
Bluemix Demo program
Dependencies: WNCInterface mbed-rtos mbed
main.cpp
00001 //#define MQTT_DEBUG 00002 00003 00004 #include "mbed.h" 00005 #include "MQTTClient.h" 00006 #include "MQTTFormat.h" 00007 00008 #include "MQTTWNCInterface.h" 00009 #include "rtos.h" 00010 #include "k64f.h" 00011 #include "HTS221.h" 00012 00013 I2C i2c(PTC11, PTC10); //SDA, SCL -- define the I2C pins being used 00014 MODSERIAL pc(USBTX,USBRX,256,256); 00015 00016 #include "hardware.h" 00017 // 00018 // When the Bluemix device is created, the following information is provided: 00019 // 00020 // Organization ID 9k09br 00021 // Device Type ATTIOTK 00022 // Device ID Mz-11027214-7010 00023 // Authentication Method token 00024 // Authentication Token SecurityToken99 00025 // Use this information for the following defines: 00026 00027 #define ORG_ID "k1hdoc" 00028 #define DEVICE_TYPE "Avnet-WNC_card" 00029 #define DEVICE_NAME "IoT-11027214-2016" 00030 #define USERNAME "use-token-auth" // not required for demo app 00031 #define PASSWORD "SecurityToken99" // not required for demo app 00032 00033 #define URL ORG_ID ".messaging.internetofthings.ibmcloud.com" 00034 #define CLIENTSTR "d:" ORG_ID ":" DEVICE_TYPE ":%s" 00035 00036 #define PORT 1883 // MQTT broker port number 00037 #define PUBLISH_TOPIC "iot-2/evt/status/fmt/json" // MQTT topic 00038 #define SUBSCRIBTOPIC "iot-2/cmd/+/fmt/+" 00039 00040 00041 Queue<uint32_t, 6> messageQ; 00042 00043 // LED color control function 00044 void controlLED(color_t led_color) { 00045 switch(led_color) { 00046 case red : 00047 greenLED = blueLED = 1; 00048 redLED = 0.7; 00049 break; 00050 case green : 00051 redLED = blueLED = 1; 00052 greenLED = 0.7; 00053 break; 00054 case blue : 00055 redLED = greenLED = 1; 00056 blueLED = 0.7; 00057 break; 00058 case off : 00059 redLED = greenLED = blueLED = 1; 00060 break; 00061 } 00062 } 00063 00064 // Switch 2 interrupt handler 00065 void sw2_ISR(void) { 00066 messageQ.put((uint32_t*)22); 00067 } 00068 00069 // Switch3 interrupt handler 00070 void sw3_ISR(void) { 00071 messageQ.put((uint32_t*)33); 00072 } 00073 00074 // MQTT message arrived callback function 00075 void messageArrived(MQTT::MessageData& md) { 00076 MQTT::Message &message = md.message; 00077 PRINTF("Receiving MQTT message: %.*s\r\n", message.payloadlen, (char*)message.payload); 00078 00079 if (message.payloadlen == 3) { 00080 if (strncmp((char*)message.payload, "red", 3) == 0) 00081 controlLED(red); 00082 00083 else if(strncmp((char*)message.payload, "grn", 3) == 0) 00084 controlLED(green); 00085 00086 else if(strncmp((char*)message.payload, "blu", 3) == 0) 00087 controlLED(blue); 00088 00089 else if(strncmp((char*)message.payload, "off", 3) == 0) 00090 controlLED(off); 00091 } 00092 } 00093 00094 int main() { 00095 int rc, pSW2=0, txSel=0, good = 0; 00096 Timer tmr; 00097 char* topic = PUBLISH_TOPIC; 00098 char clientID[100], buf[100]; 00099 string st, uniqueID; 00100 00101 HTS221 hts221; 00102 00103 pc.baud(115200); 00104 rc = hts221.init(); 00105 if ( rc ) { 00106 PRINTF(BLU "HTS221 Detected (0x%02X)\n\r",rc); 00107 PRINTF(" Temp is: %0.2f F \r\n Huumid is: %02d %%\r\n\r\n", 00108 CTOF(hts221.readTemperature()), hts221.readHumidity()/10); 00109 } 00110 else { 00111 PRINTF(RED "HTS221 NOT DETECTED!\n\r"); 00112 } 00113 00114 controlLED(green); 00115 00116 // set SW2 and SW3 to generate interrupt on falling edge 00117 switch2.fall(&sw2_ISR); 00118 switch3.fall(&sw3_ISR); 00119 00120 // initialize ethernet interface 00121 MQTTwnc ipstack = MQTTwnc(); 00122 00123 // get and display client network info 00124 WNCInterface& eth = ipstack.getEth(); 00125 00126 // construct the MQTT client 00127 MQTT::Client<MQTTwnc, Countdown> client = MQTT::Client<MQTTwnc, Countdown>(ipstack); 00128 00129 controlLED(blue); 00130 00131 char* hostname = URL; 00132 int port = PORT; 00133 st = eth.getMACAddress(); 00134 uniqueID="IoT-"; 00135 uniqueID += st[0]; 00136 uniqueID += st[1]; 00137 uniqueID += st[3]; 00138 uniqueID += st[4]; 00139 uniqueID += st[6]; 00140 uniqueID += st[7]; 00141 uniqueID += st[9]; 00142 uniqueID += st[10]; 00143 uniqueID += "-2016"; 00144 00145 sprintf(clientID, CLIENTSTR, uniqueID.c_str()); 00146 00147 PRINTF("\r\n\r\n"); 00148 PRINTF(" _____\r\n"); 00149 PRINTF(" * *\r\n"); 00150 PRINTF(" *____ *____ Bluemix IIoT Demo using\r\n"); 00151 PRINTF(" * *===* *==* the AT&T IoT Starter Kit\r\n"); 00152 PRINTF(" *___*===*___** AVNET\r\n"); 00153 PRINTF(" *======*\r\n"); 00154 PRINTF(" *====*\r\n"); 00155 PRINTF("\r\n"); 00156 PRINTF("This demonstration program operates the same as the original \r\n"); 00157 PRINTF("MicroZed IIoT Starter Kit except it only reads from the HTS221 \r\n"); 00158 PRINTF("temp sensor (no 31855 currently present and no generated data).\r\n"); 00159 PRINTF("\r\n"); 00160 PRINTF("Local network info...\r\n"); 00161 PRINTF("IP address is %s\r\n", eth.getIPAddress()); 00162 PRINTF("MAC address is %s\r\n", eth.getMACAddress()); 00163 PRINTF("Gateway address is %s\r\n", eth.getGateway()); 00164 PRINTF("Your <uniqueID> is: %s\r\n", uniqueID.c_str()); 00165 PRINTF("---------------------------------------------------------------\r\n"); 00166 00167 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00168 00169 int tries; 00170 00171 while( !good ) { 00172 tries=0; 00173 // connect to TCP socket and check return code 00174 tmr.start(); 00175 rc = 1; 00176 while( rc && tries < 3) { 00177 PRINTF("\r\n\r\n(%d) Attempting TCP connect to %s:%d: ", tries++, hostname, port); 00178 rc = ipstack.connect(hostname, port); 00179 if( rc ) { 00180 PRINTF("Failed (%d)!\r\n",rc); 00181 while( tmr.read_ms() < 5000 ) ; 00182 tmr.reset(); 00183 } 00184 else { 00185 PRINTF("Success!\r\n"); 00186 rc = 0; 00187 } 00188 } 00189 if( tries < 3 ) 00190 tries = 0; 00191 else 00192 continue; 00193 00194 data.willFlag = 0; 00195 data.MQTTVersion = 3; 00196 00197 data.clientID.cstring = clientID; 00198 data.username.cstring = USERNAME; 00199 data.password.cstring = PASSWORD; 00200 data.keepAliveInterval = 10; 00201 data.cleansession = 1; 00202 00203 rc = 1; 00204 tmr.reset(); 00205 while( !client.isConnected() && rc && tries < 3) { 00206 PRINTF("(%d) Attempting MQTT connect to '%s': ", tries++, clientID); 00207 rc = client.connect(data); 00208 if( rc ) { 00209 PRINTF("Failed (%d)!\r\n",rc); 00210 while( tmr.read_ms() < 5000 ); 00211 tmr.reset(); 00212 } 00213 else 00214 PRINTF("Success!\r\n"); 00215 } 00216 00217 if( tries < 3 ) 00218 tries = 0; 00219 else 00220 continue; 00221 00222 // subscribe to MQTT topic 00223 tmr.reset(); 00224 rc = 1; 00225 while( rc && client.isConnected() && tries < 3) { 00226 PRINTF("(%d) Attempting to subscribing to MQTT topic '%s': ", tries, SUBSCRIBTOPIC); 00227 rc = client.subscribe(SUBSCRIBTOPIC, MQTT::QOS0, messageArrived); 00228 if( rc ) { 00229 PRINTF("Failed (%d)!\r\n", rc); 00230 while( tmr.read_ms() < 5000 ); 00231 tries++; 00232 tmr.reset(); 00233 } 00234 else { 00235 good=1; 00236 PRINTF("Subscribe successful!\r\n"); 00237 } 00238 } 00239 while (!good); 00240 } 00241 00242 MQTT::Message message; 00243 message.qos = MQTT::QOS0; 00244 message.retained = false; 00245 message.dup = false; 00246 message.payload = (void*)buf; 00247 00248 while(true) { 00249 osEvent switchEvent = messageQ.get(100); 00250 00251 if( switchEvent.value.v == 22 ) //switch between sending humidity & temp 00252 txSel = !txSel; 00253 00254 if( switchEvent.value.v == 33) //user wants to run in Quickstart of Demo mode 00255 pSW2 = !pSW2; 00256 00257 memset(buf,0x00,sizeof(buf)); 00258 if( txSel ) { 00259 rc = hts221.readHumidity(); 00260 sprintf(buf, "{\"d\" : {\"humd\" : \"%2d.%d\" }}", rc/10, rc-((rc/10)*10)); 00261 PRINTF("Publishing MQTT message '%s' ", (char*)message.payload); 00262 } 00263 else { 00264 sprintf(buf, "{\"d\" : {\"temp\" : %5d }}", (int)CTOF(hts221.readTemperature())*10); 00265 PRINTF("Publishing MQTT message '%s' ", (char*)message.payload); 00266 } 00267 message.payloadlen = strlen(buf); 00268 PRINTF("(%d)\r\n",message.payloadlen); 00269 rc = client.publish(topic, message); 00270 if( rc ) { 00271 PRINTF("Publish request failed! (%d)\r\n",rc); 00272 FATAL_WNC_ERROR(resume); 00273 } 00274 00275 client.yield(6000); 00276 } 00277 }
Generated on Fri Jul 15 2022 21:54:07 by
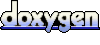