
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_spi.h
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_spi.h 00004 * @brief: ADI Sense OS-dependent wrapper layer for SPI interface 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /* 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 */ 00042 00043 #ifndef __ADI_SENSE_SPI_H__ 00044 #define __ADI_SENSE_SPI_H__ 00045 00046 #include "inc/adi_sense_types.h" 00047 #include "inc/adi_sense_platform.h" 00048 00049 /*! @ingroup ADI_Sense_Host */ 00050 00051 /*! @addtogroup ADI_Sense_Spi ADI Sense Host SPI interface functions 00052 * @{ 00053 */ 00054 00055 /*! A handle used in all API functions to identify the SPI interface context */ 00056 typedef void * ADI_SENSE_SPI_HANDLE ; 00057 00058 #ifdef __cplusplus 00059 extern "C" 00060 { 00061 #endif 00062 00063 /*! 00064 * @brief Open the SPI interface and allocate resources 00065 * 00066 * @param[in] pConfig Pointer to platform-specific SPI interface details 00067 * @param[out] phDevice Pointer to return a SPI interface context handle 00068 * 00069 * @return Status 00070 * - #ADI_SENSE_SUCCESS Call completed successfully 00071 * - #ADI_SENSE_NO_MEM Failed to allocate memory for interface context 00072 */ 00073 ADI_SENSE_RESULT adi_sense_SpiOpen( 00074 ADI_SENSE_PLATFORM_SPI_CONFIG * pConfig, 00075 ADI_SENSE_SPI_HANDLE * phDevice); 00076 00077 /*! 00078 * @brief Close SPI interface and free resources 00079 * 00080 * @param[in] hDevice SPI interface context handle (@ref adi_sense_SpiOpen) 00081 */ 00082 void adi_sense_SpiClose( 00083 ADI_SENSE_SPI_HANDLE hDevice); 00084 00085 /*! 00086 * @brief Transfer data to slave device 00087 * 00088 * @param[in] hDevice SPI interface context handle (@ref adi_sense_SpiOpen) 00089 * @param[in] pTxData Transmit data buffer, or NULL for read-only transfers 00090 * @param[in] pRxData Receive data buffer, or NULL for write-only transfers 00091 * @param[in] nLength Number of bytes to transfer 00092 * @param[in] bCsHold Leave the chip-select asserted when the transfer is done 00093 * 00094 * @return Status 00095 * - #ADI_SENSE_SUCCESS Call completed successfully 00096 * - #ADI_SENSE_FAILURE Failed to complete SPI transfer 00097 */ 00098 ADI_SENSE_RESULT adi_sense_SpiTransfer( 00099 ADI_SENSE_SPI_HANDLE hDevice, 00100 void * pTxData, 00101 void * pRxData, 00102 unsigned nLength, 00103 bool bCsHold); 00104 00105 #ifdef __cplusplus 00106 } 00107 #endif 00108 00109 /*! 00110 * @} 00111 */ 00112 00113 #endif /* __ADI_SENSE_SPI_H__ */ 00114
Generated on Tue Jul 12 2022 21:13:17 by
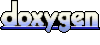