
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_types.h
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_types.h 00004 * @brief: Type definitions for ADI Sense API. 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /* 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 */ 00042 00043 #ifndef __ADI_SENSE_TYPES_H__ 00044 #define __ADI_SENSE_TYPES_H__ 00045 00046 #include "inc/adi_sense_platform.h" 00047 00048 /*! 00049 ***************************************************************************** 00050 * \enum ADI_SENSE_RESULT 00051 * 00052 * ADI Sense API Error Codes. #ADI_SENSE_SUCCESS is always zero 00053 * The return value of all ADI Sense APIs returning #ADI_SENSE_RESULT 00054 * should always be tested at the application level for success or failure. 00055 * 00056 *****************************************************************************/ 00057 typedef enum 00058 { 00059 /*! Generic success. */ 00060 ADI_SENSE_SUCCESS , 00061 /*! Generic Failure. */ 00062 ADI_SENSE_FAILURE , 00063 /*! Operation incomplete, call again */ 00064 ADI_SENSE_INCOMPLETE , 00065 /*! Device is already initialized. */ 00066 ADI_SENSE_IN_USE , 00067 /*! Invalid device handle. */ 00068 ADI_SENSE_INVALID_HANDLE , 00069 /*! Invalid device ID. */ 00070 ADI_SENSE_INVALID_DEVICE_NUM , 00071 /*! Device is uninitialized. */ 00072 ADI_SENSE_ERR_NOT_INITIALIZED , 00073 /*! NULL data pointer not allowed. */ 00074 ADI_SENSE_INVALID_POINTER , 00075 /*! Parameter is out of range. */ 00076 ADI_SENSE_INVALID_PARAM , 00077 /*! Unsupported mode of operation. */ 00078 ADI_SENSE_UNSUPPORTED_MODE , 00079 /*! Invalid operation */ 00080 ADI_SENSE_INVALID_OPERATION , 00081 /*! No data available, or buffer full */ 00082 ADI_SENSE_NO_DATA , 00083 /*! No buffer space available */ 00084 ADI_SENSE_NO_SPACE , 00085 /*! Square root of a negative number */ 00086 ADI_SENSE_NEGATIVE_SQRT , 00087 /*! Division by 0 or 0.0 */ 00088 ADI_SENSE_DIVIDE_BY_ZERO , 00089 /*! Invalid signature */ 00090 ADI_SENSE_INVALID_SIGNATURE , 00091 /*! Wrong size */ 00092 ADI_SENSE_WRONG_SIZE , 00093 /*! Sample Out of the dsp data limits */ 00094 ADI_SENSE_OUT_OF_RANGE , 00095 /*! Unable to operate with not a number */ 00096 ADI_SENSE_NAN_FOUND , 00097 /*! Timeout error */ 00098 ADI_SENSE_TIMEOUT , 00099 /*! Memory allocation error */ 00100 ADI_SENSE_NO_MEM , 00101 /*! CRC validation error */ 00102 ADI_SENSE_CRC_ERROR , 00103 } ADI_SENSE_RESULT ; 00104 00105 #endif /* __ADI_SENSE_TYPES_H__ */ 00106
Generated on Tue Jul 12 2022 21:13:17 by
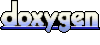