
huohuoh
Dependencies: NetServices mbed C027_Supports mbed-rpcx
Modem.cpp
00001 #include "MDM.h" 00002 #include "mbed.h" 00003 #include "Modem.h" 00004 #include <sstream> 00005 00006 extern Serial dbg; 00007 00008 void SetModem(MDMSerial &mdm) 00009 { 00010 MDMParser::DevStatus devStatus = {}; 00011 MDMParser::NetStatus netStatus = {}; 00012 bool mdmOk = mdm.init(SIMPIN, &devStatus); 00013 mdm.dumpDevStatus(&devStatus); 00014 if (mdmOk) { 00015 mdmOk = mdm.registerNet(&netStatus); 00016 mdm.dumpNetStatus(&netStatus); 00017 } 00018 if (mdmOk) 00019 { 00020 MDMParser::IP ip = mdm.join(APN,USERNAME,PASSWORD); 00021 if (ip != NOIP) 00022 { 00023 mdm.dumpIp(ip); 00024 dbg.printf("\r\n<Modem Connected>"); 00025 } 00026 } 00027 } 00028 00029 std::string Convertint(int data) 00030 { 00031 std::stringstream c; 00032 c << data; 00033 00034 return c.str(); 00035 } 00036 00037 std::string Convert(double data) 00038 { 00039 std::stringstream c; 00040 c << data; 00041 00042 return c.str(); 00043 } 00044 00045 bool PostData(MDMSerial &mdm, const int &identifier_, const std::vector<int> &input, bool mode) 00046 { 00047 char buf[512] = ""; 00048 int ret; 00049 bool res = false; 00050 int socket = mdm.socketSocket(MDMParser::IPPROTO_TCP); 00051 00052 std::string body; 00053 body += "{\"val\":\""; 00054 body += identifier_; 00055 body += std::string(input.begin(), input.end()); 00056 body += "\"}"; 00057 00058 std::string http; 00059 if(mode == SKADA) 00060 { 00061 http += "PUT /todo/api/v1.0/scadas/1 HTTP/1.1\r\n"; 00062 } 00063 else if(mode == TASK) 00064 { 00065 http += "POST /todo/api/v1.0/tasks HTTP/1.1\r\n"; 00066 } 00067 http += "Host: 182.23.67.168:5000\r\n"; 00068 http += "Authorization: Basic am9objpoZWxsbw==\r\n"; 00069 http += "Connection: close\r\n"; 00070 http += "Content-Type: application/json\r\n"; 00071 http += "Content-Length: "; 00072 http += Convertint((int)body.size()); 00073 http += "\r\n\r\n"; 00074 http += body; 00075 http += "\r\n\r\n"; 00076 00077 dbg.printf("Request:\r\n%s",http.c_str()); 00078 00079 dbg.printf("Ready to post\r\n"); 00080 mdm.socketSetBlocking(socket, 10000); 00081 if (mdm.socketConnect(socket, "182.23.67.168", 5000)) 00082 { 00083 dbg.printf("Sending request\r\n"); 00084 mdm.socketSend(socket, http.c_str(), http.size()); 00085 00086 ret = mdm.socketRecv(socket, buf, sizeof(buf)-1); 00087 if (ret > 0) 00088 { 00089 dbg.printf("Socket Recv \"%*s\"\r\n", ret, buf); 00090 if(strstr(buf, "HTTP/1.0 200") || strstr(buf, "HTTP/1.0 201")) 00091 { 00092 res = true; 00093 } 00094 else 00095 { 00096 res = false; 00097 } 00098 } 00099 else 00100 { 00101 dbg.printf("return 0\r\n"); 00102 res = false; 00103 } 00104 00105 mdm.socketClose(socket); 00106 mdm.socketFree(socket); 00107 } 00108 else 00109 { 00110 mdm.socketSetBlocking(socket, 10000); 00111 mdm.socketConnect(socket, "182.23.67.168", 5000); 00112 res = false; 00113 } 00114 return res; 00115 } 00116 00117 bool chekConect(MDMSerial &mdm) 00118 { 00119 bool stat = mdm.CheckCon(); 00120 if(!stat) 00121 { 00122 MDMParser::IP ip = mdm.join(APN,USERNAME,PASSWORD); 00123 if (ip != NOIP) 00124 { 00125 mdm.dumpIp(ip); 00126 return true; 00127 } 00128 else 00129 { 00130 mdm.join(APN,USERNAME,PASSWORD); 00131 return false; 00132 } 00133 } 00134 else 00135 return true; 00136 }
Generated on Thu Jul 14 2022 15:19:50 by
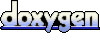