Programme d'utilisation servomotors MX12 V1
Embed:
(wiki syntax)
Show/hide line numbers
CAN.h
00001 /* mbed Microcontroller Library - can 00002 * Copyright (c) 2009-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_CAN_H 00006 #define MBED_CAN_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_CAN 00011 00012 #include "Base.h" 00013 #include "platform.h" 00014 #include "PinNames.h" 00015 #include "PeripheralNames.h" 00016 00017 #include "can_helper.h" 00018 #include "FunctionPointer.h" 00019 00020 #include <string.h> 00021 00022 namespace mbed { 00023 00024 /* Class: CANMessage 00025 * 00026 */ 00027 class CANMessage : public CAN_Message { 00028 00029 public: 00030 00031 /* Constructor: CANMessage 00032 * Creates empty CAN message. 00033 */ 00034 CANMessage() { 00035 len = 8; 00036 type = CANData; 00037 format = CANStandard; 00038 id = 0; 00039 memset(data, 0, 8); 00040 } 00041 00042 /* Constructor: CANMessage 00043 * Creates CAN message with specific content. 00044 */ 00045 CANMessage(int _id, const char *_data, char _len = 8, CANType _type = CANData, CANFormat _format = CANStandard) { 00046 len = _len & 0xF; 00047 type = _type; 00048 format = _format; 00049 id = _id; 00050 memcpy(data, _data, _len); 00051 } 00052 00053 /* Constructor: CANMessage 00054 * Creates CAN remote message. 00055 */ 00056 CANMessage(int _id, CANFormat _format = CANStandard) { 00057 len = 0; 00058 type = CANRemote; 00059 format = _format; 00060 id = _id; 00061 memset(data, 0, 8); 00062 } 00063 #if 0 // Inhereted from CAN_Message, for documentation only 00064 00065 /* Variable: id 00066 * The message id. 00067 * 00068 * If format is CANStandard it must be an 11 bit long id 00069 * If format is CANExtended it must be an 29 bit long id 00070 */ 00071 unsigned int id; 00072 00073 /* Variable: data 00074 * Space for 8 byte payload. 00075 * 00076 * If type is CANData data can store up to 8 byte data. 00077 */ 00078 unsigned char data[8]; 00079 00080 /* Variable: len 00081 * Length of data in bytes. 00082 * 00083 * If type is CANData data can store up to 8 byte data. 00084 */ 00085 unsigned char len; 00086 00087 /* Variable: format 00088 * Defines if the message has standard or extended format. 00089 * 00090 * Defines the type of message id: 00091 * Default is CANStandard which implies 11 bit id. 00092 * CANExtended means 29 bit message id. 00093 */ 00094 CANFormat format; 00095 00096 /* Variable: type 00097 * Defines the type of a message. 00098 * 00099 * The message type can rather be CANData for a message with data (default). 00100 * Or CANRemote for a request of a specific CAN message. 00101 */ 00102 CANType type; // 0 - DATA FRAME, 1 - REMOTE FRAME 00103 #endif 00104 }; 00105 00106 /* Class: CAN 00107 * A can bus client, used for communicating with can devices 00108 */ 00109 class CAN : public Base { 00110 00111 public: 00112 00113 /* Constructor: CAN 00114 * Creates an CAN interface connected to specific pins. 00115 * 00116 * Example: 00117 * > #include "mbed.h" 00118 * > 00119 * > Ticker ticker; 00120 * > DigitalOut led1(LED1); 00121 * > DigitalOut led2(LED2); 00122 * > CAN can1(p9, p10); 00123 * > CAN can2(p30, p29); 00124 * > 00125 * > char counter = 0; 00126 * > 00127 * > void send() { 00128 * > if(can1.write(CANMessage(1337, &counter, 1))) { 00129 * > printf("Message sent: %d\n", counter); 00130 * > counter++; 00131 * > } 00132 * > led1 = !led1; 00133 * > } 00134 * > 00135 * > int main() { 00136 * > ticker.attach(&send, 1); 00137 * > CANMessage msg; 00138 * > while(1) { 00139 * > if(can2.read(msg)) { 00140 * > printf("Message received: %d\n\n", msg.data[0]); 00141 * > led2 = !led2; 00142 * > } 00143 * > wait(0.2); 00144 * > } 00145 * > } 00146 * 00147 * Variables: 00148 * rd - read from transmitter 00149 * td - transmit to transmitter 00150 */ 00151 CAN(PinName rd, PinName td); 00152 virtual ~CAN(); 00153 00154 /* Function: frequency 00155 * Set the frequency of the CAN interface 00156 * 00157 * Variables: 00158 * hz - The bus frequency in hertz 00159 * returns - 1 if successful, 0 otherwise 00160 */ 00161 int frequency(int hz); 00162 00163 /* Function: write 00164 * Write a CANMessage to the bus. 00165 * 00166 * Variables: 00167 * msg - The CANMessage to write. 00168 * 00169 * Returns: 00170 * 0 - If write failed. 00171 * 1 - If write was successful. 00172 */ 00173 int write(CANMessage msg); 00174 00175 /* Function: read 00176 * Read a CANMessage from the bus. 00177 * 00178 * Variables: 00179 * msg - A CANMessage to read to. 00180 * 00181 * Returns: 00182 * 0 - If no message arrived. 00183 * 1 - If message arrived. 00184 */ 00185 int read(CANMessage &msg); 00186 00187 /* Function: reset 00188 * Reset CAN interface. 00189 * 00190 * To use after error overflow. 00191 */ 00192 void reset(); 00193 00194 /* Function: monitor 00195 * Puts or removes the CAN interface into silent monitoring mode 00196 * 00197 * Variables: 00198 * silent - boolean indicating whether to go into silent mode or not 00199 */ 00200 void monitor(bool silent); 00201 00202 /* Function: rderror 00203 * Returns number of read errors to detect read overflow errors. 00204 */ 00205 unsigned char rderror(); 00206 00207 /* Function: tderror 00208 * Returns number of write errors to detect write overflow errors. 00209 */ 00210 unsigned char tderror(); 00211 00212 /* Function: attach 00213 * Attach a function to call whenever a CAN frame received interrupt is 00214 * generated. 00215 * 00216 * Variables: 00217 * fptr - A pointer to a void function, or 0 to set as none 00218 */ 00219 void attach(void (*fptr)(void)); 00220 00221 /* Function attach 00222 * Attach a member function to call whenever a CAN frame received interrupt 00223 * is generated. 00224 * 00225 * Variables: 00226 * tptr - pointer to the object to call the member function on 00227 * mptr - pointer to the member function to be called 00228 */ 00229 template<typename T> 00230 void attach(T* tptr, void (T::*mptr)(void)) { 00231 if((mptr != NULL) && (tptr != NULL)) { 00232 _rxirq.attach(tptr, mptr); 00233 setup_interrupt(); 00234 } else { 00235 remove_interrupt(); 00236 } 00237 } 00238 00239 private: 00240 00241 CANName _id; 00242 FunctionPointer _rxirq; 00243 00244 void setup_interrupt(void); 00245 void remove_interrupt(void); 00246 }; 00247 00248 } // namespace mbed 00249 00250 #endif // MBED_CAN_H 00251 00252 #endif
Generated on Wed Jul 13 2022 21:22:54 by
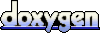