
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_log.cpp
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_log.c 00004 * @brief: ADI Sense OS-dependent wrapper layer for log functions 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /****************************************************************************** 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 * 00042 *****************************************************************************/ 00043 00044 #include <mbed.h> 00045 #include "inc/adi_sense_log.h" 00046 00047 #define ADI_SENSE_LOG_UART_BAUDRATE (115200) 00048 00049 #ifdef TARGET_STM32F411xE 00050 #define ADI_SENSE_LOG_UART_TX_PIN (PA_11) 00051 #define ADI_SENSE_LOG_UART_RX_PIN (PA_12) 00052 #else 00053 #error "unsupported target platform" 00054 #endif 00055 00056 static Serial *gpUartDevice; 00057 00058 #ifdef __cplusplus 00059 extern "C" { 00060 #endif 00061 00062 /* 00063 * Open the Log interface and allocate resources 00064 */ 00065 ADI_SENSE_RESULT adi_sense_LogOpen(void) 00066 { 00067 gpUartDevice = new Serial(ADI_SENSE_LOG_UART_TX_PIN, 00068 ADI_SENSE_LOG_UART_RX_PIN, 00069 ADI_SENSE_LOG_UART_BAUDRATE); 00070 if (!gpUartDevice) 00071 { 00072 ADI_SENSE_LOG_ERROR("Failed to allocate memory for Log UART context"); 00073 return ADI_SENSE_NO_MEM ; 00074 } 00075 00076 return ADI_SENSE_SUCCESS ; 00077 } 00078 00079 /* 00080 * Close the Log interface and free resources 00081 */ 00082 void adi_sense_LogClose(void) 00083 { 00084 if (gpUartDevice) 00085 { 00086 delete gpUartDevice; 00087 gpUartDevice = 0; 00088 } 00089 } 00090 00091 /* 00092 * Print a log message to the platform log interface 00093 */ 00094 void adi_sense_Log(ADI_SENSE_LOG_LEVEL level, const char* format, ...) 00095 { 00096 char buffer[256]; 00097 va_list va_args; 00098 00099 if (!gpUartDevice) 00100 return; 00101 00102 va_start(va_args, format); 00103 vsnprintf(buffer, sizeof(buffer), format, va_args); 00104 va_end(va_args); 00105 00106 gpUartDevice->printf("%s\r\n", buffer); 00107 } 00108 00109 #ifdef __cplusplus 00110 } 00111 #endif 00112
Generated on Tue Jul 12 2022 21:13:17 by
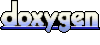