 self.comButtonState = "Scan" 00439 if self.ser.isOpen(): 00440 00441 #If we can open the serial port, change the button name to "disconnect" and change color 00442 self.pushButton.setText(_translate("MainWindow", "Disconnect", None)) 00443 self.pushButton.setStyleSheet("color: black; background-color: green;") 00444 self.comButtonState = "Disconnect" 00445 self.ser.close() 00446 00447 elif self.comButtonState == "Disconnect": 00448 00449 #Change the button text to "Scan" once disconnect it pressed, and change color 00450 self.pushButton.setText(_translate("MainWindow", "Scan", None)) 00451 self.pushButton.setStyleSheet("color: black; background-color: red;") 00452 self.comButtonState = "Scan" 00453 00454 #Disable play and clear buttons 00455 self.pushButton_2.setEnabled( False ) 00456 self.pushButton_3.setEnabled( False ) 00457 00458 def beepThread( self, tone ): 00459 print "No sound" 00460 #Produce a tone using winsound 00461 00462 # Uncomment below for sound 00463 #winsound.Beep(tone, 150) 00464 00465 def handleCellClicked(self, row, column): 00466 00467 self.noteExists = False 00468 00469 #Check to see if any notes are already selected in the row 00470 for i in xrange(self.tableView.rowCount()): 00471 if self.selectedCells[i][column] == self.cellSelected: 00472 self.noteExists = True 00473 00474 00475 #If a cell is clicked and unselected, change it's color, mark it as selected, and start the tone thread 00476 if self.selectedCells[row][column] == self.cellUnselected: 00477 00478 #Do not select cell if a note already exists in the column 00479 if self.noteExists == False: 00480 00481 self.tableView.item(row, column).setBackground(QtGui.QColor(100,100,150)) 00482 self.selectedCells[row][column] = self.cellSelected 00483 thread.start_new_thread(self.beepThread, (self.noteFrequencies[row],)) 00484 00485 #If a cell is clicked and selected, change it's color and mark it as unselected 00486 else: 00487 self.tableView.item(row, column).setBackground(QtGui.QColor(255,255,255)) 00488 self.selectedCells[row][column] = self.cellUnselected 00489 00490 def handleSaveSongButton( self ): 00491 00492 #Create a save file dialog 00493 self.fileName = QFileDialog.getSaveFileName(self,"Save File", 00494 "", 00495 "FDRM-KL46z Song (*.song)"); 00496 try: 00497 #Create a file with the filename provided from the user 00498 self.f = open(self.fileName, 'w') 00499 00500 #Write the note number index 00501 self.f.write( str(self.comboBox2.currentIndex()) + '\n' ) 00502 00503 #Write each note as a coordinate on a newline 00504 for i in xrange(self.tableView.rowCount()): 00505 for j in xrange(self.tableView.columnCount()): 00506 if self.selectedCells[i][j] == self.cellSelected: 00507 self.f.write( str(i)+ ',' + str(j) + '\n') 00508 00509 #Write the end of song marker 00510 self.f.write( "end song") 00511 self.f.close() 00512 00513 except Exception ,e: 00514 print(e) 00515 00516 def handleLoadSongButton( self ): 00517 00518 #Create and open file dialog 00519 self.fileName = QFileDialog.getOpenFileName(self,"Open File", 00520 "", 00521 "FDRM-KL46z Song (*.song)"); 00522 00523 try: 00524 #Open the user defined file for reading 00525 self.f = open(self.fileName, 'r') 00526 00527 #cleat all notes from tableview 00528 self.handleClearButton(); 00529 00530 #Read index number and change the note number combo box 00531 index = int(self.f.readline()) 00532 self.handleNoteNumberChange(index) 00533 self.comboBox2.setCurrentIndex(index) 00534 00535 #Read the first cell location 00536 self.cellID = self.f.readline() 00537 00538 #Continue reading cell ID's and activate corresponding cells 00539 while self.cellID != "end song": 00540 i = 0 00541 columnString = "" 00542 rowString = "" 00543 00544 #Wait until we get a comma 00545 while self.cellID[i] != ',': 00546 rowString += self.cellID[i] 00547 i += 1 00548 00549 #Go to the next data point after a newline character 00550 while self.cellID[i+1] != '\n': 00551 columnString += self.cellID[i+1] 00552 i += 1 00553 00554 #Update the table view 00555 self.tableView.item(int(rowString), int(columnString)).setBackground(QtGui.QColor(100,100,150)) 00556 self.selectedCells[int(rowString)][int(columnString)] = self.cellSelected 00557 self.cellID = self.f.readline() 00558 except Exception ,e: 00559 print(e) 00560 00561 def handleNoteNumberChange( self, index ): 00562 00563 #Change the number of columns in the table if the song length changes 00564 self.tableViewColumnCount = int(self.noteNumberValues[int(index)]) 00565 self.selectedCells = [[0 for x in xrange(self.tableViewColumnCount)] for x in xrange(self.tableViewRowCount)] 00566 00567 #Reset the table 00568 self.initializeTable() 00569 self.handleClearButton() 00570 00571 def handleTempoChange( self, index ): 00572 00573 #Change necesarry variables if the tempo is chaged 00574 self.tempo = int(self.tempoValues[int(index)]) 00575 00576 def handleAboutButton( self ): 00577 00578 #Opens the about form if the about button is pressed 00579 self.dialog = QtGui.QDialog(self) 00580 self.dialog.ui = aboutForm() 00581 self.dialog.ui.setupUi(self.dialog) 00582 self.dialog.setAttribute(QtCore.Qt.WA_DeleteOnClose) 00583 self.dialog.show() 00584 00585 00586 def bind_events( self ): 00587 #Bind events to handler functions 00588 self.pushButton.clicked.connect( self.handleComButton ) 00589 self.tableView.cellClicked.connect( self.handleCellClicked ) 00590 self.pushButton_3.clicked.connect( self.handleClearButton ) 00591 self.actionSave_Song.triggered.connect( self.handleSaveSongButton ) 00592 self.actionLoad_Song.triggered.connect( self.handleLoadSongButton ) 00593 self.pushButton_2.clicked.connect( self.handlePlayButton ) 00594 self.comboBox2.currentIndexChanged.connect( self.handleNoteNumberChange ) 00595 self.comboBox3.currentIndexChanged.connect( self.handleTempoChange ) 00596 self.actionAbout.triggered.connect( self.handleAboutButton ) 00597 00598 class aboutForm(object): 00599 00600 def setupUi(self, Form): 00601 00602 #Configure the form 00603 Form.setObjectName(_fromUtf8("Form")) 00604 Form.resize(381, 233) 00605 Form.setMinimumSize(QtCore.QSize(381, 233)) 00606 Form.setMaximumSize(QtCore.QSize(381, 233)) 00607 00608 #Create label 00609 self.label = QtGui.QLabel(Form) 00610 self.label.setGeometry(QtCore.QRect(140, 160, 121, 16)) 00611 self.label.setObjectName(_fromUtf8("label")) 00612 00613 #Create label_2 00614 self.label_2 = QtGui.QLabel(Form) 00615 self.label_2.setGeometry(QtCore.QRect(140, 180, 121, 16)) 00616 self.label_2.setObjectName(_fromUtf8("label_2")) 00617 00618 #Create label_3 00619 self.label_3 = QtGui.QLabel(Form) 00620 self.label_3.setGeometry(QtCore.QRect(160, 200, 121, 16)) 00621 self.label_3.setObjectName(_fromUtf8("label_3")) 00622 00623 #Create a graphics view 00624 self.graphicsView = QtGui.QGraphicsView(Form) 00625 self.graphicsView.setGeometry(QtCore.QRect(40, 20, 300, 120)) 00626 self.graphicsView.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) 00627 self.graphicsView.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) 00628 self.graphicsView.setObjectName(_fromUtf8("graphicsView")) 00629 00630 #Set the graphics view image 00631 self.scene = QtGui.QGraphicsScene(); 00632 self.pixmap = QtGui.QPixmap( "TekBots.png" ) 00633 self.scene.addPixmap(self.pixmap) 00634 self.graphicsView.setScene( self.scene ) 00635 self.graphicsView.setObjectName(_fromUtf8("graphicsView")) 00636 00637 #Set text of all UI elements 00638 self.retranslateUi(Form) 00639 00640 #Connect Slots 00641 QtCore.QMetaObject.connectSlotsByName(Form) 00642 00643 def retranslateUi(self, Form): 00644 00645 #Set text of all UI elements 00646 Form.setWindowTitle(_translate("About", "About", None)) 00647 self.label.setText(_translate("About", "FDRM-KL46z Beat Factory", None)) 00648 self.label_2.setText(_translate("About", "Author: Ethan Takla", None)) 00649 self.label_3.setText(_translate("About", "Revision 0.1", None)) 00650 00651 #Start the app 00652 app = QApplication(sys.argv) 00653 window = QMainWindow() 00654 ui = Ui_MainWindow() 00655 ui.initializeVariables() 00656 ui.initializeUI(window) 00657 ui.initializeTable() 00658 ui.initializeSerial() 00659 ui.bind_events() 00660 00661 window.show() 00662 sys.exit(app.exec_())
Generated on Wed Jul 13 2022 09:14:43 by
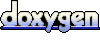