
huohuoh
Dependencies: NetServices mbed C027_Supports mbed-rpcx
CommChannel.cpp
00001 #include "mbed.h" 00002 #include "CommChannel.h" 00003 00004 extern Serial dbg; 00005 00006 //----------------------------------------------------------------- 00007 extern std::vector<int> RCV_BUFF; // temporary buffer receiving progress 00008 std::vector<int> RCVD; // buffer completed receiving 00009 extern int timerRxTOutCnt; 00010 00011 //----------------------------------------------------------------- 00012 // Mechanism for receive timeout 00013 // 00014 extern int timerRxTOutCnt; 00015 00016 //----------------------------------------------------------------- 00017 // Serial definition 00018 // 00019 extern Serial sock; 00020 00021 //----------------------------------------------------------------- 00022 // Wrapper class 00023 // 00024 CommChannel::CommChannel() 00025 { 00026 dbg.printf("<CommChannel>"); 00027 } 00028 00029 CommChannel::~CommChannel() 00030 { 00031 00032 } 00033 00034 void CommChannel::connect(const std::string &host) 00035 { 00036 } 00037 00038 int CommChannel::dataAvailable() 00039 { 00040 return RCVD.size(); 00041 } 00042 00043 std::vector<int> CommChannel::read() 00044 { 00045 std::vector<int> result; 00046 00047 for(int i=0; i<RCVD.size(); i++) 00048 result.push_back(RCVD[i]); 00049 00050 RCVD.clear(); 00051 return result; 00052 } 00053 00054 void CommChannel::write(const std::vector<int> &s) 00055 { 00056 for(int i=0; i<(int)s.size(); i++) 00057 { 00058 sock.putc(s[i] & 0x0FF); 00059 } 00060 } 00061 00062 void CommChannel::Tick10ms() 00063 { 00064 timerRxTOutCnt++; 00065 if(timerRxTOutCnt >= 10) 00066 { 00067 timerRxTOutCnt = 0; 00068 00069 if(RCV_BUFF.size() > 0) 00070 { 00071 for(int i=0; i<RCV_BUFF.size(); i++) 00072 { 00073 RCVD.push_back(RCV_BUFF[i]); 00074 dbg.printf("[%02X]", RCV_BUFF[i] & 0x0FF); 00075 } 00076 RCV_BUFF.clear(); 00077 } 00078 } 00079 } 00080 00081 //-----------------------------------------------------------------
Generated on Thu Jul 14 2022 15:19:50 by
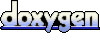