
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_1000_lut_data.h
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_lut_data_types.h 00004 * @brief: Look-Up Table data-type definitions for ADI Sense API. 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /* 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 */ 00042 00043 #ifndef __ADI_SENSE_1000_LUT_DATA_H__ 00044 #define __ADI_SENSE_1000_LUT_DATA_H__ 00045 00046 #include "adi_sense_types.h" 00047 #include "adi_sense_1000_sensor_types.h" 00048 00049 /*! @addtogroup ADI_Sense_1000_Api ADI Sense 1000 Host Library API 00050 * @{ 00051 */ 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 /*! LUT data validation signature */ 00058 #define ADI_SENSE_LUT_SIGNATURE 0x4C555473 00059 00060 /*! LUT data CRC-16-CCITT seed value */ 00061 #define ADI_SENSE_LUT_CRC_SEED 0x4153 00062 00063 /*! LUT maximum allowed size */ 00064 #define ADI_SENSE_LUT_MAX_SIZE 12288U 00065 00066 /*! Linearisation look-up table / co-efficient list geometry */ 00067 typedef enum { 00068 ADI_SENSE_1000_LUT_GEOMETRY_RESERVED = 0x00, 00069 /**< reserved - for internal use only */ 00070 ADI_SENSE_1000_LUT_GEOMETRY_COEFFS = 0x01, 00071 /**< 1-dimensional equation coefficient list */ 00072 ADI_SENSE_1000_LUT_GEOMETRY_NES_1D = 0x02, 00073 /**< 1-dimensional not-equally-spaced look-up table */ 00074 ADI_SENSE_1000_LUT_GEOMETRY_NES_2D = 0x03, 00075 /**< 2-dimensional not-equally-spaced look-up table */ 00076 ADI_SENSE_1000_LUT_GEOMETRY_ES_1D = 0x04, 00077 /**< 1-dimensional equally-spaced look-up table */ 00078 ADI_SENSE_1000_LUT_GEOMETRY_ES_2D = 0x05, 00079 /**< 2-dimensional equally-spaced look-up table */ 00080 } ADI_SENSE_1000_LUT_GEOMETRY ; 00081 00082 /*! Linearisation equation type */ 00083 typedef enum { 00084 ADI_SENSE_1000_LUT_EQUATION_POLYN, 00085 /**< Polynomial equation, typically used for Thermocouple and RTD 00086 * linearisation */ 00087 ADI_SENSE_1000_LUT_EQUATION_POLYNEXP, 00088 /**< Polynomial + exponential equation, typically used for Thermocouple 00089 * inverse linearisation */ 00090 ADI_SENSE_1000_LUT_EQUATION_QUADRATIC, 00091 /**< Quadratic linearisation equation, typically used for RTD 00092 * linearisation */ 00093 ADI_SENSE_1000_LUT_EQUATION_STEINHART, 00094 /**< Steinhart-Hart equation, typically used for Thermistor 00095 * linearisation */ 00096 ADI_SENSE_1000_LUT_EQUATION_LOGARITHMIC, 00097 /**< Beta-based logarithmic equation, typically used for Thermistor 00098 * linearisation */ 00099 ADI_SENSE_1000_LUT_EQUATION_EXPONENTIAL, 00100 /**< Exponential equation */ 00101 ADI_SENSE_1000_LUT_EQUATION_BIVARIATE_POLYN, 00102 /**< Bi-variate polynomial equation, typically used for bridge pressure 00103 * sensor linearisation 00104 * @note 2nd-degree is the maximum currently supported 00105 */ 00106 ADI_SENSE_1000_LUT_EQUATION_COUNT, 00107 /**< Enum count value - for internal use only */ 00108 ADI_SENSE_1000_LUT_EQUATION_LUT, 00109 /**< Hard-coded Look-Up Table - for internal use only */ 00110 } ADI_SENSE_1000_LUT_EQUATION ; 00111 00112 typedef enum { 00113 ADI_SENSE_1000_LUT_TC_DIRECTION_FORWARD, 00114 /**< Thermocouple forward (mV to Celsius) linearisation 00115 * Use this value by default for non-thermocouple sensors */ 00116 ADI_SENSE_1000_LUT_TC_DIRECTION_BACKWARD, 00117 /**< Thermocouple inverse (Celsius to mV) linearisation */ 00118 ADI_SENSE_1000_LUT_TC_DIRECTION_COUNT, 00119 /**< Enum count value - for internal use only */ 00120 } ADI_SENSE_1000_LUT_TC_DIRECTION ; 00121 00122 /*! Linearisation data vector format */ 00123 typedef enum { 00124 ADI_SENSE_1000_LUT_DATA_TYPE_RESERVED = 0, 00125 /**< Reserved - for internal use only */ 00126 ADI_SENSE_1000_LUT_DATA_TYPE_FLOAT32 = 1, 00127 /**< Single-precision 32-bit floating-point */ 00128 ADI_SENSE_1000_LUT_DATA_TYPE_FLOAT64 = 2, 00129 /**< Double-precision 64-bit floating-point */ 00130 } ADI_SENSE_1000_LUT_DATA_TYPE ; 00131 00132 /*! Struct for a list of coefficients to be used in an equation */ 00133 typedef struct __attribute__ ((packed, aligned(4))){ 00134 uint32_t nCoeffs; 00135 /**< number of coefficients */ 00136 float32_t rangeMin; 00137 /**< look-up table range - minimum */ 00138 float32_t rangeMax; 00139 /**< look-up table range - maximum */ 00140 float64_t coeffs[]; 00141 /**< C99 flexible array: sorted by ascending exponent in polynomials */ 00142 } ADI_SENSE_1000_LUT_COEFF_LIST; 00143 00144 /*! Struct for a 1-dimensional equally-spaced look-up table */ 00145 typedef struct __attribute__ ((packed, aligned(4))){ 00146 uint32_t nElements; 00147 /**< number of elements. */ 00148 float32_t initInputValue; 00149 /**< initial input value, corresponding to first table element */ 00150 float32_t inputValueIncrement; 00151 /**< interval between successive input values */ 00152 float32_t lut[]; 00153 /**< C99 flexible array */ 00154 } ADI_SENSE_1000_LUT_1D_ES; 00155 00156 /*! Struct for a 1-dimensional not-equally-spaced look-up table */ 00157 typedef struct __attribute__ ((packed, aligned(4))){ 00158 uint32_t nElements; 00159 /**< number of elements of each array. */ 00160 float32_t lut[]; 00161 /**< C99 flexible array, first X's array then Y's array*/ 00162 } ADI_SENSE_1000_LUT_1D_NES; 00163 00164 /*! Struct for a 2-dimensional equally-spaced look-up table */ 00165 typedef struct __attribute__ ((packed, aligned(4))){ 00166 uint16_t nElementsX; 00167 /**< number of elements for input X. */ 00168 uint16_t nElementsY; 00169 /**< number of elements for input Y. */ 00170 float32_t initInputValueX; 00171 /**< initial X input value */ 00172 float32_t inputValueIncrementX; 00173 /**< interval between successive X input values */ 00174 float32_t initInputValueY; 00175 /**< initial Y input value */ 00176 float32_t inputValueIncrementY; 00177 /**< interval between successive Y input values */ 00178 float32_t lut[]; 00179 /**< C99 flexible array, Z matrix[y][x] */ 00180 } ADI_SENSE_1000_LUT_2D_ES; 00181 00182 /*! Struct for a 2-dimensional not-equally-spaced look-up table */ 00183 typedef struct __attribute__ ((packed, aligned(4))){ 00184 uint16_t nElementsX; 00185 /**< number of elements in array X. */ 00186 uint16_t nElementsY; 00187 /**< number of elements in array Y. */ 00188 float32_t lut[]; 00189 /**< C99 flexible array, Order: X's array, Y's array, Z matrix[y][x] */ 00190 } ADI_SENSE_1000_LUT_2D_NES; 00191 00192 /*! Struct for a 2-dimensional list of coefficients to be used in a 00193 * bi-variate polynomial equation */ 00194 typedef struct __attribute__ ((packed, aligned(4))){ 00195 uint32_t maxDegree; 00196 /**< number of coefficients */ 00197 float32_t rangeMinX; 00198 /**< look-up table range - minimum X input value */ 00199 float32_t rangeMaxX; 00200 /**< look-up table range - maximum X input value */ 00201 float32_t rangeMinY; 00202 /**< look-up table range - minimum Y input value */ 00203 float32_t rangeMaxY; 00204 /**< look-up table range - maximum Y input value */ 00205 float64_t coeffs[]; 00206 /**< C99 flexible array: sorted by ascending X degree then sorted by 00207 * ascending Y exponent */ 00208 } ADI_SENSE_1000_LUT_2D_POLYN_COEFF_LIST; 00209 00210 /*! Macro to calculate the number of elements in 00211 * a @ref ADI_SENSE_1000_LUT_COEFF_LIST table */ 00212 #define ADI_SENSE_1000_LUT_COEFF_LIST_NELEMENTS(_t) \ 00213 ((_t).nCoeffs) 00214 00215 /*! Macro to calculate the number of elements in 00216 * a @ref ADI_SENSE_1000_LUT_1D_ES table */ 00217 #define ADI_SENSE_1000_LUT_1D_ES_NELEMENTS(_t) \ 00218 ((_t).nElements) 00219 00220 /*! Macro to calculate the number of elements in 00221 * a @ref ADI_SENSE_1000_LUT_1D_NES table */ 00222 #define ADI_SENSE_1000_LUT_1D_NES_NELEMENTS(_t) \ 00223 ((_t).nElements * 2) 00224 00225 /*! Macro to calculate the number of elements in 00226 * a @ref ADI_SENSE_1000_LUT_2D_ES table */ 00227 #define ADI_SENSE_1000_LUT_2D_ES_NELEMENTS(_t) \ 00228 ((_t).nElementsX * (_t).nElementsX) 00229 00230 /*! Macro to calculate the number of elements in 00231 * a @ref ADI_SENSE_1000_LUT_2D_NES table */ 00232 #define ADI_SENSE_1000_LUT_2D_NES_NELEMENTS(_t) \ 00233 ((_t).nElementsX + (_t).nElementsY + ((_t).nElementsX * (_t).nElementsY)) 00234 00235 /*! Macro to calculate the number of elements in 00236 * a @ref ADI_SENSE_1000_LUT_2D_POLYN_COEFF_LIST table */ 00237 #define ADI_SENSE_1000_LUT_2D_POLYN_COEFF_LIST_NELEMENTS(_t) \ 00238 (((_t).maxDegree + 1) * ((_t).maxDegree + 2) / 2) 00239 00240 /*! Macro to calculate the storage size in bytes of 00241 * a @ref ADI_SENSE_1000_LUT_COEFF_LIST table */ 00242 #define ADI_SENSE_1000_LUT_COEFF_LIST_SIZE(_t) \ 00243 (sizeof(_t) + (sizeof(float64_t) * ADI_SENSE_1000_LUT_COEFF_LIST_NELEMENTS(_t))) 00244 00245 /*! Macro to calculate the storage size in bytes of 00246 * a @ref ADI_SENSE_1000_LUT_1D_ES table */ 00247 #define ADI_SENSE_1000_LUT_1D_ES_SIZE(_t) \ 00248 (sizeof(_t) + (sizeof(float32_t) * ADI_SENSE_1000_LUT_1D_ES_NELEMENTS(_t))) 00249 00250 /*! Macro to calculate the storage size in bytes of 00251 * a @ref ADI_SENSE_1000_LUT_1D_NES table */ 00252 #define ADI_SENSE_1000_LUT_1D_NES_SIZE(_t) \ 00253 (sizeof(_t) + (sizeof(float32_t) * ADI_SENSE_1000_LUT_1D_NES_NELEMENTS(_t))) 00254 00255 /*! Macro to calculate the storage size in bytes of 00256 * a @ref ADI_SENSE_1000_LUT_2D_ES table */ 00257 #define ADI_SENSE_1000_LUT_2D_ES_SIZE(_t) \ 00258 (sizeof(_t) + (sizeof(float32_t) * ADI_SENSE_1000_LUT_2D_ES_NELEMENTS(_t))) 00259 00260 /*! Macro to calculate the storage size in bytes of 00261 * a @ref ADI_SENSE_1000_LUT_2D_NES table */ 00262 #define ADI_SENSE_1000_LUT_2D_NES_SIZE(_t) \ 00263 (sizeof(_t) + (sizeof(float32_t) * ADI_SENSE_1000_LUT_2D_NES_NELEMENTS(_t))) 00264 00265 /*! Macro to calculate the storage size in bytes of 00266 * a @ref ADI_SENSE_1000_LUT_2D_POLYN_COEFF_LIST table */ 00267 #define ADI_SENSE_1000_LUT_2D_POLYN_COEFF_LIST_SIZE(_t) \ 00268 (sizeof(_t) + (sizeof(float64_t) * ADI_SENSE_1000_LUT_2D_POLYN_COEFF_LIST_NELEMENTS(_t))) 00269 00270 /*! Look-Up Table descriptor */ 00271 typedef union __attribute__ ((packed, aligned(4))) { 00272 struct { 00273 ADI_SENSE_1000_LUT_GEOMETRY geometry : 6; 00274 /**< Table geometry */ 00275 ADI_SENSE_1000_LUT_EQUATION equation : 6; 00276 /**< Equation type */ 00277 ADI_SENSE_1000_LUT_TC_DIRECTION dir : 4; 00278 /**< Thermocouple linearisation direction */ 00279 ADI_SENSE_1000_ADC_SENSOR_TYPE sensor : 12; 00280 /**< Sensor Type ID */ 00281 ADI_SENSE_1000_LUT_DATA_TYPE dataType : 4; 00282 /**< Table vector data type */ 00283 uint16_t length; 00284 /**< Length in bytes of table data section 00285 * (excluding this header) */ 00286 uint16_t crc16; 00287 /**< CRC-16-CCITT of the data */ 00288 }; 00289 uint64_t value64; 00290 } ADI_SENSE_1000_LUT_DESCRIPTOR; 00291 00292 /*! Look-Up Table geometry-specific data structures */ 00293 typedef union { 00294 ADI_SENSE_1000_LUT_COEFF_LIST coeffList; 00295 /**< Data format for tables with ADI_SENSE_1000_LUT_GEOMETRY_COEFFS geometry 00296 * except where equation is ADI_SENSE_1000_LUT_EQUATION_BIVARIATE_POLYN */ 00297 ADI_SENSE_1000_LUT_1D_ES lut1dEs; 00298 /**< Data format for tables with ADI_SENSE_1000_LUT_GEOMETRY_ES_1D geometry */ 00299 ADI_SENSE_1000_LUT_1D_NES lut1dNes; 00300 /**< Data format for tables with ADI_SENSE_1000_LUT_GEOMETRY_NES_1D geometry */ 00301 ADI_SENSE_1000_LUT_2D_ES lut2dEs; 00302 /**< Data format for tables with ADI_SENSE_1000_LUT_GEOMETRY_ES_2D geometry */ 00303 ADI_SENSE_1000_LUT_2D_NES lut2dNes; 00304 /**< Data format for tables with ADI_SENSE_1000_LUT_GEOMETRY_NES_2D geometry */ 00305 ADI_SENSE_1000_LUT_2D_POLYN_COEFF_LIST coeffList2d; 00306 /**< Data format for tables with ADI_SENSE_1000_LUT_GEOMETRY_COEFFS geometry 00307 * and ADI_SENSE_1000_LUT_EQUATION_BIVARIATE_POLYN equation */ 00308 } ADI_SENSE_1000_LUT_TABLE_DATA ; 00309 00310 /*! Look-Up Table structure */ 00311 typedef struct __attribute__ ((packed, aligned(4))) { 00312 ADI_SENSE_1000_LUT_DESCRIPTOR descriptor; 00313 /**< Look-Up Table descriptor */ 00314 ADI_SENSE_1000_LUT_TABLE_DATA data; 00315 /**< Look-Up Table data */ 00316 } ADI_SENSE_1000_LUT_TABLE; 00317 00318 /*! LUT data format versions */ 00319 typedef struct __attribute__ ((packed, aligned(4))) { 00320 uint8_t major; /*!< Major version number */ 00321 uint8_t minor; /*!< Minor version number */ 00322 } ADI_SENSE_1000_LUT_VERSION; 00323 00324 /*! LUT data header structure */ 00325 typedef struct __attribute__ ((packed, aligned(4))) { 00326 uint32_t signature; 00327 /**< Hard-coded signature value (@ref ADI_SENSE_LUT_SIGNATURE) */ 00328 ADI_SENSE_1000_LUT_VERSION version; 00329 /**< LUT data format version (@ref ADI_SENSE_LUT_VERSION) */ 00330 uint16_t numTables; 00331 /**< Total number of tables */ 00332 uint32_t totalLength; 00333 /**< Total length (in bytes) of all table descriptors and data 00334 * (excluding this header) 00335 * This, plus the header length, must not exceed ADI_SENSE_LUT_MAX_SIZE 00336 */ 00337 } ADI_SENSE_1000_LUT_HEADER; 00338 00339 /*! LUT data top-level structure */ 00340 typedef struct __attribute__ ((packed, aligned(4))) { 00341 ADI_SENSE_1000_LUT_HEADER header; 00342 /*!< LUT data top-level header structure */ 00343 ADI_SENSE_1000_LUT_TABLE tables[]; 00344 /*!< Variable-length array of one-or-more look-up table structures */ 00345 } ADI_SENSE_1000_LUT; 00346 00347 /*! Alternative top-level structure for raw LUT data representation 00348 * 00349 * @note This is intended to be used for encapsulating the storage of static 00350 * LUT data declarations in C files. The rawTableData can be cast 00351 * to the ADI_SENSE_LUT type for further parsing/processing. 00352 */ 00353 typedef struct __attribute__ ((packed, aligned(4))) { 00354 ADI_SENSE_1000_LUT_HEADER header; 00355 /*!< LUT data top-level header structure */ 00356 uint8_t rawTableData[]; 00357 /*!< Variable-length byte array of look-up tables in raw binary format */ 00358 } ADI_SENSE_1000_LUT_RAW; 00359 00360 #ifdef __cplusplus 00361 } 00362 #endif 00363 00364 /*! 00365 * @} 00366 */ 00367 00368 #endif /* __ADI_SENSE_1000_LUT_DATA_H__ */ 00369
Generated on Tue Jul 12 2022 21:13:16 by
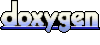