Samples of how to use the micro:bit DAL with mbed. This is a hg clone of the real git repo that can be found here: https://github.com/lancaster-university/microbit-samples
Dependencies: microbit
Fork of microbit-samples by
LogicGates.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 #include "MicroBit.h" 00027 #include "MicroBitSamples.h" 00028 00029 #ifdef MICROBIT_SAMPLE_LOGIC_GATES 00030 00031 #define LOGIC_MODE_NOT 1 00032 #define LOGIC_MODE_AND 2 00033 #define LOGIC_MODE_OR 3 00034 #define LOGIC_MODE_OUTPUT 4 00035 00036 #define LOGIC_MODE_MIN 1 00037 #define LOGIC_MODE_MAX 4 00038 00039 #define TOOL_SELECT_DELAY 1000 00040 00041 MicroBit uBit; 00042 00043 MicroBitImage NOT("\ 00044 0 0 1 0 0\n\ 00045 0 0 1 1 0\n\ 00046 1 1 1 1 1\n\ 00047 0 0 1 1 0\n\ 00048 0 0 1 0 0\n"); 00049 00050 MicroBitImage AND("\ 00051 0 0 1 1 0\n\ 00052 1 1 1 1 1\n\ 00053 0 0 1 1 1\n\ 00054 1 1 1 1 1\n\ 00055 0 0 1 1 0\n"); 00056 00057 MicroBitImage OR("\ 00058 0 0 0 1 0\n\ 00059 1 1 1 1 1\n\ 00060 0 0 0 1 1\n\ 00061 1 1 1 1 1\n\ 00062 0 0 0 1 0\n"); 00063 00064 MicroBitImage OUTPUT_ON("\ 00065 0 1 1 1 0\n\ 00066 1 1 1 1 1\n\ 00067 1 1 1 1 1\n\ 00068 1 1 1 1 1\n\ 00069 0 1 1 1 0\n"); 00070 00071 MicroBitImage OUTPUT_OFF("\ 00072 0 1 1 1 0\n\ 00073 1 0 0 0 1\n\ 00074 1 0 0 0 1\n\ 00075 1 0 0 0 1\n\ 00076 0 1 1 1 0\n"); 00077 00078 int mode = LOGIC_MODE_NOT; 00079 00080 void onShake(MicroBitEvent) 00081 { 00082 // The micro:bit has been shaken, so move on to the next logic gate. 00083 mode++; 00084 00085 // Wrap back to the start if necessary. 00086 if (mode > LOGIC_MODE_MAX) 00087 mode = LOGIC_MODE_MIN; 00088 00089 // Update the display to 00090 switch (mode) 00091 { 00092 case LOGIC_MODE_NOT: 00093 uBit.display.print(NOT); 00094 break; 00095 00096 case LOGIC_MODE_AND: 00097 uBit.display.print(AND); 00098 break; 00099 00100 case LOGIC_MODE_OR: 00101 uBit.display.print(OR); 00102 break; 00103 00104 case LOGIC_MODE_OUTPUT: 00105 uBit.display.print(OUTPUT_OFF); 00106 break; 00107 } 00108 } 00109 00110 int main() 00111 { 00112 // Initialise the micro:bit runtime. 00113 uBit.init(); 00114 00115 // Register to receive events when the micro:bit is shaken. 00116 uBit.messageBus.listen(MICROBIT_ID_GESTURE, MICROBIT_ACCELEROMETER_EVT_SHAKE, onShake); 00117 00118 // 00119 // Create a simple logic gate simulator, using the P0, P1 and P2 pins. 00120 // The micro:bit can then be configured as an NOT / AND / OR gate, by shaking the device. 00121 // 00122 int output = 0; 00123 00124 // Our icons are drawn left to right, so rotate the display so the outputs point at the pins on the edge connector. :-) 00125 uBit.display.rotateTo(MICROBIT_DISPLAY_ROTATION_270); 00126 00127 while (1) 00128 { 00129 // Check inputs and update outputs accordingly. 00130 switch (mode) 00131 { 00132 int o1; 00133 int o2; 00134 00135 case LOGIC_MODE_NOT: 00136 output = uBit.buttonA.isPressed() ? 0 : !uBit.io.P0.getDigitalValue(); 00137 uBit.display.print(NOT); 00138 break; 00139 00140 case LOGIC_MODE_AND: 00141 o1 = uBit.buttonA.isPressed() || uBit.io.P0.getDigitalValue(); 00142 o2 = uBit.buttonB.isPressed() || uBit.io.P1.getDigitalValue(); 00143 output = o1 && o2; 00144 break; 00145 00146 case LOGIC_MODE_OR: 00147 output = uBit.buttonA.isPressed() || uBit.io.P0.getDigitalValue() || uBit.buttonB.isPressed() || uBit.io.P1.getDigitalValue(); 00148 break; 00149 00150 case LOGIC_MODE_OUTPUT: 00151 if (uBit.io.P0.getDigitalValue()) 00152 uBit.display.print(OUTPUT_ON); 00153 else 00154 uBit.display.print(OUTPUT_OFF); 00155 00156 output = 0; 00157 break; 00158 } 00159 00160 // Update output pin value 00161 uBit.io.P2.setDigitalValue(output); 00162 00163 // Perform a power efficient sleep for a little while. No need to run too quickly! 00164 uBit.sleep(1000); 00165 } 00166 } 00167 00168 #endif
Generated on Thu Jul 14 2022 08:55:58 by
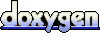