Mouse code for the MacroRat
Embed:
(wiki syntax)
Show/hide line numbers
serial_api.h
00001 00002 /** \addtogroup hal */ 00003 /** @{*/ 00004 /* mbed Microcontroller Library 00005 * Copyright (c) 2006-2013 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 #ifndef MBED_SERIAL_API_H 00020 #define MBED_SERIAL_API_H 00021 00022 #include "device.h" 00023 #include "hal/buffer.h" 00024 #include "hal/dma_api.h" 00025 00026 #if DEVICE_SERIAL 00027 00028 #define SERIAL_EVENT_TX_SHIFT (2) 00029 #define SERIAL_EVENT_RX_SHIFT (8) 00030 00031 #define SERIAL_EVENT_TX_MASK (0x00FC) 00032 #define SERIAL_EVENT_RX_MASK (0x3F00) 00033 00034 #define SERIAL_EVENT_ERROR (1 << 1) 00035 00036 /** 00037 * @defgroup SerialTXEvents Serial TX Events Macros 00038 * 00039 * @{ 00040 */ 00041 #define SERIAL_EVENT_TX_COMPLETE (1 << (SERIAL_EVENT_TX_SHIFT + 0)) 00042 #define SERIAL_EVENT_TX_ALL (SERIAL_EVENT_TX_COMPLETE) 00043 /**@}*/ 00044 00045 /** 00046 * @defgroup SerialRXEvents Serial RX Events Macros 00047 * 00048 * @{ 00049 */ 00050 #define SERIAL_EVENT_RX_COMPLETE (1 << (SERIAL_EVENT_RX_SHIFT + 0)) 00051 #define SERIAL_EVENT_RX_OVERRUN_ERROR (1 << (SERIAL_EVENT_RX_SHIFT + 1)) 00052 #define SERIAL_EVENT_RX_FRAMING_ERROR (1 << (SERIAL_EVENT_RX_SHIFT + 2)) 00053 #define SERIAL_EVENT_RX_PARITY_ERROR (1 << (SERIAL_EVENT_RX_SHIFT + 3)) 00054 #define SERIAL_EVENT_RX_OVERFLOW (1 << (SERIAL_EVENT_RX_SHIFT + 4)) 00055 #define SERIAL_EVENT_RX_CHARACTER_MATCH (1 << (SERIAL_EVENT_RX_SHIFT + 5)) 00056 #define SERIAL_EVENT_RX_ALL (SERIAL_EVENT_RX_OVERFLOW | SERIAL_EVENT_RX_PARITY_ERROR | \ 00057 SERIAL_EVENT_RX_FRAMING_ERROR | SERIAL_EVENT_RX_OVERRUN_ERROR | \ 00058 SERIAL_EVENT_RX_COMPLETE | SERIAL_EVENT_RX_CHARACTER_MATCH) 00059 /**@}*/ 00060 00061 #define SERIAL_RESERVED_CHAR_MATCH (255) 00062 00063 typedef enum { 00064 ParityNone = 0, 00065 ParityOdd = 1, 00066 ParityEven = 2, 00067 ParityForced1 = 3, 00068 ParityForced0 = 4 00069 } SerialParity; 00070 00071 typedef enum { 00072 RxIrq, 00073 TxIrq 00074 } SerialIrq; 00075 00076 typedef enum { 00077 FlowControlNone, 00078 FlowControlRTS, 00079 FlowControlCTS, 00080 FlowControlRTSCTS 00081 } FlowControl; 00082 00083 typedef void (*uart_irq_handler)(uint32_t id, SerialIrq event); 00084 00085 #if DEVICE_SERIAL_ASYNCH 00086 /** Asynch serial HAL structure 00087 */ 00088 typedef struct { 00089 struct serial_s serial; /**< Target specific serial structure */ 00090 struct buffer_s tx_buff; /**< TX buffer */ 00091 struct buffer_s rx_buff; /**< RX buffer */ 00092 uint8_t char_match; /**< Character to be matched */ 00093 uint8_t char_found; /**< State of the matched character */ 00094 } serial_t; 00095 00096 #else 00097 /** Non-asynch serial HAL structure 00098 */ 00099 typedef struct serial_s serial_t; 00100 00101 #endif 00102 00103 #ifdef __cplusplus 00104 extern "C" { 00105 #endif 00106 00107 /** 00108 * \defgroup hal_GeneralSerial Serial Configuration Functions 00109 * @{ 00110 */ 00111 00112 /** Initialize the serial peripheral. It sets the default parameters for serial 00113 * peripheral, and configures its specifieds pins. 00114 * 00115 * @param obj The serial object 00116 * @param tx The TX pin name 00117 * @param rx The RX pin name 00118 */ 00119 void serial_init(serial_t *obj, PinName tx, PinName rx); 00120 00121 /** Release the serial peripheral, not currently invoked. It requires further 00122 * resource management. 00123 * 00124 * @param obj The serial object 00125 */ 00126 void serial_free(serial_t *obj); 00127 00128 /** Configure the baud rate 00129 * 00130 * @param obj The serial object 00131 * @param baudrate The baud rate to be configured 00132 */ 00133 void serial_baud(serial_t *obj, int baudrate); 00134 00135 /** Configure the format. Set the number of bits, parity and the number of stop bits 00136 * 00137 * @param obj The serial object 00138 * @param data_bits The number of data bits 00139 * @param parity The parity 00140 * @param stop_bits The number of stop bits 00141 */ 00142 void serial_format(serial_t *obj, int data_bits, SerialParity parity, int stop_bits); 00143 00144 /** The serial interrupt handler registration 00145 * 00146 * @param obj The serial object 00147 * @param handler The interrupt handler which will be invoked when the interrupt fires 00148 * @param id The SerialBase object 00149 */ 00150 void serial_irq_handler(serial_t *obj, uart_irq_handler handler, uint32_t id); 00151 00152 /** Configure serial interrupt. This function is used for word-approach 00153 * 00154 * @param obj The serial object 00155 * @param irq The serial IRQ type (RX or TX) 00156 * @param enable Set to non-zero to enable events, or zero to disable them 00157 */ 00158 void serial_irq_set(serial_t *obj, SerialIrq irq, uint32_t enable); 00159 00160 /** Get character. This is a blocking call, waiting for a character 00161 * 00162 * @param obj The serial object 00163 */ 00164 int serial_getc(serial_t *obj); 00165 00166 /** Send a character. This is a blocking call, waiting for a peripheral to be available 00167 * for writing 00168 * 00169 * @param obj The serial object 00170 * @param c The character to be sent 00171 */ 00172 void serial_putc(serial_t *obj, int c); 00173 00174 /** Check if the serial peripheral is readable 00175 * 00176 * @param obj The serial object 00177 * @return Non-zero value if a character can be read, 0 if nothing to read 00178 */ 00179 int serial_readable(serial_t *obj); 00180 00181 /** Check if the serial peripheral is writable 00182 * 00183 * @param obj The serial object 00184 * @return Non-zero value if a character can be written, 0 otherwise. 00185 */ 00186 int serial_writable(serial_t *obj); 00187 00188 /** Clear the serial peripheral 00189 * 00190 * @param obj The serial object 00191 */ 00192 void serial_clear(serial_t *obj); 00193 00194 /** Set the break 00195 * 00196 * @param obj The serial object 00197 */ 00198 void serial_break_set(serial_t *obj); 00199 00200 /** Clear the break 00201 * 00202 * @param obj The serial object 00203 */ 00204 void serial_break_clear(serial_t *obj); 00205 00206 /** Configure the TX pin for UART function. 00207 * 00208 * @param tx The pin name used for TX 00209 */ 00210 void serial_pinout_tx(PinName tx); 00211 00212 /** Configure the serial for the flow control. It sets flow control in the hardware 00213 * if a serial peripheral supports it, otherwise software emulation is used. 00214 * 00215 * @param obj The serial object 00216 * @param type The type of the flow control. Look at the available FlowControl types. 00217 * @param rxflow The TX pin name 00218 * @param txflow The RX pin name 00219 */ 00220 void serial_set_flow_control(serial_t *obj, FlowControl type, PinName rxflow, PinName txflow); 00221 00222 #if DEVICE_SERIAL_ASYNCH 00223 00224 /**@}*/ 00225 00226 /** 00227 * \defgroup hal_AsynchSerial Asynchronous Serial Hardware Abstraction Layer 00228 * @{ 00229 */ 00230 00231 /** Begin asynchronous TX transfer. The used buffer is specified in the serial object, 00232 * tx_buff 00233 * 00234 * @param obj The serial object 00235 * @param tx The transmit buffer 00236 * @param tx_length The number of bytes to transmit 00237 * @param tx_width Deprecated argument 00238 * @param handler The serial handler 00239 * @param event The logical OR of events to be registered 00240 * @param hint A suggestion for how to use DMA with this transfer 00241 * @return Returns number of data transfered, otherwise returns 0 00242 */ 00243 int serial_tx_asynch(serial_t *obj, const void *tx, size_t tx_length, uint8_t tx_width, uint32_t handler, uint32_t event, DMAUsage hint); 00244 00245 /** Begin asynchronous RX transfer (enable interrupt for data collecting) 00246 * The used buffer is specified in the serial object - rx_buff 00247 * 00248 * @param obj The serial object 00249 * @param rx The receive buffer 00250 * @param rx_length The number of bytes to receive 00251 * @param rx_width Deprecated argument 00252 * @param handler The serial handler 00253 * @param event The logical OR of events to be registered 00254 * @param handler The serial handler 00255 * @param char_match A character in range 0-254 to be matched 00256 * @param hint A suggestion for how to use DMA with this transfer 00257 */ 00258 void serial_rx_asynch(serial_t *obj, void *rx, size_t rx_length, uint8_t rx_width, uint32_t handler, uint32_t event, uint8_t char_match, DMAUsage hint); 00259 00260 /** Attempts to determine if the serial peripheral is already in use for TX 00261 * 00262 * @param obj The serial object 00263 * @return Non-zero if the RX transaction is ongoing, 0 otherwise 00264 */ 00265 uint8_t serial_tx_active(serial_t *obj); 00266 00267 /** Attempts to determine if the serial peripheral is already in use for RX 00268 * 00269 * @param obj The serial object 00270 * @return Non-zero if the RX transaction is ongoing, 0 otherwise 00271 */ 00272 uint8_t serial_rx_active(serial_t *obj); 00273 00274 /** The asynchronous TX and RX handler. 00275 * 00276 * @param obj The serial object 00277 * @return Returns event flags if an RX transfer termination condition was met; otherwise returns 0 00278 */ 00279 int serial_irq_handler_asynch(serial_t *obj); 00280 00281 /** Abort the ongoing TX transaction. It disables the enabled interupt for TX and 00282 * flushes the TX hardware buffer if TX FIFO is used 00283 * 00284 * @param obj The serial object 00285 */ 00286 void serial_tx_abort_asynch(serial_t *obj); 00287 00288 /** Abort the ongoing RX transaction. It disables the enabled interrupt for RX and 00289 * flushes the RX hardware buffer if RX FIFO is used 00290 * 00291 * @param obj The serial object 00292 */ 00293 void serial_rx_abort_asynch(serial_t *obj); 00294 00295 /**@}*/ 00296 00297 #endif 00298 00299 #ifdef __cplusplus 00300 } 00301 #endif 00302 00303 #endif 00304 00305 #endif 00306 00307 /** @}*/
Generated on Tue Jul 12 2022 17:41:25 by
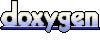