mbed RPC
Embed:
(wiki syntax)
Show/hide line numbers
parse_pins.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "port_api.h" 00017 00018 namespace mbed { 00019 00020 PinName parse_pins(const char *str) { 00021 #if defined(TARGET_LPC1768) || defined(TARGET_LPC11U24) || defined(TARGET_LPC2368) 00022 00023 #if defined(TARGET_UBLOX_C027) 00024 #define p5 P0_9 00025 #define p6 P0_8 00026 #define p7 P0_7 00027 #define p8 P0_6 00028 #define p9 P0_0 00029 #define p10 P0_1 00030 #define p11 P0_18 00031 #define p12 P0_17 00032 #define p13 P0_15 00033 #define p14 P0_16 00034 #define p15 P0_23 00035 #define p16 P0_24 00036 #define p17 P0_25 00037 #define p18 P0_26 00038 #define p19 P1_30 00039 #define p20 P1_30 00040 #define p21 P2_5 00041 #define p22 P2_4 00042 #define p23 P2_3 00043 #define p24 P2_2 00044 #define p25 P2_1 00045 #define p26 P2_0 00046 #define p27 P0_11 00047 #define p28 P0_10 00048 #define p29 P0_5 00049 #define p30 P0_4 00050 00051 #endif 00052 static const PinName pin_names[] = {p5, p6, p7, p8, p9, p10, p11, p12, p13, p14 00053 , p15, p16, p17, p18, p19, p20, p21, p22, p23 00054 , p24, p25, p26, p27, p28, p29, p30}; 00055 00056 #elif defined(TARGET_LPC1114) 00057 static const PinName pin_names[] = {dp1, dp2, dp4, dp5, dp6, dp9, dp10, dp11 00058 , dp13, dp14, dp15, dp16, dp17, dp18, dp23 00059 , dp24, dp25, dp26, dp27, dp28}; 00060 #elif defined(TARGET_LPC4088) 00061 static const PinName pin_names[] = {NC, NC, NC, NC, p5, p6, p7, p8, p9, p10, p11, p12, p13, p14 00062 , p15, p16, p17, p18, p19, p20, NC, NC, p23 00063 , p24, p25, p26, p27, p28, p29, p30, p31, p32 00064 , p33, p34, NC, NC, p37, p38, p39, NC, NC, NC, NC, NC, NC, NC}; 00065 #elif defined(TARGET_LPC4088_DM) 00066 static const PinName pin_names[] = {p1, p2, p3, p4, NC, NC, p7, p8, p9, p10, p11, p12, p13, p14 00067 , p15, p16, p17, p18, p19, p20, p21, p22, p23 00068 , p24, p25, p26, NC, NC, p29, p30, NC, NC 00069 , NC, NC, NC, NC, NC, NC, NC, NC, p41, p42, p43, p44, p45, p46}; 00070 #endif 00071 00072 #if defined(TARGET_LPC1768) || defined(TARGET_LPC11U24) || defined(TARGET_LPC2368) || defined(TARGET_LPC812) || defined(TARGET_LPC4088) || defined(TARGET_LPC4088_DM) || defined(TARGET_LPC1114) 00073 if (str[0] == 'P') { // Pn_n 00074 uint32_t port = str[1] - '0'; 00075 uint32_t pin = str[3] - '0'; // Pn_n 00076 uint32_t pin2 = str[4] - '0'; // Pn_nn 00077 if (pin2 <= 9) { 00078 pin = pin * 10 + pin2; 00079 } 00080 return port_pin((PortName)port, pin); 00081 00082 #elif defined(TARGET_KL25Z) || defined(TARGET_KL05Z) || defined(TARGET_KL46Z) || defined(TARGET_K64F) 00083 if (str[0] == 'P' && str[1] == 'T') { // PTxn 00084 uint32_t port = str[2] - 'A'; 00085 uint32_t pin = str[3] - '0'; // PTxn 00086 uint32_t pin2 = str[4] - '0'; // PTxnn 00087 00088 if (pin2 <= 9) { 00089 pin = pin * 10 + pin2; 00090 } 00091 return port_pin((PortName)port, pin); 00092 #endif 00093 00094 #if defined(TARGET_LPC1768) || defined(TARGET_LPC11U24) || defined(TARGET_LPC2368) 00095 } else if (str[0] == 'p') { // pn 00096 uint32_t pin = str[1] - '0'; // pn 00097 uint32_t pin2 = str[2] - '0'; // pnn 00098 if (pin2 <= 9) { 00099 pin = pin * 10 + pin2; 00100 } 00101 if (pin < 5 || pin > 30) { 00102 return NC; 00103 } 00104 return pin_names[pin - 5]; 00105 #elif defined(TARGET_LPC4088) || defined(TARGET_LPC4088_DM) 00106 } else if (str[0] == 'p') { // pn 00107 uint32_t pin = str[1] - '0'; // pn 00108 uint32_t pin2 = str[2] - '0'; // pnn 00109 if (pin2 <= 9) { 00110 pin = pin * 10 + pin2; 00111 } 00112 if (pin < 1 || pin > 46) { 00113 return NC; 00114 } 00115 return pin_names[pin - 1]; 00116 #endif 00117 00118 } else if (str[0] == 'L') { // LEDn 00119 switch (str[3]) { 00120 case '1' : return LED1; 00121 case '2' : return LED2; 00122 case '3' : return LED3; 00123 case '4' : return LED4; 00124 } 00125 00126 } else if (str[0] == 'U') { // USB?X 00127 switch (str[3]) { 00128 case 'T' : return USBTX; 00129 case 'R' : return USBRX; 00130 } 00131 } 00132 00133 return NC; 00134 } 00135 00136 }
Generated on Sun Jul 24 2022 15:11:06 by
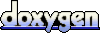