TMRh20 ported to MBED
Fork of TMRh20 by
Embed:
(wiki syntax)
Show/hide line numbers
RF24.h
Go to the documentation of this file.
00001 /* 00002 Copyright (C) 2011 J. Coliz <maniacbug@ymail.com> 00003 00004 This program is free software; you can redistribute it and/or 00005 modify it under the terms of the GNU General Public License 00006 version 2 as published by the Free Software Foundation. 00007 */ 00008 00009 /** 00010 * @file RF24.h 00011 * 00012 * Class declaration for RF24 and helper enums 00013 */ 00014 00015 #ifndef __RF24_H__ 00016 #define __RF24_H__ 00017 00018 #include "RF24_config.h" 00019 #include "mbed.h" 00020 00021 /** 00022 * Power Amplifier level. 00023 * 00024 * For use with setPALevel() 00025 */ 00026 typedef enum { RF24_PA_MIN = 0,RF24_PA_LOW, RF24_PA_HIGH, RF24_PA_MAX, RF24_PA_ERROR } rf24_pa_dbm_e ; 00027 00028 /** 00029 * Data rate. How fast data moves through the air. 00030 * 00031 * For use with setDataRate() 00032 */ 00033 typedef enum { RF24_1MBPS = 0, RF24_2MBPS, RF24_250KBPS } rf24_datarate_e; 00034 00035 /** 00036 * CRC Length. How big (if any) of a CRC is included. 00037 * 00038 * For use with setCRCLength() 00039 */ 00040 typedef enum { RF24_CRC_DISABLED = 0, RF24_CRC_8, RF24_CRC_16 } rf24_crclength_e; 00041 00042 /** 00043 * Driver for nRF24L01(+) 2.4GHz Wireless Transceiver 00044 */ 00045 00046 class RF24 00047 { 00048 private: 00049 00050 SPI *spi; 00051 00052 DigitalOut ce_pin; /**< "Chip Enable" pin, activates the RX or TX role */ 00053 DigitalOut csn_pin; /**< SPI Chip select */ 00054 00055 bool p_variant; /* False for RF24L01 and true for RF24L01P */ 00056 uint8_t payload_size; /**< Fixed size of payloads */ 00057 bool dynamic_payloads_enabled; /**< Whether dynamic payloads are enabled. */ 00058 uint8_t pipe0_reading_address[5]; /**< Last address set on pipe 0 for reading. */ 00059 uint8_t addr_width; /**< The address width to use - 3,4 or 5 bytes. */ 00060 00061 00062 protected: 00063 /** 00064 * SPI transactions 00065 * 00066 * Common code for SPI transactions including CSN toggle 00067 * 00068 */ 00069 inline void beginTransaction(); 00070 00071 inline void endTransaction(); 00072 00073 public: 00074 00075 /** 00076 * @name Primary public interface 00077 * 00078 * These are the main methods you need to operate the chip 00079 */ 00080 /**@{*/ 00081 00082 /** 00083 * MBED Constructor 00084 * 00085 * Creates a new instance of this driver. Before using, you create an instance 00086 * and send in the unique pins that this chip is connected to. 00087 * 00088 * @param _cepin The pin attached to Chip Enable on the RF module 00089 * @param _cspin The pin attached to Chip Select 00090 * @param spispeed For RPi, the SPI speed in MHZ ie: BCM2835_SPI_SPEED_8MHZ 00091 */ 00092 00093 RF24(SPI *spi, PinName _cepin, PinName _cspin); 00094 00095 /** 00096 * Begin operation of the chip 00097 * 00098 * Call this in setup(), before calling any other methods. 00099 * @code radio.begin() @endcode 00100 */ 00101 bool begin(void); 00102 00103 /** 00104 * Checks if the chip is connected to the SPI bus 00105 */ 00106 bool isChipConnected(); 00107 00108 /** 00109 * Start listening on the pipes opened for reading. 00110 * 00111 * 1. Be sure to call openReadingPipe() first. 00112 * 2. Do not call write() while in this mode, without first calling stopListening(). 00113 * 3. Call available() to check for incoming traffic, and read() to get it. 00114 * 00115 * @code 00116 * Open reading pipe 1 using address CCCECCCECC 00117 * 00118 * byte address[] = { 0xCC,0xCE,0xCC,0xCE,0xCC }; 00119 * radio.openReadingPipe(1,address); 00120 * radio.startListening(); 00121 * @endcode 00122 */ 00123 void startListening(void); 00124 00125 /** 00126 * Stop listening for incoming messages, and switch to transmit mode. 00127 * 00128 * Do this before calling write(). 00129 * @code 00130 * radio.stopListening(); 00131 * radio.write(&data,sizeof(data)); 00132 * @endcode 00133 */ 00134 void stopListening(void); 00135 00136 /** 00137 * Check whether there are bytes available to be read 00138 * @code 00139 * if(radio.available()){ 00140 * radio.read(&data,sizeof(data)); 00141 * } 00142 * @endcode 00143 * @return True if there is a payload available, false if none is 00144 */ 00145 bool available(void); 00146 00147 /** 00148 * Read the available payload 00149 * 00150 * The size of data read is the fixed payload size, see getPayloadSize() 00151 * 00152 * @note I specifically chose 'void*' as a data type to make it easier 00153 * for beginners to use. No casting needed. 00154 * 00155 * @note No longer boolean. Use available to determine if packets are 00156 * available. Interrupt flags are now cleared during reads instead of 00157 * when calling available(). 00158 * 00159 * @param buf Pointer to a buffer where the data should be written 00160 * @param len Maximum number of bytes to read into the buffer 00161 * 00162 * @code 00163 * if(radio.available()){ 00164 * radio.read(&data,sizeof(data)); 00165 * } 00166 * @endcode 00167 * @return No return value. Use available(). 00168 */ 00169 void read( void* buf, uint8_t len ); 00170 00171 /** 00172 * Be sure to call openWritingPipe() first to set the destination 00173 * of where to write to. 00174 * 00175 * This blocks until the message is successfully acknowledged by 00176 * the receiver or the timeout/retransmit maxima are reached. In 00177 * the current configuration, the max delay here is 60-70ms. 00178 * 00179 * The maximum size of data written is the fixed payload size, see 00180 * getPayloadSize(). However, you can write less, and the remainder 00181 * will just be filled with zeroes. 00182 * 00183 * TX/RX/RT interrupt flags will be cleared every time write is called 00184 * 00185 * @param buf Pointer to the data to be sent 00186 * @param len Number of bytes to be sent 00187 * 00188 * @code 00189 * radio.stopListening(); 00190 * radio.write(&data,sizeof(data)); 00191 * @endcode 00192 * @return True if the payload was delivered successfully false if not 00193 */ 00194 bool write( const void* buf, uint8_t len ); 00195 00196 /** 00197 * New: Open a pipe for writing via byte array. Old addressing format retained 00198 * for compatibility. 00199 * 00200 * Only one writing pipe can be open at once, but you can change the address 00201 * you'll write to. Call stopListening() first. 00202 * 00203 * Addresses are assigned via a byte array, default is 5 byte address length 00204 s * 00205 * @code 00206 * uint8_t addresses[][6] = {"1Node","2Node"}; 00207 * radio.openWritingPipe(addresses[0]); 00208 * @endcode 00209 * @code 00210 * uint8_t address[] = { 0xCC,0xCE,0xCC,0xCE,0xCC }; 00211 * radio.openWritingPipe(address); 00212 * address[0] = 0x33; 00213 * radio.openReadingPipe(1,address); 00214 * @endcode 00215 * @see setAddressWidth 00216 * 00217 * @param address The address of the pipe to open. Coordinate these pipe 00218 * addresses amongst nodes on the network. 00219 */ 00220 00221 void openWritingPipe(const uint8_t *address); 00222 00223 /** 00224 * Open a pipe for reading 00225 * 00226 * Up to 6 pipes can be open for reading at once. Open all the required 00227 * reading pipes, and then call startListening(). 00228 * 00229 * @see openWritingPipe 00230 * @see setAddressWidth 00231 * 00232 * @note Pipes 0 and 1 will store a full 5-byte address. Pipes 2-5 will technically 00233 * only store a single byte, borrowing up to 4 additional bytes from pipe #1 per the 00234 * assigned address width. 00235 * @warning Pipes 1-5 should share the same address, except the first byte. 00236 * Only the first byte in the array should be unique, e.g. 00237 * @code 00238 * uint8_t addresses[][6] = {"1Node","2Node"}; 00239 * openReadingPipe(1,addresses[0]); 00240 * openReadingPipe(2,addresses[1]); 00241 * @endcode 00242 * 00243 * @warning Pipe 0 is also used by the writing pipe. So if you open 00244 * pipe 0 for reading, and then startListening(), it will overwrite the 00245 * writing pipe. Ergo, do an openWritingPipe() again before write(). 00246 * 00247 * @param number Which pipe# to open, 0-5. 00248 * @param address The 24, 32 or 40 bit address of the pipe to open. 00249 */ 00250 00251 void openReadingPipe(uint8_t number, const uint8_t *address); 00252 00253 /**@}*/ 00254 /** 00255 * @name Advanced Operation 00256 * 00257 * Methods you can use to drive the chip in more advanced ways 00258 */ 00259 /**@{*/ 00260 00261 /** 00262 * Print a giant block of debugging information to stdout 00263 * 00264 * @warning Does nothing if stdout is not defined. See fdevopen in stdio.h 00265 * The printf.h file is included with the library for Arduino. 00266 * @code 00267 * #include <printf.h> 00268 * setup(){ 00269 * Serial.begin(115200); 00270 * printf_begin(); 00271 * ... 00272 * } 00273 * @endcode 00274 */ 00275 void printDetails(void); 00276 00277 /** 00278 * Test whether there are bytes available to be read in the 00279 * FIFO buffers. 00280 * 00281 * @param[out] pipe_num Which pipe has the payload available 00282 * 00283 * @code 00284 * uint8_t pipeNum; 00285 * if(radio.available(&pipeNum)){ 00286 * radio.read(&data,sizeof(data)); 00287 * Serial.print("Got data on pipe"); 00288 * Serial.println(pipeNum); 00289 * } 00290 * @endcode 00291 * @return True if there is a payload available, false if none is 00292 */ 00293 bool available(uint8_t* pipe_num); 00294 00295 /** 00296 * Check if the radio needs to be read. Can be used to prevent data loss 00297 * @return True if all three 32-byte radio buffers are full 00298 */ 00299 bool rxFifoFull(); 00300 00301 /** 00302 * Enter low-power mode 00303 * 00304 * To return to normal power mode, call powerUp(). 00305 * 00306 * @note After calling startListening(), a basic radio will consume about 13.5mA 00307 * at max PA level. 00308 * During active transmission, the radio will consume about 11.5mA, but this will 00309 * be reduced to 26uA (.026mA) between sending. 00310 * In full powerDown mode, the radio will consume approximately 900nA (.0009mA) 00311 * 00312 * @code 00313 * radio.powerDown(); 00314 * avr_enter_sleep_mode(); // Custom function to sleep the device 00315 * radio.powerUp(); 00316 * @endcode 00317 */ 00318 void powerDown(void); 00319 00320 /** 00321 * Leave low-power mode - required for normal radio operation after calling powerDown() 00322 * 00323 * To return to low power mode, call powerDown(). 00324 * @note This will take up to 5ms for maximum compatibility 00325 */ 00326 void powerUp(void) ; 00327 00328 /** 00329 * Write for single NOACK writes. Optionally disables acknowledgements/autoretries for a single write. 00330 * 00331 * @note enableDynamicAck() must be called to enable this feature 00332 * 00333 * Can be used with enableAckPayload() to request a response 00334 * @see enableDynamicAck() 00335 * @see setAutoAck() 00336 * @see write() 00337 * 00338 * @param buf Pointer to the data to be sent 00339 * @param len Number of bytes to be sent 00340 * @param multicast Request ACK (0), NOACK (1) 00341 */ 00342 bool write( const void* buf, uint8_t len, const bool multicast ); 00343 00344 /** 00345 * This will not block until the 3 FIFO buffers are filled with data. 00346 * Once the FIFOs are full, writeFast will simply wait for success or 00347 * timeout, and return 1 or 0 respectively. From a user perspective, just 00348 * keep trying to send the same data. The library will keep auto retrying 00349 * the current payload using the built in functionality. 00350 * @warning It is important to never keep the nRF24L01 in TX mode and FIFO full for more than 4ms at a time. If the auto 00351 * retransmit is enabled, the nRF24L01 is never in TX mode long enough to disobey this rule. Allow the FIFO 00352 * to clear by issuing txStandBy() or ensure appropriate time between transmissions. 00353 * 00354 * @code 00355 * Example (Partial blocking): 00356 * 00357 * radio.writeFast(&buf,32); // Writes 1 payload to the buffers 00358 * txStandBy(); // Returns 0 if failed. 1 if success. Blocks only until MAX_RT timeout or success. Data flushed on fail. 00359 * 00360 * radio.writeFast(&buf,32); // Writes 1 payload to the buffers 00361 * txStandBy(1000); // Using extended timeouts, returns 1 if success. Retries failed payloads for 1 seconds before returning 0. 00362 * @endcode 00363 * 00364 * @see txStandBy() 00365 * @see write() 00366 * @see writeBlocking() 00367 * 00368 * @param buf Pointer to the data to be sent 00369 * @param len Number of bytes to be sent 00370 * @return True if the payload was delivered successfully false if not 00371 */ 00372 bool writeFast( const void* buf, uint8_t len ); 00373 00374 /** 00375 * WriteFast for single NOACK writes. Disables acknowledgements/autoretries for a single write. 00376 * 00377 * @note enableDynamicAck() must be called to enable this feature 00378 * @see enableDynamicAck() 00379 * @see setAutoAck() 00380 * 00381 * @param buf Pointer to the data to be sent 00382 * @param len Number of bytes to be sent 00383 * @param multicast Request ACK (0) or NOACK (1) 00384 */ 00385 bool writeFast( const void* buf, uint8_t len, const bool multicast ); 00386 00387 /** 00388 * This function extends the auto-retry mechanism to any specified duration. 00389 * It will not block until the 3 FIFO buffers are filled with data. 00390 * If so the library will auto retry until a new payload is written 00391 * or the user specified timeout period is reached. 00392 * @warning It is important to never keep the nRF24L01 in TX mode and FIFO full for more than 4ms at a time. If the auto 00393 * retransmit is enabled, the nRF24L01 is never in TX mode long enough to disobey this rule. Allow the FIFO 00394 * to clear by issuing txStandBy() or ensure appropriate time between transmissions. 00395 * 00396 * @code 00397 * Example (Full blocking): 00398 * 00399 * radio.writeBlocking(&buf,32,1000); //Wait up to 1 second to write 1 payload to the buffers 00400 * txStandBy(1000); //Wait up to 1 second for the payload to send. Return 1 if ok, 0 if failed. 00401 * //Blocks only until user timeout or success. Data flushed on fail. 00402 * @endcode 00403 * @note If used from within an interrupt, the interrupt should be disabled until completion, and sei(); called to enable millis(). 00404 * @see txStandBy() 00405 * @see write() 00406 * @see writeFast() 00407 * 00408 * @param buf Pointer to the data to be sent 00409 * @param len Number of bytes to be sent 00410 * @param timeout User defined timeout in milliseconds. 00411 * @return True if the payload was loaded into the buffer successfully false if not 00412 */ 00413 bool writeBlocking( const void* buf, uint8_t len, uint32_t timeout ); 00414 00415 /** 00416 * This function should be called as soon as transmission is finished to 00417 * drop the radio back to STANDBY-I mode. If not issued, the radio will 00418 * remain in STANDBY-II mode which, per the data sheet, is not a recommended 00419 * operating mode. 00420 * 00421 * @note When transmitting data in rapid succession, it is still recommended by 00422 * the manufacturer to drop the radio out of TX or STANDBY-II mode if there is 00423 * time enough between sends for the FIFOs to empty. This is not required if auto-ack 00424 * is enabled. 00425 * 00426 * Relies on built-in auto retry functionality. 00427 * 00428 * @code 00429 * Example (Partial blocking): 00430 * 00431 * radio.writeFast(&buf,32); 00432 * radio.writeFast(&buf,32); 00433 * radio.writeFast(&buf,32); //Fills the FIFO buffers up 00434 * bool ok = txStandBy(); //Returns 0 if failed. 1 if success. 00435 * //Blocks only until MAX_RT timeout or success. Data flushed on fail. 00436 * @endcode 00437 * @see txStandBy(unsigned long timeout) 00438 * @return True if transmission is successful 00439 * 00440 */ 00441 bool txStandBy(); 00442 00443 /** 00444 * This function allows extended blocking and auto-retries per a user defined timeout 00445 * @code 00446 * Fully Blocking Example: 00447 * 00448 * radio.writeFast(&buf,32); 00449 * radio.writeFast(&buf,32); 00450 * radio.writeFast(&buf,32); //Fills the FIFO buffers up 00451 * bool ok = txStandBy(1000); //Returns 0 if failed after 1 second of retries. 1 if success. 00452 * //Blocks only until user defined timeout or success. Data flushed on fail. 00453 * @endcode 00454 * @note If used from within an interrupt, the interrupt should be disabled until completion, and sei(); called to enable millis(). 00455 * @param timeout Number of milliseconds to retry failed payloads 00456 * @return True if transmission is successful 00457 * 00458 */ 00459 bool txStandBy(uint32_t timeout, bool startTx = 0); 00460 00461 /** 00462 * Write an ack payload for the specified pipe 00463 * 00464 * The next time a message is received on @p pipe, the data in @p buf will 00465 * be sent back in the acknowledgement. 00466 * @see enableAckPayload() 00467 * @see enableDynamicPayloads() 00468 * @warning Only three of these can be pending at any time as there are only 3 FIFO buffers.<br> Dynamic payloads must be enabled. 00469 * @note Ack payloads are handled automatically by the radio chip when a payload is received. Users should generally 00470 * write an ack payload as soon as startListening() is called, so one is available when a regular payload is received. 00471 * @note Ack payloads are dynamic payloads. This only works on pipes 0&1 by default. Call 00472 * enableDynamicPayloads() to enable on all pipes. 00473 * 00474 * @param pipe Which pipe# (typically 1-5) will get this response. 00475 * @param buf Pointer to data that is sent 00476 * @param len Length of the data to send, up to 32 bytes max. Not affected 00477 * by the static payload set by setPayloadSize(). 00478 */ 00479 void writeAckPayload(uint8_t pipe, const void* buf, uint8_t len); 00480 00481 /** 00482 * Determine if an ack payload was received in the most recent call to 00483 * write(). The regular available() can also be used. 00484 * 00485 * Call read() to retrieve the ack payload. 00486 * 00487 * @return True if an ack payload is available. 00488 */ 00489 bool isAckPayloadAvailable(void); 00490 00491 /** 00492 * Call this when you get an interrupt to find out why 00493 * 00494 * Tells you what caused the interrupt, and clears the state of 00495 * interrupts. 00496 * 00497 * @param[out] tx_ok The send was successful (TX_DS) 00498 * @param[out] tx_fail The send failed, too many retries (MAX_RT) 00499 * @param[out] rx_ready There is a message waiting to be read (RX_DS) 00500 */ 00501 void whatHappened(bool& tx_ok,bool& tx_fail,bool& rx_ready); 00502 00503 /** 00504 * Non-blocking write to the open writing pipe used for buffered writes 00505 * 00506 * @note Optimization: This function now leaves the CE pin high, so the radio 00507 * will remain in TX or STANDBY-II Mode until a txStandBy() command is issued. Can be used as an alternative to startWrite() 00508 * if writing multiple payloads at once. 00509 * @warning It is important to never keep the nRF24L01 in TX mode with FIFO full for more than 4ms at a time. If the auto 00510 * retransmit/autoAck is enabled, the nRF24L01 is never in TX mode long enough to disobey this rule. Allow the FIFO 00511 * to clear by issuing txStandBy() or ensure appropriate time between transmissions. 00512 * 00513 * @see write() 00514 * @see writeFast() 00515 * @see startWrite() 00516 * @see writeBlocking() 00517 * 00518 * For single noAck writes see: 00519 * @see enableDynamicAck() 00520 * @see setAutoAck() 00521 * 00522 * @param buf Pointer to the data to be sent 00523 * @param len Number of bytes to be sent 00524 * @param multicast Request ACK (0) or NOACK (1) 00525 * @return True if the payload was delivered successfully false if not 00526 */ 00527 void startFastWrite( const void* buf, uint8_t len, const bool multicast, bool startTx = 1 ); 00528 00529 /** 00530 * Non-blocking write to the open writing pipe 00531 * 00532 * Just like write(), but it returns immediately. To find out what happened 00533 * to the send, catch the IRQ and then call whatHappened(). 00534 * 00535 * @see write() 00536 * @see writeFast() 00537 * @see startFastWrite() 00538 * @see whatHappened() 00539 * 00540 * For single noAck writes see: 00541 * @see enableDynamicAck() 00542 * @see setAutoAck() 00543 * 00544 * @param buf Pointer to the data to be sent 00545 * @param len Number of bytes to be sent 00546 * @param multicast Request ACK (0) or NOACK (1) 00547 * 00548 */ 00549 void startWrite( const void* buf, uint8_t len, const bool multicast ); 00550 00551 /** 00552 * This function is mainly used internally to take advantage of the auto payload 00553 * re-use functionality of the chip, but can be beneficial to users as well. 00554 * 00555 * The function will instruct the radio to re-use the data in the FIFO buffers, 00556 * and instructs the radio to re-send once the timeout limit has been reached. 00557 * Used by writeFast and writeBlocking to initiate retries when a TX failure 00558 * occurs. Retries are automatically initiated except with the standard write(). 00559 * This way, data is not flushed from the buffer until switching between modes. 00560 * 00561 * @note This is to be used AFTER auto-retry fails if wanting to resend 00562 * using the built-in payload reuse features. 00563 * After issuing reUseTX(), it will keep reending the same payload forever or until 00564 * a payload is written to the FIFO, or a flush_tx command is given. 00565 */ 00566 void reUseTX(); 00567 00568 /** 00569 * Empty the transmit buffer. This is generally not required in standard operation. 00570 * May be required in specific cases after stopListening() , if operating at 250KBPS data rate. 00571 * 00572 * @return Current value of status register 00573 */ 00574 uint8_t flush_tx(void); 00575 00576 /** 00577 * Test whether there was a carrier on the line for the 00578 * previous listening period. 00579 * 00580 * Useful to check for interference on the current channel. 00581 * 00582 * @return true if was carrier, false if not 00583 */ 00584 bool testCarrier(void); 00585 00586 /** 00587 * Test whether a signal (carrier or otherwise) greater than 00588 * or equal to -64dBm is present on the channel. Valid only 00589 * on nRF24L01P (+) hardware. On nRF24L01, use testCarrier(). 00590 * 00591 * Useful to check for interference on the current channel and 00592 * channel hopping strategies. 00593 * 00594 * @code 00595 * bool goodSignal = radio.testRPD(); 00596 * if(radio.available()){ 00597 * Serial.println(goodSignal ? "Strong signal > 64dBm" : "Weak signal < 64dBm" ); 00598 * radio.read(0,0); 00599 * } 00600 * @endcode 00601 * @return true if signal => -64dBm, false if not 00602 */ 00603 bool testRPD(void) ; 00604 00605 /** 00606 * Test whether this is a real radio, or a mock shim for 00607 * debugging. Setting either pin to 0xff is the way to 00608 * indicate that this is not a real radio. 00609 * 00610 * @return true if this is a legitimate radio 00611 */ 00612 bool isValid() { return ce_pin != 0xff && csn_pin != 0xff; } 00613 00614 /** 00615 * Close a pipe after it has been previously opened. 00616 * Can be safely called without having previously opened a pipe. 00617 * @param pipe Which pipe # to close, 0-5. 00618 */ 00619 void closeReadingPipe( uint8_t pipe ) ; 00620 00621 /** 00622 * Enable error detection by un-commenting #define FAILURE_HANDLING in RF24_config.h 00623 * If a failure has been detected, it usually indicates a hardware issue. By default the library 00624 * will cease operation when a failure is detected. 00625 * This should allow advanced users to detect and resolve intermittent hardware issues. 00626 * 00627 * In most cases, the radio must be re-enabled via radio.begin(); and the appropriate settings 00628 * applied after a failure occurs, if wanting to re-enable the device immediately. 00629 * 00630 * Usage: (Failure handling must be enabled per above) 00631 * @code 00632 * if(radio.failureDetected){ 00633 * radio.begin(); // Attempt to re-configure the radio with defaults 00634 * radio.failureDetected = 0; // Reset the detection value 00635 * radio.openWritingPipe(addresses[1]); // Re-configure pipe addresses 00636 * radio.openReadingPipe(1,addresses[0]); 00637 * report_failure(); // Blink leds, send a message, etc. to indicate failure 00638 * } 00639 * @endcode 00640 */ 00641 //#if defined (FAILURE_HANDLING) 00642 bool failureDetected; 00643 //#endif 00644 00645 /**@}*/ 00646 00647 /**@}*/ 00648 /** 00649 * @name Optional Configurators 00650 * 00651 * Methods you can use to get or set the configuration of the chip. 00652 * None are required. Calling begin() sets up a reasonable set of 00653 * defaults. 00654 */ 00655 /**@{*/ 00656 00657 /** 00658 * Set the address width from 3 to 5 bytes (24, 32 or 40 bit) 00659 * 00660 * @param a_width The address width to use: 3,4 or 5 00661 */ 00662 00663 void setAddressWidth(uint8_t a_width); 00664 00665 /** 00666 * Set the number and delay of retries upon failed submit 00667 * 00668 * @param delay How long to wait between each retry, in multiples of 250us, 00669 * max is 15. 0 means 250us, 15 means 4000us. 00670 * @param count How many retries before giving up, max 15 00671 */ 00672 void setRetries(uint8_t delay, uint8_t count); 00673 00674 /** 00675 * Set RF communication channel 00676 * 00677 * @param channel Which RF channel to communicate on, 0-125 00678 */ 00679 void setChannel(uint8_t channel); 00680 00681 /** 00682 * Get RF communication channel 00683 * 00684 * @return The currently configured RF Channel 00685 */ 00686 uint8_t getChannel(void); 00687 00688 /** 00689 * Set Static Payload Size 00690 * 00691 * This implementation uses a pre-stablished fixed payload size for all 00692 * transmissions. If this method is never called, the driver will always 00693 * transmit the maximum payload size (32 bytes), no matter how much 00694 * was sent to write(). 00695 * 00696 * @todo Implement variable-sized payloads feature 00697 * 00698 * @param size The number of bytes in the payload 00699 */ 00700 void setPayloadSize(uint8_t size); 00701 00702 /** 00703 * Get Static Payload Size 00704 * 00705 * @see setPayloadSize() 00706 * 00707 * @return The number of bytes in the payload 00708 */ 00709 uint8_t getPayloadSize(void); 00710 00711 /** 00712 * Get Dynamic Payload Size 00713 * 00714 * For dynamic payloads, this pulls the size of the payload off 00715 * the chip 00716 * 00717 * @note Corrupt packets are now detected and flushed per the 00718 * manufacturer. 00719 * @code 00720 * if(radio.available()){ 00721 * if(radio.getDynamicPayloadSize() < 1){ 00722 * // Corrupt payload has been flushed 00723 * return; 00724 * } 00725 * radio.read(&data,sizeof(data)); 00726 * } 00727 * @endcode 00728 * 00729 * @return Payload length of last-received dynamic payload 00730 */ 00731 uint8_t getDynamicPayloadSize(void); 00732 00733 /** 00734 * Enable custom payloads on the acknowledge packets 00735 * 00736 * Ack payloads are a handy way to return data back to senders without 00737 * manually changing the radio modes on both units. 00738 * 00739 * @note Ack payloads are dynamic payloads. This only works on pipes 0&1 by default. Call 00740 * enableDynamicPayloads() to enable on all pipes. 00741 */ 00742 void enableAckPayload(void); 00743 00744 /** 00745 * Enable dynamically-sized payloads 00746 * 00747 * This way you don't always have to send large packets just to send them 00748 * once in a while. This enables dynamic payloads on ALL pipes. 00749 * 00750 */ 00751 void enableDynamicPayloads(void); 00752 00753 /** 00754 * Disable dynamically-sized payloads 00755 * 00756 * This disables dynamic payloads on ALL pipes. Since Ack Payloads 00757 * requires Dynamic Payloads, Ack Payloads are also disabled. 00758 * If dynamic payloads are later re-enabled and ack payloads are desired 00759 * then enableAckPayload() must be called again as well. 00760 * 00761 */ 00762 void disableDynamicPayloads(void); 00763 00764 /** 00765 * Enable dynamic ACKs (single write multicast or unicast) for chosen messages 00766 * 00767 * @note To enable full multicast or per-pipe multicast, use setAutoAck() 00768 * 00769 * @warning This MUST be called prior to attempting single write NOACK calls 00770 * @code 00771 * radio.enableDynamicAck(); 00772 * radio.write(&data,32,1); // Sends a payload with no acknowledgement requested 00773 * radio.write(&data,32,0); // Sends a payload using auto-retry/autoACK 00774 * @endcode 00775 */ 00776 void enableDynamicAck(); 00777 00778 /** 00779 * Determine whether the hardware is an nRF24L01+ or not. 00780 * 00781 * @return true if the hardware is nRF24L01+ (or compatible) and false 00782 * if its not. 00783 */ 00784 bool isPVariant(void) ; 00785 00786 /** 00787 * Enable or disable auto-acknowlede packets 00788 * 00789 * This is enabled by default, so it's only needed if you want to turn 00790 * it off for some reason. 00791 * 00792 * @param enable Whether to enable (true) or disable (false) auto-acks 00793 */ 00794 void setAutoAck(bool enable); 00795 00796 /** 00797 * Enable or disable auto-acknowlede packets on a per pipeline basis. 00798 * 00799 * AA is enabled by default, so it's only needed if you want to turn 00800 * it off/on for some reason on a per pipeline basis. 00801 * 00802 * @param pipe Which pipeline to modify 00803 * @param enable Whether to enable (true) or disable (false) auto-acks 00804 */ 00805 void setAutoAck( uint8_t pipe, bool enable ) ; 00806 00807 /** 00808 * Set Power Amplifier (PA) level to one of four levels: 00809 * RF24_PA_MIN, RF24_PA_LOW, RF24_PA_HIGH and RF24_PA_MAX 00810 * 00811 * The power levels correspond to the following output levels respectively: 00812 * NRF24L01: -18dBm, -12dBm,-6dBM, and 0dBm 00813 * 00814 * SI24R1: -6dBm, 0dBm, 3dBM, and 7dBm. 00815 * 00816 * @param level Desired PA level. 00817 */ 00818 void setPALevel ( uint8_t level ); 00819 00820 /** 00821 * Fetches the current PA level. 00822 * 00823 * NRF24L01: -18dBm, -12dBm, -6dBm and 0dBm 00824 * SI24R1: -6dBm, 0dBm, 3dBm, 7dBm 00825 * 00826 * @return Returns values 0 to 3 representing the PA Level. 00827 */ 00828 uint8_t getPALevel( void ); 00829 00830 /** 00831 * Set the transmission data rate 00832 * 00833 * @warning setting RF24_250KBPS will fail for non-plus units 00834 * 00835 * @param speed RF24_250KBPS for 250kbs, RF24_1MBPS for 1Mbps, or RF24_2MBPS for 2Mbps 00836 * @return true if the change was successful 00837 */ 00838 bool setDataRate(rf24_datarate_e speed); 00839 00840 /** 00841 * Fetches the transmission data rate 00842 * 00843 * @return Returns the hardware's currently configured datarate. The value 00844 * is one of 250kbs, RF24_1MBPS for 1Mbps, or RF24_2MBPS, as defined in the 00845 * rf24_datarate_e enum. 00846 */ 00847 rf24_datarate_e getDataRate( void ) ; 00848 00849 /** 00850 * Set the CRC length 00851 * <br>CRC checking cannot be disabled if auto-ack is enabled 00852 * @param length RF24_CRC_8 for 8-bit or RF24_CRC_16 for 16-bit 00853 */ 00854 void setCRCLength(rf24_crclength_e length); 00855 00856 /** 00857 * Get the CRC length 00858 * <br>CRC checking cannot be disabled if auto-ack is enabled 00859 * @return RF24_CRC_DISABLED if disabled or RF24_CRC_8 for 8-bit or RF24_CRC_16 for 16-bit 00860 */ 00861 rf24_crclength_e getCRCLength(void); 00862 00863 /** 00864 * Disable CRC validation 00865 * 00866 * @warning CRC cannot be disabled if auto-ack/ESB is enabled. 00867 */ 00868 void disableCRC( void ) ; 00869 00870 /** 00871 * The radio will generate interrupt signals when a transmission is complete, 00872 * a transmission fails, or a payload is received. This allows users to mask 00873 * those interrupts to prevent them from generating a signal on the interrupt 00874 * pin. Interrupts are enabled on the radio chip by default. 00875 * 00876 * @code 00877 * Mask all interrupts except the receive interrupt: 00878 * 00879 * radio.maskIRQ(1,1,0); 00880 * @endcode 00881 * 00882 * @param tx_ok Mask transmission complete interrupts 00883 * @param tx_fail Mask transmit failure interrupts 00884 * @param rx_ready Mask payload received interrupts 00885 */ 00886 void maskIRQ(bool tx_ok,bool tx_fail,bool rx_ready); 00887 00888 /** 00889 * 00890 * The driver will delay for this duration when stopListening() is called 00891 * 00892 * When responding to payloads, faster devices like ARM(RPi) are much faster than Arduino: 00893 * 1. Arduino sends data to RPi, switches to RX mode 00894 * 2. The RPi receives the data, switches to TX mode and sends before the Arduino radio is in RX mode 00895 * 3. If AutoACK is disabled, this can be set as low as 0. If AA/ESB enabled, set to 100uS minimum on RPi 00896 * 00897 * @warning If set to 0, ensure 130uS delay after stopListening() and before any sends 00898 */ 00899 00900 uint32_t txDelay; 00901 00902 /** 00903 * 00904 * On all devices but Linux and ATTiny, a small delay is added to the CSN toggling function 00905 * 00906 * This is intended to minimise the speed of SPI polling due to radio commands 00907 * 00908 * If using interrupts or timed requests, this can be set to 0 Default:5 00909 */ 00910 00911 uint32_t csDelay; 00912 00913 /**@}*/ 00914 /** 00915 * @name Deprecated 00916 * 00917 * Methods provided for backwards compabibility. 00918 */ 00919 /**@{*/ 00920 00921 00922 /** 00923 * Open a pipe for reading 00924 * @note For compatibility with old code only, see new function 00925 * 00926 * @warning Pipes 1-5 should share the first 32 bits. 00927 * Only the least significant byte should be unique, e.g. 00928 * @code 00929 * openReadingPipe(1,0xF0F0F0F0AA); 00930 * openReadingPipe(2,0xF0F0F0F066); 00931 * @endcode 00932 * 00933 * @warning Pipe 0 is also used by the writing pipe. So if you open 00934 * pipe 0 for reading, and then startListening(), it will overwrite the 00935 * writing pipe. Ergo, do an openWritingPipe() again before write(). 00936 * 00937 * @param number Which pipe# to open, 0-5. 00938 * @param address The 40-bit address of the pipe to open. 00939 */ 00940 void openReadingPipe(uint8_t number, uint64_t address); 00941 00942 /** 00943 * Open a pipe for writing 00944 * @note For compatibility with old code only, see new function 00945 * 00946 * Addresses are 40-bit hex values, e.g.: 00947 * 00948 * @code 00949 * openWritingPipe(0xF0F0F0F0F0); 00950 * @endcode 00951 * 00952 * @param address The 40-bit address of the pipe to open. 00953 */ 00954 void openWritingPipe(uint64_t address); 00955 00956 /** 00957 * Empty the receive buffer 00958 * 00959 * @return Current value of status register 00960 */ 00961 uint8_t flush_rx(void); 00962 00963 private: 00964 00965 /** 00966 * @name Low-level internal interface. 00967 * 00968 * Protected methods that address the chip directly. Regular users cannot 00969 * ever call these. They are documented for completeness and for developers who 00970 * may want to extend this class. 00971 */ 00972 /**@{*/ 00973 00974 /** 00975 * Set chip select pin 00976 * 00977 * Running SPI bus at PI_CLOCK_DIV2 so we don't waste time transferring data 00978 * and best of all, we make use of the radio's FIFO buffers. A lower speed 00979 * means we're less likely to effectively leverage our FIFOs and pay a higher 00980 * AVR runtime cost as toll. 00981 * 00982 * @param mode HIGH to take this unit off the SPI bus, LOW to put it on 00983 */ 00984 void csn(bool mode); 00985 00986 /** 00987 * Set chip enable 00988 * 00989 * @param level HIGH to actively begin transmission or LOW to put in standby. Please see data sheet 00990 * for a much more detailed description of this pin. 00991 */ 00992 void ce(bool level); 00993 00994 /** 00995 * Read a chunk of data in from a register 00996 * 00997 * @param reg Which register. Use constants from nRF24L01.h 00998 * @param buf Where to put the data 00999 * @param len How many bytes of data to transfer 01000 * @return Current value of status register 01001 */ 01002 uint8_t read_register(uint8_t reg, uint8_t* buf, uint8_t len); 01003 01004 /** 01005 * Read single byte from a register 01006 * 01007 * @param reg Which register. Use constants from nRF24L01.h 01008 * @return Current value of register @p reg 01009 */ 01010 uint8_t read_register(uint8_t reg); 01011 01012 /** 01013 * Write a chunk of data to a register 01014 * 01015 * @param reg Which register. Use constants from nRF24L01.h 01016 * @param buf Where to get the data 01017 * @param len How many bytes of data to transfer 01018 * @return Current value of status register 01019 */ 01020 uint8_t write_register(uint8_t reg, const uint8_t* buf, uint8_t len); 01021 01022 /** 01023 * Write a single byte to a register 01024 * 01025 * @param reg Which register. Use constants from nRF24L01.h 01026 * @param value The new value to write 01027 * @return Current value of status register 01028 */ 01029 uint8_t write_register(uint8_t reg, uint8_t value); 01030 01031 /** 01032 * Write the transmit payload 01033 * 01034 * The size of data written is the fixed payload size, see getPayloadSize() 01035 * 01036 * @param buf Where to get the data 01037 * @param len Number of bytes to be sent 01038 * @return Current value of status register 01039 */ 01040 uint8_t write_payload(const void* buf, uint8_t len, const uint8_t writeType); 01041 01042 /** 01043 * Read the receive payload 01044 * 01045 * The size of data read is the fixed payload size, see getPayloadSize() 01046 * 01047 * @param buf Where to put the data 01048 * @param len Maximum number of bytes to read 01049 * @return Current value of status register 01050 */ 01051 uint8_t read_payload(void* buf, uint8_t len); 01052 01053 /** 01054 * Retrieve the current status of the chip 01055 * 01056 * @return Current value of status register 01057 */ 01058 uint8_t get_status(void); 01059 01060 #if !defined (MINIMAL) 01061 /** 01062 * Decode and print the given status to stdout 01063 * 01064 * @param status Status value to print 01065 * 01066 * @warning Does nothing if stdout is not defined. See fdevopen in stdio.h 01067 */ 01068 void print_status(uint8_t status); 01069 01070 /** 01071 * Decode and print the given 'observe_tx' value to stdout 01072 * 01073 * @param value The observe_tx value to print 01074 * 01075 * @warning Does nothing if stdout is not defined. See fdevopen in stdio.h 01076 */ 01077 void print_observe_tx(uint8_t value); 01078 01079 /** 01080 * Print the name and value of an 8-bit register to stdout 01081 * 01082 * Optionally it can print some quantity of successive 01083 * registers on the same line. This is useful for printing a group 01084 * of related registers on one line. 01085 * 01086 * @param name Name of the register 01087 * @param reg Which register. Use constants from nRF24L01.h 01088 * @param qty How many successive registers to print 01089 */ 01090 void print_byte_register(const char* name, uint8_t reg, uint8_t qty = 1); 01091 01092 /** 01093 * Print the name and value of a 40-bit address register to stdout 01094 * 01095 * Optionally it can print some quantity of successive 01096 * registers on the same line. This is useful for printing a group 01097 * of related registers on one line. 01098 * 01099 * @param name Name of the register 01100 * @param reg Which register. Use constants from nRF24L01.h 01101 * @param qty How many successive registers to print 01102 */ 01103 void print_address_register(const char* name, uint8_t reg, uint8_t qty = 1); 01104 #endif 01105 /** 01106 * Turn on or off the special features of the chip 01107 * 01108 * The chip has certain 'features' which are only available when the 'features' 01109 * are enabled. See the datasheet for details. 01110 */ 01111 void toggle_features(void); 01112 01113 /** 01114 * Built in spi transfer function to simplify repeating code repeating code 01115 */ 01116 01117 uint8_t spiTrans(uint8_t cmd); 01118 01119 #if defined (FAILURE_HANDLING) || defined (RF24_LINUX) 01120 void errNotify(void); 01121 #endif 01122 01123 /**@}*/ 01124 01125 }; 01126 01127 01128 #endif // __RF24_H__
Generated on Wed Jul 13 2022 22:18:21 by
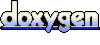