
This is THE 447 FINAL PROJECT this is the frame work put your code in the spot where the it suppose to go and. Make sure you import this into your complier and work on your section, once you done just commit the changes and fork to a new folder
Dependencies: mbed-rtos mbed draw_test EALib SWSPI
main.cpp
00001 #include <stdio.h> 00002 #include <stdlib.h> 00003 #include <iostream> 00004 #include <time.h> 00005 #include "mbed.h" 00006 #include "cmsis_os.h" 00007 #include "MMA7455.h" 00008 #include "SWSPI.h" 00009 #include "game_board.h" //duck soup 00010 // need to configure uart to 115200 for game shot placement using uart; 00011 //SPI 2 is use// SPI0 is use 00012 using namespace std; 00013 game_board battle; 00014 RawSerial pc(USBTX, USBRX); // uart //not conflit 00015 RawSerial xbee(P4_22, P4_23); // uart //not conflit 00016 MMA7455 accSensor (P0_27, P0_28); //acceleronmeter i2c // no conflit 00017 SPI LEDs(p5, NC, p7); // LEDs driver spi used 00018 //SPISlave test(p18,p19,p20,p17); 00019 //SPI between board 00020 DigitalOut cs (p30); //no conflict 00021 //SWSPI Sender(p17,p18,p19); // p11,p12,p13 00022 //DigitalOut b_cs (p20); 00023 //SPI SLAVE to receive; 00024 //SPISlave Receiver(p11,p12,p13,p14); 00025 00026 AnalogIn trimpot(p15);//might need to change the trimpot location if conflict with spi 00027 //SPI (PinName mosi, PinName miso, PinName sclk, PinName ssel=NC) //check mapping for pin 00028 //SPISlave (mosi, miso, sclk, ssel) //check mapping for pin 00029 00030 //joystick 00031 DigitalIn Up(p32); 00032 DigitalIn Down(p38); 00033 DigitalIn Left(p39); 00034 DigitalIn Right(p37); 00035 DigitalIn Center(p31); 00036 //joystick done 00037 00038 //RGB LED 00039 DigitalOut led1(p25); //red 00040 DigitalOut led2(p26);//green 00041 DigitalOut led3(p28);//blue 00042 //RGB LED 00043 int initlize = 0; 00044 //declare your input pin and output pin here: 00045 //DigitalOut led1(p25); 00046 //DigitalOut led2(p26); 00047 //DigitalOut led3(p28); 00048 // 00049 // declare you globle variable here: 00050 unsigned char my_X_coor = 1, my_Y_coor = 1; 00051 uint8_t my_LEDs = 0x1A; 00052 uint8_t SPI_X_send = 0x01,SPI_Y_send = 0x01; 00053 unsigned char other_X_coor = 0, other_Y_coor = 0; 00054 int friendly [6][6]; // array to determine friendly location 00055 int winner; 00056 int A_x_old = 0,A_y_old = 0,A_z_old = 0; 00057 int send_check = 0; 00058 int update_game_look = 0; 00059 int turns = 0; 00060 //X_coor is x coordinate from 0 to 6 00061 //Y_coor is y coordinate form A to F 00062 // 00063 // 00064 // 00065 void LEDsoutput(uint8_t value) { 00066 cs.write(0); 00067 LEDs.write(value); 00068 cs.write(1); 00069 } 00070 // this thread is your SPI communication between 2 board 00071 void SPI_communication(void const *args) { 00072 //send my_X_coor and my_Y_coor if the center button is hit 00073 //receive other board x_coor and y_coor 00074 //spi code here 00075 //turn 1 :friendly 00076 // player1 send shooting location 00077 // player1 get shooting location and whether it hit or miss 00078 //turn 1 :enemy 00079 // get enemy shooting location 00080 // check wheather is it a hit or miss 00081 // echo enemy shooting location and hit/miss flag 00082 //send out winner status 00083 // 0 = still no winner 00084 // 1 = i am lose 00085 // so if you receive a 1 that mean you win 00086 //SPI implementation could not get it to work due to run out out spi 00087 //port for design purpose software spi does not compatible 00088 //while (true) { 00089 //if (send_check == 1) { 00090 // int message = 0; 00091 // int Echo = 0; 00092 // message = (SPI_X_send << 4)|SPI_Y_send; 00093 // pc.printf ("send message %d\n\r",message); 00094 // //b_cs.write(0); 00095 // //Echo = Sender.write(0x12); 00096 // //osDelay (10); 00097 // b_cs.write(1); 00098 // pc.printf("get from echo 1 %d\n\r",Echo); 00099 // b_cs.write(0); 00100 // Echo = Sender.write (0x1FF); 00101 // b_cs.write(1); 00102 // pc.printf("get from echo 2 %d\n\r",Echo); 00103 // bool stat = (Echo &0x100)>>8; 00104 // int x_coor = (Echo & 0x0F0)>>4; 00105 // int y_coor = (Echo & 0x00F); 00106 // battle.place_hit_miss_enemy(x_coor,y_coor,stat); 00107 // update_game_look = 1; 00108 // send_check = 0; 00109 //} 00110 //if (//Receiver.receive()) { 00111 // bool status = 0; 00112 // int R_message = Receiver.read(); 00113 // pc.printf("receive message = %d\n\r",R_message); 00114 // if ((R_message & 0x200) != 0) { 00115 // winner = 1; 00116 // } 00117 // else if (R_message != 0x1FF) { 00118 // int Y_coor = R_message & 0x00F; 00119 // int X_coor = (R_message & 0x0F0) >> 4; 00120 // status = battle.place_hit_miss_friendly (X_coor,Y_coor); 00121 // int E_message = (status << 8)|R_message; 00122 // Receiver.reply(E_message); 00123 // pc.printf("echo message = %d",E_message); 00124 // update_game_look = 1; 00125 // } 00126 //} 00127 //osDelay (30); 00128 00129 //} 00130 //uart xbee impletation it is/ 00131 while(true) 00132 { 00133 char R_message [5] = {0,0,0,0,0}; 00134 int got_mess = 0;//temp 00135 int i = 0; 00136 00137 if(send_check == 1) 00138 { 00139 xbee.printf ("S00%d%dE",SPI_X_send,SPI_Y_send); 00140 send_check = 0; 00141 } 00142 00143 while(xbee.readable()) 00144 { 00145 char temp; 00146 temp = xbee.getc(); 00147 R_message[i] = temp; 00148 i = i+1; 00149 got_mess = 1; 00150 osDelay(10); 00151 } 00152 if (R_message[0] == 'S') 00153 { 00154 int x_coor,y_coor; 00155 int hit_miss; 00156 x_coor = (int)R_message[3] - 48; 00157 y_coor = (int)R_message[4] - 48; 00158 hit_miss = battle.place_hit_miss_friendly(y_coor,x_coor); 00159 xbee.printf ("E0%d%d%dE",hit_miss,x_coor,y_coor); 00160 //pc.printf ("x=%d y=%d\n\r",x_coor,y_coor); 00161 update_game_look = 1; 00162 } 00163 else if (R_message[0] == 'E') 00164 { 00165 int x_coor,y_coor; 00166 int hit_miss; 00167 hit_miss = (int)R_message[2] - 48; 00168 x_coor = (int)R_message[3] - 48; 00169 y_coor = (int)R_message[4] - 48; 00170 battle.place_hit_miss_enemy(y_coor,x_coor,(bool)hit_miss); 00171 update_game_look = 1; 00172 } 00173 else if (R_message[0] == 'W') 00174 { 00175 winner = 1; 00176 } 00177 //osDelay(30); 00178 } 00179 } 00180 00181 // joystick update x_coor and y_coor here 00182 void joy_stick_read(void const *args) { //might be an interrupt thread here for interrupt read of joystick 00183 // joy stick to update global val of x_coor and y_coor; 00184 // convert y coor to hex offset 00185 // update the 8 LEDs here 00186 while (true) { 00187 //pc.printf ("in joystick"); 00188 if (Up.read() == 0) { 00189 my_Y_coor = my_Y_coor + 1; 00190 if (my_Y_coor == 7) { 00191 my_Y_coor = 1; 00192 } 00193 } else if (Down.read() == 0) { 00194 my_Y_coor = my_Y_coor - 1; 00195 if (my_Y_coor == 0) { 00196 my_Y_coor = 6; 00197 } 00198 } else if (Left.read() == 0) { 00199 my_X_coor = my_X_coor + 1 ; 00200 if (my_X_coor == 7) { 00201 my_X_coor = 1; 00202 } 00203 //my_LEDs = my_X_coor; 00204 } else if (Right.read() == 0) { 00205 my_X_coor = my_X_coor - 1; 00206 if (my_X_coor == 0) { 00207 my_X_coor = 6; 00208 } 00209 } 00210 //my_LEDs = my_X_coor; 00211 else if (Center.read() == 0) { 00212 //pc.printf ("got middle\n\r"); 00213 SPI_X_send = my_X_coor; 00214 SPI_Y_send = my_Y_coor; 00215 //pc.printf ("spi x = %d\n\r",SPI_X_send); 00216 //pc.printf ("spi y = %d\n\r",SPI_Y_send); 00217 send_check = 1; 00218 } else { 00219 my_X_coor = my_X_coor; 00220 my_Y_coor = my_Y_coor; 00221 } 00222 my_LEDs = (my_X_coor << 5)|(0x0F-(my_Y_coor-1)); 00223 LEDsoutput (my_LEDs); 00224 osDelay (170); 00225 } 00226 //joystick is done and over with DO NOT touch 00227 00228 } 00229 00230 // this thread use to update the game board 00231 void game_view_update (void const *args) { 00232 // board of this game will be a class this update and get info to and from the class 00233 // update the by led game update 00234 // Uart runing at 921600 max speed; hopefully print 1 line and out; 00235 // using an interger then cycle through the get string function to put it inti the printf function 00236 00237 // * = hit 00238 // o = miss 00239 // > = your ship ship 00240 00241 // 1) check for a hit 00242 // 2) update the game board 00243 00244 // BLANK game Board 00245 // F _ _ _ _ _ _ F _ _ _ _ _ _ 00246 // E _ _ _ _ _ _ E _ _ _ _ _ _ 00247 // D _ _ _ _ _ _ D _ _ _ _ _ _ 00248 // C _ _ _ _ _ _ C _ _ _ _ _ _ 00249 // B _ _ _ _ _ _ B _ _ _ _ _ _ 00250 // A _ _ _ _ _ _ A _ _ _ _ _ _ 00251 // 1 2 3 4 5 6 1 2 3 4 5 6 00252 // Friendly Enemy 00253 00254 // Example of running game 00255 // F _ O _ > _ _ F _ O _ _ _ _ 00256 // E > _ O _ > _ E _ _ * _ _ _ 00257 // D _ _ > _ _ _ D _ _ _ O _ _ 00258 // C O _ _ _ _ _ C _ O _ _ _ _ 00259 // B _ * _ O _ _ B _ _ _ * _ _ //X on friendly mean your ship got destroy 00260 // A _ _ _ > _ _ A _ _ _ _ _ _ 00261 // 1 2 3 4 5 6 1 2 3 4 5 6 00262 // Friendly Enemy 00263 // Turn 1 = miss at 2F 00264 // Turn 2 = We sink an enemy battle ship at 3E 00265 // Turn 3 = miss at 4D 00266 // Turn 4 = miss at 2C 00267 // Tunr 5 = We sink an enemy battle ship at 4B 00268 while (true) { 00269 if (update_game_look == 1) { 00270 turns = turns + 1; 00271 update_game_look = 0; 00272 int i; 00273 pc.printf ("\fTurn :%d\n\r",turns); 00274 for (i = 1; i < 9; i++) { 00275 string a; 00276 a = battle.get1row(i); 00277 pc.printf ("%s", a); 00278 } 00279 } 00280 osDelay (30); 00281 } 00282 00283 } 00284 00285 // code here to check for winer and turn on the RGB 00286 void winnercheck (void const *args) 00287 { 00288 //check for winner by destroy all enemy ship; 00289 while (true) { 00290 bool gamelost = 0; 00291 gamelost = battle.looser(); 00292 //winner = 1; 00293 if (gamelost == 1) { 00294 led1 = 1; 00295 led2 = 1; 00296 led3 = 1; 00297 xbee.printf("W0000E"); 00298 while(winner == 0) 00299 { 00300 osDelay(30); 00301 } 00302 //this board is loser 00303 //send a message of 0x200 to the winner 00304 } else if (winner == 1) { //spi message is the winner) 00305 pc.printf("Spectacular Battle commander\n\r"); 00306 while (winner == 1) { 00307 //pc.printf ("%d \n\r",rand()%2); 00308 led1 = (rand()%2); 00309 led2 = (rand()%2); 00310 led3 = (rand()%2); 00311 osDelay(30); 00312 } 00313 } 00314 else { 00315 led1 = 1; 00316 led2 = 1; 00317 led3 = 1; 00318 } 00319 00320 } 00321 } 00322 00323 //read the accele 00324 void checkreset (void const *args) 00325 { 00326 00327 //read the acceleron meter and compare against the previous value to 00328 //determine if the board have been shake the reset the game board; 00329 //the determinant is the x axis 00330 while (true) { 00331 00332 int Ax,Ay,Az; 00333 int x_old = 0, y_old = 0; 00334 int x, y; 00335 int i; 00336 int seed = trimpot.read()*1000; 00337 00338 srand (seed); 00339 if(! accSensor.read(Ax,Ay,Az)) {}; 00340 if (abs(Ax-A_x_old) > 20) { 00341 winner = 0; 00342 turns = 0; 00343 pc.printf ("\fNew Game: Battle Ship Θ\n\r"); 00344 battle.new_game_board(); 00345 //randomize ship position 6 ship total 00346 for (i = 0; i < 7; i++) { 00347 x = (rand()%6 +1); 00348 while (x == x_old) { 00349 x = (rand()%6 +1); 00350 } 00351 x_old = x; 00352 y = (rand()%5 +1); 00353 while (y == y_old) { 00354 y = (rand()%5 +1); 00355 } 00356 y_old = y; 00357 battle.place_ship(x,y); 00358 } 00359 //pc.printf ("\f"); 00360 for (i = 1; i < 9; i++) { 00361 string a; 00362 a = battle.get1row(i); 00363 pc.printf ("%s", a); 00364 } 00365 Ax = 0; 00366 A_x_old = 0; 00367 } else 00368 A_x_old = Ax; 00369 osDelay(500); 00370 } 00371 //reset is done and working do not touch 00372 } 00373 00374 00375 osThreadDef(SPI_communication, osPriorityNormal, DEFAULT_STACK_SIZE); //comm between 2 board define thread 00376 osThreadDef(joy_stick_read, osPriorityNormal, DEFAULT_STACK_SIZE);//might be an interrupt define thread 00377 osThreadDef(game_view_update, osPriorityNormal, DEFAULT_STACK_SIZE);//update game view write to the fifo uart buffer define thread 00378 osThreadDef(winnercheck, osPriorityNormal, DEFAULT_STACK_SIZE); //check for winner define thread 00379 osThreadDef(checkreset, osPriorityNormal, DEFAULT_STACK_SIZE); //check for reset define thread 00380 00381 int main() { 00382 osThreadCreate(osThread(SPI_communication), NULL);//done but now is uart 00383 osThreadCreate(osThread(joy_stick_read), NULL); //done 00384 osThreadCreate(osThread(game_view_update), NULL);//done 00385 osThreadCreate(osThread(winnercheck), NULL);//buggy 00386 osThreadCreate(osThread(checkreset), NULL);//done 00387 00388 if (initlize == 0) 00389 { 00390 int x_old = 0, y_old = 0; 00391 int x, y; 00392 int i; 00393 int seed = trimpot.read()*1000; 00394 winner = 0; 00395 srand (seed); 00396 pc.baud(921600); 00397 xbee.baud(115200); 00398 00399 pc.printf("Battle Ship by Andy, Adam, Loc, Ivan\n\r"); 00400 while(!accSensor.setMode(MMA7455::ModeMeasurement)) { 00401 pc.printf("Unable to set measurement mode.\n\r"); 00402 } 00403 while(!accSensor.setRange(MMA7455::Range_8g)) { 00404 pc.printf("Unable to set the range.\n\r"); 00405 } 00406 while(!accSensor.calibrate()) { 00407 pc.printf("Unable to calibrate\n\r"); 00408 } 00409 battle.new_game_board(); 00410 for (i = 1; i < 9; i++){ 00411 string a; 00412 a = battle.get1row(i); 00413 pc.printf ("%s", a); 00414 00415 } 00416 //randomize ship position 6 ship total 00417 for (i = 0; i < 7; i++) { 00418 x = (rand()%6 +1); 00419 while (x == x_old) 00420 { 00421 x = (rand()%6 +1); 00422 } 00423 x_old = x; 00424 y = (rand()%5 +1); 00425 while (y == y_old) 00426 { 00427 y = (rand()%5 +1); 00428 } 00429 y_old = y; 00430 battle.place_ship(x,y); 00431 } 00432 pc.printf("\fBattle Ship by Andy, Adam, Loc, Ivan\n\r"); 00433 for (i = 1; i < 9; i++){ 00434 string a; 00435 a = battle.get1row(i); 00436 pc.printf ("%s", a); 00437 } 00438 initlize = 1; 00439 00440 } 00441 //Main Thread 00442 while (true) { 00443 osDelay (30); 00444 } 00445 }
Generated on Sat Jul 23 2022 03:05:08 by
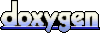