
Working reset, flipped logic
Dependencies: SDFileSystem emic2 mbed-rtos mbed
Fork of BAT_senior_design_Nhi by
button.h
00001 #include "mbed.h" 00002 00003 #ifndef BUTTON_H 00004 #define BUTTON_H 00005 00006 // This is a button class for our custom button 00007 class button { 00008 00009 // pins connected to the button 00010 private: 00011 PwmOut servo; 00012 DigitalIn pb; 00013 int state; // where is the button (0 - 4) 00014 int press; // is the button up or down 00015 int id; // this is the ID, each button should have a unique id 00016 // int mode; // is the system in reading or typing mode 00017 //AnalogIn linpot; 00018 00019 public: 00020 // constructors 00021 button(); // Default 00022 button(PwmOut servo, DigitalIn pb, int id); 00023 //button(PwmOut servo, DigitalIn pb, AnalogIn linpot); 00024 00025 // button(PwmOut servo, DigitalIn pb, AnalogIn linpot); 00026 // functions 00027 PwmOut getServoPin(); // get the servo pin 00028 //void setState(int state); // set state 00029 //void setMode(int mode); // set mode 00030 void setState(int); // set what state the button is in - up or down 00031 void setPress(int); // set the button press 00032 void moveServoIn(); // move servo into the slot 00033 void moveServoOut(); // move servo out of the slot 00034 int getID(); 00035 int updateState(); 00036 int getState(); 00037 int getPress(); 00038 int getLp(); 00039 void setup(); 00040 }; 00041 00042 #endif
Generated on Mon Jul 18 2022 15:26:09 by
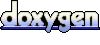