Programme d'utilisation servomotors MX12 V1
Embed:
(wiki syntax)
Show/hide line numbers
I2CSlave.h
00001 /* mbed Microcontroller Library - I2CSlave 00002 * Copyright (c) 2007-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_I2C_SLAVE_H 00006 #define MBED_I2C_SLAVE_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_I2CSLAVE 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /* Class: I2CSlave 00020 * An I2C Slave, used for communicating with an I2C Master device 00021 * 00022 * Example: 00023 * > // Simple I2C responder 00024 * > #include <mbed.h> 00025 * > 00026 * > I2CSlave slave(p9, p10); 00027 * > 00028 * > int main() { 00029 * > char buf[10]; 00030 * > char msg[] = "Slave!"; 00031 * > 00032 * > slave.address(0xA0); 00033 * > while (1) { 00034 * > int i = slave.receive(); 00035 * > switch (i) { 00036 * > case I2CSlave::ReadAddressed: 00037 * > slave.write(msg, strlen(msg) + 1); // Includes null char 00038 * > break; 00039 * > case I2CSlave::WriteGeneral: 00040 * > slave.read(buf, 10); 00041 * > printf("Read G: %s\n", buf); 00042 * > break; 00043 * > case I2CSlave::WriteAddressed: 00044 * > slave.read(buf, 10); 00045 * > printf("Read A: %s\n", buf); 00046 * > break; 00047 * > } 00048 * > for(int i = 0; i < 10; i++) buf[i] = 0; // Clear buffer 00049 * > } 00050 * > } 00051 * > 00052 */ 00053 class I2CSlave : public Base { 00054 00055 public: 00056 00057 enum RxStatus { 00058 NoData = 0 00059 , ReadAddressed = 1 00060 , WriteGeneral = 2 00061 , WriteAddressed = 3 00062 }; 00063 00064 /* Constructor: I2CSlave 00065 * Create an I2C Slave interface, connected to the specified pins. 00066 * 00067 * Variables: 00068 * sda - I2C data line pin 00069 * scl - I2C clock line pin 00070 */ 00071 I2CSlave(PinName sda, PinName scl, const char *name = NULL); 00072 00073 /* Function: frequency 00074 * Set the frequency of the I2C interface 00075 * 00076 * Variables: 00077 * hz - The bus frequency in hertz 00078 */ 00079 void frequency(int hz); 00080 00081 /* Function: receive 00082 * Checks to see if this I2C Slave has been addressed. 00083 * 00084 * Variables: 00085 * returns - a status indicating if the device has been addressed, and how 00086 * > NoData - the slave has not been addressed 00087 * > ReadAddressed - the master has requested a read from this slave 00088 * > WriteAddressed - the master is writing to this slave 00089 * > WriteGeneral - the master is writing to all slave 00090 */ 00091 int receive(void); 00092 00093 /* Function: read 00094 * Read from an I2C master. 00095 * 00096 * Variables: 00097 * data - pointer to the byte array to read data in to 00098 * length - maximum number of bytes to read 00099 * returns - 0 on success, non-0 otherwise 00100 */ 00101 int read(char *data, int length); 00102 00103 /* Function: read 00104 * Read a single byte from an I2C master. 00105 * 00106 * Variables: 00107 * returns - the byte read 00108 */ 00109 int read(void); 00110 00111 /* Function: write 00112 * Write to an I2C master. 00113 * 00114 * Variables: 00115 * data - pointer to the byte array to be transmitted 00116 * length - the number of bytes to transmite 00117 * returns - a 0 on success, non-0 otherwise 00118 */ 00119 int write(const char *data, int length); 00120 00121 /* Function: write 00122 * Write a single byte to an I2C master. 00123 * 00124 * Variables 00125 * data - the byte to write 00126 * returns - a '1' if an ACK was received, a '0' otherwise 00127 */ 00128 int write(int data); 00129 00130 /* Function: address 00131 * Sets the I2C slave address. 00132 * 00133 * Variables 00134 * address - the address to set for the slave (ignoring the least 00135 * signifcant bit). If set to 0, the slave will only respond to the 00136 * general call address. 00137 */ 00138 void address(int address); 00139 00140 /* Function: stop 00141 * Reset the I2C slave back into the known ready receiving state. 00142 */ 00143 void stop(void); 00144 00145 protected: 00146 00147 I2CName _i2c; 00148 }; 00149 00150 } // namespace mbed 00151 00152 #endif 00153 00154 #endif 00155
Generated on Wed Jul 13 2022 21:22:54 by
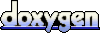