code de start qui marche a la fin du premier match, base pour la suite
Fork of CRAC-Strat_2017_homologation_petit_rob by
Strategie_big.cpp
00001 00002 #include "StrategieManager.h" 00003 #ifdef ROBOT_BIG 00004 #include "Config_big.h" 00005 00006 unsigned char isStopEnable = 1;//Permet de savoir si il faut autoriser le stop via les balises 00007 00008 /****************************************************************************************/ 00009 /* FUNCTION NAME: doFunnyAction */ 00010 /* DESCRIPTION : Permet de faire la funny action en fin de partie */ 00011 /****************************************************************************************/ 00012 void doFunnyAction(void) { 00013 //envoie de la funny action 00014 // 0x007, 01, 01 00015 CANMessage msgTx=CANMessage(); 00016 msgTx.id=GLOBAL_FUNNY_ACTION; 00017 msgTx.format=CANStandard; 00018 msgTx.type=CANData; 00019 msgTx.len=2; 00020 msgTx.data[0]=0x01; 00021 msgTx.data[1]=0x01; 00022 can1.write(msgTx); 00023 } 00024 00025 /****************************************************************************************/ 00026 /* FUNCTION NAME: doAction */ 00027 /* DESCRIPTION : Effectuer une action specifique */ 00028 /****************************************************************************************/ 00029 unsigned char doAction(unsigned char id, unsigned short speed, short angle) { 00030 CANMessage msgTx=CANMessage(); 00031 int localData = 0, localData2; 00032 switch(id) { 00033 case 100: // position initiale 00034 msgTx.id=SERVO_AX12_ACTION; 00035 msgTx.format=CANStandard; 00036 msgTx.type=CANData; 00037 msgTx.len=2; 00038 00039 if (InversStrat){ // si on est inversé, on echange les bras 00040 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00041 else speed = BRAS_AVANT; 00042 } 00043 00044 msgTx.data[0]=1; 00045 msgTx.data[1]=speed; 00046 can1.write(msgTx); 00047 break; 00048 00049 case 101: /// preparation prise 00050 msgTx.id=SERVO_AX12_ACTION; 00051 msgTx.format=CANStandard; 00052 msgTx.type=CANData; 00053 msgTx.len=2; 00054 00055 if (InversStrat){ // si on est inversé, on echange les bras 00056 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00057 else speed = BRAS_AVANT; 00058 } 00059 00060 msgTx.data[0]=2; 00061 msgTx.data[1]=speed; 00062 can1.write(msgTx); 00063 break; 00064 00065 case 102: // stockage haut 00066 msgTx.id=SERVO_AX12_ACTION; 00067 msgTx.format=CANStandard; 00068 msgTx.type=CANData; 00069 msgTx.len=2; 00070 00071 if (InversStrat){ // si on est inversé, on echange les bras 00072 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00073 else speed = BRAS_AVANT; 00074 } 00075 00076 msgTx.data[0]=3; 00077 msgTx.data[1]=speed; 00078 can1.write(msgTx); 00079 break; 00080 00081 case 103: // stockage bas 00082 msgTx.id=SERVO_AX12_ACTION; 00083 msgTx.format=CANStandard; 00084 msgTx.type=CANData; 00085 msgTx.len=2; 00086 00087 if (InversStrat){ // si on est inversé, on echange les bras 00088 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00089 else speed = BRAS_AVANT; 00090 } 00091 00092 msgTx.data[0]=4; 00093 msgTx.data[1]=speed; 00094 can1.write(msgTx); 00095 break; 00096 00097 case 104: // pousser module 00098 msgTx.id=SERVO_AX12_ACTION; 00099 msgTx.format=CANStandard; 00100 msgTx.type=CANData; 00101 msgTx.len=2; 00102 00103 if (InversStrat){ // si on est inversé, on echange les bras 00104 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00105 else speed = BRAS_AVANT; 00106 } 00107 00108 msgTx.data[0]=7; 00109 msgTx.data[1]=speed; 00110 can1.write(msgTx); 00111 break; 00112 00113 case 105: // preparation prise 00114 msgTx.id=SERVO_AX12_ACTION; 00115 msgTx.format=CANStandard; 00116 msgTx.type=CANData; 00117 msgTx.len=2; 00118 00119 if (InversStrat){ // si on est inversé, on echange les bras 00120 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00121 else speed = BRAS_AVANT; 00122 } 00123 00124 msgTx.data[0]=6; 00125 msgTx.data[1]=speed; 00126 can1.write(msgTx); 00127 break; 00128 00129 case 106: //deposer module 00130 msgTx.id=SERVO_AX12_ACTION; 00131 msgTx.format=CANStandard; 00132 msgTx.type=CANData; 00133 msgTx.len=2; 00134 00135 if (InversStrat){ // si on est inversé, on echange les bras 00136 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00137 else speed = BRAS_AVANT; 00138 } 00139 00140 msgTx.data[0]=5; 00141 msgTx.data[1]=speed; 00142 can1.write(msgTx); 00143 break; 00144 00145 case 110: // repos bras avant 00146 msgTx.id=SERVO_AX12_ACTION; 00147 msgTx.format=CANStandard; 00148 msgTx.type=CANData; 00149 msgTx.len=2; 00150 speed = BRAS_AVANT; 00151 if (InversStrat){ // si on est inversé, on echange les bras 00152 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00153 else speed = BRAS_AVANT; 00154 } 00155 if (speed == BRAS_AVANT)localData = 0x10; 00156 else localData = 0x24; 00157 00158 msgTx.data[0]=localData; 00159 msgTx.data[1]=speed; 00160 can1.write(msgTx); 00161 break; 00162 00163 case 111: // init bras module 00164 msgTx.id=SERVO_AX12_ACTION; 00165 msgTx.format=CANStandard; 00166 msgTx.type=CANData; 00167 msgTx.len=2; 00168 speed = BRAS_AVANT; 00169 if (InversStrat){ // si on est inversé, on echange les bras 00170 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00171 else speed = BRAS_AVANT; 00172 } 00173 if (speed == BRAS_AVANT)localData = 0x0A; 00174 else localData = 0x1E; 00175 00176 msgTx.data[0]=localData; 00177 msgTx.data[1]=speed; 00178 can1.write(msgTx); 00179 break; 00180 00181 case 112: // bouger module avant la gouttière 00182 msgTx.id=SERVO_AX12_ACTION; 00183 msgTx.format=CANStandard; 00184 msgTx.type=CANData; 00185 msgTx.len=2; 00186 speed = BRAS_AVANT; 00187 if (InversStrat){ // si on est inversé, on echange les bras 00188 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00189 else speed = BRAS_AVANT; 00190 } 00191 if (speed == BRAS_AVANT)localData = 0x0B; 00192 else localData = 0x1F; 00193 00194 msgTx.data[0]=localData; 00195 msgTx.data[1]=speed; 00196 can1.write(msgTx); 00197 break; 00198 00199 case 113: // deposer module 00200 msgTx.id=SERVO_AX12_ACTION; 00201 msgTx.format=CANStandard; 00202 msgTx.type=CANData; 00203 msgTx.len=2; 00204 localData2 = BRAS_AVANT; 00205 00206 if (InversStrat){ // si on est inversé, on echange les bras 00207 if (localData2 == BRAS_AVANT) localData2 = BRAS_ARRIERE; 00208 else localData2 = BRAS_AVANT; 00209 } 00210 00211 if(localData2 == BRAS_AVANT){ 00212 if(speed == INV){ 00213 localData = 0x0E; 00214 }else{ 00215 localData = 0x0C; 00216 } 00217 }else{ 00218 if(speed == INV){ 00219 localData = 0x22; 00220 }else{ 00221 localData = 0x20; 00222 } 00223 } 00224 00225 msgTx.data[0]=localData; 00226 msgTx.data[1]=localData2; 00227 can1.write(msgTx); 00228 break; 00229 00230 case 114: // repositionner bras avant 00231 msgTx.id=SERVO_AX12_ACTION; 00232 msgTx.format=CANStandard; 00233 msgTx.type=CANData; 00234 msgTx.len=2; 00235 speed = BRAS_AVANT; 00236 if (InversStrat){ // si on est inversé, on echange les bras 00237 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00238 else speed = BRAS_AVANT; 00239 } 00240 if (speed == BRAS_AVANT)localData = 0x0F; 00241 else localData = 0x23; 00242 00243 msgTx.data[0]=localData; 00244 msgTx.data[1]=speed; 00245 can1.write(msgTx); 00246 break; 00247 00248 case 115: // pompe avant ON 00249 msgTx.id=0x400; 00250 msgTx.format=CANStandard; 00251 msgTx.type=CANData; 00252 msgTx.len=2; 00253 localData = BRAS_AVANT; 00254 if (InversStrat){ // si on est inversé, on echange les bras 00255 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00256 else localData = BRAS_AVANT; 00257 } 00258 00259 msgTx.data[0]=1; 00260 msgTx.data[1]=localData; 00261 00262 can1.write(msgTx); 00263 wait_ms(1); 00264 can1.write(msgTx); 00265 break; 00266 00267 case 116: // pompe avant OFF 00268 msgTx.id=0x400; 00269 msgTx.format=CANStandard; 00270 msgTx.type=CANData; 00271 msgTx.len=2; 00272 localData = BRAS_AVANT; 00273 if (InversStrat){ // si on est inversé, on echange les bras 00274 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00275 else localData = BRAS_AVANT; 00276 } 00277 00278 msgTx.data[0]=0; 00279 msgTx.data[1]=localData; 00280 00281 can1.write(msgTx); 00282 wait_ms(1); 00283 can1.write(msgTx); 00284 break; 00285 00286 case 120: // repos bras arrière 00287 msgTx.id=SERVO_AX12_ACTION; 00288 msgTx.format=CANStandard; 00289 msgTx.type=CANData; 00290 msgTx.len=2; 00291 speed = BRAS_ARRIERE; 00292 if (InversStrat){ // si on est inversé, on echange les bras 00293 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00294 else speed = BRAS_AVANT; 00295 } 00296 if (speed == BRAS_AVANT)localData = 0x10; 00297 else localData = 0x24; 00298 00299 msgTx.data[0]=localData; 00300 msgTx.data[1]=speed; 00301 can1.write(msgTx); 00302 break; 00303 00304 case 121: // position d'init avant de prendre un module bras arriere 00305 msgTx.id=SERVO_AX12_ACTION; 00306 msgTx.format=CANStandard; 00307 msgTx.type=CANData; 00308 msgTx.len=2; 00309 speed = BRAS_ARRIERE; 00310 if (InversStrat){ // si on est inversé, on echange les bras 00311 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00312 else speed = BRAS_AVANT; 00313 } 00314 if (speed == BRAS_AVANT)localData = 0xA0; 00315 else localData = 0x1E; 00316 00317 msgTx.data[0]=localData; 00318 msgTx.data[1]=speed; 00319 can1.write(msgTx); 00320 break; 00321 00322 case 122: // bouger le bras arriere avant la gouttiere 00323 msgTx.id=SERVO_AX12_ACTION; 00324 msgTx.format=CANStandard; 00325 msgTx.type=CANData; 00326 msgTx.len=2; 00327 speed = BRAS_ARRIERE; 00328 if (InversStrat){ // si on est inversé, on echange les bras 00329 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00330 else speed = BRAS_AVANT; 00331 } 00332 if (speed == BRAS_AVANT)localData = 0x0B; 00333 else localData = 0x1F; 00334 00335 msgTx.data[0]=localData; 00336 msgTx.data[1]=speed; 00337 can1.write(msgTx); 00338 break; 00339 00340 case 123: // deposer module 00341 msgTx.id=SERVO_AX12_ACTION; 00342 msgTx.format=CANStandard; 00343 msgTx.type=CANData; 00344 msgTx.len=2; 00345 localData2 = BRAS_ARRIERE; 00346 00347 if (InversStrat){ // si on est inversé, on echange les bras 00348 if (localData2 == BRAS_AVANT) localData2 = BRAS_ARRIERE; 00349 else localData2 = BRAS_AVANT; 00350 } 00351 00352 if(localData2 == BRAS_AVANT){ 00353 if(speed == INV){ 00354 localData = 0x0E; 00355 }else{ 00356 localData = 0x0C; 00357 } 00358 }else{ 00359 if(speed == INV){ 00360 localData = 0x22; 00361 }else{ 00362 localData = 0x20; 00363 } 00364 } 00365 00366 msgTx.data[0]=localData; 00367 msgTx.data[1]=speed; 00368 can1.write(msgTx); 00369 break; 00370 00371 case 124: // remettre le bras arriere en posiion initiale 00372 msgTx.id=SERVO_AX12_ACTION; 00373 msgTx.format=CANStandard; 00374 msgTx.type=CANData; 00375 msgTx.len=2; 00376 speed = BRAS_ARRIERE; 00377 if (InversStrat){ // si on est inversé, on echange les bras 00378 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00379 else speed = BRAS_AVANT; 00380 } 00381 if (speed == BRAS_AVANT)localData = 0x0F; 00382 else localData = 0x23; 00383 00384 msgTx.data[0]=localData; 00385 msgTx.data[1]=speed; 00386 can1.write(msgTx); 00387 break; 00388 00389 case 125: // pompe pwm arriere ON 00390 msgTx.id=0x400; 00391 msgTx.format=CANStandard; 00392 msgTx.type=CANData; 00393 msgTx.len=2; 00394 localData = BRAS_ARRIERE; 00395 if (InversStrat){ // si on est inversé, on echange les bras 00396 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00397 else localData = BRAS_AVANT; 00398 } 00399 00400 msgTx.data[0]=1; 00401 msgTx.data[1]=localData; 00402 00403 can1.write(msgTx); 00404 wait_ms(1); 00405 can1.write(msgTx); 00406 break; 00407 00408 case 126: // pompe arriere pwm OFF 00409 msgTx.id=0x400; 00410 msgTx.format=CANStandard; 00411 msgTx.type=CANData; 00412 msgTx.len=2; 00413 localData = BRAS_ARRIERE; 00414 if (InversStrat){ // si on est inversé, on echange les bras 00415 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00416 else localData = BRAS_AVANT; 00417 } 00418 00419 msgTx.data[0]=0; 00420 msgTx.data[1]=localData; 00421 00422 can1.write(msgTx); 00423 wait_ms(1); 00424 can1.write(msgTx); 00425 break; 00426 00427 case 130: // baiser bras turbine 00428 msgTx.id=SERVO_AX12_ACTION; 00429 msgTx.format=CANStandard; 00430 msgTx.type=CANData; 00431 msgTx.len=2; 00432 msgTx.data[0]=0x14; 00433 msgTx.data[1]=BRAS_AVANT; 00434 can1.write(msgTx); 00435 break; 00436 00437 case 131: // lever bras turbine 00438 msgTx.id=SERVO_AX12_ACTION; 00439 msgTx.format=CANStandard; 00440 msgTx.type=CANData; 00441 msgTx.len=2; 00442 msgTx.data[0]=0x15; 00443 msgTx.data[1]=BRAS_AVANT; 00444 can1.write(msgTx); 00445 break; 00446 00447 case 132: // allumer turbine 00448 msgTx.id=0x403; 00449 msgTx.format=CANStandard; 00450 msgTx.type=CANData; 00451 msgTx.len=2; 00452 msgTx.data[0]=1; 00453 msgTx.data[1]=BRAS_AVANT; 00454 can1.write(msgTx); 00455 break; 00456 00457 case 133: // stop turbine 00458 msgTx.id=0x403; 00459 msgTx.format=CANStandard; 00460 msgTx.type=CANData; 00461 msgTx.len=2; 00462 msgTx.data[0]=0; 00463 msgTx.data[1]=BRAS_AVANT; 00464 can1.write(msgTx); 00465 break; 00466 00467 case 140:// lanceur ON 00468 msgTx.id=0x402; 00469 msgTx.format=CANStandard; 00470 msgTx.type=CANData; 00471 msgTx.len=2; 00472 00473 msgTx.data[0]=1; 00474 msgTx.data[1]=0x02; 00475 can1.write(msgTx); 00476 break; 00477 00478 case 141: // lanceur OFF 00479 msgTx.id=0x402; 00480 msgTx.format=CANStandard; 00481 msgTx.type=CANData; 00482 msgTx.len=2; 00483 00484 msgTx.data[0]=0; 00485 msgTx.data[1]=0x02; 00486 can1.write(msgTx); 00487 break; 00488 00489 case 150: 00490 msgTx.id=0x404; 00491 msgTx.format=CANStandard; 00492 msgTx.type=CANData; 00493 msgTx.len=2; 00494 00495 msgTx.data[0]=1; 00496 msgTx.data[1]=BRAS_AVANT; 00497 00498 can1.write(msgTx); 00499 break; 00500 00501 case 151: 00502 msgTx.id=0x404; 00503 msgTx.format=CANStandard; 00504 msgTx.type=CANData; 00505 msgTx.len=2; 00506 00507 msgTx.data[0]=0; 00508 msgTx.data[1]=BRAS_AVANT; 00509 00510 can1.write(msgTx); 00511 break; 00512 00513 case 10://Désactiver le stop 00514 isStopEnable = 0; 00515 break; 00516 case 11://Activer le stop 00517 isStopEnable = 1; 00518 break; 00519 case 20://Désactiver l'asservissement 00520 setAsservissementEtat(0); 00521 break; 00522 case 21://Activer l'asservissement 00523 setAsservissementEtat(1); 00524 break; 00525 00526 case 22://Changer la vitesse du robot 00527 SendSpeed(speed,(unsigned short)angle); 00528 break; 00529 00530 case 30://Action tempo 00531 wait_ms(speed); 00532 break; 00533 00534 /*case 40: // demande au telemetre la position d'un objet 00535 //SendRawId(TELEMETRE_RECHERCHE_OBJET); 00536 00537 modeTelemetre = 1; 00538 00539 //angle = angle /10; 00540 00541 msgTx.id=TELEMETRE_OBJET; 00542 msgTx.format=CANStandard; 00543 msgTx.type=CANData; 00544 msgTx.len=1; 00545 // indice du module sur le terrain 00546 msgTx.data[0] = (unsigned char)speed; 00547 00548 00549 // x sur 2 octets 00550 msgTx.data[0]=(unsigned char)speed; 00551 msgTx.data[1]=(unsigned char)(speed>>8); 00552 // y sur 2 octets 00553 msgTx.data[2]=(unsigned char)angle; 00554 msgTx.data[3]=(unsigned char)(angle>>8); 00555 // theta signé sur 2 octets 00556 //msgTx.data[4]=(unsigned char)theta; 00557 //msgTx.data[5]=(unsigned char)(theta>>8); 00558 msgTx.data[4]=0; 00559 msgTx.data[5]=0; 00560 00561 can1.write(msgTx); 00562 00563 break;*/ 00564 00565 /* case 130://Lancer mouvement de sortie de la zone de départ 00566 msgTx.id=ACTION_BIG_DEMARRAGE; 00567 msgTx.format=CANStandard; 00568 msgTx.type=CANData; 00569 msgTx.len=1; 00570 msgTx.data[0] = (unsigned char)speed; 00571 can1.write(msgTx); 00572 break;*/ 00573 00574 default: 00575 return 0;//L'action n'existe pas, il faut utiliser le CAN 00576 00577 } 00578 return 1;//L'action est spécifique. 00579 00580 } 00581 00582 /****************************************************************************************/ 00583 /* FUNCTION NAME: initRobot */ 00584 /* DESCRIPTION : initialiser le robot */ 00585 /****************************************************************************************/ 00586 void initRobot(void) 00587 { 00588 //Enregistrement de tous les AX12 présent sur la carte 00589 /*AX12_register(5, AX12_SERIAL2); 00590 AX12_register(18, AX12_SERIAL2); 00591 AX12_register(13, AX12_SERIAL2); 00592 AX12_register(1, AX12_SERIAL1); 00593 AX12_register(11, AX12_SERIAL1); 00594 AX12_register(8, AX12_SERIAL1); 00595 AX12_register(7, AX12_SERIAL2);*/ 00596 00597 //AX12_setGoal(AX12_ID_FUNNY_ACTION, AX12_ANGLE_FUNNY_ACTION_CLOSE,AX12_SPEED_FUNNY_ACTION); 00598 //AX12_processChange(); 00599 //runRobotTest(); 00600 00601 } 00602 00603 /****************************************************************************************/ 00604 /* FUNCTION NAME: initRobotActionneur */ 00605 /* DESCRIPTION : Initialiser la position des actionneurs du robot */ 00606 /****************************************************************************************/ 00607 void initRobotActionneur(void) 00608 { 00609 wait(2); 00610 doAction(101,1,0);// preparation prise pince arriere 00611 doAction(101,0,0);// preparation prise pince avant 00612 doAction(130,0,0);// baisser aspitr 00613 wait(2); 00614 doAction(100,1,0);// preparation prise pince arriere 00615 doAction(100,0,0);// preparation prise pince avant 00616 doAction(131,0,0);// baisser aspitr 00617 } 00618 00619 /****************************************************************************************/ 00620 /* FUNCTION NAME: runTest */ 00621 /* DESCRIPTION : tester l'ensemble des actionneurs du robot */ 00622 /****************************************************************************************/ 00623 void runRobotTest(void) 00624 { 00625 /* 00626 int waitTime = 500; 00627 00628 //Test des AX12 dans l'ordre 00629 doAction(111,0,0);//Fermeture pince arrière haute 00630 wait_ms(waitTime); 00631 doAction(110,0,0);//Ouverture pince arrière haute 00632 wait_ms(waitTime); 00633 doAction(113,0,0);//Fermeture pince arrière basse 00634 wait_ms(waitTime); 00635 doAction(112,0,0);//Ouverture pince arrière basse 00636 wait_ms(waitTime); 00637 doAction(115,0,0);//Fermeture porte arrière 00638 wait_ms(waitTime); 00639 doAction(114,0,0);//Ouverture porte arrière 00640 wait_ms(waitTime); 00641 doAction(101,0,0);//Fermer les portes avant 00642 wait_ms(waitTime); 00643 doAction(100,0,0);//Ouvrir les portes avant 00644 wait_ms(waitTime); 00645 doAction(103,0,0);//Descendre le peigne 00646 wait_ms(waitTime); 00647 doAction(102,0,0);//Remonter le peigne*/ 00648 } 00649 00650 /****************************************************************************************/ 00651 /* FUNCTION NAME: SelectStrategy */ 00652 /* DESCRIPTION : Charger le fichier de stratégie correspondante à un id */ 00653 /* RETURN : 0=> Erreur, 1=> OK si le fichier existe */ 00654 /****************************************************************************************/ 00655 int SelectStrategy(unsigned char id) 00656 { 00657 switch(id) 00658 { 00659 // strat de match 00660 case 1: 00661 strcpy(cheminFileStart,"/local/strat1.txt"); 00662 return FileExists(cheminFileStart); 00663 case 2: 00664 strcpy(cheminFileStart,"/local/strat2.txt"); 00665 return FileExists(cheminFileStart); 00666 case 3: 00667 strcpy(cheminFileStart,"/local/strat3.txt"); 00668 return FileExists(cheminFileStart); 00669 case 4: 00670 strcpy(cheminFileStart,"/local/strat4.txt"); 00671 return FileExists(cheminFileStart); 00672 case 5: 00673 strcpy(cheminFileStart,"/local/strat5.txt"); 00674 return FileExists(cheminFileStart); 00675 case 6: 00676 strcpy(cheminFileStart,"/local/strat6.txt"); 00677 return FileExists(cheminFileStart); 00678 case 7: 00679 strcpy(cheminFileStart,"/local/strat7.txt"); 00680 return FileExists(cheminFileStart); 00681 case 8: 00682 strcpy(cheminFileStart,"/local/strat8.txt"); 00683 return FileExists(cheminFileStart); 00684 case 9: 00685 strcpy(cheminFileStart,"/local/strat9.txt"); 00686 return FileExists(cheminFileStart); 00687 case 10: 00688 strcpy(cheminFileStart,"/local/strat10.txt"); 00689 return FileExists(cheminFileStart); 00690 00691 // strat de demo 00692 case 0x10: 00693 strcpy(cheminFileStart,"/local/moteur.txt"); 00694 return FileExists(cheminFileStart); 00695 case 0x11: 00696 #ifdef ROBOT_BIG 00697 strcpy(cheminFileStart,"/local/bras.txt"); 00698 #else 00699 strcpy(cheminFileStart,"/local/porteAvant.txt"); 00700 #endif 00701 return FileExists(cheminFileStart); 00702 case 0x12: 00703 #ifdef ROBOT_BIG 00704 strcpy(cheminFileStart,"/local/balancier.txt"); 00705 #else 00706 strcpy(cheminFileStart,"/local/mainTourneuse.txt"); 00707 #endif 00708 return FileExists(cheminFileStart); 00709 default: 00710 strcpy(cheminFileStart,"/local/strat1.txt"); 00711 return 0; 00712 } 00713 } 00714 00715 /****************************************************************************************/ 00716 /* FUNCTION NAME: needToStop */ 00717 /* DESCRIPTION : Savoir si il faut autoriser le stop du robot via balise */ 00718 /****************************************************************************************/ 00719 unsigned char needToStop(void) 00720 { 00721 return isStopEnable; 00722 } 00723 00724 /****************************************************************************************/ 00725 /* FUNCTION NAME: doBeforeEndAction */ 00726 /* DESCRIPTION : Terminer les actions du robot 1s avant la fin du match */ 00727 /****************************************************************************************/ 00728 void doBeforeEndAction(void) 00729 { 00730 doAction(110,0,0);//Ouverture pince arrière haute 00731 doAction(112,0,0);//Ouverture pince arrière basse 00732 doAction(114,0,0);//Ouverture porte arrière 00733 doAction(100,0,0);//Ouvrir les portes avant 00734 doAction(102,0,0);//Remonter le peigne 00735 } 00736 00737 #endif
Generated on Wed Jul 13 2022 02:05:42 by
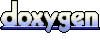