
Updated Nov. 18th.
Dependencies: SX1276Lib_modtronix
Fork of SX1276PingPong by
main.cpp
00001 #include "mbed.h" 00002 #include "sx1276Regs-LoRa.h" 00003 #include "sx1276-inAir.h" 00004 00005 #define DEBUG 1 00006 00007 #define RF_FREQUENCY 915000000 // 915MHz 00008 #define TX_OUTPUT_POWER 14 // 14 dBm for inAir9 00009 #define LORA_BANDWIDTH 7 // 0: 7.8 kHz, 1: 10.4 kHz, 2: 15.6kHz, 3: 20.8kHz, 00010 // 4: 31.25kHz, 5: 41.7 kHz, 6: 62.5 kHz, 00011 // 7: 125 kHz, 8: 250 kHz, 9: 500 kHz 00012 #define LORA_SPREADING_FACTOR 12 // SF7..SF12 00013 #define LORA_CODINGRATE 1 // 1=4/5, 2=4/6, 3=4/7, 4=4/8 00014 #define LORA_PREAMBLE_LENGTH 8 // Same for Tx and Rx 00015 #define LORA_SYMBOL_TIMEOUT 5 // Symbols 00016 #define LORA_FIX_LENGTH_PAYLOAD_ON false 00017 #define LORA_FHSS_ENABLED false 00018 #define LORA_NB_SYMB_HOP 4 00019 #define LORA_IQ_INVERSION_ON false 00020 #define LORA_CRC_ENABLED true 00021 00022 #define TX_TIMEOUT_VALUE 2000000 00023 #define RX_TIMEOUT_VALUE 3500000 // in us 00024 #define BUFFER_SIZE 32 // Define the payload size here 00025 00026 00027 void OnTxDone(void); 00028 void OnTxTimeout(void); 00029 void OnRxDone(uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr); 00030 void OnRxTimeout(void); 00031 void OnRxError(void); 00032 00033 Serial pc(USBTX, USBRX); //Use default TX and RX. Available via USB Com port when using PGM-NUCLEO programmer 00034 SX1276inAir radio(OnTxDone, OnTxTimeout, OnRxDone, OnRxTimeout, OnRxError, NULL, NULL); 00035 DigitalOut led(LED1); 00036 00037 uint8_t Buffer[BUFFER_SIZE]; 00038 uint16_t BufferSize = BUFFER_SIZE; 00039 int16_t LoRaRssi; 00040 int8_t LoRaSNR; 00041 volatile RadioState State = LOWPOWER; 00042 00043 const uint8_t PingMsg[] = "PING"; 00044 const uint8_t PongMsg[] = "PONG"; 00045 00046 int main() { 00047 wait_ms(500); // start delay 00048 00049 // configure uart port 00050 pc.baud(9600); 00051 pc.format(8, SerialBase::None, 1); 00052 pc.printf("PC printing enabled\n\r"); 00053 wait(2); 00054 // configure radio 00055 radio.SetBoardType(BOARD_INAIR9); // the correct hardware for our own board 00056 pc.printf("Board type set\n\r"); 00057 00058 /* 00059 while (true) { 00060 led = !led; 00061 pc.printf("loop reached\n\r"); 00062 wait(2); 00063 }*/ 00064 00065 led = 0; 00066 while (radio.Read(REG_VERSION) == 0x00) 00067 { 00068 pc.printf("Trying to connect to radio device\r\n"); 00069 wait_ms(200); 00070 } 00071 led = 1; 00072 00073 pc.printf("%u",radio.Read(REG_VERSION)); 00074 pc.printf("Radio is initialized\r\n"); 00075 00076 // set radio frequency 00077 radio.SetChannel(RF_FREQUENCY); 00078 00079 pc.printf("Freq set to 915 MHz\r\n"); 00080 00081 // setup the modern 00082 radio.SetTxConfig( 00083 MODEM_LORA, 00084 TX_OUTPUT_POWER, 00085 0, 00086 LORA_BANDWIDTH, 00087 LORA_SPREADING_FACTOR, 00088 LORA_CODINGRATE, 00089 LORA_PREAMBLE_LENGTH, 00090 LORA_FIX_LENGTH_PAYLOAD_ON, 00091 LORA_CRC_ENABLED, 00092 LORA_FHSS_ENABLED, 00093 LORA_NB_SYMB_HOP, 00094 LORA_IQ_INVERSION_ON, 00095 TX_TIMEOUT_VALUE 00096 ); 00097 radio.SetRxConfig( 00098 MODEM_LORA, 00099 LORA_BANDWIDTH, 00100 LORA_SPREADING_FACTOR, 00101 LORA_CODINGRATE, 00102 0, 00103 LORA_PREAMBLE_LENGTH, 00104 LORA_SYMBOL_TIMEOUT, 00105 LORA_FIX_LENGTH_PAYLOAD_ON, 00106 0, 00107 LORA_CRC_ENABLED, 00108 LORA_FHSS_ENABLED, 00109 LORA_NB_SYMB_HOP, 00110 LORA_IQ_INVERSION_ON, 00111 true 00112 ); 00113 pc.printf("TX and RX configured\r\n"); 00114 00115 uint8_t i; 00116 bool isMaster = true; 00117 00118 pc.printf("before .Rx\r\n"); 00119 radio.Rx(RX_TIMEOUT_VALUE); 00120 pc.printf("RX_TIMEOUT_VALUE RX set\r\n"); 00121 00122 State = RX_TIMEOUT; 00123 00124 while (1) 00125 { 00126 // Check for connection to radio module 00127 while (radio.Read(REG_VERSION) == 0x00) 00128 { 00129 led = !led; 00130 pc.printf("Reconnecting...\r\n"); 00131 wait_ms(200); 00132 } 00133 led = 1; 00134 00135 pc.printf("%s\r\n",State ); 00136 //pc.printf("About to check State value\r\n"); 00137 00138 switch(State) 00139 { 00140 case RX_DONE: 00141 if (isMaster) 00142 { 00143 if (BufferSize > 0) 00144 { 00145 if (strncmp((const char *)Buffer, (const char *)PongMsg, 4) == 0) 00146 { 00147 pc.printf("...Pong\r\n"); 00148 // send next ping frame 00149 strcpy((char *)Buffer, (char *)PingMsg); 00150 // fill the buffer with numbers for the payload 00151 for( i = 4; i < BufferSize; i++ ) 00152 { 00153 Buffer[i] = i - 4; 00154 } 00155 wait_ms( 10 ); 00156 radio.Send( Buffer, BufferSize ); 00157 } 00158 else if (strncmp( ( const char* )Buffer, ( const char* )PingMsg, 4 ) == 0 ) 00159 { 00160 // A master already exists then become a slave 00161 pc.printf("...Ping\r\n"); 00162 isMaster = false; 00163 // send the next pong frame 00164 strcpy( ( char* )Buffer, ( char* )PongMsg ); 00165 // We fill the buffer with numbers for the payload 00166 for( i = 4; i < BufferSize; i++ ) 00167 { 00168 Buffer[i] = i - 4; 00169 } 00170 wait_ms( 10 ); 00171 radio.Send( Buffer, BufferSize ); 00172 } 00173 else 00174 { 00175 isMaster = true; 00176 radio.Rx(RX_TIMEOUT_VALUE); 00177 } 00178 } 00179 } 00180 else 00181 { 00182 if (BufferSize > 0) 00183 { 00184 if( strncmp( ( const char* )Buffer, ( const char* )PingMsg, 4 ) == 0 ) 00185 { 00186 pc.printf( "...Ping\r\n"); 00187 // Send the reply to the PING string 00188 strcpy( ( char* )Buffer, ( char* )PongMsg ); 00189 // We fill the buffer with numbers for the payload 00190 for( i = 4; i < BufferSize; i++ ) 00191 { 00192 Buffer[i] = i - 4; 00193 } 00194 wait_ms( 10 ); 00195 radio.Send( Buffer, BufferSize ); 00196 } 00197 else // valid reception but not a PING as expected 00198 { // Set device as master and start again 00199 isMaster = true; 00200 radio.Rx( RX_TIMEOUT_VALUE ); 00201 } 00202 } 00203 } 00204 State = LOWPOWER; 00205 pc.printf( "\r\n"); 00206 break; 00207 00208 case TX_DONE: 00209 if (isMaster) 00210 { 00211 pc.printf("Ping...\r\n"); 00212 } 00213 else 00214 { 00215 pc.printf("Pong...\r\n"); 00216 } 00217 radio.Rx(RX_TIMEOUT_VALUE); 00218 State = LOWPOWER; 00219 break; 00220 00221 case RX_TIMEOUT: 00222 if( isMaster == true ) 00223 { 00224 pc.printf("trying to send ping\r\n"); 00225 // Send the next PING frame 00226 strcpy( ( char* )Buffer, ( char* )PingMsg ); 00227 for( i = 4; i < BufferSize; i++ ) 00228 { 00229 Buffer[i] = i - 4; 00230 } 00231 wait_ms( 10 ); 00232 radio.Send( Buffer, BufferSize ); 00233 } 00234 else 00235 { 00236 radio.Rx( RX_TIMEOUT_VALUE ); 00237 } 00238 State = LOWPOWER; 00239 break; 00240 00241 case TX_TIMEOUT: 00242 radio.Rx( RX_TIMEOUT_VALUE ); 00243 State = LOWPOWER; 00244 break; 00245 00246 case RX_ERROR: 00247 // We have received a Packet with a CRC error, send reply as if packet was correct 00248 if( isMaster == true ) 00249 { 00250 // Send the next PING frame 00251 strcpy( ( char* )Buffer, ( char* )PingMsg ); 00252 for( i = 4; i < BufferSize; i++ ) 00253 { 00254 Buffer[i] = i - 4; 00255 } 00256 wait_ms( 10 ); 00257 radio.Send( Buffer, BufferSize ); 00258 } 00259 else 00260 { 00261 // Send the next PONG frame 00262 strcpy( ( char* )Buffer, ( char* )PongMsg ); 00263 for( i = 4; i < BufferSize; i++ ) 00264 { 00265 Buffer[i] = i - 4; 00266 } 00267 wait_ms( 10 ); 00268 radio.Send( Buffer, BufferSize ); 00269 } 00270 State = LOWPOWER; 00271 break; 00272 00273 case LOWPOWER: 00274 break; 00275 00276 default: 00277 State = LOWPOWER; 00278 break; 00279 } 00280 } 00281 } 00282 00283 00284 void OnTxDone(void) 00285 { 00286 radio.Sleep(); 00287 State = TX_DONE; 00288 00289 #if DEBUG == 1 00290 pc.printf("OnTxDone\r\n"); 00291 #endif 00292 } 00293 00294 void OnTxTimeout(void) 00295 { 00296 radio.Sleep(); 00297 State = TX_TIMEOUT; 00298 00299 #if DEBUG == 1 00300 pc.printf("OnTxTimeout\r\n"); 00301 #endif 00302 } 00303 00304 void OnRxDone(uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr) 00305 { 00306 radio.Sleep(); 00307 BufferSize = size; 00308 memcpy(Buffer, payload, BufferSize); 00309 LoRaRssi = rssi; 00310 LoRaSNR = snr; 00311 State = RX_DONE; 00312 00313 #if DEBUG == 1 00314 pc.printf("OnRxDone\r\n"); 00315 #endif 00316 } 00317 00318 void OnRxTimeout(void) 00319 { 00320 radio.Sleep(); 00321 Buffer[BufferSize] = 0; 00322 State = RX_TIMEOUT; 00323 00324 #if DEBUG == 1 00325 pc.printf("OnRxTimeout\r\n"); 00326 #endif 00327 } 00328 00329 void OnRxError(void) 00330 { 00331 radio.Sleep(); 00332 State = RX_ERROR; 00333 00334 #if DEBUG == 1 00335 pc.printf("OnRxError\r\n"); 00336 #endif 00337 }
Generated on Wed Jul 13 2022 20:16:52 by
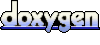