
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_1000_sensor_types.h
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_1000_sensor_types.h 00004 * @brief: Sensor type definitions for ADI Sense 1000. 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /* 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 */ 00042 00043 #ifndef __ADI_SENSE_1000_SENSOR_TYPES_H__ 00044 #define __ADI_SENSE_1000_SENSOR_TYPES_H__ 00045 00046 /*! @addtogroup ADI_Sense_1000_Api ADI Sense 1000 Host Library API 00047 * @{ 00048 */ 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif 00053 00054 /*! ADI Sense 1000 measurement channel identifiers */ 00055 typedef enum { 00056 ADI_SENSE_1000_CHANNEL_ID_NONE = -1, 00057 /*!< Used to indicate when no channel is selected (e.g. compensation channel) */ 00058 00059 ADI_SENSE_1000_CHANNEL_ID_CJC_0 = 0, 00060 /*!< Cold-Juction Compensation channel #0 */ 00061 ADI_SENSE_1000_CHANNEL_ID_CJC_1 , 00062 /*!< Cold-Juction Compensation channel #1 */ 00063 ADI_SENSE_1000_CHANNEL_ID_SENSOR_0 , 00064 /*!< Analog Sensor channel #0 */ 00065 ADI_SENSE_1000_CHANNEL_ID_SENSOR_1 , 00066 /*!< Analog Sensor channel #1 */ 00067 ADI_SENSE_1000_CHANNEL_ID_SENSOR_2 , 00068 /*!< Analog Sensor channel #2 */ 00069 ADI_SENSE_1000_CHANNEL_ID_SENSOR_3 , 00070 /*!< Analog Sensor channel #3 */ 00071 ADI_SENSE_1000_CHANNEL_ID_VOLTAGE_0 , 00072 /*!< Analog 0-10V Voltage Sensor channel #0 */ 00073 ADI_SENSE_1000_CHANNEL_ID_CURRENT_0 , 00074 /*!< Analog 4-20mA Current Sensor channel #0 */ 00075 ADI_SENSE_1000_CHANNEL_ID_I2C_0 , 00076 /*!< Digital I2C Sensor channel #0 */ 00077 ADI_SENSE_1000_CHANNEL_ID_I2C_1 , 00078 /*!< Digital I2C Sensor channel #1 */ 00079 ADI_SENSE_1000_CHANNEL_ID_SPI_0 , 00080 /*!< Digital SPI Sensor channel #0 */ 00081 ADI_SENSE_1000_CHANNEL_ID_SPI_1 , 00082 /*!< Digital SPI Sensor channel #1 */ 00083 ADI_SENSE_1000_CHANNEL_ID_SPI_2 , 00084 /*!< Digital SPI Sensor channel #2 */ 00085 00086 ADI_SENSE_1000_MAX_CHANNELS , 00087 /*!< Maximum number of measurement channels on the ADI Sense 1000 */ 00088 } ADI_SENSE_1000_CHANNEL_ID ; 00089 00090 /*! ADI Sense 1000 analog sensor type options 00091 * 00092 * Select the sensor type that is connected to an ADC analog measurement 00093 * channel. 00094 * 00095 * @note Some channels may only support a subset of the available sensor types 00096 * below. 00097 * 00098 * @note The sensor type name may include a classification suffix: 00099 * - _DEF_L1: pre-defined sensor using built-in linearisation data 00100 * - _DEF_L2: pre-defined sensor using user-supplied linearisation data 00101 * Where the suffix is absent, assume the _DEF_L1 classification above. 00102 */ 00103 typedef enum { 00104 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_T_DEF_L1 = 0, 00105 /*!< Standard T-type Thermocouple temperature sensor with default 00106 * linearisation and default configuration options 00107 * 00108 * @note For use with Analog Sensor channels only 00109 */ 00110 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_J_DEF_L1 = 1, 00111 /*!< Standard J-type Thermocouple temperature sensor with default 00112 * linearisation and default configuration options 00113 * 00114 * @note For use with Analog Sensor channels only 00115 */ 00116 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_K_DEF_L1 = 2, 00117 /*!< Standard K-type Thermocouple temperature sensor with default 00118 * linearisation and default configuration options 00119 * 00120 * @note For use with Analog Sensor channels only 00121 */ 00122 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_1_DEF_L2 = 12, 00123 /*!< Standard thermocouple temperature sensor with user-defined 00124 * linearisation and default configuration options 00125 * 00126 * @note For use with Analog Sensor channels only 00127 */ 00128 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_2_DEF_L2 = 13, 00129 /*!< Standard thermocouple temperature sensor with user-defined 00130 * linearisation and default configuration options 00131 * 00132 * @note For use with Analog Sensor channels only 00133 */ 00134 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_3_DEF_L2 = 14, 00135 /*!< Standard thermocouple temperature sensor with user-defined 00136 * linearisation and default configuration options 00137 * 00138 * @note For use with Analog Sensor channels only 00139 */ 00140 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_4_DEF_L2 = 15, 00141 /*!< Standard thermocouple temperature sensor with user-defined 00142 * linearisation and default configuration options 00143 * 00144 * @note For use with Analog Sensor channels only 00145 */ 00146 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_T_ADV_L1 = 16, 00147 /*!< T-type thermocouple temperature sensor with default linearisation and 00148 * advanced configuration options 00149 * 00150 * @note For use with Analog Sensor channels only 00151 */ 00152 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_K_ADV_L1 = 17, 00153 /*!< T-type thermocouple temperature sensor with default linearisation and 00154 * advanced configuration options 00155 * 00156 * @note For use with Analog Sensor channels only 00157 */ 00158 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_J_ADV_L1 = 18, 00159 /*!< T-type thermocouple temperature sensor with default linearisation and 00160 * advanced configuration options 00161 * 00162 * @note For use with Analog Sensor channels only 00163 */ 00164 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_1_ADV_L2 = 28, 00165 /*!< Thermocouple temperature sensor with user-defined 00166 * linearisation and advanced configuration options 00167 * 00168 * @note For use with Analog Sensor channels only 00169 */ 00170 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_2_ADV_L2 = 29, 00171 /*!< Thermocouple temperature sensor with user-defined 00172 * linearisation and advanced configuration options 00173 * 00174 * @note For use with Analog Sensor channels only 00175 */ 00176 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_3_ADV_L2 = 30, 00177 /*!< Thermocouple temperature sensor with user-defined 00178 * linearisation and advanced configuration options 00179 * 00180 * @note For use with Analog Sensor channels only 00181 */ 00182 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_4_ADV_L2 = 31, 00183 /*!< Thermocouple temperature sensor with user-defined 00184 * linearisation and advanced configuration options 00185 * 00186 * @note For use with Analog Sensor channels only 00187 */ 00188 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT100_DEF_L1 = 32, 00189 /*!< Standard 2-wire PT100 RTD temperature sensor with default 00190 * linearisation and default configuration options 00191 * 00192 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00193 * only 00194 */ 00195 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT1000_DEF_L1 = 33, 00196 /*!< Standard 2-wire PT1000 RTD temperature sensor with default 00197 * linearisation and default configuration options 00198 * 00199 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00200 * only 00201 */ 00202 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_1_DEF_L2 = 44, 00203 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00204 * default configuration options 00205 * 00206 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00207 * only 00208 */ 00209 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_2_DEF_L2 = 45, 00210 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00211 * default configuration options 00212 * 00213 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00214 * only 00215 */ 00216 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_3_DEF_L2 = 46, 00217 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00218 * default configuration options 00219 * 00220 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00221 * only 00222 */ 00223 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_4_DEF_L2 = 47, 00224 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00225 * default configuration options 00226 * 00227 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00228 * only 00229 */ 00230 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT100_ADV_L1 = 48, 00231 /*!< Standard 2-wire PT100 RTD temperature sensor with default 00232 * linearisation and advanced configuration options 00233 * 00234 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00235 * only 00236 */ 00237 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT1000_ADV_L1 = 49, 00238 /*!< Standard 2-wire PT1000 RTD temperature sensor with default 00239 * linearisation and advanced configuration options 00240 * 00241 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00242 * only 00243 */ 00244 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_1_ADV_L2 = 60, 00245 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00246 * advanced configuration options 00247 * 00248 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00249 * only 00250 */ 00251 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_2_ADV_L2 = 61, 00252 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00253 * advanced configuration options 00254 * 00255 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00256 * only 00257 */ 00258 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_3_ADV_L2 = 62, 00259 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00260 * advanced configuration options 00261 * 00262 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00263 * only 00264 */ 00265 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_4_ADV_L2 = 63, 00266 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00267 * advanced configuration options 00268 * 00269 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00270 * only 00271 */ 00272 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT100_DEF_L1 = 64, 00273 /*!< Standard 3-wire PT100 RTD temperature sensor with default 00274 * linearisation and default configuration options 00275 * 00276 * @note For use with Analog Sensor channels only 00277 */ 00278 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT1000_DEF_L1 = 65, 00279 /*!< Standard 3-wire PT1000 RTD temperature sensor with default 00280 * linearisation and default configuration options 00281 * 00282 * @note For use with Analog Sensor channels only 00283 */ 00284 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_1_DEF_L2 = 76, 00285 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00286 * default configuration options 00287 * 00288 * @note For use with Analog Sensor channels only 00289 */ 00290 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_2_DEF_L2 = 77, 00291 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00292 * default configuration options 00293 * 00294 * @note For use with Analog Sensor channels only 00295 */ 00296 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_3_DEF_L2 = 78, 00297 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00298 * default configuration options 00299 * 00300 * @note For use with Analog Sensor channels only 00301 */ 00302 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_4_DEF_L2 = 79, 00303 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00304 * default configuration options 00305 * 00306 * @note For use with Analog Sensor channels only 00307 */ 00308 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT100_ADV_L1 = 80, 00309 /*!< Standard 3-wire PT100 RTD temperature sensor with default 00310 * linearisation and advanced configuration options 00311 * 00312 * @note For use with Analog Sensor channels only 00313 */ 00314 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT1000_ADV_L1 = 81, 00315 /*!< Standard 3-wire PT1000 RTD temperature sensor with default 00316 * linearisation and advanced configuration options 00317 * 00318 * @note For use with Analog Sensor channels only 00319 */ 00320 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_1_ADV_L2 = 92, 00321 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00322 * advanced configuration options 00323 * 00324 * @note For use with Analog Sensor channels only 00325 */ 00326 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_2_ADV_L2 = 93, 00327 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00328 * advanced configuration options 00329 * 00330 * @note For use with Analog Sensor channels only 00331 */ 00332 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_3_ADV_L2 = 94, 00333 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00334 * advanced configuration options 00335 * 00336 * @note For use with Analog Sensor channels only 00337 */ 00338 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_4_ADV_L2 = 95, 00339 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00340 * advanced configuration options 00341 * 00342 * @note For use with Analog Sensor channels only 00343 */ 00344 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT100_DEF_L1 = 96, 00345 /*!< Standard 4-wire PT100 RTD temperature sensor with default 00346 * linearisation and default configuration options 00347 * 00348 * @note For use with Analog Sensor channels only 00349 */ 00350 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT1000_DEF_L1 = 97, 00351 /*!< Standard 4-wire PT1000 RTD temperature sensor with default 00352 * linearisation and default configuration options 00353 * 00354 * @note For use with Analog Sensor channels only 00355 */ 00356 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_1_DEF_L2 = 108, 00357 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00358 * default configuration options 00359 * 00360 * @note For use with Analog Sensor channels only 00361 */ 00362 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_2_DEF_L2 = 109, 00363 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00364 * default configuration options 00365 * 00366 * @note For use with Analog Sensor channels only 00367 */ 00368 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_3_DEF_L2 = 110, 00369 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00370 * default configuration options 00371 * 00372 * @note For use with Analog Sensor channels only 00373 */ 00374 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_4_DEF_L2 = 111, 00375 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00376 * default configuration options 00377 * 00378 * @note For use with Analog Sensor channels only 00379 */ 00380 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT100_ADV_L1 = 112, 00381 /*!< Standard 4-wire PT100 RTD temperature sensor with default 00382 * linearisation and advanced configuration options 00383 * 00384 * @note For use with Analog Sensor channels only 00385 */ 00386 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT1000_ADV_L1 = 113, 00387 /*!< Standard 4-wire PT1000 RTD temperature sensor with default 00388 * linearisation and advanced configuration options 00389 * 00390 * @note For use with Analog Sensor channels only 00391 */ 00392 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_1_ADV_L2 = 124, 00393 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00394 * advanced configuration options 00395 * 00396 * @note For use with Analog Sensor channels only 00397 */ 00398 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_2_ADV_L2 = 125, 00399 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00400 * advanced configuration options 00401 * 00402 * @note For use with Analog Sensor channels only 00403 */ 00404 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_3_ADV_L2 = 126, 00405 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00406 * advanced configuration options 00407 * 00408 * @note For use with Analog Sensor channels only 00409 */ 00410 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_4_ADV_L2 = 127, 00411 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00412 * advanced configuration options 00413 * 00414 * @note For use with Analog Sensor channels only 00415 */ 00416 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_A_10K_DEF_L1 = 128, 00417 /*!< Standard 10kOhm NTC Thermistor temperature sensor with Steinhart–Hart 00418 * linearisation equation and default configuration options 00419 * 00420 * @note For use with Analog Sensor channels only 00421 */ 00422 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_B_10K_DEF_L1 = 129, 00423 /*!< Standard 10kOhm NTC Thermistor temperature sensor with Beta 00424 * linearisation equation and default configuration options 00425 * 00426 * @note For use with Analog Sensor channels only 00427 */ 00428 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_1_DEF_L2 = 140, 00429 /*!< Thermistor sensor with user-defined linearisation and 00430 * default configuration options 00431 * 00432 * @note For use with Analog Sensor channels only 00433 */ 00434 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_2_DEF_L2 = 141, 00435 /*!< Thermistor sensor with user-defined linearisation and 00436 * default configuration options 00437 * 00438 * @note For use with Analog Sensor channels only 00439 */ 00440 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_3_DEF_L2 = 142, 00441 /*!< Thermistor sensor with user-defined linearisation and 00442 * default configuration options 00443 * 00444 * @note For use with Analog Sensor channels only 00445 */ 00446 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_4_DEF_L2 = 143, 00447 /*!< Thermistor sensor with user-defined linearisation and 00448 * default configuration options 00449 * 00450 * @note For use with Analog Sensor channels only 00451 */ 00452 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_A_10K_ADV_L1 = 144, 00453 /*!< 10kOhm NTC Thermistor temperature sensor with Steinhart–Hart 00454 * linearisation equation and advanced configuration options 00455 * 00456 * @note For use with Analog Sensor channels only 00457 */ 00458 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_B_10K_ADV_L1 = 145, 00459 /*!< 10kOhm NTC Thermistor temperature sensor with Beta 00460 * linearisation equation and advanced configuration options 00461 * 00462 * @note For use with Analog Sensor channels only 00463 */ 00464 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_1_ADV_L2 = 156, 00465 /*!< Thermistor sensor with user-defined linearisation and 00466 * advanced configuration options 00467 * 00468 * @note For use with Analog Sensor channels only 00469 */ 00470 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_2_ADV_L2 = 157, 00471 /*!< Thermistor sensor with user-defined linearisation and 00472 * advanced configuration options 00473 * 00474 * @note For use with Analog Sensor channels only 00475 */ 00476 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_3_ADV_L2 = 158, 00477 /*!< Thermistor sensor with user-defined linearisation and 00478 * advanced configuration options 00479 * 00480 * @note For use with Analog Sensor channels only 00481 */ 00482 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_4_ADV_L2 = 159, 00483 /*!< Thermistor sensor with user-defined linearisation and 00484 * advanced configuration options 00485 * 00486 * @note For use with Analog Sensor channels only 00487 */ 00488 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_1_DEF_L2 = 160, 00489 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00490 * linearisation and default configuration options 00491 * 00492 * @note For use with Analog Sensor channels only 00493 * @note Bridge Excition Voltage must be selected as reference 00494 */ 00495 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_2_DEF_L2 = 161, 00496 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00497 * linearisation and default configuration options 00498 * 00499 * @note For use with Analog Sensor channels only 00500 * @note Bridge Excition Voltage must be selected as reference 00501 */ 00502 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_3_DEF_L2 = 162, 00503 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00504 * linearisation and default configuration options 00505 * 00506 * @note For use with Analog Sensor channels only 00507 * @note Bridge Excition Voltage must be selected as reference 00508 */ 00509 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_4_DEF_L2 = 163, 00510 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00511 * linearisation and default configuration options 00512 * 00513 * @note For use with Analog Sensor channels only 00514 * @note Bridge Excition Voltage must be selected as reference 00515 */ 00516 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_1_ADV_L2 = 176, 00517 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00518 * linearisation and advanced configuration options 00519 * 00520 * @note For use with Analog Sensor channels only 00521 * @note Bridge Excition Voltage must be selected as reference 00522 */ 00523 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_2_ADV_L2 = 177, 00524 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00525 * linearisation and advanced configuration options 00526 * 00527 * @note For use with Analog Sensor channels only 00528 * @note Bridge Excition Voltage must be selected as reference 00529 */ 00530 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_3_ADV_L2 = 178, 00531 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00532 * linearisation and advanced configuration options 00533 * 00534 * @note For use with Analog Sensor channels only 00535 * @note Bridge Excition Voltage must be selected as reference 00536 */ 00537 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_4_ADV_L2 = 179, 00538 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00539 * linearisation and advanced configuration options 00540 * 00541 * @note For use with Analog Sensor channels only 00542 * @note Bridge Excition Voltage must be selected as reference 00543 */ 00544 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_1_DEF_L2 = 192, 00545 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00546 * linearisation and default configuration options 00547 * 00548 * @note For use with Analog Sensor channels only 00549 * @note Bridge Excition Voltage must be selected as reference 00550 */ 00551 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_2_DEF_L2 = 193, 00552 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00553 * linearisation and default configuration options 00554 * 00555 * @note For use with Analog Sensor channels only 00556 * @note Bridge Excition Voltage must be selected as reference 00557 */ 00558 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_3_DEF_L2 = 194, 00559 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00560 * linearisation and default configuration options 00561 * 00562 * @note For use with Analog Sensor channels only 00563 * @note Bridge Excition Voltage must be selected as reference 00564 */ 00565 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_4_DEF_L2 = 195, 00566 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00567 * linearisation and default configuration options 00568 * 00569 * @note For use with Analog Sensor channels only 00570 * @note Bridge Excition Voltage must be selected as reference 00571 */ 00572 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_1_ADV_L2 = 208, 00573 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00574 * linearisation and advanced configuration options 00575 * 00576 * @note For use with Analog Sensor channels only 00577 * @note Bridge Excition Voltage must be selected as reference 00578 */ 00579 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_2_ADV_L2 = 209, 00580 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00581 * linearisation and advanced configuration options 00582 * 00583 * @note For use with Analog Sensor channels only 00584 * @note Bridge Excition Voltage must be selected as reference 00585 */ 00586 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_3_ADV_L2 = 210, 00587 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00588 * linearisation and advanced configuration options 00589 * 00590 * @note For use with Analog Sensor channels only 00591 * @note Bridge Excition Voltage must be selected as reference 00592 */ 00593 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_4_ADV_L2 = 211, 00594 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00595 * linearisation and advanced configuration options 00596 * 00597 * @note For use with Analog Sensor channels only 00598 * @note Bridge Excition Voltage must be selected as reference 00599 */ 00600 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE = 256, 00601 /*!< Generic voltage sensor with no linearisation applied 00602 * 00603 * @note For use with Analog 0-10V Voltage Sensor channels only 00604 */ 00605 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_HONEYWELL_TRUSTABILITY = 272, 00606 /*!< Honeywell Pressure voltage sensor (HSCMRNN1.6BAAA3) with default 00607 * linearisation and default configuration options 00608 * 00609 * @note For use with Analog 0-10V Voltage Sensor channels only 00610 */ 00611 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_AMPHENOL_NPA300X = 273, 00612 /*!< Amphenol Pressure voltage sensor (NPA-300B-015A) with default 00613 * linearisation and default configuration options 00614 * 00615 * @note For use with Analog 0-10V Voltage Sensor channels only 00616 */ 00617 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_3_DEF = 274, 00618 /*!< Generic pressure voltage sensor with user-defined 00619 * linearisation and default configuration options 00620 * 00621 * @note For use with Analog 0-10V Voltage Sensor channels only 00622 */ 00623 ADI_SENSE_1000_ADC_SENSOR_CURRENT = 384, 00624 /*!< Generic current sensor with no linearisation applied 00625 * 00626 * @note For use with Analog 4-20mA Current Sensor channels only 00627 */ 00628 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_HONEYWELL_PX2 = 385, 00629 /*!< Honeywell Pressure current sensor (PX2CN2XX100PACH) with default 00630 * linearisation and default configuration options 00631 * 00632 * @note For use with Analog 4-20mA Current Sensor channels only 00633 */ 00634 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_2_DEF = 386, 00635 /*!< Generic pressure current sensor with user-defined 00636 * linearisation and default configuration options 00637 * 00638 * @note For use with Analog 4-20mA Current Sensor channels only 00639 */ 00640 } ADI_SENSE_1000_ADC_SENSOR_TYPE ; 00641 00642 /*! ADI Sense 1000 I2C digital sensor type options 00643 * 00644 * Select the sensor type that is connected to an I2C digital measurement 00645 * channel. 00646 * 00647 * @note These are pre-defined sensors using built-in linearisation data 00648 */ 00649 typedef enum { 00650 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_HONEYWELL_HUMIDICON = 2112, 00651 /*!< Honeywell HiH9000-series humidity sensor with default linearisation 00652 * and default configuration options 00653 * 00654 * @note For use with I2C Digital Sensor channels only 00655 */ 00656 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_SENSIRION_SHT3X = 2113, 00657 /*!< Sensirion SHT35-DIS-B humidity sensor with default linearisation 00658 * and default configuration options 00659 * 00660 * @note For use with I2C Digital Sensor channels only 00661 */ 00662 } ADI_SENSE_1000_I2C_SENSOR_TYPE ; 00663 00664 /*! ADI Sense 1000 SPI digital sensor type options 00665 * 00666 * Select the sensor type that is connected to an SPI digital measurement 00667 * channel. 00668 * 00669 * @note These are pre-defined sensors using built-in linearisation data 00670 */ 00671 typedef enum { 00672 ADI_SENSE_1000_SPI_SENSOR_PRESSURE_HONEYWELL_TRUSTABILITY = 3072, 00673 /*!< Honeywell HSCDRNN1.6BASA3 pressure sensor with default linearisation 00674 * and default configuration options 00675 * 00676 * @note For use with SPI Digital Sensor channels only 00677 */ 00678 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_ADI_ADXL362 = 3200, 00679 /*!< Analog Devices ADxL362 3-axis accelerometer sensor with default 00680 * linearisation and default configuration options(*) 00681 * 00682 * @note (*) Custom configuration command can be optionally specified 00683 * 00684 * @note For use with SPI Digital Sensor channels only 00685 * 00686 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00687 * the sensor measurements from the X/Y/Z axes each output on a 00688 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00689 */ 00690 } ADI_SENSE_1000_SPI_SENSOR_TYPE ; 00691 00692 #ifdef __cplusplus 00693 } 00694 #endif 00695 00696 /*! 00697 * @} 00698 */ 00699 00700 #endif /* __ADI_SENSE_1000_SENSOR_TYPES_H__ */ 00701
Generated on Tue Jul 12 2022 21:13:17 by
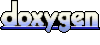