
Transistor Gijutsu, October 2014, Special Features Chapter 9, Software of the Function Generator トランジスタ技術2014年10月号 特集第9章のソフトウェア わがまま波形発生器のソフトウェア
DDS.h
00001 #ifndef DDS_h 00002 #define DDS_h 00003 00004 #define PACCLEN (4294967296.0) // 位相積算レジスタの最大値 2^23 00005 #define PI (3.1415926535897932385) // 円周率 00006 00007 // 出力電圧の設定 00008 #define VCC (3.27) // 電源電圧、実測 00009 #define BUFMAG (2.0) // 出力バッファの増幅率 00010 #define WFMAG (1.0/(VCC*BUFMAG)) // PWM値算出のための係数 00011 00012 class DDS { 00013 private: 00014 static const int MAINCLK = 48000000; // CPUのシステムクロック値 00015 static const int DDSCLK = 100000; // DDSの基準クロック値 00016 static const int TBLSIZEBITS = 10; // 波形配列の長さを決めるビット数 00017 static const int WFVGND = 0; // バースト波形の無信号状態の出力値 00018 // 以下は上の値から設定されるので変更不可 00019 static const int TBLSIZE = 1 << TBLSIZEBITS;// 波形配列の長さ 00020 static const int WFRES = 32 - TBLSIZEBITS; // 位相積算レジスタを波形配列の引数に変換するためのビットシフト値 00021 static const int WFVSPN = MAINCLK / DDSCLK; // PWMの最大値 00022 00023 static int16_t waveForm1[]; 00024 static int16_t waveForm2[]; 00025 00026 static unsigned int phaseAcc1; 00027 static unsigned int phaseInc1; 00028 static unsigned int phaseShift1; 00029 static int bstBgn1; 00030 static int bstEnd1; 00031 static int bstLen1; 00032 static int bstDcL1; 00033 static int wc1; 00034 static int lp1; 00035 00036 static unsigned int phaseAcc2; 00037 static unsigned int phaseInc2; 00038 static unsigned int phaseShift2; 00039 static int bstBgn2; 00040 static int bstEnd2; 00041 static int bstLen2; 00042 static int bstDcL2; 00043 static int wc2; 00044 static int lp2; 00045 00046 void fillTable(int16_t wftbl[], float level, float ofst, float (*waveform)(float x)); 00047 00048 public: 00049 static int trigger1; 00050 static int trigger2; 00051 00052 DDS(); 00053 static void start(void); 00054 static void stop(void); 00055 static void reset(void); 00056 int getPhase(void){ 00057 return phaseAcc1; 00058 }; 00059 static inline int getNextPw1(void); 00060 static inline int getNextPw2(void); 00061 void osc1(float freq, float phase, float level, float ofst, float (*waveform)(float x)); 00062 void osc1(float freq, float phase); 00063 void osc2(float freq, float phase, float level, float ofst, float (*waveform)(float x)); 00064 void osc2(float freq, float phase); 00065 void burst1(int begin, int end, int length, float dcLevel); 00066 void burst2(int begin, int end, int length, float dcLevel); 00067 }; 00068 00069 float triangle(float x); 00070 float square(float x); 00071 00072 00073 00074 #endif
Generated on Wed Jul 13 2022 01:52:22 by
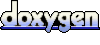