
Code APP3
Dependencies: mbed EthernetInterface WebSocketClient mbed-rtos BufferedSerial
Fork of APP3_Lab by
MMA8452Q.cpp
00001 // Library for our MMA8452Q 3-axis accelerometer 00002 // Based on the MMA8452Q Arduino Library by Jim Lindblom (SparkFun Electronics) 00003 00004 #include "mbed.h" 00005 #include "MMA8452Q.h" 00006 00007 // Constructor 00008 MMA8452Q::MMA8452Q(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr) 00009 { 00010 // Initialize members 00011 scale = DEFAULT_FSR; 00012 } 00013 00014 // Destructor 00015 MMA8452Q::~MMA8452Q() 00016 { 00017 00018 } 00019 00020 // Initialization 00021 bool MMA8452Q::init() 00022 { 00023 // Check to make sure the chip's ID matches the factory ID 00024 uint8_t c = readRegister(REG_WHO_AM_I); 00025 if( c != FACTORY_ID ) { 00026 return false; 00027 } 00028 00029 // Set default scale and data rate 00030 standby(); 00031 setScale(DEFAULT_FSR); 00032 setODR(DEFAULT_ODR); 00033 active(); 00034 00035 return true; 00036 } 00037 00038 // Set the full-scale range for x, y, and z data 00039 void MMA8452Q::setScale(uint8_t fsr) 00040 { 00041 uint8_t config = readRegister(REG_XYZ_DATA_CFG); 00042 scale = fsr; 00043 config &= 0xFC; // Mask out FSR bits 00044 fsr = fsr >> 2; // Trick to translate scale to FSR bits 00045 fsr &= 0x03; // Mask out acceptable FSRs 00046 config |= fsr; // Write FSR bits to config byte 00047 writeRegister(REG_XYZ_DATA_CFG, config); // Write config back to register 00048 } 00049 00050 // Set the Output Data Rate 00051 void MMA8452Q::setODR(uint8_t odr) 00052 { 00053 uint8_t ctrl = readRegister(REG_CTRL_REG1); 00054 ctrl &= 0xCF; // Mask out data rate bits 00055 odr &= 0x07; // Mask out acceptable ODRs 00056 ctrl |= (odr << 3); // Write ODR bits to control byte 00057 writeRegister(REG_CTRL_REG1, ctrl); // Write control back to register 00058 } 00059 00060 // Set accelerometer into standby mode 00061 void MMA8452Q::standby() 00062 { 00063 uint8_t c = readRegister(REG_CTRL_REG1); 00064 c &= ~(0x01); // Clear bit 0 to go into standby 00065 writeRegister(REG_CTRL_REG1, c); // Write back to CONTROL register 00066 } 00067 00068 // Set accelerometer into active mode 00069 void MMA8452Q::active() 00070 { 00071 uint8_t c = readRegister(REG_CTRL_REG1); 00072 c |= 0x01; // Set bit 0 to go into active mode 00073 writeRegister(REG_CTRL_REG1, c); // Write back to CONTROL register 00074 } 00075 00076 // Read X registers 00077 float MMA8452Q::readX() 00078 { 00079 int16_t x = 0; 00080 float cx = 0; 00081 00082 // Read MSB and LSB from X registers 00083 x = readRegister(OUT_X_MSB); 00084 x = x << 8; 00085 x |= readRegister(OUT_X_LSB); 00086 x = x >> 4; 00087 00088 // Calculate human readable X 00089 cx = (float)x / (float)2048 * (float)(scale); 00090 00091 return cx; 00092 } 00093 00094 // Read Y registers 00095 float MMA8452Q::readY() 00096 { 00097 int16_t y = 0; 00098 float cy = 0; 00099 00100 // Read MSB and LSB from Y registers 00101 y = readRegister(OUT_Y_MSB); 00102 y = y << 8; 00103 y |= readRegister(OUT_Y_LSB); 00104 y = y >> 4; 00105 00106 // Calculate human readable Y 00107 cy = (float)y / (float)2048 * (float)(scale); 00108 00109 return cy; 00110 } 00111 00112 // Read Z registers 00113 float MMA8452Q::readZ() 00114 { 00115 int16_t z = 0; 00116 float cz = 0; 00117 00118 // Read MSB and LSB from Z registers 00119 z = readRegister(OUT_Z_MSB); 00120 z = z << 8; 00121 z |= readRegister(OUT_Z_LSB); 00122 z = z >> 4; 00123 00124 // Calculate human readable Z 00125 cz = (float)z / (float)2048 * (float)(scale); 00126 00127 return cz; 00128 } 00129 00130 // Raw read register over I2C 00131 uint8_t MMA8452Q::readRegister(uint8_t reg) 00132 { 00133 uint8_t dev_addr; 00134 uint8_t data; 00135 00136 // I2C address are bits [6..1] in the transmitted byte, so we shift by 1 00137 dev_addr = m_addr << 1; 00138 00139 // Write device address with a trailing 'write' bit 00140 m_i2c.start(); 00141 m_i2c.write(dev_addr & 0xFE); 00142 00143 // Write register address 00144 m_i2c.write(reg); 00145 00146 // Write a start bit and device address with a trailing 'read' bit 00147 m_i2c.start(); 00148 m_i2c.write(dev_addr | 0x01); 00149 00150 // Read single byte from I2C device 00151 data = m_i2c.read(0); 00152 m_i2c.stop(); 00153 00154 return data; 00155 } 00156 00157 // Raw write data to a register over I2C 00158 void MMA8452Q::writeRegister(uint8_t reg, uint8_t data) 00159 { 00160 uint8_t dev_addr; 00161 00162 // I2C address are bits [6..1] in the transmitted byte, so we shift by 1 00163 dev_addr = m_addr << 1; 00164 00165 // Write device address with a trailing 'write' bit 00166 m_i2c.start(); 00167 m_i2c.write(dev_addr & 0xFE); 00168 00169 // Write register address 00170 m_i2c.write(reg); 00171 00172 // Write the data to the register 00173 m_i2c.write(data); 00174 m_i2c.stop(); 00175 }
Generated on Sat Jul 30 2022 02:06:50 by
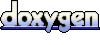