
huohuoh
Dependencies: NetServices mbed C027_Supports mbed-rpcx
AruChannel.cpp
00001 #include "mbed.h" 00002 #include "AruChannel.h" 00003 00004 extern Serial dbg; 00005 00006 AruChannel::AruChannel() 00007 { 00008 dbg.printf("<AruChannel>"); 00009 00010 COM.connect("127.0.0.1:123"); 00011 commId = 0; 00012 } 00013 00014 AruChannel::~AruChannel() 00015 { 00016 00017 } 00018 00019 int AruChannel::ReceiveCount() 00020 { 00021 return Frm.RCV_QUE.size(); 00022 } 00023 00024 AruFrm AruChannel::Receive() 00025 { 00026 AruFrm res = Frm.RCV_QUE.front(); 00027 Frm.RCV_QUE.pop(); 00028 return res; 00029 } 00030 00031 void AruChannel::Transmit(const AruFrm &f) 00032 { 00033 XMT_QUE.push(f); 00034 } 00035 00036 void AruChannel::Process() 00037 { 00038 if(COM.dataAvailable() > 0) 00039 { 00040 std::vector<int> r = COM.read(); 00041 00042 Frm.ProcessFromAru(r); 00043 } 00044 00045 while(XMT_QUE.size() > 0) 00046 { 00047 AruFrm t = XMT_QUE.front(); 00048 XMT_QUE.pop(); 00049 00050 // TCP/IP 00051 std::vector<int> x = AruFraming::CreateFrame(t); 00052 COM.write(x); 00053 00054 commId++; 00055 } 00056 } 00057 00058 void AruChannel::Tick10ms() 00059 { 00060 COM.Tick10ms(); 00061 }
Generated on Thu Jul 14 2022 15:19:50 by
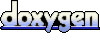