Programme d'utilisation servomotors MX12 V1
Embed:
(wiki syntax)
Show/hide line numbers
I2C.h
00001 /* mbed Microcontroller Library - I2C 00002 * Copyright (c) 2007-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_I2C_H 00006 #define MBED_I2C_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_I2C 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /* Class: I2C 00020 * An I2C Master, used for communicating with I2C slave devices 00021 * 00022 * Example: 00023 * > // Read from I2C slave at address 0x62 00024 * > 00025 * > #include "mbed.h" 00026 * > 00027 * > I2C i2c(p28, p27); 00028 * > 00029 * > int main() { 00030 * > int address = 0x62; 00031 * > char data[2]; 00032 * > i2c.read(address, data, 2); 00033 * > } 00034 */ 00035 class I2C : public Base { 00036 00037 public: 00038 00039 enum RxStatus { 00040 NoData 00041 , MasterGeneralCall 00042 , MasterWrite 00043 , MasterRead 00044 }; 00045 00046 enum Acknowledge { 00047 NoACK = 0 00048 , ACK = 1 00049 }; 00050 00051 /* Constructor: I2C 00052 * Create an I2C Master interface, connected to the specified pins 00053 * 00054 * Variables: 00055 * sda - I2C data line pin 00056 * scl - I2C clock line pin 00057 */ 00058 I2C(PinName sda, PinName scl, const char *name = NULL); 00059 00060 /* Function: frequency 00061 * Set the frequency of the I2C interface 00062 * 00063 * Variables: 00064 * hz - The bus frequency in hertz 00065 */ 00066 void frequency(int hz); 00067 00068 /* Function: read 00069 * Read from an I2C slave 00070 * 00071 * Performs a complete read transaction. The bottom bit of 00072 * the address is forced to 1 to indicate a read. 00073 * 00074 * Variables: 00075 * address - 8-bit I2C slave address [ addr | 1 ] 00076 * data - Pointer to the byte-array to read data in to 00077 * length - Number of bytes to read 00078 * repeated - Repeated start, true - don't send stop at end 00079 * returns - 0 on success (ack), or non-0 on failure (nack) 00080 */ 00081 int read(int address, char *data, int length, bool repeated = false); 00082 00083 /* Function: read 00084 * Read a single byte from the I2C bus 00085 * 00086 * Variables: 00087 * ack - indicates if the byte is to be acknowledged (1 = acknowledge) 00088 * returns - the byte read 00089 */ 00090 int read(int ack); 00091 00092 /* Function: write 00093 * Write to an I2C slave 00094 * 00095 * Performs a complete write transaction. The bottom bit of 00096 * the address is forced to 0 to indicate a write. 00097 * 00098 * Variables: 00099 * address - 8-bit I2C slave address [ addr | 0 ] 00100 * data - Pointer to the byte-array data to send 00101 * length - Number of bytes to send 00102 * repeated - Repeated start, true - do not send stop at end 00103 * returns - 0 on success (ack), or non-0 on failure (nack) 00104 */ 00105 int write(int address, const char *data, int length, bool repeated = false); 00106 00107 /* Function: write 00108 * Write single byte out on the I2C bus 00109 * 00110 * Variables: 00111 * data - data to write out on bus 00112 * returns - a '1' if an ACK was received, a '0' otherwise 00113 */ 00114 int write(int data); 00115 00116 /* Function: start 00117 * Creates a start condition on the I2C bus 00118 */ 00119 00120 void start(void); 00121 00122 /* Function: stop 00123 * Creates a stop condition on the I2C bus 00124 */ 00125 void stop(void); 00126 00127 protected: 00128 00129 void aquire(); 00130 00131 I2CName _i2c; 00132 static I2C *_owner; 00133 int _hz; 00134 00135 }; 00136 00137 } // namespace mbed 00138 00139 #endif 00140 00141 #endif 00142
Generated on Wed Jul 13 2022 21:22:54 by
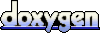