Baseline for testing
Embed:
(wiki syntax)
Show/hide line numbers
AddressMap.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file AddressMap.h 00003 * @brief Base class for storage of Address Mapping data. 00004 * @version: V1.0 00005 * @date: 9/17/2019 00006 00007 * 00008 * @note 00009 * Copyright (C) 2019 E3 Design. All rights reserved. 00010 * 00011 * @par 00012 * E3 Designers LLC is supplying this software for use with Cortex-M3 LPC1768 00013 * processor based microcontroller for the ESCM 2000 Monitor and Display. 00014 * * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 #ifndef _ADDRESS_MAP_ 00024 #define _ADDRESS_MAP_ 00025 00026 #include "mbed.h" 00027 00028 #define MAX_ADDR_LENGTH 20 00029 #define MAX_ADDRESSES 100 00030 00031 class Address 00032 { 00033 public: 00034 00035 int address; 00036 char description[MAX_ADDR_LENGTH]; 00037 00038 Address () { 00039 } 00040 00041 Address (const Address &obj) { 00042 // body of constructor 00043 address = obj.address; 00044 strcpy(description, obj.description); 00045 } 00046 00047 Address operator=(const Address& b) { 00048 Address a (b); 00049 return a; 00050 } 00051 00052 }; 00053 00054 class AddressMap 00055 { 00056 00057 public: 00058 00059 Address addresses[MAX_ADDRESSES]; 00060 00061 int reset(); 00062 int init(); 00063 int load(); 00064 int save(); 00065 00066 void display(Serial *pc); 00067 00068 char* getDescription(unsigned char idx ); 00069 00070 }; 00071 00072 #endif
Generated on Tue Jul 19 2022 06:22:36 by
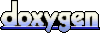