
sakura.io Evalution board's example.
Dependencies: AQM0802A BME280 MPU9250_SPI SakuraIO gps mbed
Fork of SakuraIO_Evaluation_Board_Standard by
main.cpp
00001 #include <mbed.h> 00002 #include <AQM0802A.h> 00003 #include <BME280.h> 00004 #include <MPU9250.h> 00005 #include <SakuraIO.h> 00006 #include "SakuraPinNames.h" 00007 #include "sensors.h" 00008 #include "gps.h" 00009 00010 // Serial over CMSIS_DAP 00011 Serial pc(DAP_UART_TX, DAP_UART_RX, 9600); 00012 00013 // GPS 00014 Serial gps(GPS_TX, GPS_RX, 9600); 00015 DigitalOut gps_en(GPS_EN); 00016 00017 // LED 00018 DigitalOut led_1(LED1); 00019 DigitalOut led_2(LED2); 00020 DigitalOut led_3(LED3); 00021 DigitalOut led_4(LED4); 00022 00023 // LCD backlight 00024 DigitalOut lcd_led(LED_LCD); 00025 00026 // Switch 00027 DigitalIn sw_1(SW1); 00028 DigitalIn sw_2(SW2); 00029 DigitalIn sw_3(SW3); 00030 DigitalIn sw_4(SW4); 00031 DigitalIn sw_5(SW5); 00032 DigitalIn sw_6(SW6); 00033 00034 // Internal I2C 00035 I2C internal_i2c(I2C_INTERNAL_SDA, I2C_INTERNAL_SCL); 00036 AQM0802A lcd(internal_i2c); 00037 BME280 bme280(internal_i2c); 00038 00039 // SPI 00040 SPI internal_mpu9250_spi(SPI_MPU_MOSI, SPI_MPU_MISO, SPI_MPU_SCK); 00041 mpu9250_spi mpu9250(internal_mpu9250_spi, SPI_MPU_CS); 00042 00043 // sakura.io 00044 SakuraIO_I2C sakuraio(I2C_SDA, I2C_SCL); 00045 00046 SensorData sensor_data; 00047 00048 // GPS UART buffer 00049 char uart_buffer[128] = {0}; 00050 int uart_buffer_index = 0; 00051 00052 // NMEA Decoder 00053 GPSDecoder gps_decoder; 00054 00055 void gps_uart_buffering_handler(); 00056 00057 const int SEND_INTERVAL_TICKS_PAR_COUNT = 1500; 00058 00059 void setup() 00060 { 00061 lcd_led = 1; 00062 pc.printf("Hello World !\r\n"); 00063 lcd.cls(); 00064 lcd.printf("Hello"); 00065 00066 // Initialize sensors 00067 bme280.initialize(); 00068 pc.printf("BME280 ok.\r\n"); 00069 mpu9250.init(1, BITS_DLPF_CFG_188HZ); 00070 pc.printf("MPU9250 ok. WHOAMI=%02x\r\n", mpu9250.whoami()); 00071 if (mpu9250.whoami() != 0x71) { 00072 pc.printf("[ERROR] MPU9250 init fail.\r\n"); 00073 } 00074 00075 mpu9250.set_gyro_scale(BITS_FS_2000DPS); //Set full scale range for gyros 00076 mpu9250.set_acc_scale(BITS_FS_16G); //Set full scale range for accs 00077 mpu9250.calib_acc(); 00078 mpu9250.AK8963_calib_Magnetometer(); 00079 00080 // active high 00081 gps_en = 1; 00082 gps.attach(&gps_uart_buffering_handler, Serial::RxIrq); 00083 00084 led_1 = 1; 00085 led_2 = 0; 00086 00087 pc.printf("Send par %d seconds.\r\n", (SEND_INTERVAL_TICKS_PAR_COUNT * 200) / 1000); 00088 } 00089 00090 void read_sensor_data() 00091 { 00092 sensor_data.bme280.temperature = bme280.getTemperature(); 00093 sensor_data.bme280.pressure = bme280.getPressure(); 00094 sensor_data.bme280.humidity = bme280.getHumidity(); 00095 00096 mpu9250.read_all(); 00097 sensor_data.mpu9250.temperature = mpu9250.Temperature; 00098 for (int cnt_send = 0; cnt_send < 3; cnt_send++) { 00099 sensor_data.mpu9250.accelerometer[cnt_send] = mpu9250.accelerometer_data[cnt_send]; 00100 sensor_data.mpu9250.gyroscope[cnt_send] = mpu9250.gyroscope_data[cnt_send]; 00101 sensor_data.mpu9250.magnetometer[cnt_send] = mpu9250.Magnetometer[cnt_send]; 00102 } 00103 } 00104 00105 void enqueue_sensor_data(int counter) 00106 { 00107 sakuraio.enqueueTx(0, (int32_t)counter); 00108 sakuraio.enqueueTx(1, sensor_data.bme280.temperature); 00109 sakuraio.enqueueTx(2, sensor_data.bme280.pressure); 00110 sakuraio.enqueueTx(3, sensor_data.bme280.humidity); 00111 sakuraio.enqueueTx(4, sensor_data.mpu9250.accelerometer[0]); 00112 sakuraio.enqueueTx(5, sensor_data.mpu9250.accelerometer[1]); 00113 sakuraio.enqueueTx(6, sensor_data.mpu9250.accelerometer[2]); 00114 sakuraio.enqueueTx(7, sensor_data.mpu9250.gyroscope[0]); 00115 sakuraio.enqueueTx(8, sensor_data.mpu9250.gyroscope[1]); 00116 sakuraio.enqueueTx(9, sensor_data.mpu9250.gyroscope[2]); 00117 sakuraio.enqueueTx(10, sensor_data.mpu9250.magnetometer[0]); 00118 sakuraio.enqueueTx(11, sensor_data.mpu9250.magnetometer[1]); 00119 sakuraio.enqueueTx(12, sensor_data.mpu9250.magnetometer[2]); 00120 sakuraio.enqueueTx(13, gps_decoder.get_longitude()); 00121 sakuraio.enqueueTx(14, gps_decoder.get_latitude()); 00122 sakuraio.enqueueTx(15, gps_decoder.get_unixtime()); 00123 } 00124 00125 void gps_uart_buffering_handler() 00126 { 00127 while (gps.readable() == 1) { 00128 char c = gps.getc(); 00129 if (c == '\r') { 00130 continue; 00131 } 00132 uart_buffer[uart_buffer_index] = c; 00133 uart_buffer_index++; 00134 if (c == '\n') { 00135 uart_buffer[uart_buffer_index - 1] = '\0'; 00136 gps_decoder.set_nmea_message(uart_buffer); 00137 gps_decoder.decode(); 00138 uart_buffer_index = 0; 00139 } 00140 } 00141 } 00142 00143 00144 void loop() 00145 { 00146 static int cnt_send = 1; 00147 static int tick_by_200ms = 0; 00148 static int stat_sw5 = -1; 00149 00150 if((sakuraio.getConnectionStatus() & 0x80) == 0x00) { 00151 //Offline 00152 lcd.cls(); 00153 lcd.printf("Offline"); 00154 pc.printf("Network is offline.\r\n(After 1 sec to running retry.)\r\n"); 00155 wait(1); 00156 return; 00157 } 00158 00159 if (stat_sw5 != sw_5) { 00160 stat_sw5 = sw_5; 00161 led_3 = stat_sw5; //State: `Send Enable' 00162 if (stat_sw5 == 0) { 00163 lcd.cls(); 00164 lcd.printf("Send:OFF"); 00165 } else { 00166 cnt_send = 1; 00167 tick_by_200ms = 0; 00168 lcd.cls(); 00169 lcd.printf("Send:ON"); 00170 } 00171 } 00172 00173 if (stat_sw5 == 1) { 00174 if ((tick_by_200ms % SEND_INTERVAL_TICKS_PAR_COUNT) == 0) { //Send data intarval is 5 minutes. 00175 pc.printf("\r\n\r\n--------------------\r\n"); 00176 read_sensor_data(); 00177 pc.printf("BME280\r\n"); 00178 pc.printf("\tTemp: %.2fC\r\n", sensor_data.bme280.temperature); 00179 pc.printf("\tPres: %.2fhPa\r\n", sensor_data.bme280.pressure); 00180 pc.printf("\tHum: %.2f%%\r\n", sensor_data.bme280.humidity); 00181 pc.printf("MPU9250\r\n"); 00182 pc.printf("\tTemp: %.2fC\r\n", sensor_data.mpu9250.temperature); 00183 for (int j = 0; j < 3; j++) { 00184 pc.printf("\tacc[%d]: %.2f\r\n", j, sensor_data.mpu9250.accelerometer[j]); 00185 pc.printf("\tgyro[%d]: %.2f\r\n", j, sensor_data.mpu9250.gyroscope[j]); 00186 pc.printf("\tmag[%d]: %.2f\r\n", j, sensor_data.mpu9250.magnetometer[j]); 00187 } 00188 pc.printf("GPS\r\n"); 00189 pc.printf("\tlat: %f%c\r\n", 00190 gps_decoder.get_latitude(), 00191 gps_decoder.get_latitude() >= 0 ? 'N' : 'S'); 00192 pc.printf("\tlon: %f%c\r\n", 00193 gps_decoder.get_longitude(), 00194 gps_decoder.get_longitude() >= 0 ? 'E' : 'W'); 00195 pc.printf("\tspeed: %fkm/h\r\n", gps_decoder.get_speed()); 00196 pc.printf("\tmove_direction: %f\r\n", gps_decoder.get_move_direction()); 00197 pc.printf("\tdate: %d/%02d/%02d %02d:%02d:%02d (UTC)\r\n", 00198 gps_decoder.get_year(), gps_decoder.get_month(), gps_decoder.get_day(), 00199 gps_decoder.get_hour(), gps_decoder.get_min(), gps_decoder.get_sec()); 00200 pc.printf("\tUNIX time: %d\r\n", gps_decoder.get_unixtime()); 00201 led_2 = 1; 00202 pc.printf("Send:%d\r\n", cnt_send); 00203 lcd.setCursor(0, 1); 00204 lcd.printf("%d", cnt_send); 00205 enqueue_sensor_data(cnt_send); 00206 sakuraio.send(); 00207 cnt_send++; 00208 led_2 = 0; 00209 pc.printf("After %d sec to send.\r\n", (int)(SEND_INTERVAL_TICKS_PAR_COUNT * 0.2)); 00210 } 00211 } 00212 led_1 = !led_1; 00213 led_4 = !sw_4; 00214 tick_by_200ms++; 00215 wait(0.2); 00216 } 00217 00218 00219 int main() 00220 { 00221 setup(); 00222 while(1) { 00223 loop(); 00224 } 00225 }
Generated on Sun Jul 17 2022 11:39:17 by
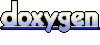