
Single UART with I2C-bus/SPI interface, 64 bytes of transmit and receive FIFOs, IrDA SIR built-in support
Dependencies: mbed
SC16IS750.cpp
00001 #include "mbed.h" 00002 #include "SC16IS750.h" 00003 00004 I2C i2c(p28,p27); // sda, scl 00005 Serial pc(USBTX, USBRX); // tx, rx 00006 char cmd[128]; 00007 char str[128]; 00008 char i, j; 00009 00010 void set_ch(char sel) 00011 { // PCA9541のサンプル 00012 // MST_0側の自分にスレーブ側の制御権を得る場合 00013 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00014 i2c.write( 0xe2, cmd, 1); // Cont Regを指定 00015 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00016 wait(0.1); // 0.1s待つ 00017 switch(cmd[0] & 0xf) 00018 { 00019 case 0: // bus off, has control 00020 case 1: // bus off, no control 00021 case 5: // bus on, no control 00022 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00023 cmd[1] = 4; // bus on, has control 00024 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00025 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00026 break; 00027 case 2: // bus off, no control 00028 case 3: // bus off, has control 00029 case 6: // bus on, no control 00030 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00031 cmd[1] = 5; // bus on, has control 00032 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00033 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00034 break; 00035 case 9: // bus on, no control 00036 case 0xc: // bus on, no control 00037 case 0xd: // bus off, no control 00038 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00039 cmd[1] = 0; // bus on, has control 00040 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00041 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00042 break; 00043 case 0xa: // bus on, no control 00044 case 0xe: // bus off, no control 00045 case 0xf: // bus on, has control 00046 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00047 cmd[1] = 1; // bus on, has control 00048 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00049 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00050 break; 00051 default: 00052 break; 00053 } 00054 00055 cmd[0] = sel; // PCA9546 Cont Reg sel channel enabled 00056 i2c.write( 0xe8, cmd, 1); // Send command string 00057 } 00058 00059 int main () 00060 { 00061 i2c.frequency(100000); 00062 pc.printf("\r\nSC16IS750 Sample Program\r\n"); 00063 00064 set_ch(1); // SC16IS750はch0に接続 00065 // 00066 00067 cmd[0] = LCR << 3; 00068 cmd[1] = 0x83; // DLL,H Latch enable 00069 i2c.write(SC16IS750_ADDR, cmd, 2); 00070 00071 cmd[0] = DLL << 3; 00072 cmd[1] = 117; // baud = 18e6 / 16 /117 = 9615 00073 i2c.write(SC16IS750_ADDR, cmd, 2); 00074 00075 cmd[0] = DLH << 3; 00076 cmd[1] = 0x0; 00077 i2c.write(SC16IS750_ADDR, cmd, 2); 00078 00079 cmd[0] = LCR << 3; 00080 cmd[1] = 0x03; // NoParity 1Stop 8bits 00081 i2c.write(SC16IS750_ADDR, cmd, 2); 00082 00083 cmd[0] = FCR << 3; 00084 cmd[1] = 0x07; // reset TX,RX FIFO, FIFO enable 00085 i2c.write(SC16IS750_ADDR, cmd, 2); 00086 00087 while(1) 00088 { 00089 pc.printf("\r\n文字列を入力してください>"); 00090 pc.scanf("%s" , str); 00091 j = strlen(str); 00092 pc.printf("\r\n送信された文字列 = %s %d文字\r\n" , str, j); 00093 cmd[0] = THR << 3; 00094 for (i = 0; i < j; i++) cmd[i + 1] = str[i]; 00095 i2c.write(SC16IS750_ADDR, cmd, j + 1); 00096 00097 wait(1); 00098 00099 cmd[0] = RHR << 3; // 00100 i2c.write(SC16IS750_ADDR, cmd, 1, true); 00101 i2c.read(SC16IS750_ADDR, cmd, j); 00102 cmd[j] = 0; 00103 pc.printf("受信した文字列 = %s\r\n", cmd); 00104 } 00105 }
Generated on Thu Jul 21 2022 14:45:08 by
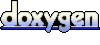