
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_api.h
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_api.h 00004 * @brief: ADI Sense Host Library Application Programming Interface (API) 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /* 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 */ 00042 00043 #ifndef __ADI_SENSE_API_H__ 00044 #define __ADI_SENSE_API_H__ 00045 00046 #include "inc/adi_sense_types.h" 00047 #include "inc/adi_sense_config_types.h" 00048 #include "inc/adi_sense_platform.h" 00049 #include "inc/adi_sense_gpio.h" 00050 #include "inc/adi_sense_spi.h" 00051 #include "inc/adi_sense_log.h" 00052 #include "inc/adi_sense_time.h" 00053 00054 /*! @defgroup ADI_Sense_Api ADI Sense Host Library API 00055 * Host library API common to the ADI Sense product line. 00056 * @{ 00057 */ 00058 00059 #ifdef __cplusplus 00060 extern "C" { 00061 #endif 00062 00063 /*! The maximum number of channels supported by this API 00064 * @note Specific ADI Sense products may implement a lower number of channels */ 00065 #define ADI_SENSE_MAX_CHANNELS 16 00066 00067 /*! A handle used in all API functions to identify the ADI Sense device. */ 00068 typedef void* ADI_SENSE_DEVICE_HANDLE ; 00069 00070 /*! Supported connection types for communication with the ADI Sense device. */ 00071 typedef enum { 00072 ADI_SENSE_CONNECTION_TYPE_SPI = 1, 00073 /*!< Serial Peripheral Interface (SPI) connection type */ 00074 } ADI_SENSE_CONNECTION_TYPE ; 00075 00076 /*! Connection details for communication with a ADI Sense device instance. */ 00077 typedef struct { 00078 ADI_SENSE_CONNECTION_TYPE type; 00079 /*!< Connection type selection */ 00080 ADI_SENSE_PLATFORM_SPI_CONFIG spi; 00081 /*!< SPI connection parameters, required if SPI connection type is used */ 00082 ADI_SENSE_PLATFORM_GPIO_CONFIG gpio; 00083 /*!< GPIO connection parameters, for device reset and status I/O signals */ 00084 } ADI_SENSE_CONNECTION ; 00085 00086 /*! Bit masks (flags) for the different device status indicators. */ 00087 typedef enum { 00088 ADI_SENSE_DEVICE_STATUS_BUSY = (1 << 0), 00089 /*!< Indicates that a command is currently running on the device */ 00090 ADI_SENSE_DEVICE_STATUS_DATAREADY = (1 << 1), 00091 /*!< Indicates the availability of measurement data for retrieval */ 00092 ADI_SENSE_DEVICE_STATUS_ERROR = (1 << 2), 00093 /*!< Indicates that an error condition has been detected by the device */ 00094 ADI_SENSE_DEVICE_STATUS_ALERT = (1 << 3), 00095 /*!< Indicates that an alert condition has been detected by the device */ 00096 ADI_SENSE_DEVICE_STATUS_FIFO_ERROR = (1 << 4), 00097 /*!< Indicates that a FIFO error condition has been detected by the device */ 00098 ADI_SENSE_DEVICE_STATUS_CONFIG_ERROR = (1 << 5), 00099 /*!< Indicates that a configuration error condition has been detected by the device */ 00100 ADI_SENSE_DEVICE_STATUS_LUT_ERROR = (1 << 6), 00101 /*!< Indicates that a look-up table error condition has been detected by the device */ 00102 } ADI_SENSE_DEVICE_STATUS_FLAGS ; 00103 00104 /*! Bit masks (flags) for the different diagnostics status indicators. */ 00105 typedef enum { 00106 ADI_SENSE_DIAGNOSTICS_STATUS_CHECKSUM_ERROR = (1 << 0), 00107 /*!< Indicates Error on Internal Checksum Calculations */ 00108 ADI_SENSE_DIAGNOSTICS_STATUS_COMMS_ERROR = (1 << 1), 00109 /*!< Indicates Error on Internal Device Communications */ 00110 ADI_SENSE_DIAGNOSTICS_STATUS_SUPPLY_MONITOR_ERROR = (1 << 2), 00111 /*!< Indicates Low Voltage on Internal Supply Voltages */ 00112 ADI_SENSE_DIAGNOSTICS_STATUS_SUPPLY_CAP_ERROR = (1 << 3), 00113 /*!< Indicates Fault on Internal Supply Regulator Capacitor */ 00114 ADI_SENSE_DIAGNOSTICS_STATUS_AINM_UV_ERROR = (1 << 4), 00115 /*!< Indicates Under-Voltage Error on Negative Analog Input */ 00116 ADI_SENSE_DIAGNOSTICS_STATUS_AINM_OV_ERROR = (1 << 5), 00117 /*!< Indicates Over-Voltage Error on Negative Analog Input */ 00118 ADI_SENSE_DIAGNOSTICS_STATUS_AINP_UV_ERROR = (1 << 6), 00119 /*!< Indicates Under-Voltage Error on Positive Analog Input */ 00120 ADI_SENSE_DIAGNOSTICS_STATUS_AINP_OV_ERROR = (1 << 7), 00121 /*!< Indicates Over-Voltage Error on Positive Analog Input */ 00122 ADI_SENSE_DIAGNOSTICS_STATUS_CONVERSION_ERROR = (1 << 8), 00123 /*!< Indicates Error During Internal ADC Conversions */ 00124 ADI_SENSE_DIAGNOSTICS_STATUS_CALIBRATION_ERROR = (1 << 9), 00125 /*!< Indicates Error During Internal Device Calibrations */ 00126 } ADI_SENSE_DIAGNOSTICS_STATUS_FLAGS ; 00127 00128 /*! Bit masks (flags) for the different channel alert indicators. */ 00129 typedef enum { 00130 ADI_SENSE_CHANNEL_ALERT_TIMEOUT = (1 << 0), 00131 /*!< Indicates timeout condition detected on the channel */ 00132 ADI_SENSE_CHANNEL_ALERT_UNDER_RANGE = (1 << 1), 00133 /*!< Indicates raw sample under valid input range, possibly clamped */ 00134 ADI_SENSE_CHANNEL_ALERT_OVER_RANGE = (1 << 2), 00135 /*!< Indicates raw sample over valid input range, possibly clamped */ 00136 ADI_SENSE_CHANNEL_ALERT_LOW_LIMIT = (1 << 3), 00137 /*!< Indicates measurement result was below configured minimum threshold */ 00138 ADI_SENSE_CHANNEL_ALERT_HIGH_LIMIT = (1 << 4), 00139 /*!< Indicates measurement result was above configured maximum threshold */ 00140 ADI_SENSE_CHANNEL_ALERT_SENSOR_OPEN = (1 << 5), 00141 /*!< Indicates open circuit or mis-wire condition detected on the channel */ 00142 ADI_SENSE_CHANNEL_ALERT_REF_DETECT = (1 << 6), 00143 /*!< Indicates reference-detect error condition detected on the channel */ 00144 ADI_SENSE_CHANNEL_ALERT_CONFIG_ERR = (1 << 7), 00145 /*!< Indicates configuration error condition detected on the channel */ 00146 ADI_SENSE_CHANNEL_ALERT_LUT_ERR = (1 << 8), 00147 /*!< Indicates look-up table error condition detected on the channel */ 00148 ADI_SENSE_CHANNEL_ALERT_SENSOR_NOT_READY = (1 << 9), 00149 /*!< Indicates digital sensor not-ready error condition detected on the channel */ 00150 ADI_SENSE_CHANNEL_ALERT_COMP_NOT_READY = (1 << 10), 00151 /*!< Indicates compensation channel not-ready error condition detected on the channel */ 00152 ADI_SENSE_CHANNEL_ALERT_UNDER_VOLTAGE = (1 << 11), 00153 /*!< Indicates under-voltage condition detected on the channel */ 00154 ADI_SENSE_CHANNEL_ALERT_OVER_VOLTAGE = (1 << 12), 00155 /*!< Indicates over-voltage condition detected on the channel */ 00156 ADI_SENSE_CHANNEL_ALERT_LUT_UNDER_RANGE = (1 << 13), 00157 /*!< Indicates raw sample was under the available LUT/equation range */ 00158 ADI_SENSE_CHANNEL_ALERT_LUT_OVER_RANGE = (1 << 14), 00159 /*!< Indicates raw sample was over the available LUT/equation range */ 00160 } ADI_SENSE_CHANNEL_ALERT_FLAGS ; 00161 00162 /*! Status details retreived from the ADI Sense device. */ 00163 typedef struct { 00164 ADI_SENSE_DEVICE_STATUS_FLAGS deviceStatus; 00165 /*!< General summary status information from the device */ 00166 ADI_SENSE_DIAGNOSTICS_STATUS_FLAGS diagnosticsStatus; 00167 /*!< Diagnostic error status information from the device */ 00168 ADI_SENSE_CHANNEL_ALERT_FLAGS channelAlerts[ADI_SENSE_MAX_CHANNELS]; 00169 /*!< Per-channel alert status information from the device */ 00170 uint32_t errorCode; 00171 /*!< Code identifying the last error signalled by the device */ 00172 uint32_t alertCode; 00173 /*!< Code identifying the last alert signalled by the device */ 00174 uint32_t channelAlertCodes[ADI_SENSE_MAX_CHANNELS]; 00175 /*!< Per-channel code identifying the last alert signalled for each channel */ 00176 } ADI_SENSE_STATUS ; 00177 00178 /*! Data sample details retreived from the ADI Sense device. */ 00179 typedef struct { 00180 ADI_SENSE_DEVICE_STATUS_FLAGS status; 00181 /*!< Device summary status snapshot when the sample was recorded */ 00182 uint32_t channelId; 00183 /*!< The measurement channel from which this sample was obtained */ 00184 uint32_t rawValue; 00185 /*!< The raw (unprocessed) value obtained directly from the measurement 00186 * channel, if available 00187 */ 00188 float32_t processedValue; 00189 /*!< The processed value obtained from the measurement channel, as a final 00190 * measurement value, following calibration and linearisation correction, 00191 * and conversion into an appropriate unit of measurement. 00192 */ 00193 } ADI_SENSE_DATA_SAMPLE ; 00194 00195 /*! Measurement mode options for the ADI Sense device. 00196 * @ref adi_sense_StartMeasurement 00197 */ 00198 typedef enum { 00199 ADI_SENSE_MEASUREMENT_MODE_HEALTHCHECK = 1, 00200 /*!< In this mode, a special health-check measurement cycle is executed, 00201 * carrying out a single conversion per channel with measurement 00202 * diagnostics enabled, intended for use as a system health check. */ 00203 ADI_SENSE_MEASUREMENT_MODE_NORMAL , 00204 /*!< In this mode, normal measurement cycle(s) are executed and data samples 00205 * are returned with raw measurement values included. */ 00206 ADI_SENSE_MEASUREMENT_MODE_OMIT_RAW , 00207 /*!< In this mode, normal measurement cycle(s) are executed and data samples 00208 * are returned with raw measurement values omitted for efficiency. */ 00209 } ADI_SENSE_MEASUREMENT_MODE ; 00210 00211 00212 /****************************************************************************** 00213 * ADI Sense High-Level API function prototypes 00214 *****************************************************************************/ 00215 00216 /*! 00217 * @brief Open ADI Sense device handle and set up communication interface. 00218 * 00219 * @param[in] nDeviceIndex Zero-based index number identifying this device 00220 * instance. Note that this will be used to 00221 * retrieve a specific device configuration for 00222 * this device (see @ref adi_sense_SetConfig 00223 * and @ref ADI_SENSE_CONFIG) 00224 * @param[in] pConnectionInfo Host-specific connection details (e.g. SPI, GPIO) 00225 * @param[out] phDevice Pointer to return an ADI Sense device handle 00226 * 00227 * @return Status 00228 * - #ADI_SENSE_SUCCESS Call completed successfully. 00229 * - #ADI_SENSE_NO_MEM Failed to allocate memory resources. 00230 * - #ADI_SENSE_INVALID_DEVICE_NUM Invalid device index specified 00231 * 00232 * @details Configure and initialise the Log interface and the SPI/GPIO 00233 * communication interface to the ADISense module. 00234 */ 00235 ADI_SENSE_RESULT adi_sense_Open( 00236 unsigned const nDeviceIndex, 00237 ADI_SENSE_CONNECTION * const pConnectionInfo, 00238 ADI_SENSE_DEVICE_HANDLE * const phDevice); 00239 00240 /*! 00241 * @brief Close ADI Sense device context and free resources. 00242 * 00243 * @param[in] hDevice ADI Sense device context handle 00244 * 00245 * @return Status 00246 * - #ADI_SENSE_SUCCESS Call completed successfully. 00247 */ 00248 ADI_SENSE_RESULT adi_sense_Close( 00249 ADI_SENSE_DEVICE_HANDLE const hDevice); 00250 00251 /*! 00252 * @brief Get the current state of the specified GPIO input signal. 00253 * 00254 * @param[in] hDevice ADI Sense device context handle 00255 * @param[in] ePin GPIO pin to query 00256 * @param[out] pbError Pointer to return the state of the status signal GPIO pin 00257 * 00258 * @return Status 00259 * - #ADI_SENSE_SUCCESS Call completed successfully. 00260 * - #ADI_SENSE_INVALID_DEVICE_NUM Invalid GPIO pin specified. 00261 * 00262 * @details Sets *pbAsserted to true if the status signal is asserted, or false 00263 * otherwise. 00264 */ 00265 ADI_SENSE_RESULT adi_sense_GetGpioState( 00266 ADI_SENSE_DEVICE_HANDLE const hDevice, 00267 ADI_SENSE_GPIO_PIN const ePinId, 00268 bool_t * const pbAsserted); 00269 00270 /*! 00271 * @brief Register an application-defined callback function for GPIO interrupts 00272 * 00273 * @param[in] hDevice ADI Sense context handle (@ref adi_sense_Open) 00274 * @param[in] ePin GPIO pin on which to enable/disable interrupts 00275 * @param[in] callbackFunction Function to be called when an interrupt occurs. 00276 * Specify NULL here to disable interrupts. 00277 * @param[in] pCallbackParam Optional opaque parameter passed to the callback 00278 * 00279 * @return Status 00280 * - #ADI_SENSE_SUCCESS Call completed successfully. 00281 * - #ADI_SENSE_INVALID_DEVICE_NUM Invalid GPIO pin specified. 00282 */ 00283 ADI_SENSE_RESULT adi_sense_RegisterGpioCallback( 00284 ADI_SENSE_DEVICE_HANDLE const hDevice, 00285 ADI_SENSE_GPIO_PIN const ePinId, 00286 ADI_SENSE_GPIO_CALLBACK const callbackFunction, 00287 void * const pCallbackParam); 00288 00289 /*! 00290 * @brief Reset the ADI Sense device. 00291 * 00292 * @param[in] hDevice ADI Sense device context handle 00293 * 00294 * @return Status 00295 * - #ADI_SENSE_SUCCESS Call completed successfully. 00296 * 00297 * @details Trigger a hardware-reset of the ADI Sense device. 00298 * 00299 * @note The device may require several seconds before it is ready for use 00300 * again. @ref adi_sense_GetDeviceReadyState may be used to check if 00301 * the device is ready. 00302 */ 00303 ADI_SENSE_RESULT adi_sense_Reset( 00304 ADI_SENSE_DEVICE_HANDLE const hDevice); 00305 00306 /*! 00307 * @brief Check if the device is ready, following power-up or a reset. 00308 * 00309 * @param[in] hDevice ADI Sense device context handle 00310 * @param[out] pbReady Pointer to return true if the device is ready, or false 00311 * otherwise 00312 * 00313 * @return Status 00314 * - #ADI_SENSE_SUCCESS Call completed successfully. 00315 * 00316 * @details This function attempts to read a fixed-value device register via 00317 * the communication interface. 00318 */ 00319 ADI_SENSE_RESULT adi_sense_GetDeviceReadyState( 00320 ADI_SENSE_DEVICE_HANDLE const hDevice, 00321 bool_t * const pbReady); 00322 00323 /*! 00324 * @brief Obtain the product ID from the device. 00325 * 00326 * @param[in] hDevice ADI Sense device context handle 00327 * @param[out] pProductId Pointer to return the product ID value 00328 * 00329 * @return Status 00330 * - #ADI_SENSE_SUCCESS Call completed successfully. 00331 * 00332 * @details Reads the product ID registers on the device and returns the value. 00333 */ 00334 ADI_SENSE_RESULT adi_sense_GetProductID( 00335 ADI_SENSE_DEVICE_HANDLE const hDevice, 00336 ADI_SENSE_PRODUCT_ID * const pProductId); 00337 00338 /*! 00339 * @brief Write full configuration settings to the device registers. 00340 * 00341 * @param[in] hDevice ADI Sense device context handle 00342 * @param[out] pConfig Pointer to the configuration data structure 00343 * 00344 * @return Status 00345 * - #ADI_SENSE_SUCCESS Call completed successfully. 00346 * 00347 * @details Translates configuration details provided into device-specific 00348 * register settings and updates device configuration registers. 00349 * 00350 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00351 */ 00352 ADI_SENSE_RESULT adi_sense_SetConfig( 00353 ADI_SENSE_DEVICE_HANDLE const hDevice, 00354 ADI_SENSE_CONFIG * const pConfig); 00355 00356 /*! 00357 * @brief Apply the configuration settings currently stored in device registers 00358 * 00359 * @param[in] hDevice ADI Sense device context handle 00360 * 00361 * @return Status 00362 * - #ADI_SENSE_SUCCESS Call completed successfully. 00363 * 00364 * @details Instructs the ADI Sense device to reload and apply configuration 00365 * from the device configuration registers. Changes to configuration 00366 * registers are ignored by the device until this function is called. 00367 * 00368 * @note No other command must be running when this is called. 00369 */ 00370 ADI_SENSE_RESULT adi_sense_ApplyConfigUpdates( 00371 ADI_SENSE_DEVICE_HANDLE const hDevice); 00372 00373 /*! 00374 * @brief Store the configuration settings to persistent memory on the device. 00375 * 00376 * @param[in] hDevice ADI Sense device context handle 00377 * 00378 * @return Status 00379 * - #ADI_SENSE_SUCCESS Call completed successfully. 00380 * 00381 * @details Instructs the ADI Sense device to save the current contents of its 00382 * device configuration registers to non-volatile memory. 00383 * 00384 * @note No other command must be running when this is called. 00385 * @note Do not power down the device while this command is running. 00386 */ 00387 ADI_SENSE_RESULT adi_sense_SaveConfig( 00388 ADI_SENSE_DEVICE_HANDLE const hDevice); 00389 00390 /*! 00391 * @brief Restore configuration settings from persistent memory on the device. 00392 * 00393 * @param[in] hDevice ADI Sense device context handle 00394 * 00395 * @return Status 00396 * - #ADI_SENSE_SUCCESS Call completed successfully. 00397 * 00398 * @details Instructs the ADI Sense device to restore the contents of its 00399 * device configuration registers from non-volatile memory. 00400 * 00401 * @note No other command must be running when this is called. 00402 */ 00403 ADI_SENSE_RESULT adi_sense_RestoreConfig( 00404 ADI_SENSE_DEVICE_HANDLE const hDevice); 00405 00406 /*! 00407 * @brief Store the LUT data to persistent memory on the device. 00408 * 00409 * @param[in] hDevice ADI Sense device context handle 00410 * 00411 * @return Status 00412 * - #ADI_SENSE_SUCCESS Call completed successfully. 00413 * 00414 * @details Instructs the ADI Sense device to save the current contents of its 00415 * LUT data buffer, set using @ref adi_sense_SetLutData(), to 00416 * non-volatile memory. 00417 * 00418 * @note No other command must be running when this is called. 00419 * @note Do not power down the device while this command is running. 00420 */ 00421 ADI_SENSE_RESULT adi_sense_SaveLutData( 00422 ADI_SENSE_DEVICE_HANDLE const hDevice); 00423 00424 /*! 00425 * @brief Restore LUT data from persistent memory on the device. 00426 * 00427 * @param[in] hDevice ADI Sense device context handle 00428 * 00429 * @return Status 00430 * - #ADI_SENSE_SUCCESS Call completed successfully. 00431 * 00432 * @details Instructs the ADI Sense device to restore the contents of its 00433 * LUT data, previously stored with @ref adi_sense_SaveLutData, from 00434 * non-volatile memory. 00435 * 00436 * @note No other command must be running when this is called. 00437 */ 00438 ADI_SENSE_RESULT adi_sense_RestoreLutData( 00439 ADI_SENSE_DEVICE_HANDLE const hDevice); 00440 00441 /*! 00442 * @brief Start the measurement cycles on the device. 00443 * 00444 * @param[in] hDevice ADI Sense device context handle 00445 * @param[in] bMeasurementMode Allows a choice of special modes for the 00446 * measurement. See @ref ADI_SENSE_MEASUREMENT_MODE 00447 * for further information. 00448 * 00449 * @return Status 00450 * - #ADI_SENSE_SUCCESS Call completed successfully. 00451 * 00452 * @details Instructs the ADI Sense device to start executing measurement cycles 00453 * according to the current applied configuration settings. The 00454 * DATAREADY status signal will be asserted whenever new measurement 00455 * data is published, according to selected settings. 00456 * Measurement cycles may be stopped by calling @ref 00457 * adi_sense_StopMeasurement. 00458 * 00459 * @note No other command must be running when this is called. 00460 */ 00461 ADI_SENSE_RESULT adi_sense_StartMeasurement( 00462 ADI_SENSE_DEVICE_HANDLE const hDevice, 00463 ADI_SENSE_MEASUREMENT_MODE const eMeasurementMode); 00464 00465 /*! 00466 * @brief Stop the measurement cycles on the device. 00467 * 00468 * @param[in] hDevice ADI Sense device context handle 00469 * 00470 * @return Status 00471 * - #ADI_SENSE_SUCCESS Call completed successfully. 00472 * 00473 * @details Instructs the ADI Sense device to stop executing measurement cycles. 00474 * The command may be delayed until the current conversion, if any, has 00475 * been completed and published. 00476 * 00477 * @note To be used only if a measurement command is currently running. 00478 */ 00479 ADI_SENSE_RESULT adi_sense_StopMeasurement( 00480 ADI_SENSE_DEVICE_HANDLE const hDevice); 00481 00482 /*! 00483 * @brief Run built-in diagnostic checks on the device. 00484 * 00485 * @param[in] hDevice ADI Sense device context handle 00486 * 00487 * @return Status 00488 * - #ADI_SENSE_SUCCESS Call completed successfully. 00489 * 00490 * @details Instructs the ADI Sense device to execute its built-in diagnostic 00491 * tests, on any enabled measurement channels, according to the current 00492 * applied configuration settings. Device status registers will be 00493 * updated to indicate if any errors were detected by the diagnostics. 00494 * 00495 * @note No other command must be running when this is called. 00496 */ 00497 ADI_SENSE_RESULT adi_sense_RunDiagnostics( 00498 ADI_SENSE_DEVICE_HANDLE const hDevice); 00499 00500 /*! 00501 * @brief Run built-in calibration on the device. 00502 * 00503 * @param[in] hDevice ADI Sense device context handle 00504 * 00505 * @return Status 00506 * - #ADI_SENSE_SUCCESS Call completed successfully. 00507 * 00508 * @details Instructs the ADI Sense device to execute its self-calibration 00509 * routines, on any enabled measurement channels, according to the 00510 * current applied configuration settings. Device status registers 00511 * will be updated to indicate if any errors were detected. 00512 * 00513 * @note No other command must be running when this is called. 00514 */ 00515 ADI_SENSE_RESULT adi_sense_RunCalibration( 00516 ADI_SENSE_DEVICE_HANDLE const hDevice); 00517 00518 /*! 00519 * @brief Read the current status from the device registers. 00520 * 00521 * @param[in] hDevice ADI Sense device context handle 00522 * @param[out] pStatus Pointer to return the status summary obtained from the 00523 * device. 00524 * 00525 * @return Status 00526 * - #ADI_SENSE_SUCCESS Call completed successfully. 00527 * 00528 * @details Reads the status registers and extracts the relevant information 00529 * to return to the caller. 00530 * 00531 * @note This may be called at any time, assuming the device is ready. 00532 */ 00533 ADI_SENSE_RESULT adi_sense_GetStatus( 00534 ADI_SENSE_DEVICE_HANDLE const hDevice, 00535 ADI_SENSE_STATUS * const pStatus); 00536 00537 /*! 00538 * @brief Read measurement data samples from the device registers. 00539 * 00540 * @param[in] hDevice ADI Sense device context handle 00541 * @param[in] bMeasurementMode Must be set to the same value used for @ref 00542 * adi_sense_StartMeasurement(). 00543 * @param[out] pSamples Pointer to return a set of requested data samples. 00544 * @param[in] nRequested Number of requested data samples. 00545 * @param[out] pnReturned Number of valid data samples successfully retrieved. 00546 * 00547 * @return Status 00548 * - #ADI_SENSE_SUCCESS Call completed successfully. 00549 * 00550 * @details Reads the status registers and extracts the relevant information 00551 * to return to the caller. 00552 * 00553 * @note This is intended to be called only when the DATAREADY status signal 00554 * is asserted. 00555 */ 00556 ADI_SENSE_RESULT adi_sense_GetData( 00557 ADI_SENSE_DEVICE_HANDLE const hDevice, 00558 ADI_SENSE_MEASUREMENT_MODE const eMeasurementMode, 00559 ADI_SENSE_DATA_SAMPLE * const pSamples, 00560 uint32_t const nRequested, 00561 uint32_t * const pnReturned); 00562 00563 /*! 00564 * @brief Check if a command is currently running on the device. 00565 * 00566 * @param[in] hDevice ADI Sense device context handle 00567 * @param[out] pbCommandRunning Pointer to return the command running status 00568 * 00569 * @return Status 00570 * - #ADI_SENSE_SUCCESS Call completed successfully. 00571 * 00572 * @details Reads the device status register to check if a command is running. 00573 */ 00574 ADI_SENSE_RESULT adi_sense_GetCommandRunningState( 00575 ADI_SENSE_DEVICE_HANDLE hDevice, 00576 bool_t *pbCommandRunning); 00577 00578 #ifdef __cplusplus 00579 } 00580 #endif 00581 00582 /*! 00583 * @} 00584 */ 00585 00586 #endif /* __ADI_SENSE_API_H__ */ 00587
Generated on Tue Jul 12 2022 21:13:17 by
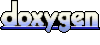