
nbiot mqtt test
Dependencies: mbed itoa DFRobot_SIM7000-master millis
main.cpp
00001 #include "mbed.h" 00002 #include <iostream> 00003 #include <string> 00004 #include "DFRobot_SIM7000.h" 00005 00006 #define server_ip "140.114.78.140" //140.114.89.66 //140.114.78.140 00007 #define PORT 1883 //TCP: 3307 //MQTT: 1883 00008 #define CLIENT_ID "" 00009 #define USERNAME "delta" 00010 #define PASSWORD "delta" 00011 #define SUBSCRIBE_TOPIC_PREFIX "nbiot/" 00012 #define PUBLISH_TOPIC "nbiot/gw" 00013 00014 DFRobot_SIM7000 sim7000; 00015 DigitalOut myled(LED1); 00016 00017 Serial pc(USBTX, USBRX, 57600); 00018 Serial nbiot_shield(PA_9, PA_10, 19200); //(tx,rx,baud) 00019 00020 char IMSI[20]; 00021 string str_IMSI; 00022 char *cTopic; 00023 00024 void PC_callback() { //useless now 00025 // Note: you need to actually read from the serial to clear the RX interrupt 00026 // nbiot_shield.putc(pc.getc()); 00027 char cmd[20]; 00028 pc.gets(cmd, 20); 00029 pc.printf("Command from PC: %s", cmd); 00030 nbiot_shield.printf(cmd); 00031 } 00032 00033 void NBIOT_callback() { //useless now 00034 //pc.printf("NBIOT_callback\r\n"); 00035 pc.putc(nbiot_shield.getc()); 00036 00037 //char msg[20]; 00038 //nbiot_shield.gets(msg, 20); 00039 //pc.printf("Message from SIM: %s", msg); 00040 } 00041 00042 00043 void connect_to_server(){ 00044 pc.printf("Connecting to the server %s......\r\n", server_ip); 00045 while(1){ 00046 if(sim7000.openNetwork(TCP,server_ip,PORT)){ //Start up TCP connection //TCP: 3307 //MQTT: 1883 00047 pc.printf("Connect OK\r\n"); 00048 break; 00049 }else{ 00050 pc.printf("Fail to connect\r\n"); 00051 wait(10); 00052 } 00053 } 00054 00055 pc.printf("Connecting to the MQTT......\r\n"); 00056 if(sim7000.mqttConnect(IMSI,USERNAME,PASSWORD)){ //MQTT connect request 00057 pc.printf("OK\r\n"); 00058 }else{ 00059 pc.printf("Failed\r\n"); 00060 //return; 00061 } 00062 00063 string topic = SUBSCRIBE_TOPIC_PREFIX + str_IMSI.substr(5); //first 5 number are fixed 00064 00065 cTopic = new char[topic.length() + 1]; 00066 strcpy(cTopic, topic.c_str()); 00067 pc.printf("Subscribe to the MQTT Topic %s......\r\n", cTopic); 00068 if(sim7000.mqttSubscribe(cTopic)){ 00069 pc.printf("OK\r\n"); 00070 }else{ 00071 pc.printf("Failed\r\n"); 00072 //return; 00073 } 00074 00075 } 00076 00077 00078 int main() { 00079 millisStart(); 00080 00081 sim7000.begin(nbiot_shield); 00082 00083 pc.printf("Turn ON SIM7000......\r\n"); 00084 if(sim7000.turnON()){ //Turn ON SIM7000 00085 pc.printf("Turn ON !\r\n"); 00086 } 00087 00088 pc.printf("Set baud rate......\r\n"); 00089 while(1){ 00090 if(sim7000.setBaudRate(19200)){ //Set SIM7000 baud rate from 115200 to 19200 reduce the baud rate to avoid distortion 00091 pc.printf("Set baud rate:19200\r\n"); 00092 break; 00093 }else{ 00094 pc.printf("Faile to set baud rate\r\n"); 00095 wait(1); 00096 } 00097 } 00098 00099 pc.printf("Set the APN......\r\n"); 00100 while(1){ 00101 if (sim7000.check_send_cmd("AT+CSTT=\"internet.iot\"\r\n","OK")){ 00102 pc.printf("OK\r\n"); 00103 break; 00104 } 00105 else{ 00106 pc.printf("Faile to set the APN\r\n"); 00107 wait_ms(1000); 00108 } 00109 } 00110 00111 pc.printf("Bring Up Wireless Connection with GPRS......\r\n"); 00112 while(1){ 00113 if(sim7000.check_send_cmd("AT+CIICR\r\n","OK")){ 00114 pc.printf("Successfully\r\n"); 00115 break; 00116 }else{ 00117 pc.printf("Faile to bring Up Wireless Connection with GPRS\r\n"); 00118 wait_ms(1000); 00119 } 00120 } 00121 00122 pc.printf("Get local IP address......\r\n"); 00123 char gprsBuffer[32]; 00124 while(1){ 00125 sim7000.cleanBuffer(gprsBuffer,32); 00126 sim7000.send_cmd("AT+CIFSR\r\n"); 00127 sim7000.readBuffer(gprsBuffer, 32, DEFAULT_TIMEOUT); 00128 00129 if(NULL != strstr(gprsBuffer, "ERROR")){ 00130 pc.printf("Faile to Get local IP address\r\n"); 00131 wait_ms(1000); 00132 } 00133 else{ 00134 //strcpy(local_IP, gprsBuffer+strlen("AT+CIFSR\r\n")+1); //implement issue here 00135 pc.printf("OK\r\n"); 00136 break; 00137 } 00138 } 00139 00140 00141 pc.printf("Get IMSI......\r\n"); 00142 while(1){ 00143 sim7000.cleanBuffer(gprsBuffer,32); 00144 sim7000.send_cmd("AT+CIMI\r\n"); 00145 sim7000.readBuffer(gprsBuffer, 32, DEFAULT_TIMEOUT); 00146 00147 if(NULL != strstr(gprsBuffer, "ERROR")){ 00148 pc.printf("Faile to Get IMSI\r\n"); 00149 wait_ms(1000); 00150 } 00151 else{ 00152 strncpy(IMSI, gprsBuffer+strlen("AT+CIMI\r\n")+1, 15); //implement issue here 00153 //Serial.println(gprsBuffer); 00154 pc.printf("%s\r\n", IMSI); 00155 str_IMSI = IMSI; 00156 break; 00157 } 00158 } 00159 00160 connect_to_server(); 00161 00162 00163 bool send_flag = true; 00164 int count = 0; 00165 char msg[100]; 00166 string str_msg; 00167 unsigned long previousTime = 0; 00168 unsigned long currentTime; 00169 int disconnection_count = 0; 00170 //string str_test = "aaaaaaaaaabbbbbbbbbbccccccccccddddddddddeeeeeeeeeeffffffffffgggggggggghhhhhhhhhhiiiiiiiiiijjjjjjjjjj"; //100bytes 00171 00172 //pc.attach(&PC_callback); 00173 //nbiot_shield.attach(&NBIOT_callback); 00174 00175 00176 while(1) { 00177 00178 if(nbiot_shield.readable()){ //check downlink 00179 char recv_msg[64]; 00180 string str_recv_msg; 00181 pc.printf("received: "); 00182 sim7000.cleanBuffer(recv_msg,64); 00183 //wait_ms(100); 00184 sim7000.readBuffer(recv_msg, 64, DEFAULT_TIMEOUT); 00185 00186 pc.printf("%s\r\n", recv_msg); 00187 00188 str_recv_msg = recv_msg; 00189 int i = str_recv_msg.find(cTopic); 00190 if (i>=0){ 00191 str_recv_msg = str_recv_msg.substr(i+strlen(cTopic)); 00192 } 00193 pc.printf("payload: %s\r\n", str_recv_msg); 00194 00195 } 00196 00197 currentTime = millis(); 00198 if(currentTime - previousTime > 600000 || send_flag == true){ 00199 send_flag = false; 00200 pc.printf("---------------------------\r\n"); 00201 sprintf(msg, "delta%d,%s", count, IMSI); 00202 pc.printf("%s\r\n", msg); 00203 str_msg = msg; 00204 if (sim7000.mqttPublish(PUBLISH_TOPIC, str_msg)){ 00205 pc.printf("Send OK\r\n"); 00206 }else{ 00207 pc.printf("Failed to send\r\n"); //Can do reconnection here 00208 disconnection_count++; 00209 sim7000.closeNetwork(); 00210 wait_ms(1000); 00211 connect_to_server(); 00212 send_flag = true; 00213 } 00214 pc.printf("Disconnection count: %d\r\n", disconnection_count); 00215 previousTime = currentTime; 00216 count++; 00217 } 00218 //wait_ms(10); 00219 00220 } 00221 00222 }
Generated on Wed Jul 27 2022 19:26:10 by
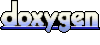