
huohuoh
Dependencies: NetServices mbed C027_Supports mbed-rpcx
DataProcess.h
00001 #ifndef DATAPROCESS_H 00002 #define DATAPROCESS_H 00003 00004 #include <vector> 00005 #include <queue> 00006 #include <string> 00007 #include "AruFraming.h" 00008 00009 class FtlMessage 00010 { 00011 public: 00012 FtlMessage(); 00013 ~FtlMessage(); 00014 00015 enum msg 00016 { 00017 OK = 0, 00018 FAILED = -1, 00019 PROERROR = -2, 00020 NOTANSWER = -3 00021 }ftl_msg; 00022 00023 msg checkMessage(const int &lastIdentifier, const int &identifier_); 00024 00025 private: 00026 00027 }; 00028 00029 //------------------------------------------------------------- 00030 class DataMessage 00031 { 00032 public: 00033 DataMessage(); 00034 ~DataMessage(); 00035 00036 enum msg 00037 { 00038 OK = 0, 00039 FAILED = -1, 00040 CHANGE = 1, 00041 00042 STATECHANGE = 2, 00043 00044 }data_msg; 00045 00046 typedef int (*_CALLBACKPTR)(const char* buf, int len, void* param); 00047 msg processFromSlave(int identifier_=0, std::string buf="", _CALLBACKPTR cb = NULL, void* param = NULL); 00048 00049 template<class T> 00050 inline msg processFromSlave(int identifier_, std::string buf, int (*cb)(const char* buf, int len, T* param), 00051 T* param) 00052 { 00053 return processFromSlave(identifier_, buf, (_CALLBACKPTR)cb, (void*)param); 00054 } 00055 00056 msg checkStateMessage(const int &identifier_, const std::string &stream, int *pStep); 00057 msg checkOrderState(const int &identifier_, const std::string &stream, int *pStep); 00058 msg checkInterlock(const int &identifier_, const std::string &stream, int *pStep); 00059 //private: 00060 00061 protected: 00062 00063 typedef struct{ int *state;} MeterState; 00064 static int _cbstate(const char* buf, int len, MeterState* param); 00065 00066 typedef struct{ int *state;} OrderState; 00067 static int _cbOstate(const char* buf, int len, OrderState* param); 00068 00069 typedef struct{int *state;} InterlockState; 00070 static int _cbInterlock(const char* buf, int len, InterlockState* param); 00071 }; 00072 00073 //------------------------------------------------------------- 00074 class DataProcess : public FtlMessage, public DataMessage 00075 { 00076 public: 00077 DataProcess(); 00078 ~DataProcess(); 00079 00080 typedef enum state_ 00081 { 00082 OK = 0, 00083 ERROR = -1, 00084 00085 STAY = 0, 00086 STEPUP = 1, 00087 STEPDOWN = 2, 00088 STEPJUMP = 3, 00089 STEPEND = -1, 00090 STAYNOL = -2, 00091 FINISH = 4, 00092 00093 NOORDER = 0, 00094 ORDERRCV = 2, 00095 PLANORDERPROC = 3, 00096 UNPLANORDERPROC = 4, 00097 ORDERFINERROR = 9, 00098 ORDERFINNOERROR = 10, 00099 00100 ACCCLOSED = 0, 00101 ACCOPEN = 1 00102 }STATE; 00103 00104 void CheckFtlMassage(const int &lastIdentifier, const AruFrm &frm); 00105 FtlMessage::msg getFtlMessage(); 00106 00107 void CheckDtMassage(const AruFrm &frm); 00108 DataMessage::msg getDtMessage(); 00109 state_ checkState(); 00110 state_ checkOrderState(); 00111 state_ chackInterlock(); 00112 00113 private: 00114 FtlMessage::msg FtlMsg; 00115 00116 DataMessage::msg DataMsg; 00117 int stateTmp; 00118 int lastState; 00119 int OrderStateTmp; 00120 int LastOrderState; 00121 int InterlockState; 00122 }; 00123 00124 //------------------------------------------------------------- 00125 #endif // DATAPROCESS_H
Generated on Thu Jul 14 2022 15:19:50 by
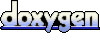