Mouse code for the MacroRat
Embed:
(wiki syntax)
Show/hide line numbers
DirHandle.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_DIRHANDLE_H 00017 #define MBED_DIRHANDLE_H 00018 00019 #include <stdint.h> 00020 #include "platform/platform.h" 00021 00022 #include "FileHandle.h" 00023 00024 namespace mbed { 00025 /** \addtogroup drivers */ 00026 /** @{*/ 00027 00028 /** Represents a directory stream. Objects of this type are returned 00029 * by a FileSystemLike's opendir method. Implementations must define 00030 * at least closedir, readdir and rewinddir. 00031 * 00032 * If a FileSystemLike class defines the opendir method, then the 00033 * directories of an object of that type can be accessed by 00034 * DIR *d = opendir("/example/directory") (or opendir("/example") 00035 * to open the root of the filesystem), and then using readdir(d) etc. 00036 * 00037 * The root directory is considered to contain all FileLike and 00038 * FileSystemLike objects, so the DIR* returned by opendir("/") will 00039 * reflect this. 00040 * 00041 * @Note Synchronization level: Set by subclass 00042 */ 00043 class DirHandle { 00044 public: 00045 MBED_DEPRECATED_SINCE("mbed-os-5.4", 00046 "The mbed 2 filesystem classes have been superseeded by the FileSystem api, " 00047 "Replaced by File") 00048 DirHandle() {} 00049 00050 /** Closes the directory. 00051 * 00052 * @returns 00053 * 0 on success, 00054 * -1 on error. 00055 */ 00056 virtual int closedir()=0; 00057 00058 /** Return the directory entry at the current position, and 00059 * advances the position to the next entry. 00060 * 00061 * @returns 00062 * A pointer to a dirent structure representing the 00063 * directory entry at the current position, or NULL on reaching 00064 * end of directory or error. 00065 */ 00066 virtual struct dirent *readdir()=0; 00067 00068 /** Resets the position to the beginning of the directory. 00069 */ 00070 virtual void rewinddir()=0; 00071 00072 /** Returns the current position of the DirHandle. 00073 * 00074 * @returns 00075 * the current position, 00076 * -1 on error. 00077 */ 00078 virtual off_t telldir() { return -1; } 00079 00080 /** Sets the position of the DirHandle. 00081 * 00082 * @param location The location to seek to. Must be a value returned by telldir. 00083 */ 00084 virtual void seekdir(off_t location) { (void)location;} 00085 00086 virtual ~DirHandle() {} 00087 00088 protected: 00089 00090 /** Acquire exclusive access to this object. 00091 */ 00092 virtual void lock() { 00093 // Stub 00094 } 00095 00096 /** Release exclusive access to this object. 00097 */ 00098 virtual void unlock() { 00099 // Stub 00100 } 00101 00102 protected: 00103 /** Internal-only constructor to work around deprecated notices when not used 00104 *. due to nested deprecations and difficulty of compilers finding their way around 00105 * the class hierarchy 00106 */ 00107 friend class FileSystemLike; 00108 DirHandle(int) {} 00109 }; 00110 00111 } // namespace mbed 00112 00113 #endif /* MBED_DIRHANDLE_H */ 00114 00115 /** @}*/
Generated on Tue Jul 12 2022 17:41:24 by
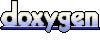