Programme d'utilisation servomotors MX12 V1
Embed:
(wiki syntax)
Show/hide line numbers
FileSystemLike.h
00001 /* mbed Microcontroller Library - FileSystemLike 00002 * Copyright (c) 2008-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_FILESYSTEMLIKE_H 00006 #define MBED_FILESYSTEMLIKE_H 00007 00008 #ifdef __ARMCC_VERSION 00009 # define O_RDONLY 0 00010 # define O_WRONLY 1 00011 # define O_RDWR 2 00012 # define O_CREAT 0x0200 00013 # define O_TRUNC 0x0400 00014 # define O_APPEND 0x0008 00015 typedef int mode_t; 00016 #else 00017 # include <sys/fcntl.h> 00018 #endif 00019 #include "Base.h" 00020 #include "FileHandle.h" 00021 #include "DirHandle.h" 00022 00023 namespace mbed { 00024 00025 /* Class FileSystemLike 00026 * A filesystem-like object is one that can be used to open files 00027 * though it by fopen("/name/filename", mode) 00028 * 00029 * Implementations must define at least open (the default definitions 00030 * of the rest of the functions just return error values). 00031 */ 00032 class FileSystemLike : public Base { 00033 00034 public: 00035 00036 /* Constructor FileSystemLike 00037 * 00038 * Variables 00039 * name - The name to use for the filesystem. 00040 */ 00041 FileSystemLike(const char *name) : Base(name) {} 00042 00043 /* Function open 00044 * 00045 * Variables 00046 * filename - The name of the file to open. 00047 * flags - One of O_RDONLY, O_WRONLY, or O_RDWR, OR'd with 00048 * zero or more of O_CREAT, O_TRUNC, or O_APPEND. 00049 * returns - A pointer to a FileHandle object representing the 00050 * file on success, or NULL on failure. 00051 */ 00052 virtual FileHandle *open(const char *filename, int flags) = 0; 00053 00054 /* Function remove 00055 * Remove a file from the filesystem. 00056 * 00057 * Variables 00058 * filename - the name of the file to remove. 00059 * returns - 0 on success, -1 on failure. 00060 */ 00061 virtual int remove(const char *filename) { return -1; }; 00062 00063 /* Function rename 00064 * Rename a file in the filesystem. 00065 * 00066 * Variables 00067 * oldname - the name of the file to rename. 00068 * newname - the name to rename it to. 00069 * returns - 0 on success, -1 on failure. 00070 */ 00071 virtual int rename(const char *oldname, const char *newname) { return -1; }; 00072 00073 /* Function opendir 00074 * Opens a directory in the filesystem and returns a DirHandle 00075 * representing the directory stream. 00076 * 00077 * Variables 00078 * name - The name of the directory to open. 00079 * returns - A DirHandle representing the directory stream, or 00080 * NULL on failure. 00081 */ 00082 virtual DirHandle *opendir(const char *name) { return NULL; }; 00083 00084 /* Function mkdir 00085 * Creates a directory in the filesystem. 00086 * 00087 * Variables 00088 * name - The name of the directory to create. 00089 * mode - The permissions to create the directory with. 00090 * returns - 0 on success, -1 on failure. 00091 */ 00092 virtual int mkdir(const char *name, mode_t mode) { return -1; } 00093 00094 // TODO other filesystem functions (mkdir, rm, rn, ls etc) 00095 00096 }; 00097 00098 } // namespace mbed 00099 00100 #endif
Generated on Wed Jul 13 2022 21:22:54 by
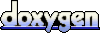