
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
utils.h
00001 #ifndef __UTILS_H__ 00002 #define __UTILS_H__ 00003 00004 #include "inc/adi_sense_api.h" 00005 #include "inc/adi_sense_1000/adi_sense_1000_api.h" 00006 00007 #ifdef __cplusplus 00008 extern "C" { 00009 #endif 00010 00011 /* Utility function to print the status read from the ADI Sense device */ 00012 void utils_printStatus( 00013 ADI_SENSE_STATUS *pStatus); 00014 00015 /* Utility function to print data samples read from the ADI Sense device */ 00016 void utils_printSamples( 00017 ADI_SENSE_DATA_SAMPLE *pSampleBuffer, 00018 uint32_t numSamples); 00019 00020 /* Utility function to register callbacks for ADI Sense device notification signals */ 00021 ADI_SENSE_RESULT utils_registerCallbacks( 00022 ADI_SENSE_DEVICE_HANDLE hDevice, 00023 volatile bool_t *pbDataReady, 00024 volatile bool_t *pbError, 00025 volatile bool_t *pbAlert); 00026 00027 /* Utility function to de-register callbacks for ADI Sense device notification signals */ 00028 ADI_SENSE_RESULT utils_deregisterCallbacks( 00029 ADI_SENSE_DEVICE_HANDLE hDevice); 00030 00031 /* Utility function to run measurements on ADI Sense device, according to its current 00032 * configuration, and display data samples and device status following each cycle */ 00033 ADI_SENSE_RESULT utils_runMeasurement( 00034 ADI_SENSE_DEVICE_HANDLE hDevice, 00035 ADI_SENSE_MEASUREMENT_MODE eMeasurementMode); 00036 00037 /* Utility function to retrieve and print the factory calibration coefficients table from the ADI Sense device */ 00038 ADI_SENSE_RESULT utils_printCalTable( 00039 ADI_SENSE_DEVICE_HANDLE hDevice); 00040 00041 #ifdef __cplusplus 00042 } 00043 #endif 00044 00045 #endif /* __UTILS_H__ */ 00046
Generated on Tue Jul 12 2022 21:13:17 by
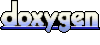