
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_1000_api.h
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_api.h 00004 * @brief: ADI Sense Host Library Application Programming Interface (API) 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /* 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 */ 00042 00043 #ifndef __ADI_SENSE_1000_API_H__ 00044 #define __ADI_SENSE_1000_API_H__ 00045 00046 #include "inc/adi_sense_types.h" 00047 #include "inc/adi_sense_config_types.h" 00048 #include "inc/adi_sense_platform.h" 00049 #include "adi_sense_1000_config.h" 00050 #include "adi_sense_1000_lut_data.h" 00051 00052 /*! @ingroup ADI_Sense_Api */ 00053 00054 /*! @defgroup ADI_Sense_1000_Api ADI Sense 1000 Host Library API 00055 * ADI Sense 1000 device-specific API function prototypes. 00056 * These are supplementary to the common ADI Sense Host Library API. 00057 * @{ 00058 */ 00059 00060 #ifdef __cplusplus 00061 extern "C" { 00062 #endif 00063 00064 /*! 00065 * @brief Read one or more device registers at the specified register address. 00066 * 00067 * @param[in] hDevice ADI Sense device context handle 00068 * @param[in] nAddress Register map address to read from 00069 * @param[out] pData Pointer to return the register map data 00070 * @param[in] nLength Number of bytes of data to read from the register map 00071 * 00072 * @return Status 00073 * - #ADI_SENSE_SUCCESS Call completed successfully. 00074 * 00075 * @details Provides direct byte-level read access to the device register map. 00076 * The size and format of the register(s) must be known. 00077 * 00078 * @note Reads from special "keyhole" or "FIFO" registers will be handled 00079 * according to documentation for those registers. 00080 */ 00081 ADI_SENSE_RESULT adi_sense_1000_ReadRegister( 00082 ADI_SENSE_DEVICE_HANDLE const hDevice, 00083 uint16_t const nAddress, 00084 void * const pData, 00085 unsigned const nLength); 00086 00087 /*! 00088 * @brief Write one or more device registers at the specified register address. 00089 * 00090 * @param[in] hDevice ADI Sense device context handle 00091 * @param[in] nAddress Register map address to read from 00092 * @param[out] pData Pointer to return the register map data 00093 * @param[in] nLength Number of bytes of data to read from the register map 00094 * 00095 * @return Status 00096 * - #ADI_SENSE_SUCCESS Call completed successfully. 00097 * 00098 * @details Provides direct byte-level write access to the device register map. 00099 * The size and format of the register(s) must be known. 00100 * 00101 * @note Writes to read-only registers will be ignored by the device. 00102 * @note Writes to special "keyhole" registers will be handled according to 00103 * documentation for those registers. 00104 */ 00105 ADI_SENSE_RESULT adi_sense_1000_WriteRegister( 00106 ADI_SENSE_DEVICE_HANDLE const hDevice, 00107 uint16_t const nAddress, 00108 void * const pData, 00109 unsigned const nLength); 00110 00111 /*! 00112 * @brief Update power configuration settings on the device. 00113 * 00114 * @param[in] hDevice ADI Sense device context handle 00115 * @param[in] pPowerConfig Power configuration details 00116 * 00117 * @return Status 00118 * - #ADI_SENSE_SUCCESS Call completed successfully. 00119 * 00120 * @details Translates configuration details provided into device-specific 00121 * register settings and updates device configuration registers. 00122 * 00123 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00124 */ 00125 ADI_SENSE_RESULT adi_sense_1000_SetPowerConfig( 00126 ADI_SENSE_DEVICE_HANDLE hDevice, 00127 ADI_SENSE_1000_POWER_CONFIG *pPowerConfig); 00128 00129 /*! 00130 * @brief Update measurement configuration settings on the device. 00131 * 00132 * @param[in] hDevice ADI Sense device context handle 00133 * @param[in] pMeasurementConfig Measurement configuration details 00134 * 00135 * @return Status 00136 * - #ADI_SENSE_SUCCESS Call completed successfully. 00137 * 00138 * @details Translates configuration details provided into device-specific 00139 * register settings and updates device configuration registers. 00140 * 00141 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00142 */ 00143 ADI_SENSE_RESULT adi_sense_1000_SetMeasurementConfig( 00144 ADI_SENSE_DEVICE_HANDLE hDevice, 00145 ADI_SENSE_1000_MEASUREMENT_CONFIG *pMeasurementConfig); 00146 00147 /*! 00148 * @brief Update diagnostics configuration settings on the device. 00149 * 00150 * @param[in] hDevice ADI Sense device context handle 00151 * @param[in] pDiagnosticsConfig Diagnostics configuration details 00152 * 00153 * @return Status 00154 * - #ADI_SENSE_SUCCESS Call completed successfully. 00155 * 00156 * @details Translates configuration details provided into device-specific 00157 * register settings and updates device configuration registers. 00158 * 00159 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00160 */ 00161 ADI_SENSE_RESULT adi_sense_1000_SetDiagnosticsConfig( 00162 ADI_SENSE_DEVICE_HANDLE hDevice, 00163 ADI_SENSE_1000_DIAGNOSTICS_CONFIG *pDiagnosticsConfig); 00164 00165 /*! 00166 * @brief Update channel configuration settings for a specific channel. 00167 * 00168 * @param[in] hDevice ADI Sense device context handle 00169 * @param[in] eChannelId Selects the channel to be updated 00170 * @param[in] pChannelConfig Channel configuration details 00171 * 00172 * @return Status 00173 * - #ADI_SENSE_SUCCESS Call completed successfully. 00174 * 00175 * @details Translates configuration details provided into device-specific 00176 * register settings and updates device configuration registers. 00177 * Allows individual channel configuration details to be dynamically 00178 * adjusted without rewriting the full device configuration. 00179 * 00180 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00181 */ 00182 ADI_SENSE_RESULT adi_sense_1000_SetChannelConfig( 00183 ADI_SENSE_DEVICE_HANDLE hDevice, 00184 ADI_SENSE_1000_CHANNEL_ID eChannelId, 00185 ADI_SENSE_1000_CHANNEL_CONFIG *pChannelConfig); 00186 00187 /*! 00188 * @brief Update number of measurements-per-cycle for a specific channel. 00189 * 00190 * @param[in] hDevice ADI Sense device context handle 00191 * @param[in] eChannelId Selects the channel to be updated 00192 * @param[in] nMeasurementsPerCycle Specifies the number of measurements to be 00193 * obtained from this channel in each 00194 * measurement cycle. Set as 0 to disable the 00195 * channel (omit from measurement cycle). 00196 * 00197 * @return Status 00198 * - #ADI_SENSE_SUCCESS Call completed successfully. 00199 * 00200 * @details Translates configuration details provided into device-specific 00201 * register settings and updates device configuration registers. 00202 * Allows individual channels to be dynamically enabled/disabled, and 00203 * measurements-per-cycle to be adjusted. 00204 * 00205 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00206 */ 00207 ADI_SENSE_RESULT adi_sense_1000_SetChannelCount( 00208 ADI_SENSE_DEVICE_HANDLE hDevice, 00209 ADI_SENSE_1000_CHANNEL_ID eChannelId, 00210 uint32_t nMeasurementsPerCycle); 00211 00212 /*! 00213 * @brief Update the measurement threshold limits for a specified channel. 00214 * 00215 * @param[in] hDevice ADI Sense device context handle 00216 * @param[in] eChannelId Selects the channel to be updated 00217 * @param[in] fHighThresholdLimit Optional maximum threshold value for each 00218 * processed sample, to be checked prior to 00219 * publishing. A channel ALERT condition is 00220 * raised if the processed value is higher than 00221 * this threshold. Set to NaN if not required. 00222 * @param[in] fLowThresholdLimit Optional minimum threshold value for each 00223 * processed sample, to be checked prior to 00224 * publishing. A channel ALERT condition is 00225 * raised if the processed value is lower than 00226 * this threshold. Set to NaN if not required. 00227 * 00228 * @return Status 00229 * - #ADI_SENSE_SUCCESS Call completed successfully. 00230 * 00231 * @details Translates configuration details provided into device-specific 00232 * register settings and updates device configuration registers. 00233 * Allows individual channel thresholds to be dynamically adjusted. 00234 * 00235 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00236 */ 00237 ADI_SENSE_RESULT adi_sense_1000_SetChannelThresholdLimits( 00238 ADI_SENSE_DEVICE_HANDLE hDevice, 00239 ADI_SENSE_1000_CHANNEL_ID eChannelId, 00240 float32_t fHighThresholdLimit, 00241 float32_t fLowThresholdLimit); 00242 00243 /*! 00244 * @brief Update the extra settling time for a specified channel. 00245 * 00246 * @param[in] hDevice ADI Sense device context handle 00247 * @param[in] eChannelId Selects the channel to be updated 00248 * @param[in] nSettlingTime A minimum settling time is applied internally for 00249 * each channel, based on the sensor type. However, 00250 * additional settling time (microseconds) can 00251 * optionally be specified here. Set to 0 if not 00252 * required. 00253 * 00254 * @return Status 00255 * - #ADI_SENSE_SUCCESS Call completed successfully. 00256 * 00257 * @details Translates configuration details provided into device-specific 00258 * register settings and updates device configuration registers. 00259 * Allows individual channel settling times to be dynamically adjusted. 00260 * 00261 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00262 */ 00263 ADI_SENSE_RESULT adi_sense_1000_SetChannelSettlingTime( 00264 ADI_SENSE_DEVICE_HANDLE hDevice, 00265 ADI_SENSE_1000_CHANNEL_ID eChannelId, 00266 uint32_t nSettlingTime); 00267 00268 /*! 00269 * @brief Assemble a list of separate Look-Up Tables into a single buffer 00270 * 00271 * @param[out] pLutBuffer Pointer to the Look-Up Table data buffer where 00272 * the assembled Look-Up Table data will be placed 00273 * @param[in] nLutBufferSize Allocated size, in bytes, of the output data buffer 00274 * @param[in] nNumTables Number of tables to add to the Look-Up Table buffer 00275 * @param[in] ppDesc Array of pointers to the table descriptors to be added 00276 * @param[in] ppData Array of pointers to the table data to be added 00277 * 00278 * @return Status 00279 * - #ADI_SENSE_SUCCESS Call completed successfully. 00280 * 00281 * @details This utiliity function fills the Look-up Table header fields; then 00282 * walks through the array of individual table descriptor and data 00283 * pointers provided, appending (copying) each one to the Look-Up Table 00284 * data buffer. The length and crc16 fields of each table descriptor 00285 * will be calculated and filled by this function, but other fields in 00286 * the descriptor structure must be filled by the caller beforehand. 00287 * 00288 * @note The assembled LUT data buffer filled by this function can then be 00289 * written to the device memory using @adi_sense_1000_SetLutData. 00290 */ 00291 ADI_SENSE_RESULT adi_sense_1000_AssembleLutData( 00292 ADI_SENSE_1000_LUT * pLutBuffer, 00293 unsigned nLutBufferSize, 00294 unsigned const nNumTables, 00295 ADI_SENSE_1000_LUT_DESCRIPTOR * const ppDesc[], 00296 ADI_SENSE_1000_LUT_TABLE_DATA * const ppData[]); 00297 00298 /*! 00299 * @brief Write Look-Up Table data to the device memory 00300 * 00301 * @param[in] hDevice ADI Sense device context handle 00302 * @param[out] pLutData Pointer to the Look-Up Table data structure 00303 * 00304 * @return Status 00305 * - #ADI_SENSE_SUCCESS Call completed successfully. 00306 * 00307 * @details Validates the Look-Up Table data format and loads it into 00308 * device memory via dedicated keyhole registers. 00309 * 00310 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00311 */ 00312 ADI_SENSE_RESULT adi_sense_1000_SetLutData( 00313 ADI_SENSE_DEVICE_HANDLE const hDevice, 00314 ADI_SENSE_1000_LUT * const pLutData); 00315 00316 /*! 00317 * @brief Write Look-Up Table raw data to the device memory 00318 * 00319 * @param[in] hDevice ADI Sense device context handle 00320 * @param[out] pLutData Pointer to the Look-Up Table raw data structure 00321 * 00322 * @return Status 00323 * - #ADI_SENSE_SUCCESS Call completed successfully. 00324 * 00325 * @details This can be used instead of @adi_sense_1000_SetLutData for 00326 * loading LUT data from the alternative raw data format. See 00327 * @adi_sense_1000_SetLutData for more information. 00328 * 00329 * @note Settings are not applied until adi_sense_ApplyConfigUpdates() is called 00330 */ 00331 ADI_SENSE_RESULT adi_sense_1000_SetLutDataRaw( 00332 ADI_SENSE_DEVICE_HANDLE const hDevice, 00333 ADI_SENSE_1000_LUT_RAW * const pLutData); 00334 00335 /*! 00336 * @brief Get the number of samples available when DATAREADY status is asserted. 00337 * 00338 * @param[in] hDevice ADI Sense device context handle 00339 * @param[in] bMeasurementMode Must be set to the same value used for @ref 00340 * adi_sense_StartMeasurement(). 00341 * @param[out] peOperatingMode Pointer to return the configured operating mode 00342 * @param[out] peDataReadMode Pointer to return the configured data publishing mode 00343 * @param[out] pnSamplesPerDataready Pointer to return the calculated number of samples 00344 * available when DATAREADY is asserted 00345 * @param[out] pnSamplesPerCycle Pointer to return the calculated number of samples 00346 * produced per measurement cycle 00347 * 00348 * @return Status 00349 * - #ADI_SENSE_SUCCESS Call completed successfully. 00350 * 00351 * @details Examines the current configuration settings in the device registers 00352 * to calculate the number of samples available whenever the DATAREADY 00353 * signal is asserted, along with other related information. This may 00354 * be used to allocate buffers to store samples and to determine how 00355 * many samples to retrieve whenever the DATAREADY status is asserted. 00356 */ 00357 ADI_SENSE_RESULT adi_sense_1000_GetDataReadyModeInfo( 00358 ADI_SENSE_DEVICE_HANDLE const hDevice, 00359 ADI_SENSE_MEASUREMENT_MODE const eMeasurementMode, 00360 ADI_SENSE_1000_OPERATING_MODE * const peOperatingMode, 00361 ADI_SENSE_1000_DATAREADY_MODE * const peDataReadyMode, 00362 uint32_t * const pnSamplesPerDataready, 00363 uint32_t * const pnSamplesPerCycle); 00364 00365 /*! 00366 * @brief Read the contents of the ADI Sense internal factory calibration table 00367 * 00368 * Calibration coefficients/gains/offsets are stored internally in a 2D table of 00369 * 32-bit floating point values. Refer to product documentation for details of 00370 * the rows and columns. 00371 * 00372 * @param[in] hDevice ADI Sense device context handle 00373 * @param[in] pfBuffer Pointer to destination buffer for the calibration data 00374 * @param[in] nMaxLen The buffer capacity in bytes (e.g. 672 for 56x3 table) 00375 * @param[out] pnDataLen The number of bytes written to the buffer 00376 * @param[out] pnRows Pointer to return the number of table rows (e.g. 56) 00377 * @param[out] pnColumns Pointer to return the number of table columns (e.g. 3) 00378 * 00379 * @return Status 00380 * - #ADI_SENSE_SUCCESS Call completed successfully. 00381 * - #ADI_SENSE_FAILURE 00382 * - #ADI_SENSE_INVALID_OPERATION Invalid register identifier. 00383 */ 00384 ADI_SENSE_RESULT adi_sense_1000_ReadCalTable( 00385 ADI_SENSE_DEVICE_HANDLE hDevice, 00386 float *pfBuffer, 00387 unsigned nMaxLen, 00388 unsigned *pnDataLen, 00389 unsigned *pnRows, 00390 unsigned *pnColumns); 00391 00392 #ifdef __cplusplus 00393 } 00394 #endif 00395 00396 /*! 00397 * @} 00398 */ 00399 00400 #endif /* __ADI_SENSE_1000_API_H__ */ 00401
Generated on Tue Jul 12 2022 21:13:16 by
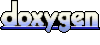