
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
ADISENSE1000_REGISTERS_typedefs.h
00001 /* ================================================================================ 00002 00003 Created by : sherry 00004 Created on : 2017 Nov 14, 10:55 GMT 00005 00006 Project : ADISENSE1000_REGISTERS 00007 File : ADISENSE1000_REGISTERS_typedefs.h 00008 Description : C Register Structures 00009 00010 !! ADI Confidential !! 00011 INTERNAL USE ONLY 00012 00013 Copyright (c) 2017 Analog Devices, Inc. All Rights Reserved. 00014 This software is proprietary and confidential to Analog Devices, Inc. and 00015 its licensors. 00016 00017 This file was auto-generated. Do not make local changes to this file. 00018 00019 Auto generation script information: 00020 Script: /usr/cadtools/bin/yoda.dir/generators/inc/genHeaders 00021 Last modified: 26-SEP-2017 00022 00023 ================================================================================ */ 00024 00025 #ifndef _ADISENSE1000_REGISTERS_TYPEDEFS_H 00026 #define _ADISENSE1000_REGISTERS_TYPEDEFS_H 00027 00028 /* pickup integer types */ 00029 #if defined(_LANGUAGE_C) || (defined(__GNUC__) && !defined(__ASSEMBLER__)) 00030 #include <stdint.h> 00031 #endif /* _LANGUAGE_C */ 00032 00033 #if defined ( __CC_ARM ) 00034 #pragma push 00035 #pragma anon_unions 00036 #endif 00037 00038 /** @defgroup Interface_Config_A Interface Configuration A (Interface_Config_A) Register 00039 * Interface Configuration A (Interface_Config_A) Register. 00040 * @{ 00041 */ 00042 00043 /* ========================================================================= 00044 *! \enum ADI_ADISENSE_SPI_Interface_Config_A_Addr_Ascension 00045 *! \brief Determines Sequential Addressing Behavior (Addr_Ascension) Enumerations 00046 * ========================================================================= */ 00047 typedef enum 00048 { 00049 ADISENSE_SPI_INTERFACE_CONFIG_A_DESCEND = 0, /**< Address accessed is decremented by one for each data byte when streaming */ 00050 ADISENSE_SPI_INTERFACE_CONFIG_A_ASCEND = 1 /**< Address accessed is incremented by one for each data byte when streaming */ 00051 } ADI_ADISENSE_SPI_Interface_Config_A_Addr_Ascension ; 00052 00053 00054 /* ========================================================================== 00055 *! \struct ADI_ADISENSE_SPI_Interface_Config_A_Struct 00056 *! \brief Interface Configuration A Register bit field structure 00057 * ========================================================================== */ 00058 typedef struct _ADI_ADISENSE_SPI_Interface_Config_A_t { 00059 union { 00060 struct { 00061 uint8_t SW_ResetX : 1; /**< Second of Two of SW_RESET Bits. */ 00062 uint8_t reserved1 : 3; 00063 uint8_t SDO_Enable : 1; /**< SDO Pin Enable */ 00064 uint8_t Addr_Ascension : 1; /**< Determines Sequential Addressing Behavior */ 00065 uint8_t reserved6 : 1; 00066 uint8_t SW_Reset : 1; /**< First of Two of SW_RESET Bits. */ 00067 }; 00068 uint8_t VALUE8; 00069 }; 00070 } ADI_ADISENSE_SPI_Interface_Config_A_t; 00071 00072 /*@}*/ 00073 00074 /** @defgroup Interface_Config_B Interface Configuration B (Interface_Config_B) Register 00075 * Interface Configuration B (Interface_Config_B) Register. 00076 * @{ 00077 */ 00078 00079 /* ========================================================================= 00080 *! \enum ADI_ADISENSE_SPI_Interface_Config_B_Single_Inst 00081 *! \brief Select Streaming or Single Instruction Mode (Single_Inst) Enumerations 00082 * ========================================================================= */ 00083 typedef enum 00084 { 00085 ADISENSE_SPI_INTERFACE_CONFIG_B_STREAMING_MODE = 0, /**< Streaming mode is enabled */ 00086 ADISENSE_SPI_INTERFACE_CONFIG_B_SINGLE_INSTRUCTION_MODE = 1 /**< Single Instruction mode is enabled */ 00087 } ADI_ADISENSE_SPI_Interface_Config_B_Single_Inst ; 00088 00089 00090 /* ========================================================================== 00091 *! \struct ADI_ADISENSE_SPI_Interface_Config_B_Struct 00092 *! \brief Interface Configuration B Register bit field structure 00093 * ========================================================================== */ 00094 typedef struct _ADI_ADISENSE_SPI_Interface_Config_B_t { 00095 union { 00096 struct { 00097 uint8_t reserved0 : 7; 00098 uint8_t Single_Inst : 1; /**< Select Streaming or Single Instruction Mode */ 00099 }; 00100 uint8_t VALUE8; 00101 }; 00102 } ADI_ADISENSE_SPI_Interface_Config_B_t; 00103 00104 /*@}*/ 00105 00106 /** @defgroup Device_Config Device Configuration (Device_Config) Register 00107 * Device Configuration (Device_Config) Register. 00108 * @{ 00109 */ 00110 00111 /* ========================================================================= 00112 *! \enum ADI_ADISENSE_SPI_Device_Config_Operating_Modes 00113 *! \brief Power Modes (Operating_Modes) Enumerations 00114 * ========================================================================= */ 00115 typedef enum 00116 { 00117 ADISENSE_SPI_DEVICE_CONFIG_NORMAL = 0, /**< Normal Operating Mode */ 00118 ADISENSE_SPI_DEVICE_CONFIG_SLEEP = 3 /**< Low Power Mode */ 00119 } ADI_ADISENSE_SPI_Device_Config_Operating_Modes ; 00120 00121 00122 /* ========================================================================== 00123 *! \struct ADI_ADISENSE_SPI_Device_Config_Struct 00124 *! \brief Device Configuration Register bit field structure 00125 * ========================================================================== */ 00126 typedef struct _ADI_ADISENSE_SPI_Device_Config_t { 00127 union { 00128 struct { 00129 uint8_t Operating_Modes : 2; /**< Power Modes */ 00130 uint8_t reserved2 : 6; 00131 }; 00132 uint8_t VALUE8; 00133 }; 00134 } ADI_ADISENSE_SPI_Device_Config_t; 00135 00136 /*@}*/ 00137 00138 /** @defgroup Chip_Type Chip Type (Chip_Type) Register 00139 * Chip Type (Chip_Type) Register. 00140 * @{ 00141 */ 00142 00143 /* ========================================================================== 00144 *! \struct ADI_ADISENSE_SPI_Chip_Type_Struct 00145 *! \brief Chip Type Register bit field structure 00146 * ========================================================================== */ 00147 typedef struct _ADI_ADISENSE_SPI_Chip_Type_t { 00148 union { 00149 struct { 00150 uint8_t Chip_Type : 4; /**< Precision ADC */ 00151 uint8_t reserved4 : 4; 00152 }; 00153 uint8_t VALUE8; 00154 }; 00155 } ADI_ADISENSE_SPI_Chip_Type_t; 00156 00157 /*@}*/ 00158 00159 /** @defgroup Product_ID_L Product ID Low (Product_ID_L) Register 00160 * Product ID Low (Product_ID_L) Register. 00161 * @{ 00162 */ 00163 00164 /* ========================================================================== 00165 *! \struct ADI_ADISENSE_SPI_Product_ID_L_Struct 00166 *! \brief Product ID Low Register bit field structure 00167 * ========================================================================== */ 00168 typedef struct _ADI_ADISENSE_SPI_Product_ID_L_t { 00169 union { 00170 struct { 00171 uint8_t Product_ID_Trim_Bits : 4; /**< These Bits Vary on Die Configured for Multiple Generics */ 00172 uint8_t Product_ID_Fixed_Bits : 4; /**< Product_ID_Fixed_Bits[3:0] These Bits are Fixed on Die Configured for Multiple Generics */ 00173 }; 00174 uint8_t VALUE8; 00175 }; 00176 } ADI_ADISENSE_SPI_Product_ID_L_t; 00177 00178 /*@}*/ 00179 00180 /** @defgroup Product_ID_H Product ID High (Product_ID_H) Register 00181 * Product ID High (Product_ID_H) Register. 00182 * @{ 00183 */ 00184 00185 /* ========================================================================== 00186 *! \struct ADI_ADISENSE_SPI_Product_ID_H_Struct 00187 *! \brief Product ID High Register bit field structure 00188 * ========================================================================== */ 00189 typedef struct _ADI_ADISENSE_SPI_Product_ID_H_t { 00190 union { 00191 struct { 00192 uint8_t Product_ID_Fixed_Bits : 8; /**< Product_ID_Fixed_Bits[11:4] These Bits are Fixed on Die Configured for Multiple Generics */ 00193 }; 00194 uint8_t VALUE8; 00195 }; 00196 } ADI_ADISENSE_SPI_Product_ID_H_t; 00197 00198 /*@}*/ 00199 00200 /** @defgroup Chip_Grade Chip Grade (Chip_Grade) Register 00201 * Chip Grade (Chip_Grade) Register. 00202 * @{ 00203 */ 00204 00205 /* ========================================================================== 00206 *! \struct ADI_ADISENSE_SPI_Chip_Grade_Struct 00207 *! \brief Chip Grade Register bit field structure 00208 * ========================================================================== */ 00209 typedef struct _ADI_ADISENSE_SPI_Chip_Grade_t { 00210 union { 00211 struct { 00212 uint8_t Device_Revision : 4; /**< This is the Device Hardware Revision */ 00213 uint8_t Grade : 4; /**< This is the Device Performance Grade */ 00214 }; 00215 uint8_t VALUE8; 00216 }; 00217 } ADI_ADISENSE_SPI_Chip_Grade_t; 00218 00219 /*@}*/ 00220 00221 /** @defgroup Scratch_Pad Scratch Pad (Scratch_Pad) Register 00222 * Scratch Pad (Scratch_Pad) Register. 00223 * @{ 00224 */ 00225 00226 /* ========================================================================== 00227 *! \struct ADI_ADISENSE_SPI_Scratch_Pad_Struct 00228 *! \brief Scratch Pad Register bit field structure 00229 * ========================================================================== */ 00230 typedef struct _ADI_ADISENSE_SPI_Scratch_Pad_t { 00231 union { 00232 struct { 00233 uint8_t Scratch_Value : 8; /**< Software Scratchpad */ 00234 }; 00235 uint8_t VALUE8; 00236 }; 00237 } ADI_ADISENSE_SPI_Scratch_Pad_t; 00238 00239 /*@}*/ 00240 00241 /** @defgroup SPI_Revision SPI Revision (SPI_Revision) Register 00242 * SPI Revision (SPI_Revision) Register. 00243 * @{ 00244 */ 00245 00246 /* ========================================================================= 00247 *! \enum ADI_ADISENSE_SPI_SPI_Revision_Version 00248 *! \brief SPI Version (Version) Enumerations 00249 * ========================================================================= */ 00250 typedef enum 00251 { 00252 ADISENSE_SPI_SPI_REVISION_REV1_0 = 2 /**< Revision 1.0 */ 00253 } ADI_ADISENSE_SPI_SPI_Revision_Version ; 00254 00255 00256 /* ========================================================================= 00257 *! \enum ADI_ADISENSE_SPI_SPI_Revision_SPI_Type 00258 *! \brief Always Reads as 0x2 (SPI_Type) Enumerations 00259 * ========================================================================= */ 00260 typedef enum 00261 { 00262 ADISENSE_SPI_SPI_REVISION_ADI_SPI = 0, /**< */ 00263 ADISENSE_SPI_SPI_REVISION_LPT_SPI = 2 /**< */ 00264 } ADI_ADISENSE_SPI_SPI_Revision_SPI_Type; 00265 00266 00267 /* ========================================================================== 00268 *! \struct ADI_ADISENSE_SPI_SPI_Revision_Struct 00269 *! \brief SPI Revision Register bit field structure 00270 * ========================================================================== */ 00271 typedef struct _ADI_ADISENSE_SPI_SPI_Revision_t { 00272 union { 00273 struct { 00274 uint8_t Version : 6; /**< SPI Version */ 00275 uint8_t SPI_Type : 2; /**< Always Reads as 0x2 */ 00276 }; 00277 uint8_t VALUE8; 00278 }; 00279 } ADI_ADISENSE_SPI_SPI_Revision_t; 00280 00281 /*@}*/ 00282 00283 /** @defgroup Vendor_L Vendor ID Low (Vendor_L) Register 00284 * Vendor ID Low (Vendor_L) Register. 00285 * @{ 00286 */ 00287 00288 /* ========================================================================== 00289 *! \struct ADI_ADISENSE_SPI_Vendor_L_Struct 00290 *! \brief Vendor ID Low Register bit field structure 00291 * ========================================================================== */ 00292 typedef struct _ADI_ADISENSE_SPI_Vendor_L_t { 00293 union { 00294 struct { 00295 uint8_t VID : 8; /**< VID[7:0] Analog Devices Vendor ID */ 00296 }; 00297 uint8_t VALUE8; 00298 }; 00299 } ADI_ADISENSE_SPI_Vendor_L_t; 00300 00301 /*@}*/ 00302 00303 /** @defgroup Vendor_H Vendor ID High (Vendor_H) Register 00304 * Vendor ID High (Vendor_H) Register. 00305 * @{ 00306 */ 00307 00308 /* ========================================================================== 00309 *! \struct ADI_ADISENSE_SPI_Vendor_H_Struct 00310 *! \brief Vendor ID High Register bit field structure 00311 * ========================================================================== */ 00312 typedef struct _ADI_ADISENSE_SPI_Vendor_H_t { 00313 union { 00314 struct { 00315 uint8_t VID : 8; /**< VID[15:8] Analog Devices Vendor ID */ 00316 }; 00317 uint8_t VALUE8; 00318 }; 00319 } ADI_ADISENSE_SPI_Vendor_H_t; 00320 00321 /*@}*/ 00322 00323 /** @defgroup Stream_Mode Stream Mode (Stream_Mode) Register 00324 * Stream Mode (Stream_Mode) Register. 00325 * @{ 00326 */ 00327 00328 /* ========================================================================== 00329 *! \struct ADI_ADISENSE_SPI_Stream_Mode_Struct 00330 *! \brief Stream Mode Register bit field structure 00331 * ========================================================================== */ 00332 typedef struct _ADI_ADISENSE_SPI_Stream_Mode_t { 00333 union { 00334 struct { 00335 uint8_t Loop_Count : 8; /**< Sets the Data Byte Count Before Looping to Start Address */ 00336 }; 00337 uint8_t VALUE8; 00338 }; 00339 } ADI_ADISENSE_SPI_Stream_Mode_t; 00340 00341 /*@}*/ 00342 00343 /** @defgroup Transfer_Config Transfer Config (Transfer_Config) Register 00344 * Transfer Config (Transfer_Config) Register. 00345 * @{ 00346 */ 00347 00348 /* ========================================================================= 00349 *! \enum ADI_ADISENSE_SPI_Transfer_Config_Stream_Mode 00350 *! \brief When Streaming, Controls Master-Slave Transfer (Stream_Mode) Enumerations 00351 * ========================================================================= */ 00352 typedef enum 00353 { 00354 ADISENSE_SPI_TRANSFER_CONFIG_UPDATE_ON_WRITE = 0, /**< Transfers after each byte/mulit-byte register */ 00355 ADISENSE_SPI_TRANSFER_CONFIG_UPDATE_ON_ADDRESS_LOOP = 1 /**< Transfers when address loops */ 00356 } ADI_ADISENSE_SPI_Transfer_Config_Stream_Mode ; 00357 00358 00359 /* ========================================================================== 00360 *! \struct ADI_ADISENSE_SPI_Transfer_Config_Struct 00361 *! \brief Transfer Config Register bit field structure 00362 * ========================================================================== */ 00363 typedef struct _ADI_ADISENSE_SPI_Transfer_Config_t { 00364 union { 00365 struct { 00366 uint8_t reserved0 : 1; 00367 uint8_t Stream_Mode : 1; /**< When Streaming, Controls Master-Slave Transfer */ 00368 uint8_t reserved2 : 6; 00369 }; 00370 uint8_t VALUE8; 00371 }; 00372 } ADI_ADISENSE_SPI_Transfer_Config_t; 00373 00374 /*@}*/ 00375 00376 /** @defgroup Interface_Config_C Interface Configuration C (Interface_Config_C) Register 00377 * Interface Configuration C (Interface_Config_C) Register. 00378 * @{ 00379 */ 00380 00381 /* ========================================================================= 00382 *! \enum ADI_ADISENSE_SPI_Interface_Config_C_Strict_Register_Access 00383 *! \brief Multi-byte Registers Must Be Read/Written in Full (Strict_Register_Access) Enumerations 00384 * ========================================================================= */ 00385 typedef enum 00386 { 00387 ADISENSE_SPI_INTERFACE_CONFIG_C_NORMAL_ACCESS = 0, /**< Normal mode, no access restrictions */ 00388 ADISENSE_SPI_INTERFACE_CONFIG_C_STRICT_ACCESS = 1 /**< Strict mode, multi-byte registers require all bytes read/written */ 00389 } ADI_ADISENSE_SPI_Interface_Config_C_Strict_Register_Access ; 00390 00391 00392 /* ========================================================================= 00393 *! \enum ADI_ADISENSE_SPI_Interface_Config_C_CRC_Enable 00394 *! \brief CRC Enable (CRC_Enable) Enumerations 00395 * ========================================================================= */ 00396 typedef enum 00397 { 00398 ADISENSE_SPI_INTERFACE_CONFIG_C_DISABLED = 0, /**< CRC Disabled */ 00399 ADISENSE_SPI_INTERFACE_CONFIG_C_ENABLED = 1 /**< CRC Enabled */ 00400 } ADI_ADISENSE_SPI_Interface_Config_C_CRC_Enable ; 00401 00402 00403 /* ========================================================================== 00404 *! \struct ADI_ADISENSE_SPI_Interface_Config_C_Struct 00405 *! \brief Interface Configuration C Register bit field structure 00406 * ========================================================================== */ 00407 typedef struct _ADI_ADISENSE_SPI_Interface_Config_C_t { 00408 union { 00409 struct { 00410 uint8_t CRC_EnableB : 2; /**< Inverted CRC Enable */ 00411 uint8_t reserved2 : 3; 00412 uint8_t Strict_Register_Access : 1; /**< Multi-byte Registers Must Be Read/Written in Full */ 00413 uint8_t CRC_Enable : 2; /**< CRC Enable */ 00414 }; 00415 uint8_t VALUE8; 00416 }; 00417 } ADI_ADISENSE_SPI_Interface_Config_C_t; 00418 00419 /*@}*/ 00420 00421 /** @defgroup Interface_Status_A Interface Status A (Interface_Status_A) Register 00422 * Interface Status A (Interface_Status_A) Register. 00423 * @{ 00424 */ 00425 00426 /* ========================================================================== 00427 *! \struct ADI_ADISENSE_SPI_Interface_Status_A_Struct 00428 *! \brief Interface Status A Register bit field structure 00429 * ========================================================================== */ 00430 typedef struct _ADI_ADISENSE_SPI_Interface_Status_A_t { 00431 union { 00432 struct { 00433 uint8_t Address_Invalid_Error : 1; /**< Attempt to Read/Write Non-existent Register Address */ 00434 uint8_t Register_Partial_Access_Error : 1; /**< Set When Fewer Than Expected Number of Bytes Read/Written */ 00435 uint8_t Wr_To_Rd_Only_Reg_Error : 1; /**< Write to Read-Only Register Attempted */ 00436 uint8_t CRC_Error : 1; /**< Invalid/No CRC Received */ 00437 uint8_t Clock_Count_Error : 1; /**< Incorrect Number of Clocks Detected in a Transaction */ 00438 uint8_t reserved5 : 2; 00439 uint8_t Not_Ready_Error : 1; /**< Device Not Ready for Transaction */ 00440 }; 00441 uint8_t VALUE8; 00442 }; 00443 } ADI_ADISENSE_SPI_Interface_Status_A_t; 00444 00445 /*@}*/ 00446 00447 /** @defgroup Command Special Command (Command) Register 00448 * Special Command (Command) Register. 00449 * @{ 00450 */ 00451 00452 /* ========================================================================= 00453 *! \enum ADI_ADISENSE_CORE_Command_Special_Command 00454 *! \brief Special Command (Special_Command) Enumerations 00455 * ========================================================================= */ 00456 typedef enum 00457 { 00458 ADISENSE_CORE_COMMAND_NOP = 0, /**< No Command */ 00459 ADISENSE_CORE_COMMAND_CONVERT = 1, /**< Start ADC Conversions */ 00460 ADISENSE_CORE_COMMAND_CONVERT_WITH_RAW = 2, /**< Start Conversions with Added RAW ADC Data */ 00461 ADISENSE_CORE_COMMAND_RUN_DIAGNOSTICS = 3, /**< Initiate a Diagnostics Cycle */ 00462 ADISENSE_CORE_COMMAND_SELF_CALIBRATION = 4, /**< Initiate a Self-Calibration Cycle */ 00463 ADISENSE_CORE_COMMAND_LOAD_CONFIG = 5, /**< Load Registers with Configuration from FLASH */ 00464 ADISENSE_CORE_COMMAND_SAVE_CONFIG = 6, /**< Store Current Register Configuration to FLASH */ 00465 ADISENSE_CORE_COMMAND_LATCH_CONFIG = 7, /**< Latch Configuration. */ 00466 ADISENSE_CORE_COMMAND_LOAD_LUT = 8, /**< Load LUT from FLASH */ 00467 ADISENSE_CORE_COMMAND_SAVE_LUT2 = 9, /**< Save LUT to FLASH */ 00468 ADISENSE_CORE_COMMAND_SYSTEM_CHECK = 10 /**< Full Suite of Measurement Diagnostics */ 00469 } ADI_ADISENSE_CORE_Command_Special_Command ; 00470 00471 00472 /* ========================================================================== 00473 *! \struct ADI_ADISENSE_CORE_Command_Struct 00474 *! \brief Special Command Register bit field structure 00475 * ========================================================================== */ 00476 typedef struct _ADI_ADISENSE_CORE_Command_t { 00477 union { 00478 struct { 00479 uint8_t Special_Command : 8; /**< Special Command */ 00480 }; 00481 uint8_t VALUE8; 00482 }; 00483 } ADI_ADISENSE_CORE_Command_t; 00484 00485 /*@}*/ 00486 00487 /** @defgroup Mode Operating Mode and DRDY Control (Mode) Register 00488 * Operating Mode and DRDY Control (Mode) Register. 00489 * @{ 00490 */ 00491 00492 /* ========================================================================= 00493 *! \enum ADI_ADISENSE_CORE_Mode_Conversion_Mode 00494 *! \brief Conversion Mode (Conversion_Mode) Enumerations 00495 * ========================================================================= */ 00496 typedef enum 00497 { 00498 ADISENSE_CORE_MODE_SINGLECYCLE = 0, /**< Single Cycle */ 00499 ADISENSE_CORE_MODE_MULTICYCLE = 1, /**< Multi Cycle */ 00500 ADISENSE_CORE_MODE_CONTINUOUS = 2, /**< Continuous Conversion */ 00501 ADISENSE_CORE_MODE_MODE3 = 3 /**< Undefined */ 00502 } ADI_ADISENSE_CORE_Mode_Conversion_Mode ; 00503 00504 00505 /* ========================================================================= 00506 *! \enum ADI_ADISENSE_CORE_Mode_Drdy_Mode 00507 *! \brief Indicates Behavior of DRDY with Respect to FIFO State (Drdy_Mode) Enumerations 00508 * ========================================================================= */ 00509 typedef enum 00510 { 00511 ADISENSE_CORE_MODE_DRDY_PER_CONVERSION = 0, /**< Data Ready Per Conversion */ 00512 ADISENSE_CORE_MODE_DRDY_PER_CYCLE = 1, /**< Data Ready Per Cycle */ 00513 ADISENSE_CORE_MODE_DRDY_PER_FIFO_FILL = 2, /**< Data Ready Per FIFO Fill */ 00514 ADISENSE_CORE_MODE_DRDY_MODE3 = 3 /**< Undefined */ 00515 } ADI_ADISENSE_CORE_Mode_Drdy_Mode ; 00516 00517 00518 /* ========================================================================== 00519 *! \struct ADI_ADISENSE_CORE_Mode_Struct 00520 *! \brief Operating Mode and DRDY Control Register bit field structure 00521 * ========================================================================== */ 00522 typedef struct _ADI_ADISENSE_CORE_Mode_t { 00523 union { 00524 struct { 00525 uint8_t Conversion_Mode : 2; /**< Conversion Mode */ 00526 uint8_t Drdy_Mode : 2; /**< Indicates Behavior of DRDY with Respect to FIFO State */ 00527 uint8_t reserved4 : 4; 00528 }; 00529 uint8_t VALUE8; 00530 }; 00531 } ADI_ADISENSE_CORE_Mode_t; 00532 00533 /*@}*/ 00534 00535 /** @defgroup Power_Config General Configuration (Power_Config) Register 00536 * General Configuration (Power_Config) Register. 00537 * @{ 00538 */ 00539 00540 /* ========================================================================= 00541 *! \enum ADI_ADISENSE_CORE_Power_Config_Power_Mode_ADC 00542 *! \brief ADC Power Mode (Power_Mode_ADC) Enumerations 00543 * ========================================================================= */ 00544 typedef enum 00545 { 00546 ADISENSE_CORE_POWER_CONFIG_ADC_LOW_POWER = 0, /**< ADC Low Power Mode */ 00547 ADISENSE_CORE_POWER_CONFIG_ADC_MID_POWER = 1, /**< ADC Mid Power Mode */ 00548 ADISENSE_CORE_POWER_CONFIG_ADC_FULL_POWER = 2, /**< ADC Full Power Mode */ 00549 ADISENSE_CORE_POWER_CONFIG_ADC_FULL_POWER2 = 3 /**< ADC Full Power Mode2 */ 00550 } ADI_ADISENSE_CORE_Power_Config_Power_Mode_ADC ; 00551 00552 00553 /* ========================================================================== 00554 *! \struct ADI_ADISENSE_CORE_Power_Config_Struct 00555 *! \brief General Configuration Register bit field structure 00556 * ========================================================================== */ 00557 typedef struct _ADI_ADISENSE_CORE_Power_Config_t { 00558 union { 00559 struct { 00560 uint8_t Power_Mode_ADC : 2; /**< ADC Power Mode */ 00561 uint8_t Power_Mode_MCU : 2; /**< MCU Power Mode */ 00562 uint8_t Stdby_En : 1; /**< Standby */ 00563 uint8_t reserved5 : 3; 00564 }; 00565 uint8_t VALUE8; 00566 }; 00567 } ADI_ADISENSE_CORE_Power_Config_t; 00568 00569 /*@}*/ 00570 00571 /** @defgroup Cycle_Control Measurement Cycle (Cycle_Control) Register 00572 * Measurement Cycle (Cycle_Control) Register. 00573 * @{ 00574 */ 00575 00576 /* ========================================================================= 00577 *! \enum ADI_ADISENSE_CORE_Cycle_Control_Cycle_Time_Units 00578 *! \brief Units for Cycle Time (Cycle_Time_Units) Enumerations 00579 * ========================================================================= */ 00580 typedef enum 00581 { 00582 ADISENSE_CORE_CYCLE_CONTROL_MICROSECONDS = 0, /**< Micro-Seconds */ 00583 ADISENSE_CORE_CYCLE_CONTROL_MILLISECONDS = 1, /**< Milli-Seconds */ 00584 ADISENSE_CORE_CYCLE_CONTROL_SECONDS = 2, /**< Seconds */ 00585 ADISENSE_CORE_CYCLE_CONTROL_UNDEFINED = 3 /**< Undefined */ 00586 } ADI_ADISENSE_CORE_Cycle_Control_Cycle_Time_Units ; 00587 00588 00589 /* ========================================================================== 00590 *! \struct ADI_ADISENSE_CORE_Cycle_Control_Struct 00591 *! \brief Measurement Cycle Register bit field structure 00592 * ========================================================================== */ 00593 typedef struct _ADI_ADISENSE_CORE_Cycle_Control_t { 00594 union { 00595 struct { 00596 uint16_t Cycle_Time : 12; /**< Duration of a Full Measurement Cycle */ 00597 uint16_t reserved12 : 2; 00598 uint16_t Cycle_Time_Units : 2; /**< Units for Cycle Time */ 00599 }; 00600 uint16_t VALUE16; 00601 }; 00602 } ADI_ADISENSE_CORE_Cycle_Control_t; 00603 00604 /*@}*/ 00605 00606 /** @defgroup Fifo_Num_Cycles Number of Measurement Cycles to Store in FIFO (Fifo_Num_Cycles) Register 00607 * Number of Measurement Cycles to Store in FIFO (Fifo_Num_Cycles) Register. 00608 * @{ 00609 */ 00610 00611 /* ========================================================================== 00612 *! \struct ADI_ADISENSE_CORE_Fifo_Num_Cycles_Struct 00613 *! \brief Number of Measurement Cycles to Store in FIFO Register bit field structure 00614 * ========================================================================== */ 00615 typedef struct _ADI_ADISENSE_CORE_Fifo_Num_Cycles_t { 00616 union { 00617 struct { 00618 uint8_t Fifo_Num_Cycles : 8; /**< How Many Cycles to Fill FIFO */ 00619 }; 00620 uint8_t VALUE8; 00621 }; 00622 } ADI_ADISENSE_CORE_Fifo_Num_Cycles_t; 00623 00624 /*@}*/ 00625 00626 /** @defgroup Multi_Cycle_Repeat_Interval Time Between Repeats of Multi-Cycle Conversions.... (Multi_Cycle_Repeat_Interval) Register 00627 * Time Between Repeats of Multi-Cycle Conversions.... (Multi_Cycle_Repeat_Interval) Register. 00628 * @{ 00629 */ 00630 00631 /* ========================================================================== 00632 *! \struct ADI_ADISENSE_CORE_Multi_Cycle_Repeat_Interval_Struct 00633 *! \brief Time Between Repeats of Multi-Cycle Conversions.... Register bit field structure 00634 * ========================================================================== */ 00635 typedef struct _ADI_ADISENSE_CORE_Multi_Cycle_Repeat_Interval_t { 00636 union { 00637 struct { 00638 uint32_t Multi_Cycle_Repeat_Interval : 24; /**< Defines Time Between Repetitions of Measurement Cycles. */ 00639 uint32_t reserved24 : 8; 00640 }; 00641 uint32_t VALUE32; 00642 }; 00643 } ADI_ADISENSE_CORE_Multi_Cycle_Repeat_Interval_t; 00644 00645 /*@}*/ 00646 00647 /** @defgroup Status General Status (Status) Register 00648 * General Status (Status) Register. 00649 * @{ 00650 */ 00651 00652 /* ========================================================================== 00653 *! \struct ADI_ADISENSE_CORE_Status_Struct 00654 *! \brief General Status Register bit field structure 00655 * ========================================================================== */ 00656 typedef struct _ADI_ADISENSE_CORE_Status_t { 00657 union { 00658 struct { 00659 uint8_t reserved0 : 1; 00660 uint8_t Alert_Active : 1; /**< Indicates One or More Sensors Alerts are Active */ 00661 uint8_t Error : 1; /**< Indicates an Error */ 00662 uint8_t Drdy : 1; /**< Indicates a New Sensor Result is Available to Be Read */ 00663 uint8_t Cmd_Running : 1; /**< Indicates a Special Command is Active */ 00664 uint8_t FIFO_Error : 1; /**< Indicates Error with FIFO */ 00665 uint8_t reserved6 : 2; 00666 }; 00667 uint8_t VALUE8; 00668 }; 00669 } ADI_ADISENSE_CORE_Status_t; 00670 00671 /*@}*/ 00672 00673 /** @defgroup Diagnostics_Status Diagnostics Status (Diagnostics_Status) Register 00674 * Diagnostics Status (Diagnostics_Status) Register. 00675 * @{ 00676 */ 00677 00678 /* ========================================================================== 00679 *! \struct ADI_ADISENSE_CORE_Diagnostics_Status_Struct 00680 *! \brief Diagnostics Status Register bit field structure 00681 * ========================================================================== */ 00682 typedef struct _ADI_ADISENSE_CORE_Diagnostics_Status_t { 00683 union { 00684 struct { 00685 uint16_t Diag_Checksum_Error : 1; /**< Indicates Error on Internal Checksum Calculations */ 00686 uint16_t Diag_Comms_Error : 1; /**< Indicates Error on Internal Device Communications */ 00687 uint16_t Diag_Supply_Monitor_Error : 1; /**< Indicates Low Voltage on Internal Supply Voltages */ 00688 uint16_t Diag_Supply_Cap_Error : 1; /**< Indicates Fault on Internal Supply Regulator Capacitor */ 00689 uint16_t reserved4 : 4; 00690 uint16_t Diag_Ainm_UV_Error : 1; /**< Indicates Under-Voltage Error on Negative Analog Input */ 00691 uint16_t Diag_Ainm_OV_Error : 1; /**< Indicates Over-Voltage Error on Negative Analog Input */ 00692 uint16_t Diag_Ainp_UV_Error : 1; /**< Indicates Under-Voltage Error on Positive Analog Input */ 00693 uint16_t Diag_Ainp_OV_Error : 1; /**< Indicates Over-Voltage Error on Positive Analog Input */ 00694 uint16_t Diag_Conversion_Error : 1; /**< Indicates Error During Internal ADC Conversions */ 00695 uint16_t Diag_Calibration_Error : 1; /**< Indicates Error During Internal Device Calibrations */ 00696 uint16_t Diagnostics_Status_Sundry : 2; /**< Sundry Diagnostics Status */ 00697 }; 00698 uint16_t VALUE16; 00699 }; 00700 } ADI_ADISENSE_CORE_Diagnostics_Status_t; 00701 00702 /*@}*/ 00703 00704 /** @defgroup Channel_Alert_Status Alert Status Summary (Channel_Alert_Status) Register 00705 * Alert Status Summary (Channel_Alert_Status) Register. 00706 * @{ 00707 */ 00708 00709 /* ========================================================================== 00710 *! \struct ADI_ADISENSE_CORE_Channel_Alert_Status_Struct 00711 *! \brief Alert Status Summary Register bit field structure 00712 * ========================================================================== */ 00713 typedef struct _ADI_ADISENSE_CORE_Channel_Alert_Status_t { 00714 union { 00715 struct { 00716 uint16_t Alert_Ch0 : 1; /**< Indicates Channel Alert is Active */ 00717 uint16_t Alert_Ch1 : 1; /**< Indicates Channel Alert is Active */ 00718 uint16_t Alert_Ch2 : 1; /**< Indicates Channel Alert is Active */ 00719 uint16_t Alert_Ch3 : 1; /**< Indicates Channel Alert is Active */ 00720 uint16_t Alert_Ch4 : 1; /**< Indicates Channel Alert is Active */ 00721 uint16_t Alert_Ch5 : 1; /**< Indicates Channel Alert is Active */ 00722 uint16_t Alert_Ch6 : 1; /**< Indicates Channel Alert is Active */ 00723 uint16_t Alert_Ch7 : 1; /**< Indicates Channel Alert is Active */ 00724 uint16_t Alert_Ch8 : 1; /**< Indicates Channel Alert is Active */ 00725 uint16_t Alert_Ch9 : 1; /**< Indicates Channel Alert is Active */ 00726 uint16_t Alert_Ch10 : 1; /**< Indicates Channel Alert is Active */ 00727 uint16_t Alert_Ch11 : 1; /**< Indicates Channel Alert is Active */ 00728 uint16_t Alert_Ch12 : 1; /**< Indicates Channel Alert is Active */ 00729 uint16_t reserved13 : 3; 00730 }; 00731 uint16_t VALUE16; 00732 }; 00733 } ADI_ADISENSE_CORE_Channel_Alert_Status_t; 00734 00735 /*@}*/ 00736 00737 /** @defgroup Alert_Status_2 Additional Alert Status Information (Alert_Status_2) Register 00738 * Additional Alert Status Information (Alert_Status_2) Register. 00739 * @{ 00740 */ 00741 00742 /* ========================================================================== 00743 *! \struct ADI_ADISENSE_CORE_Alert_Status_2_Struct 00744 *! \brief Additional Alert Status Information Register bit field structure 00745 * ========================================================================== */ 00746 typedef struct _ADI_ADISENSE_CORE_Alert_Status_2_t { 00747 union { 00748 struct { 00749 uint16_t reserved0 : 1; 00750 uint16_t LUT_Error : 1; /**< Indicates Error with One or More Look-Up-Tables */ 00751 uint16_t Configuration_Error : 1; /**< Indicates Error with Programmed Configuration */ 00752 uint16_t reserved3 : 13; 00753 }; 00754 uint16_t VALUE16; 00755 }; 00756 } ADI_ADISENSE_CORE_Alert_Status_2_t; 00757 00758 /*@}*/ 00759 00760 /** @defgroup Alert_Detail_Ch Detailed Error Information (Alert_Detail_Ch) Register 00761 * Detailed Error Information (Alert_Detail_Ch) Register. 00762 * @{ 00763 */ 00764 00765 /* ========================================================================== 00766 *! \struct ADI_ADISENSE_CORE_Alert_Detail_Ch_Struct 00767 *! \brief Detailed Error Information Register bit field structure 00768 * ========================================================================== */ 00769 typedef struct _ADI_ADISENSE_CORE_Alert_Detail_Ch_t { 00770 union { 00771 struct { 00772 uint16_t Time_Out : 1; /**< Indicates Time-Out Error from Digital Sensor */ 00773 uint16_t Under_Range : 1; /**< Indicates Channel Under-Range */ 00774 uint16_t Over_Range : 1; /**< Indicates Channel Over-Range */ 00775 uint16_t Low_Limit : 1; /**< Indicates Sensor Result is Less Than Low Limit */ 00776 uint16_t High_Limit : 1; /**< Indicates Sensor Result is Greater Than High Limit */ 00777 uint16_t Sensor_Open : 1; /**< Indicates Sensor Input is Open Circuit */ 00778 uint16_t Ref_Detect : 1; /**< Indicates Whether ADC Reference is Valid */ 00779 uint16_t reserved7 : 1; 00780 uint16_t Config_Err : 1; /**< Indicates Configuration Error on Channel */ 00781 uint16_t LUT_Error_Ch : 1; /**< Indicates Error with Channel Look-Up-Table */ 00782 uint16_t Under_Voltage : 1; /**< Indicates Channel Under-Voltage */ 00783 uint16_t Over_Voltage : 1; /**< Indicates Channel Over-Voltage */ 00784 uint16_t Correction_UnderRange : 1; /**< Indicates Result Less Than LUT/Equation Range */ 00785 uint16_t Correction_OverRange : 1; /**< Indicates Result Larger Than LUT/Equation Range */ 00786 uint16_t Sensor_Not_Ready : 1; /**< Indicates Digital Sensor Not Ready When Read */ 00787 uint16_t Comp_Not_Ready : 1; /**< Indicates Compensation Channel Not Ready When Required */ 00788 }; 00789 uint16_t VALUE16; 00790 }; 00791 } ADI_ADISENSE_CORE_Alert_Detail_Ch_t; 00792 00793 /*@}*/ 00794 00795 /** @defgroup Error_Code Code Indicating Source of Error (Error_Code) Register 00796 * Code Indicating Source of Error (Error_Code) Register. 00797 * @{ 00798 */ 00799 00800 /* ========================================================================== 00801 *! \struct ADI_ADISENSE_CORE_Error_Code_Struct 00802 *! \brief Code Indicating Source of Error Register bit field structure 00803 * ========================================================================== */ 00804 typedef struct _ADI_ADISENSE_CORE_Error_Code_t { 00805 union { 00806 struct { 00807 uint16_t Error_Code : 16; /**< Code Indicating Type of Error */ 00808 }; 00809 uint16_t VALUE16; 00810 }; 00811 } ADI_ADISENSE_CORE_Error_Code_t; 00812 00813 /*@}*/ 00814 00815 /** @defgroup Alert_Code Code Indicating Source of Alert (Alert_Code) Register 00816 * Code Indicating Source of Alert (Alert_Code) Register. 00817 * @{ 00818 */ 00819 00820 /* ========================================================================== 00821 *! \struct ADI_ADISENSE_CORE_Alert_Code_Struct 00822 *! \brief Code Indicating Source of Alert Register bit field structure 00823 * ========================================================================== */ 00824 typedef struct _ADI_ADISENSE_CORE_Alert_Code_t { 00825 union { 00826 struct { 00827 uint16_t Alert_Code : 16; /**< Code Indicating Type of Alert */ 00828 }; 00829 uint16_t VALUE16; 00830 }; 00831 } ADI_ADISENSE_CORE_Alert_Code_t; 00832 00833 /*@}*/ 00834 00835 /** @defgroup External_Reference1 External Reference Information (External_Reference1) Register 00836 * External Reference Information (External_Reference1) Register. 00837 * @{ 00838 */ 00839 00840 /* ========================================================================== 00841 *! \struct ADI_ADISENSE_CORE_External_Reference1_Struct 00842 *! \brief External Reference Information Register bit field structure 00843 * ========================================================================== */ 00844 typedef struct _ADI_ADISENSE_CORE_External_Reference1_t { 00845 union { 00846 struct { 00847 float Ext_Refin1_Value; /**< Refin1 Value */ 00848 }; 00849 float VALUE32; 00850 }; 00851 } ADI_ADISENSE_CORE_External_Reference1_t; 00852 00853 /*@}*/ 00854 00855 /** @defgroup External_Reference2 External Reference Information (External_Reference2) Register 00856 * External Reference Information (External_Reference2) Register. 00857 * @{ 00858 */ 00859 00860 /* ========================================================================== 00861 *! \struct ADI_ADISENSE_CORE_External_Reference2_Struct 00862 *! \brief External Reference Information Register bit field structure 00863 * ========================================================================== */ 00864 typedef struct _ADI_ADISENSE_CORE_External_Reference2_t { 00865 union { 00866 struct { 00867 float Ext_Refin2_Value; /**< Refin2 Value */ 00868 }; 00869 float VALUE32; 00870 }; 00871 } ADI_ADISENSE_CORE_External_Reference2_t; 00872 00873 /*@}*/ 00874 00875 /** @defgroup AVDD_Voltage AVDD Voltage (AVDD_Voltage) Register 00876 * AVDD Voltage (AVDD_Voltage) Register. 00877 * @{ 00878 */ 00879 00880 /* ========================================================================== 00881 *! \struct ADI_ADISENSE_CORE_AVDD_Voltage_Struct 00882 *! \brief AVDD Voltage Register bit field structure 00883 * ========================================================================== */ 00884 typedef struct _ADI_ADISENSE_CORE_AVDD_Voltage_t { 00885 union { 00886 struct { 00887 float Avdd_Voltage; /**< AVDD Voltage */ 00888 }; 00889 float VALUE32; 00890 }; 00891 } ADI_ADISENSE_CORE_AVDD_Voltage_t; 00892 00893 /*@}*/ 00894 00895 /** @defgroup Diagnostics_Control Diagnostic Control (Diagnostics_Control) Register 00896 * Diagnostic Control (Diagnostics_Control) Register. 00897 * @{ 00898 */ 00899 00900 /* ========================================================================= 00901 *! \enum ADI_ADISENSE_CORE_Diagnostics_Control_Diag_OSD_Freq 00902 *! \brief Diagnostics Open Sensor Detect Frequency (Diag_OSD_Freq) Enumerations 00903 * ========================================================================= */ 00904 typedef enum 00905 { 00906 ADISENSE_CORE_DIAGNOSTICS_CONTROL_OCD_OFF = 0, /**< No Open-Circuit Detection During Measurement */ 00907 ADISENSE_CORE_DIAGNOSTICS_CONTROL_OCD_PER_1_CYCLE = 1, /**< Open-Circuit Detection Performed Once Per Measurement Cycle */ 00908 ADISENSE_CORE_DIAGNOSTICS_CONTROL_OCD_PER_100_CYCLES = 2, /**< Open-Circuit Detection Performed Once Per Hundred Measurement Cycles */ 00909 ADISENSE_CORE_DIAGNOSTICS_CONTROL_OCD_PER_1000_CYCLES = 3 /**< Open-Circuit Detection Performed Once Per Thousand Measurement Cycles */ 00910 } ADI_ADISENSE_CORE_Diagnostics_Control_Diag_OSD_Freq ; 00911 00912 00913 /* ========================================================================== 00914 *! \struct ADI_ADISENSE_CORE_Diagnostics_Control_Struct 00915 *! \brief Diagnostic Control Register bit field structure 00916 * ========================================================================== */ 00917 typedef struct _ADI_ADISENSE_CORE_Diagnostics_Control_t { 00918 union { 00919 struct { 00920 uint16_t Diag_Global_En : 1; /**< Diagnostics Global Enable */ 00921 uint16_t Diag_Meas_En : 1; /**< Diagnostics Measure Enable */ 00922 uint16_t Diag_OSD_Freq : 2; /**< Diagnostics Open Sensor Detect Frequency */ 00923 uint16_t reserved4 : 4; 00924 uint16_t Diagnostics_Extra : 8; /**< Additional Diagnostics Control */ 00925 }; 00926 uint16_t VALUE16; 00927 }; 00928 } ADI_ADISENSE_CORE_Diagnostics_Control_t; 00929 00930 /*@}*/ 00931 00932 /** @defgroup Data_FIFO FIFO of Sensor Results (Data_FIFO) Register 00933 * FIFO of Sensor Results (Data_FIFO) Register. 00934 * @{ 00935 */ 00936 00937 /* ========================================================================== 00938 *! \struct ADI_ADISENSE_CORE_Data_FIFO_Struct 00939 *! \brief FIFO of Sensor Results Register bit field structure 00940 * ========================================================================== */ 00941 typedef struct _ADI_ADISENSE_CORE_Data_FIFO_t { 00942 union { 00943 struct { 00944 float32_t Sensor_Result; /**< Linearized and Compensated Sensor Result */ 00945 uint32_t Channel_ID : 4; /**< Indicates Which Channel This FIFO Data Corresponds to */ 00946 uint32_t Ch_Error : 1; /**< Indicates Error on Channel */ 00947 uint32_t Ch_Alert : 1; /**< Indicates Alert on Channel */ 00948 uint32_t Ch_Raw : 1; /**< Indicates If RAW Data is Valid */ 00949 uint32_t Ch_Valid : 1; /**< Indicates Whether Valid Data Read from FIFO */ 00950 uint32_t Raw_Sample : 24; /**< ADC Result */ 00951 }; 00952 uint64_t VALUE64; 00953 }; 00954 } ADI_ADISENSE_CORE_Data_FIFO_t; 00955 00956 /*@}*/ 00957 00958 /** @defgroup LUT_Select Read/Write Strobe (LUT_Select) Register 00959 * Read/Write Strobe (LUT_Select) Register. 00960 * @{ 00961 */ 00962 00963 /* ========================================================================= 00964 *! \enum ADI_ADISENSE_CORE_LUT_Select_LUT_RW 00965 *! \brief Read or Write LUT Data (LUT_RW) Enumerations 00966 * ========================================================================= */ 00967 typedef enum 00968 { 00969 ADISENSE_CORE_LUT_SELECT_LUT_READ = 0, /**< Read Addressed LUT Data */ 00970 ADISENSE_CORE_LUT_SELECT_LUT_WRITE = 1 /**< Write Addressed LUT Data */ 00971 } ADI_ADISENSE_CORE_LUT_Select_LUT_RW ; 00972 00973 00974 /* ========================================================================== 00975 *! \struct ADI_ADISENSE_CORE_LUT_Select_Struct 00976 *! \brief Read/Write Strobe Register bit field structure 00977 * ========================================================================== */ 00978 typedef struct _ADI_ADISENSE_CORE_LUT_Select_t { 00979 union { 00980 struct { 00981 uint8_t reserved0 : 7; 00982 uint8_t LUT_RW : 1; /**< Read or Write LUT Data */ 00983 }; 00984 uint8_t VALUE8; 00985 }; 00986 } ADI_ADISENSE_CORE_LUT_Select_t; 00987 00988 /*@}*/ 00989 00990 /** @defgroup LUT_Offset Offset into Selected LUT (LUT_Offset) Register 00991 * Offset into Selected LUT (LUT_Offset) Register. 00992 * @{ 00993 */ 00994 00995 /* ========================================================================== 00996 *! \struct ADI_ADISENSE_CORE_LUT_Offset_Struct 00997 *! \brief Offset into Selected LUT Register bit field structure 00998 * ========================================================================== */ 00999 typedef struct _ADI_ADISENSE_CORE_LUT_Offset_t { 01000 union { 01001 struct { 01002 uint16_t LUT_Offset : 14; /**< Offset into Look-Up-Table */ 01003 uint16_t reserved14 : 2; 01004 }; 01005 uint16_t VALUE16; 01006 }; 01007 } ADI_ADISENSE_CORE_LUT_Offset_t; 01008 01009 /*@}*/ 01010 01011 /** @defgroup LUT_Data Data to Read/Write from Addressed LUT Entry (LUT_Data) Register 01012 * Data to Read/Write from Addressed LUT Entry (LUT_Data) Register. 01013 * @{ 01014 */ 01015 01016 /* ========================================================================== 01017 *! \struct ADI_ADISENSE_CORE_LUT_Data_Struct 01018 *! \brief Data to Read/Write from Addressed LUT Entry Register bit field structure 01019 * ========================================================================== */ 01020 typedef struct _ADI_ADISENSE_CORE_LUT_Data_t { 01021 union { 01022 struct { 01023 uint8_t LUT_Data : 8; /**< Data Byte to Write to / Read from Look-Up-Table */ 01024 }; 01025 uint8_t VALUE8; 01026 }; 01027 } ADI_ADISENSE_CORE_LUT_Data_t; 01028 01029 /*@}*/ 01030 01031 /** @defgroup CAL_Offset Offset into Selected Calibration Values (CAL_Offset) Register 01032 * Offset into Selected Calibration Values (CAL_Offset) Register. 01033 * @{ 01034 */ 01035 01036 /* ========================================================================== 01037 *! \struct ADI_ADISENSE_CORE_CAL_Offset_Struct 01038 *! \brief Offset into Selected Calibration Values Register bit field structure 01039 * ========================================================================== */ 01040 typedef struct _ADI_ADISENSE_CORE_CAL_Offset_t { 01041 union { 01042 struct { 01043 uint16_t CAL_Offset : 14; /**< Offset into Calibration Data */ 01044 uint16_t reserved14 : 2; 01045 }; 01046 uint16_t VALUE16; 01047 }; 01048 } ADI_ADISENSE_CORE_CAL_Offset_t; 01049 01050 /*@}*/ 01051 01052 /** @defgroup CAL_Data Data to Read/Write from Addressed Calibration Values (CAL_Data) Register 01053 * Data to Read/Write from Addressed Calibration Values (CAL_Data) Register. 01054 * @{ 01055 */ 01056 01057 /* ========================================================================== 01058 *! \struct ADI_ADISENSE_CORE_CAL_Data_Struct 01059 *! \brief Data to Read/Write from Addressed Calibration Values Register bit field structure 01060 * ========================================================================== */ 01061 typedef struct _ADI_ADISENSE_CORE_CAL_Data_t { 01062 union { 01063 struct { 01064 uint8_t CAL_Data : 8; /**< Data to Write to / Read from Calibration Data */ 01065 }; 01066 uint8_t VALUE8; 01067 }; 01068 } ADI_ADISENSE_CORE_CAL_Data_t; 01069 01070 /*@}*/ 01071 01072 /** @defgroup Revision Hardware, Firmware Revision (Revision) Register 01073 * Hardware, Firmware Revision (Revision) Register. 01074 * @{ 01075 */ 01076 01077 /* ========================================================================== 01078 *! \struct ADI_ADISENSE_CORE_Revision_Struct 01079 *! \brief Hardware, Firmware Revision Register bit field structure 01080 * ========================================================================== */ 01081 typedef struct _ADI_ADISENSE_CORE_Revision_t { 01082 union { 01083 struct { 01084 uint32_t Firmware_Revision : 8; /**< ID Info */ 01085 uint32_t Hardware_Revision : 8; /**< ID Info */ 01086 uint32_t Comms_Protocol : 8; /**< ID Info */ 01087 uint32_t reserved24 : 8; 01088 }; 01089 uint32_t VALUE32; 01090 }; 01091 } ADI_ADISENSE_CORE_Revision_t; 01092 01093 /*@}*/ 01094 01095 /** @defgroup Channel_Count Number of Channel Occurrences per Measurement Cycle (Channel_Count) Register 01096 * Number of Channel Occurrences per Measurement Cycle (Channel_Count) Register. 01097 * @{ 01098 */ 01099 01100 /* ========================================================================== 01101 *! \struct ADI_ADISENSE_CORE_Channel_Count_Struct 01102 *! \brief Number of Channel Occurrences per Measurement Cycle Register bit field structure 01103 * ========================================================================== */ 01104 typedef struct _ADI_ADISENSE_CORE_Channel_Count_t { 01105 union { 01106 struct { 01107 uint8_t Channel_Count : 7; /**< How Many Times Channel Should Appear in One Cycle */ 01108 uint8_t Channel_Enable : 1; /**< Enable Channel in Measurement Cycle */ 01109 }; 01110 uint8_t VALUE8; 01111 }; 01112 } ADI_ADISENSE_CORE_Channel_Count_t; 01113 01114 /*@}*/ 01115 01116 /** @defgroup Sensor_Type Sensor Select (Sensor_Type) Register 01117 * Sensor Select (Sensor_Type) Register. 01118 * @{ 01119 */ 01120 01121 /* ========================================================================= 01122 *! \enum ADI_ADISENSE_CORE_Sensor_Type_Sensor_Type 01123 *! \brief Sensor Type (Sensor_Type) Enumerations 01124 * ========================================================================= */ 01125 typedef enum 01126 { 01127 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_T_DEF_L1 = 0, /**< Thermocouple T-Type Sensor Defined Level 1 */ 01128 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_J_DEF_L1 = 1, /**< Thermocouple J-Type Sensor Defined Level 1 */ 01129 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_K_DEF_L1 = 2, /**< Thermocouple K-Type Sensor Defined Level 1 */ 01130 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_1_DEF_L2 = 12, /**< Thermocouple Sensor 1 Defined Level 2 */ 01131 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_2_DEF_L2 = 13, /**< Thermocouple Sensor 2 Defined Level 2 */ 01132 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_3_DEF_L2 = 14, /**< Thermocouple Sensor 3 Defined Level 2 */ 01133 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_4_DEF_L2 = 15, /**< Thermocouple Sensor 4 Defined Level 2 */ 01134 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_T_ADV_L1 = 16, /**< Thermocouple T-Type Sensor Advanced Level 1 */ 01135 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_J_ADV_L1 = 17, /**< Thermocouple J-Type Sensor Advanced Level 1 */ 01136 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_K_ADV_L1 = 18, /**< Thermocouple K-Type Sensor Advanced Level 1 */ 01137 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_1_ADV_L2 = 28, /**< Thermocouple Sensor 1 Advanced Level 2 */ 01138 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_2_ADV_L2 = 29, /**< Thermocouple Sensor 2 Advanced Level 2 */ 01139 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_3_ADV_L2 = 30, /**< Thermocouple Sensor 3 Advanced Level 2 */ 01140 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMOCOUPLE_4_ADV_L2 = 31, /**< Thermocouple Sensor 4 Advanced Level 2 */ 01141 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_PT100_DEF_L1 = 32, /**< RTD 2 Wire PT100 Sensor Defined Level 1 */ 01142 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_PT1000_DEF_L1 = 33, /**< RTD 2 Wire PT1000 Sensor Defined Level 1 */ 01143 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_1_DEF_L2 = 44, /**< RTD 2 Wire Sensor 1 Defined Level 2 */ 01144 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_2_DEF_L2 = 45, /**< RTD 2 Wire Sensor 2 Defined Level 2 */ 01145 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_3_DEF_L2 = 46, /**< RTD 2 Wire Sensor 3 Defined Level 2 */ 01146 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_4_DEF_L2 = 47, /**< RTD 2 Wire Sensor 4 Defined Level 2 */ 01147 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_PT100_ADV_L1 = 48, /**< RTD 2 Wire PT100 Sensor Advanced Level 1 */ 01148 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_PT1000_ADV_L1 = 49, /**< RTD 2 Wire PT1000 Sensor Advanced Level 1 */ 01149 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_1_ADV_L2 = 60, /**< RTD 2 Wire Sensor 1 Advanced Level 2 */ 01150 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_2_ADV_L2 = 61, /**< RTD 2 Wire Sensor 2 Advanced Level 2 */ 01151 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_3_ADV_L2 = 62, /**< RTD 2 Wire Sensor 3 Advanced Level 2 */ 01152 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_2W_4_ADV_L2 = 63, /**< RTD 2 Wire Sensor 4 Advanced Level 2 */ 01153 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_PT100_DEF_L1 = 64, /**< RTD 3 Wire PT100 Sensor Defined Level 1 */ 01154 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_PT1000_DEF_L1 = 65, /**< RTD 3 Wire PT1000 Sensor Defined Level 1 */ 01155 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_1_DEF_L2 = 76, /**< RTD 3 Wire Sensor 1 Defined Level 2 */ 01156 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_2_DEF_L2 = 77, /**< RTD 3 Wire Sensor 2 Defined Level 2 */ 01157 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_3_DEF_L2 = 78, /**< RTD 3 Wire Sensor 3 Defined Level 2 */ 01158 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_4_DEF_L2 = 79, /**< RTD 3 Wire Sensor 4 Defined Level 2 */ 01159 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_PT100_ADV_L1 = 80, /**< RTD 3 Wire PT100 Sensor Advanced Level 1 */ 01160 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_PT1000_ADV_L1 = 81, /**< RTD 3 Wire PT1000 Sensor Advanced Level 1 */ 01161 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_1_ADV_L2 = 92, /**< RTD 3 Wire Sensor 1 Advanced Level 2 */ 01162 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_2_ADV_L2 = 93, /**< RTD 3 Wire Sensor 2 Advanced Level 2 */ 01163 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_3_ADV_L2 = 94, /**< RTD 3 Wire Sensor 3 Advanced Level 2 */ 01164 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_3W_4_ADV_L2 = 95, /**< RTD 3 Wire Sensor 4 Advanced Level 2 */ 01165 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_PT100_DEF_L1 = 96, /**< RTD 4 Wire PT100 Sensor Defined Level 1 */ 01166 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_PT1000_DEF_L1 = 97, /**< RTD 4 Wire PT1000 Sensor Defined Level 1 */ 01167 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_1_DEF_L2 = 108, /**< RTD 4 Wire Sensor 1 Defined Level 2 */ 01168 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_2_DEF_L2 = 109, /**< RTD 4 Wire Sensor 2 Defined Level 2 */ 01169 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_3_DEF_L2 = 110, /**< RTD 4 Wire Sensor 3 Defined Level 2 */ 01170 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_4_DEF_L2 = 111, /**< RTD 4 Wire Sensor 4 Defined Level 2 */ 01171 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_PT100_ADV_L1 = 112, /**< RTD 4 Wire PT100 Sensor Advanced Level 1 */ 01172 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_PT1000_ADV_L1 = 113, /**< RTD 4 Wire PT1000 Sensor Advanced Level 1 */ 01173 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_1_ADV_L2 = 124, /**< RTD 4 Wire Sensor 1 Advanced Level 2 */ 01174 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_2_ADV_L2 = 125, /**< RTD 4 Wire Sensor 2 Advanced Level 2 */ 01175 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_3_ADV_L2 = 126, /**< RTD 4 Wire Sensor 3 Advanced Level 2 */ 01176 ADISENSE_CORE_SENSOR_TYPE_SENSOR_RTD_4W_4_ADV_L2 = 127, /**< RTD 4 Wire Sensor 4 Advanced Level 2 */ 01177 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_A_10K_DEF_L1 = 128, /**< Thermistor Type A 10kOhm Sensor Defined Level 1 */ 01178 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_B_10K_DEF_L1 = 129, /**< Thermistor Type B 10kOhm Sensor Defined Level 1 */ 01179 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_1_DEF_L2 = 140, /**< Thermistor Sensor 1 Defined Level 2 */ 01180 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_2_DEF_L2 = 141, /**< Thermistor Sensor 2 Defined Level 2 */ 01181 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_3_DEF_L2 = 142, /**< Thermistor Sensor 3 Defined Level 2 */ 01182 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_4_DEF_L2 = 143, /**< Thermistor Sensor 4 Defined Level 2 */ 01183 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_A_10K_ADV_L1 = 144, /**< Thermistor Type A 10kOhm Sensor Advanced Level 1 */ 01184 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_B_10K_ADV_L1 = 145, /**< Thermistor Type B 10kOhm Sensor Advanced Level 1 */ 01185 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_1_ADV_L2 = 156, /**< Thermistor Sensor 1 Advanced Level 2 */ 01186 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_2_ADV_L2 = 157, /**< Thermistor Sensor 2 Advanced Level 2 */ 01187 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_3_ADV_L2 = 158, /**< Thermistor Sensor 3 Advanced Level 2 */ 01188 ADISENSE_CORE_SENSOR_TYPE_SENSOR_THERMISTOR_4_ADV_L2 = 159, /**< Thermistor Sensor 4 Advanced Level 2 */ 01189 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_1_DEF_L2 = 160, /**< Bridge 4 Wire Sensor 1 Defined Level 2 */ 01190 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_2_DEF_L2 = 161, /**< Bridge 4 Wire Sensor 2 Defined Level 2 */ 01191 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_3_DEF_L2 = 162, /**< Bridge 4 Wire Sensor 3 Defined Level 2 */ 01192 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_4_DEF_L2 = 163, /**< Bridge 4 Wire Sensor 4 Defined Level 2 */ 01193 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_1_ADV_L2 = 176, /**< Bridge 4 Wire Sensor 1 Advanced Level 2 */ 01194 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_2_ADV_L2 = 177, /**< Bridge 4 Wire Sensor 2 Advanced Level 2 */ 01195 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_3_ADV_L2 = 178, /**< Bridge 4 Wire Sensor 2 Advanced Level 2 */ 01196 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_4W_4_ADV_L2 = 179, /**< Bridge 4 Wire Sensor 2 Advanced Level 2 */ 01197 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_1_DEF_L2 = 192, /**< Bridge 6 Wire Sensor 1 Defined Level 2 */ 01198 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_2_DEF_L2 = 193, /**< Bridge 6 Wire Sensor 2 Defined Level 2 */ 01199 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_3_DEF_L2 = 194, /**< Bridge 6 Wire Sensor 3 Defined Level 2 */ 01200 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_4_DEF_L2 = 195, /**< Bridge 6 Wire Sensor 4 Defined Level 2 */ 01201 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_1_ADV_L2 = 208, /**< Bridge 6 Wire Sensor 1 Advanced Level 2 */ 01202 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_2_ADV_L2 = 209, /**< Bridge 6 Wire Sensor 2 Advanced Level 2 */ 01203 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_3_ADV_L2 = 210, /**< Bridge 6 Wire Sensor 3 Advanced Level 2 */ 01204 ADISENSE_CORE_SENSOR_TYPE_SENSOR_BRIDGE_6W_4_ADV_L2 = 211, /**< Bridge 6 Wire Sensor 4 Advanced Level 2 */ 01205 ADISENSE_CORE_SENSOR_TYPE_SENSOR_VOLTAGE = 256, /**< Voltage Input */ 01206 ADISENSE_CORE_SENSOR_TYPE_SENSOR_VOLTAGE_PRESSURE_HONEYWELL_TRUSTABILITY = 272, /**< Voltage Output Pressure Sensor 1 */ 01207 ADISENSE_CORE_SENSOR_TYPE_SENSOR_VOLTAGE_PRESSURE_AMPHENOL_NPA300X = 273, /**< Voltage Output Pressure Sensor 2 */ 01208 ADISENSE_CORE_SENSOR_TYPE_SENSOR_VOLTAGE_PRESSURE_3_DEF = 274, /**< Voltage Output Pressure Sensor 3 */ 01209 ADISENSE_CORE_SENSOR_TYPE_SENSOR_CURRENT = 384, /**< Current Input */ 01210 ADISENSE_CORE_SENSOR_TYPE_SENSOR_CURRENT_PRESSURE_HONEYWELL_PX2 = 385, /**< Current Output Pressure Sensor 1 */ 01211 ADISENSE_CORE_SENSOR_TYPE_SENSOR_CURRENT_PRESSURE_2 = 386, /**< Current Output Pressure Sensor 2 */ 01212 ADISENSE_CORE_SENSOR_TYPE_CUSTOM1 = 512, /**< Custom1 */ 01213 ADISENSE_CORE_SENSOR_TYPE_SENSOR_I2C_PRESSURE_1 = 2048, /**< I2C Pressure Sensor 1 */ 01214 ADISENSE_CORE_SENSOR_TYPE_SENSOR_I2C_PRESSURE_2 = 2049, /**< I2C Pressure Sensor 2 */ 01215 ADISENSE_CORE_SENSOR_TYPE_SENSOR_I2C_HUMIDITY_HONEYWELL_HUMIDICON = 2112, /**< I2C Humidity Sensor 1 */ 01216 ADISENSE_CORE_SENSOR_TYPE_SENSOR_I2C_HUMIDITY_SENSIRION_SHT3X = 2113, /**< I2C Humidity Sensor 2 */ 01217 ADISENSE_CORE_SENSOR_TYPE_SENSOR_SPI_PRESSURE_HONEYWELL_TRUSTABILITY = 3072, /**< SPI Pressure Sensor 1 */ 01218 ADISENSE_CORE_SENSOR_TYPE_SENSOR_SPI_PRESSURE_2 = 3073, /**< SPI Pressure Sensor 2 */ 01219 ADISENSE_CORE_SENSOR_TYPE_SENSOR_SPI_HUMIDITY_1 = 3136, /**< SPI Humidity Sensor Type 1 */ 01220 ADISENSE_CORE_SENSOR_TYPE_SENSOR_SPI_HUMIDITY_2 = 3137, /**< SPI Humidity Sensor Type 2 */ 01221 ADISENSE_CORE_SENSOR_TYPE_SENSOR_SPI_ACCELEROMETER_1 = 3200, /**< SPI Accelerometer Sensor Type 1 3-Axis */ 01222 ADISENSE_CORE_SENSOR_TYPE_SENSOR_SPI_ACCELEROMETER_2 = 3201 /**< SPI Accelerometer Sensor Type 2 3-Axis */ 01223 } ADI_ADISENSE_CORE_Sensor_Type_Sensor_Type ; 01224 01225 01226 /* ========================================================================== 01227 *! \struct ADI_ADISENSE_CORE_Sensor_Type_Struct 01228 *! \brief Sensor Select Register bit field structure 01229 * ========================================================================== */ 01230 typedef struct _ADI_ADISENSE_CORE_Sensor_Type_t { 01231 union { 01232 struct { 01233 uint16_t Sensor_Type : 12; /**< Sensor Type */ 01234 uint16_t reserved12 : 4; 01235 }; 01236 uint16_t VALUE16; 01237 }; 01238 } ADI_ADISENSE_CORE_Sensor_Type_t; 01239 01240 /*@}*/ 01241 01242 /** @defgroup Sensor_Details Sensor Details (Sensor_Details) Register 01243 * Sensor Details (Sensor_Details) Register. 01244 * @{ 01245 */ 01246 01247 /* ========================================================================= 01248 *! \enum ADI_ADISENSE_CORE_Sensor_Details_Measurement_Units 01249 *! \brief Units of Sensor Measurement (Measurement_Units) Enumerations 01250 * ========================================================================= */ 01251 typedef enum 01252 { 01253 ADISENSE_CORE_SENSOR_DETAILS_UNITS_DEGC = 0, /**< Degrees C */ 01254 ADISENSE_CORE_SENSOR_DETAILS_UNITS_DEGF = 1 /**< Degrees F */ 01255 } ADI_ADISENSE_CORE_Sensor_Details_Measurement_Units ; 01256 01257 01258 /* ========================================================================= 01259 *! \enum ADI_ADISENSE_CORE_Sensor_Details_Reference_Select 01260 *! \brief Reference Selection (Reference_Select) Enumerations 01261 * ========================================================================= */ 01262 typedef enum 01263 { 01264 ADISENSE_CORE_SENSOR_DETAILS_REF_INT = 0, /**< Internal Reference */ 01265 ADISENSE_CORE_SENSOR_DETAILS_REF_AVDD = 1, /**< AVDD */ 01266 ADISENSE_CORE_SENSOR_DETAILS_REF_VEXT1 = 2, /**< External Voltage on Refin1 */ 01267 ADISENSE_CORE_SENSOR_DETAILS_REF_VEXT2 = 3, /**< External Voltage on Refin2 */ 01268 ADISENSE_CORE_SENSOR_DETAILS_REF_RINT1 = 4, /**< Internal Resistor1 */ 01269 ADISENSE_CORE_SENSOR_DETAILS_REF_RINT2 = 5, /**< Internal Resistor2 */ 01270 ADISENSE_CORE_SENSOR_DETAILS_REF_REXT1 = 6, /**< External Resistor on Refin1 */ 01271 ADISENSE_CORE_SENSOR_DETAILS_REF_REXT2 = 7, /**< External Resistor on Refin2 */ 01272 ADISENSE_CORE_SENSOR_DETAILS_REF_EXC = 8 /**< Bridge Excitation Voltage */ 01273 } ADI_ADISENSE_CORE_Sensor_Details_Reference_Select ; 01274 01275 01276 /* ========================================================================= 01277 *! \enum ADI_ADISENSE_CORE_Sensor_Details_PGA_Gain 01278 *! \brief PGA Gain (PGA_Gain) Enumerations 01279 * ========================================================================= */ 01280 typedef enum 01281 { 01282 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_1 = 0, /**< Gain of 1 */ 01283 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_2 = 1, /**< Gain of 2 */ 01284 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_4 = 2, /**< Gain of 4 */ 01285 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_8 = 3, /**< Gain of 8 */ 01286 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_16 = 4, /**< Gain of 16 */ 01287 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_32 = 5, /**< Gain of 32 */ 01288 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_64 = 6, /**< Gain of 64 */ 01289 ADISENSE_CORE_SENSOR_DETAILS_PGA_GAIN_128 = 7 /**< Gain of 128 */ 01290 } ADI_ADISENSE_CORE_Sensor_Details_PGA_Gain ; 01291 01292 01293 /* ========================================================================== 01294 *! \struct ADI_ADISENSE_CORE_Sensor_Details_Struct 01295 *! \brief Sensor Details Register bit field structure 01296 * ========================================================================== */ 01297 typedef struct _ADI_ADISENSE_CORE_Sensor_Details_t { 01298 union { 01299 struct { 01300 uint32_t Measurement_Units : 4; /**< Units of Sensor Measurement */ 01301 uint32_t Compensation_Channel : 4; /**< Indicates Which Channel is Used to Compensate Sensor Result */ 01302 uint32_t Compensation_Channel2 : 4; /**< Indicates Channel for Second Term of Compensation */ 01303 uint32_t Compensation_Channel3 : 4; /**< Indicates Channel for Third Term of Compensation */ 01304 uint32_t reserved16 : 1; 01305 uint32_t Do_Not_Publish : 1; /**< Do Not Publish Channel Result */ 01306 uint32_t Reference_Buffer_Disable : 1; /**< Enable or Disable ADC Reference Buffer */ 01307 uint32_t Vbias : 1; /**< Controls ADC Vbias Output */ 01308 uint32_t Reference_Select : 4; /**< Reference Selection */ 01309 uint32_t PGA_Gain : 3; /**< PGA Gain */ 01310 uint32_t reserved27 : 1; 01311 uint32_t Averaging : 3; /**< Number of ADC Results to Average */ 01312 uint32_t reserved31 : 1; 01313 }; 01314 uint32_t VALUE32; 01315 }; 01316 } ADI_ADISENSE_CORE_Sensor_Details_t; 01317 01318 /*@}*/ 01319 01320 /** @defgroup Channel_Excitation Excitation Current (Channel_Excitation) Register 01321 * Excitation Current (Channel_Excitation) Register. 01322 * @{ 01323 */ 01324 01325 /* ========================================================================= 01326 *! \enum ADI_ADISENSE_CORE_Channel_Excitation_IOUT_Excitation_Current 01327 *! \brief Current Source Value (IOUT_Excitation_Current) Enumerations 01328 * ========================================================================= */ 01329 typedef enum 01330 { 01331 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_OFF = 0, /**< Disabled */ 01332 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_50UA = 1, /**< 50 \mu;A */ 01333 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_100UA = 2, /**< 100 \mu;A */ 01334 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_250UA = 3, /**< 250 \mu;A */ 01335 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_500UA = 4, /**< 500 \mu;A */ 01336 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_750UA = 5, /**< 750 \mu;A */ 01337 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_1000UA = 6, /**< 1000 \mu;A */ 01338 ADISENSE_CORE_CHANNEL_EXCITATION_IEXC_1000UA_2 = 7 /**< 1000 \mu;A */ 01339 } ADI_ADISENSE_CORE_Channel_Excitation_IOUT_Excitation_Current ; 01340 01341 01342 /* ========================================================================== 01343 *! \struct ADI_ADISENSE_CORE_Channel_Excitation_Struct 01344 *! \brief Excitation Current Register bit field structure 01345 * ========================================================================== */ 01346 typedef struct _ADI_ADISENSE_CORE_Channel_Excitation_t { 01347 union { 01348 struct { 01349 uint8_t IOUT_Excitation_Current : 3; /**< Current Source Value */ 01350 uint8_t IOUT0_Disable : 1; /**< Disable First Current Source */ 01351 uint8_t IOUT1_Disable : 1; /**< Disable Second Current Source */ 01352 uint8_t reserved5 : 1; 01353 uint8_t IOUT_Static_Swap_3Wire : 1; /**< Indicates 3-Wire Excitation Currents Should Be Swapped */ 01354 uint8_t IOUT_Dont_Swap_3Wire : 1; /**< Indicates 3-Wire Excitation Currents Should Not Be Swapped */ 01355 }; 01356 uint8_t VALUE8; 01357 }; 01358 } ADI_ADISENSE_CORE_Channel_Excitation_t; 01359 01360 /*@}*/ 01361 01362 /** @defgroup Settling_Time Settling Time (Settling_Time) Register 01363 * Settling Time (Settling_Time) Register. 01364 * @{ 01365 */ 01366 01367 /* ========================================================================== 01368 *! \struct ADI_ADISENSE_CORE_Settling_Time_Struct 01369 *! \brief Settling Time Register bit field structure 01370 * ========================================================================== */ 01371 typedef struct _ADI_ADISENSE_CORE_Settling_Time_t { 01372 union { 01373 struct { 01374 uint16_t Settling_Time : 16; /**< Settling Time to Allow When Switching to Channel */ 01375 }; 01376 uint16_t VALUE16; 01377 }; 01378 } ADI_ADISENSE_CORE_Settling_Time_t; 01379 01380 /*@}*/ 01381 01382 /** @defgroup Filter_Select ADC Digital Filter Selection (Filter_Select) Register 01383 * ADC Digital Filter Selection (Filter_Select) Register. 01384 * @{ 01385 */ 01386 01387 /* ========================================================================= 01388 *! \enum ADI_ADISENSE_CORE_Filter_Select_ADC_Filter_Type 01389 *! \brief ADC Digital Filter Type (ADC_Filter_Type) Enumerations 01390 * ========================================================================= */ 01391 typedef enum 01392 { 01393 ADISENSE_CORE_FILTER_SELECT_FILTER_FIR_25SPS = 0, /**< FIR Filter 25 SPS */ 01394 ADISENSE_CORE_FILTER_SELECT_FILTER_FIR_20SPS = 1, /**< FIR Filter 20 SPS */ 01395 ADISENSE_CORE_FILTER_SELECT_FILTER_SINC4 = 2, /**< Sinc4 Filter */ 01396 ADISENSE_CORE_FILTER_SELECT_FILTER_TBD = 3 /**< TBD Filter */ 01397 } ADI_ADISENSE_CORE_Filter_Select_ADC_Filter_Type ; 01398 01399 01400 /* ========================================================================== 01401 *! \struct ADI_ADISENSE_CORE_Filter_Select_Struct 01402 *! \brief ADC Digital Filter Selection Register bit field structure 01403 * ========================================================================== */ 01404 typedef struct _ADI_ADISENSE_CORE_Filter_Select_t { 01405 union { 01406 struct { 01407 uint32_t ADC_FS : 11; /**< ADC Digital Filter Select */ 01408 uint32_t ADC_Filter_Type : 5; /**< ADC Digital Filter Type */ 01409 uint32_t reserved16 : 16; 01410 }; 01411 uint32_t VALUE32; 01412 }; 01413 } ADI_ADISENSE_CORE_Filter_Select_t; 01414 01415 /*@}*/ 01416 01417 /** @defgroup High_Threshold_Limit High Threshold (High_Threshold_Limit) Register 01418 * High Threshold (High_Threshold_Limit) Register. 01419 * @{ 01420 */ 01421 01422 /* ========================================================================== 01423 *! \struct ADI_ADISENSE_CORE_High_Threshold_Limit_Struct 01424 *! \brief High Threshold Register bit field structure 01425 * ========================================================================== */ 01426 typedef struct _ADI_ADISENSE_CORE_High_Threshold_Limit_t { 01427 union { 01428 struct { 01429 float High_Threshold; /**< Upper Limit for Sensor Alert Comparison */ 01430 }; 01431 float VALUE32; 01432 }; 01433 } ADI_ADISENSE_CORE_High_Threshold_Limit_t; 01434 01435 /*@}*/ 01436 01437 /** @defgroup Low_Threshold_Limit Low Threshold (Low_Threshold_Limit) Register 01438 * Low Threshold (Low_Threshold_Limit) Register. 01439 * @{ 01440 */ 01441 01442 /* ========================================================================== 01443 *! \struct ADI_ADISENSE_CORE_Low_Threshold_Limit_Struct 01444 *! \brief Low Threshold Register bit field structure 01445 * ========================================================================== */ 01446 typedef struct _ADI_ADISENSE_CORE_Low_Threshold_Limit_t { 01447 union { 01448 struct { 01449 float Low_Threshold; /**< Lower Limit for Sensor Alert Comparison */ 01450 }; 01451 float VALUE32; 01452 }; 01453 } ADI_ADISENSE_CORE_Low_Threshold_Limit_t; 01454 01455 /*@}*/ 01456 01457 /** @defgroup Sensor_Offset Sensor Offset Adjustment (Sensor_Offset) Register 01458 * Sensor Offset Adjustment (Sensor_Offset) Register. 01459 * @{ 01460 */ 01461 01462 /* ========================================================================== 01463 *! \struct ADI_ADISENSE_CORE_Sensor_Offset_Struct 01464 *! \brief Sensor Offset Adjustment Register bit field structure 01465 * ========================================================================== */ 01466 typedef struct _ADI_ADISENSE_CORE_Sensor_Offset_t { 01467 union { 01468 struct { 01469 float Sensor_Offset; /**< Sensor Offset Adjustment */ 01470 }; 01471 float VALUE32; 01472 }; 01473 } ADI_ADISENSE_CORE_Sensor_Offset_t; 01474 01475 /*@}*/ 01476 01477 /** @defgroup Sensor_Gain Sensor Gain Adjustment (Sensor_Gain) Register 01478 * Sensor Gain Adjustment (Sensor_Gain) Register. 01479 * @{ 01480 */ 01481 01482 /* ========================================================================== 01483 *! \struct ADI_ADISENSE_CORE_Sensor_Gain_Struct 01484 *! \brief Sensor Gain Adjustment Register bit field structure 01485 * ========================================================================== */ 01486 typedef struct _ADI_ADISENSE_CORE_Sensor_Gain_t { 01487 union { 01488 struct { 01489 float Sensor_Gain; /**< Sensor Gain Adjustment */ 01490 }; 01491 float VALUE32; 01492 }; 01493 } ADI_ADISENSE_CORE_Sensor_Gain_t; 01494 01495 /*@}*/ 01496 01497 /** @defgroup Alert_Code_Ch Per-Channel Detailed Alert-Code Information (Alert_Code_Ch) Register 01498 * Per-Channel Detailed Alert-Code Information (Alert_Code_Ch) Register. 01499 * @{ 01500 */ 01501 01502 /* ========================================================================== 01503 *! \struct ADI_ADISENSE_CORE_Alert_Code_Ch_Struct 01504 *! \brief Per-Channel Detailed Alert-Code Information Register bit field structure 01505 * ========================================================================== */ 01506 typedef struct _ADI_ADISENSE_CORE_Alert_Code_Ch_t { 01507 union { 01508 struct { 01509 uint16_t Alert_Code_Ch : 16; /**< Per-Channel Code Indicating Type of Alert */ 01510 }; 01511 uint16_t VALUE16; 01512 }; 01513 } ADI_ADISENSE_CORE_Alert_Code_Ch_t; 01514 01515 /*@}*/ 01516 01517 /** @defgroup Digital_Sensor_Config Digital Sensor Data Coding (Digital_Sensor_Config) Register 01518 * Digital Sensor Data Coding (Digital_Sensor_Config) Register. 01519 * @{ 01520 */ 01521 01522 /* ========================================================================= 01523 *! \enum ADI_ADISENSE_CORE_Digital_Sensor_Config_Digital_Sensor_Coding 01524 *! \brief Data Encoding of Sensor Result (Digital_Sensor_Coding) Enumerations 01525 * ========================================================================= */ 01526 typedef enum 01527 { 01528 ADISENSE_CORE_DIGITAL_SENSOR_CONFIG_CODING_NONE = 0, /**< None/Invalid */ 01529 ADISENSE_CORE_DIGITAL_SENSOR_CONFIG_CODING_UNIPOLAR = 1, /**< Unipolar */ 01530 ADISENSE_CORE_DIGITAL_SENSOR_CONFIG_CODING_TWOS_COMPL = 2, /**< Twos Complement */ 01531 ADISENSE_CORE_DIGITAL_SENSOR_CONFIG_CODING_OFFSET_BINARY = 3, /**< Offset Binary */ 01532 } ADI_ADISENSE_CORE_Digital_Sensor_Config_Digital_Sensor_Coding ; 01533 01534 /* ========================================================================== 01535 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Config_Struct 01536 *! \brief Digital Sensor Data Coding Register bit field structure 01537 * ========================================================================== */ 01538 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Config_t { 01539 union { 01540 struct { 01541 uint16_t Digital_Sensor_Coding : 2; /**< Data Encoding of Sensor Result */ 01542 uint16_t Digital_Sensor_LittleEndian : 1; /**< Data Endianness of Sensor Result */ 01543 uint16_t Digital_Sensor_LeftAligned : 1; /**< Data Alignment within the data frame */ 01544 uint16_t Digital_Sensor_Bit_Offset : 4; /**< Data Bit Offset, relative to alignment */ 01545 uint16_t Digital_Sensor_Read_Bytes : 3; /**< Number of bytes to read from the sensor, minus 1 */ 01546 uint16_t Digital_Sensor_Data_Bits : 5; /**< Number of Relevant Data Bits, minus 1 */ 01547 }; 01548 uint16_t VALUE16; 01549 }; 01550 } ADI_ADISENSE_CORE_Digital_Sensor_Config_t; 01551 01552 /*@}*/ 01553 01554 /** @defgroup Digital_Sensor_Address Sensor Address (Digital_Sensor_Address) Register 01555 * Sensor Address (Digital_Sensor_Address) Register. 01556 * @{ 01557 */ 01558 01559 /* ========================================================================== 01560 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Address_Struct 01561 *! \brief Sensor Address Register bit field structure 01562 * ========================================================================== */ 01563 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Address_t { 01564 union { 01565 struct { 01566 uint8_t Digital_Sensor_Address : 8; /**< I2C Address or Write Address Command for SPI Sensor */ 01567 }; 01568 uint8_t VALUE8; 01569 }; 01570 } ADI_ADISENSE_CORE_Digital_Sensor_Address_t; 01571 01572 /*@}*/ 01573 01574 /** @defgroup Digital_Sensor_Num_Cmds Number of Configuration, Read Commands for Digital Sensors (Digital_Sensor_Num_Cmds) Register 01575 * Number of Configuration, Read Commands for Digital Sensors (Digital_Sensor_Num_Cmds) Register. 01576 * @{ 01577 */ 01578 01579 /* ========================================================================== 01580 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Num_Cmds_Struct 01581 *! \brief Number of Configuration, Read Commands for Digital Sensors Register bit field structure 01582 * ========================================================================== */ 01583 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Num_Cmds_t { 01584 union { 01585 struct { 01586 uint8_t Digital_Sensor_Num_Cfg_Cmds : 3; /**< Number of Configuration Commands for Digital Sensor */ 01587 uint8_t reserved3 : 1; 01588 uint8_t Digital_Sensor_Num_Read_Cmds : 3; /**< Number of Read Commands for Digital Sensor */ 01589 uint8_t reserved7 : 1; 01590 }; 01591 uint8_t VALUE8; 01592 }; 01593 } ADI_ADISENSE_CORE_Digital_Sensor_Num_Cmds_t; 01594 01595 /*@}*/ 01596 01597 /** @defgroup Digital_Sensor_Command1 Sensor Configuration Command1 (Digital_Sensor_Command1) Register 01598 * Sensor Configuration Command1 (Digital_Sensor_Command1) Register. 01599 * @{ 01600 */ 01601 01602 /* ========================================================================== 01603 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Command1_Struct 01604 *! \brief Sensor Configuration Command1 Register bit field structure 01605 * ========================================================================== */ 01606 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Command1_t { 01607 union { 01608 struct { 01609 uint8_t Digital_Sensor_Command1 : 8; /**< Configuration Command to Send to Digital I2C/SPI Sensor */ 01610 }; 01611 uint8_t VALUE8; 01612 }; 01613 } ADI_ADISENSE_CORE_Digital_Sensor_Command1_t; 01614 01615 /*@}*/ 01616 01617 /** @defgroup Digital_Sensor_Command2 Sensor Configuration Command2 (Digital_Sensor_Command2) Register 01618 * Sensor Configuration Command2 (Digital_Sensor_Command2) Register. 01619 * @{ 01620 */ 01621 01622 /* ========================================================================== 01623 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Command2_Struct 01624 *! \brief Sensor Configuration Command2 Register bit field structure 01625 * ========================================================================== */ 01626 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Command2_t { 01627 union { 01628 struct { 01629 uint8_t Digital_Sensor_Command2 : 8; /**< Configuration Command to Send to Digital I2C/SPI Sensor */ 01630 }; 01631 uint8_t VALUE8; 01632 }; 01633 } ADI_ADISENSE_CORE_Digital_Sensor_Command2_t; 01634 01635 /*@}*/ 01636 01637 /** @defgroup Digital_Sensor_Command3 Sensor Configuration Command3 (Digital_Sensor_Command3) Register 01638 * Sensor Configuration Command3 (Digital_Sensor_Command3) Register. 01639 * @{ 01640 */ 01641 01642 /* ========================================================================== 01643 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Command3_Struct 01644 *! \brief Sensor Configuration Command3 Register bit field structure 01645 * ========================================================================== */ 01646 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Command3_t { 01647 union { 01648 struct { 01649 uint8_t Digital_Sensor_Command3 : 8; /**< Configuration Command to Send to Digital I2C/SPI Sensor */ 01650 }; 01651 uint8_t VALUE8; 01652 }; 01653 } ADI_ADISENSE_CORE_Digital_Sensor_Command3_t; 01654 01655 /*@}*/ 01656 01657 /** @defgroup Digital_Sensor_Command4 Sensor Configuration Command4 (Digital_Sensor_Command4) Register 01658 * Sensor Configuration Command4 (Digital_Sensor_Command4) Register. 01659 * @{ 01660 */ 01661 01662 /* ========================================================================== 01663 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Command4_Struct 01664 *! \brief Sensor Configuration Command4 Register bit field structure 01665 * ========================================================================== */ 01666 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Command4_t { 01667 union { 01668 struct { 01669 uint8_t Digital_Sensor_Command4 : 8; /**< Configuration Command to Send to Digital I2C/SPI Sensor */ 01670 }; 01671 uint8_t VALUE8; 01672 }; 01673 } ADI_ADISENSE_CORE_Digital_Sensor_Command4_t; 01674 01675 /*@}*/ 01676 01677 /** @defgroup Digital_Sensor_Command5 Sensor Configuration Command5 (Digital_Sensor_Command5) Register 01678 * Sensor Configuration Command5 (Digital_Sensor_Command5) Register. 01679 * @{ 01680 */ 01681 01682 /* ========================================================================== 01683 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Command5_Struct 01684 *! \brief Sensor Configuration Command5 Register bit field structure 01685 * ========================================================================== */ 01686 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Command5_t { 01687 union { 01688 struct { 01689 uint8_t Digital_Sensor_Command5 : 8; /**< Configuration Command to Send to Digital I2C/SPI Sensor */ 01690 }; 01691 uint8_t VALUE8; 01692 }; 01693 } ADI_ADISENSE_CORE_Digital_Sensor_Command5_t; 01694 01695 /*@}*/ 01696 01697 /** @defgroup Digital_Sensor_Command6 Sensor Configuration Command6 (Digital_Sensor_Command6) Register 01698 * Sensor Configuration Command6 (Digital_Sensor_Command6) Register. 01699 * @{ 01700 */ 01701 01702 /* ========================================================================== 01703 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Command6_Struct 01704 *! \brief Sensor Configuration Command6 Register bit field structure 01705 * ========================================================================== */ 01706 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Command6_t { 01707 union { 01708 struct { 01709 uint8_t Digital_Sensor_Command6 : 8; /**< Configuration Command to Send to Digital I2C/SPI Sensor */ 01710 }; 01711 uint8_t VALUE8; 01712 }; 01713 } ADI_ADISENSE_CORE_Digital_Sensor_Command6_t; 01714 01715 /*@}*/ 01716 01717 /** @defgroup Digital_Sensor_Command7 Sensor Configuration Command7 (Digital_Sensor_Command7) Register 01718 * Sensor Configuration Command7 (Digital_Sensor_Command7) Register. 01719 * @{ 01720 */ 01721 01722 /* ========================================================================== 01723 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Command7_Struct 01724 *! \brief Sensor Configuration Command7 Register bit field structure 01725 * ========================================================================== */ 01726 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Command7_t { 01727 union { 01728 struct { 01729 uint8_t Digital_Sensor_Command7 : 8; /**< Configuration Command to Send to Digital I2C/SPI Sensor */ 01730 }; 01731 uint8_t VALUE8; 01732 }; 01733 } ADI_ADISENSE_CORE_Digital_Sensor_Command7_t; 01734 01735 /*@}*/ 01736 01737 /** @defgroup Digital_Sensor_Read_Cmd1 Sensor Read Command1 (Digital_Sensor_Read_Cmd1) Register 01738 * Sensor Read Command1 (Digital_Sensor_Read_Cmd1) Register. 01739 * @{ 01740 */ 01741 01742 /* ========================================================================== 01743 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd1_Struct 01744 *! \brief Sensor Read Command1 Register bit field structure 01745 * ========================================================================== */ 01746 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd1_t { 01747 union { 01748 struct { 01749 uint8_t Digital_Sensor_Read_Cmd1 : 8; /**< Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01750 }; 01751 uint8_t VALUE8; 01752 }; 01753 } ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd1_t; 01754 01755 /*@}*/ 01756 01757 /** @defgroup Digital_Sensor_Read_Cmd2 Sensor Read Command2 (Digital_Sensor_Read_Cmd2) Register 01758 * Sensor Read Command2 (Digital_Sensor_Read_Cmd2) Register. 01759 * @{ 01760 */ 01761 01762 /* ========================================================================== 01763 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd2_Struct 01764 *! \brief Sensor Read Command2 Register bit field structure 01765 * ========================================================================== */ 01766 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd2_t { 01767 union { 01768 struct { 01769 uint8_t Digital_Sensor_Read_Cmd2 : 8; /**< Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01770 }; 01771 uint8_t VALUE8; 01772 }; 01773 } ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd2_t; 01774 01775 /*@}*/ 01776 01777 /** @defgroup Digital_Sensor_Read_Cmd3 Sensor Read Command3 (Digital_Sensor_Read_Cmd3) Register 01778 * Sensor Read Command3 (Digital_Sensor_Read_Cmd3) Register. 01779 * @{ 01780 */ 01781 01782 /* ========================================================================== 01783 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd3_Struct 01784 *! \brief Sensor Read Command3 Register bit field structure 01785 * ========================================================================== */ 01786 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd3_t { 01787 union { 01788 struct { 01789 uint8_t Digital_Sensor_Read_Cmd3 : 8; /**< Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01790 }; 01791 uint8_t VALUE8; 01792 }; 01793 } ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd3_t; 01794 01795 /*@}*/ 01796 01797 /** @defgroup Digital_Sensor_Read_Cmd4 Sensor Read Command4 (Digital_Sensor_Read_Cmd4) Register 01798 * Sensor Read Command4 (Digital_Sensor_Read_Cmd4) Register. 01799 * @{ 01800 */ 01801 01802 /* ========================================================================== 01803 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd4_Struct 01804 *! \brief Sensor Read Command4 Register bit field structure 01805 * ========================================================================== */ 01806 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd4_t { 01807 union { 01808 struct { 01809 uint8_t Digital_Sensor_Read_Cmd4 : 8; /**< Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01810 }; 01811 uint8_t VALUE8; 01812 }; 01813 } ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd4_t; 01814 01815 /*@}*/ 01816 01817 /** @defgroup Digital_Sensor_Read_Cmd5 Sensor Read Command5 (Digital_Sensor_Read_Cmd5) Register 01818 * Sensor Read Command5 (Digital_Sensor_Read_Cmd5) Register. 01819 * @{ 01820 */ 01821 01822 /* ========================================================================== 01823 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd5_Struct 01824 *! \brief Sensor Read Command5 Register bit field structure 01825 * ========================================================================== */ 01826 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd5_t { 01827 union { 01828 struct { 01829 uint8_t Digital_Sensor_Read_Cmd5 : 8; /**< Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01830 }; 01831 uint8_t VALUE8; 01832 }; 01833 } ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd5_t; 01834 01835 /*@}*/ 01836 01837 /** @defgroup Digital_Sensor_Read_Cmd6 Sensor Read Command6 (Digital_Sensor_Read_Cmd6) Register 01838 * Sensor Read Command6 (Digital_Sensor_Read_Cmd6) Register. 01839 * @{ 01840 */ 01841 01842 /* ========================================================================== 01843 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd6_Struct 01844 *! \brief Sensor Read Command6 Register bit field structure 01845 * ========================================================================== */ 01846 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd6_t { 01847 union { 01848 struct { 01849 uint8_t Digital_Sensor_Read_Cmd6 : 8; /**< Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01850 }; 01851 uint8_t VALUE8; 01852 }; 01853 } ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd6_t; 01854 01855 /*@}*/ 01856 01857 /** @defgroup Digital_Sensor_Read_Cmd7 Sensor Read Command7 (Digital_Sensor_Read_Cmd7) Register 01858 * Sensor Read Command7 (Digital_Sensor_Read_Cmd7) Register. 01859 * @{ 01860 */ 01861 01862 /* ========================================================================== 01863 *! \struct ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd7_Struct 01864 *! \brief Sensor Read Command7 Register bit field structure 01865 * ========================================================================== */ 01866 typedef struct _ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd7_t { 01867 union { 01868 struct { 01869 uint8_t Digital_Sensor_Read_Cmd7 : 8; /**< Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01870 }; 01871 uint8_t VALUE8; 01872 }; 01873 } ADI_ADISENSE_CORE_Digital_Sensor_Read_Cmd7_t; 01874 01875 /*@}*/ 01876 01877 /** @defgroup test_reg_0 Test Register 0 (test_reg_0) Register 01878 * Test Register 0 (test_reg_0) Register. 01879 * @{ 01880 */ 01881 01882 /* ========================================================================== 01883 *! \struct ADI_ADSENSE_TEST_test_reg_0_Struct 01884 *! \brief Test Register 0 Register bit field structure 01885 * ========================================================================== */ 01886 typedef struct _ADI_ADSENSE_TEST_test_reg_0_t { 01887 union { 01888 struct { 01889 uint8_t Test_Command : 8; /**< Test_Command */ 01890 }; 01891 }; 01892 } ADI_ADSENSE_TEST_test_reg_0_t; 01893 01894 /*@}*/ 01895 01896 01897 /* ========================================================================== 01898 *! \struct ADI_ADISENSE_CORE_test_adc_cal_temp 01899 *! \brief ADC-derived calibration temperature 01900 * ========================================================================== */ 01901 typedef struct _ADI_ADISENSE_CORE_test_adc_cal_temp_t { 01902 union { 01903 struct { 01904 float Temperature; /**< Current temperature from ADC, used for calibration unless REG_ADISENSE_TEST_USER_CAL_TEMP is set */ 01905 }; 01906 float VALUE32; 01907 }; 01908 } ADI_ADISENSE_CORE_test_adc_cal_temp_t; 01909 01910 01911 /* ========================================================================== 01912 *! \struct ADI_ADISENSE_CORE_test_user_cal_temp 01913 *! \brief User-specified calibration temperature 01914 * ========================================================================== */ 01915 typedef struct _ADI_ADISENSE_CORE_test_user_cal_temp_t { 01916 union { 01917 struct { 01918 float Temperature; /**< Fixed temperature reference to use for calibration. Ignored if set as NaN. */ 01919 }; 01920 float VALUE32; 01921 }; 01922 } ADI_ADISENSE_CORE_test_user_cal_temp_t; 01923 01924 #if defined (__CC_ARM) 01925 #pragma pop 01926 #endif 01927 01928 #endif 01929
Generated on Tue Jul 12 2022 21:13:17 by
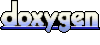