Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
gpio.cpp
00001 /***************************************************************************//** 00002 * @file gpio.cpp 00003 * @brief Implementation of GPIO Mbed platform driver interfaces 00004 ******************************************************************************** 00005 * Copyright (c) 2019 - 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 /******************************************************************************/ 00014 /************************ Includes Files *******************************/ 00015 /******************************************************************************/ 00016 #include <stdio.h> 00017 #include <stdlib.h> 00018 #include <mbed.h> 00019 00020 // Platform drivers needs to be C-compatible to work with other drivers 00021 #ifdef __cplusplus 00022 extern "C" 00023 { 00024 #endif // _cplusplus 00025 00026 #include "error.h" 00027 #include "gpio.h" 00028 #include "gpio_extra.h" 00029 00030 /******************************************************************************/ 00031 /************************ Functions Definitions *******************************/ 00032 /******************************************************************************/ 00033 00034 /** 00035 * @brief Obtain the GPIO decriptor. 00036 * @param desc - The GPIO descriptor. 00037 * @param gpio_number - The number of the GPIO. 00038 * @return SUCCESS in case of success, FAILURE otherwise. 00039 */ 00040 int32_t gpio_get(struct gpio_desc **desc, const struct gpio_init_param *param) 00041 { 00042 gpio_desc *new_gpio; 00043 00044 if (desc && param) { 00045 // Create the gpio description object for the device 00046 new_gpio = (gpio_desc *)calloc(1, sizeof(gpio_desc)) ; 00047 if (!new_gpio) { 00048 goto err_new_gpio; 00049 } 00050 00051 new_gpio->number = param->number; 00052 00053 // Create the gpio extra descriptor object to store extra mbed gpio info 00054 mbed_gpio_desc *gpio_desc_extra = (mbed_gpio_desc *)calloc(1, 00055 sizeof(mbed_gpio_desc)); 00056 if (!gpio_desc_extra) { 00057 goto err_gpio_desc_extra; 00058 } 00059 00060 gpio_desc_extra->direction = GPIO_IN; 00061 gpio_desc_extra->gpio_pin = NULL; 00062 00063 if (param->extra) { 00064 gpio_desc_extra->pin_mode = ((mbed_gpio_init_param *)param->extra)->pin_mode; 00065 } else { 00066 gpio_desc_extra->pin_mode = NULL; 00067 } 00068 00069 new_gpio->extra = gpio_desc_extra; 00070 *desc = new_gpio; 00071 00072 return SUCCESS; 00073 } 00074 00075 err_gpio_desc_extra: 00076 free(new_gpio); 00077 err_new_gpio: 00078 // Nothing to free 00079 00080 return FAILURE; 00081 } 00082 00083 00084 /** 00085 * @brief Get the value of an optional GPIO. 00086 * @param desc - The GPIO descriptor. 00087 * @param param - GPIO Initialization parameters. 00088 * @return SUCCESS in case of success, FAILURE otherwise. 00089 */ 00090 int32_t gpio_get_optional(struct gpio_desc **desc, 00091 const struct gpio_init_param *param) 00092 { 00093 if (param) { 00094 return gpio_get(desc, param); 00095 } else { 00096 *desc = NULL; 00097 return SUCCESS; 00098 } 00099 } 00100 00101 00102 /** 00103 * @brief Free the resources allocated by gpio_get(). 00104 * @param desc - The GPIO descriptor. 00105 * @return SUCCESS in case of success, FAILURE otherwise. 00106 */ 00107 int32_t gpio_remove(struct gpio_desc *desc) 00108 { 00109 if (desc) { 00110 // Free the gpio object 00111 if(((mbed_gpio_desc *)(desc->extra))->gpio_pin) { 00112 free(((mbed_gpio_desc *)(desc->extra))->gpio_pin); 00113 } 00114 00115 // Free the gpio extra descriptor object 00116 if((mbed_gpio_desc *)(desc->extra)) { 00117 free((mbed_gpio_desc *)(desc->extra)); 00118 } 00119 00120 // Free the gpio descriptor object 00121 free(desc); 00122 00123 return SUCCESS; 00124 } 00125 00126 return FAILURE; 00127 } 00128 00129 00130 /** 00131 * @brief Enable the input direction of the specified GPIO. 00132 * @param desc - The GPIO descriptor. 00133 * @return SUCCESS in case of success, FAILURE otherwise. 00134 * @note does not support reconfiguration of already set pin direction 00135 */ 00136 int32_t gpio_direction_input(struct gpio_desc *desc) 00137 { 00138 DigitalIn *gpio_input; // pointer to gpio input object 00139 mbed_gpio_desc *gpio_desc_extra; // pointer to gpio desc extra parameters 00140 00141 if (desc && desc->extra) { 00142 gpio_desc_extra = (mbed_gpio_desc *)(desc->extra); 00143 if (gpio_desc_extra->gpio_pin == NULL) { 00144 // Configure and instantiate GPIO pin as input 00145 gpio_input = new DigitalIn((PinName)desc->number); 00146 if (!gpio_input) { 00147 goto err_gpio_input; 00148 } 00149 00150 gpio_desc_extra->gpio_pin = (mbed_gpio_desc *)gpio_input; 00151 gpio_desc_extra->direction = GPIO_IN; 00152 00153 // Set the gpio pin mode 00154 gpio_input->mode((PinMode)((mbed_gpio_desc *)desc->extra)->pin_mode); 00155 00156 return SUCCESS; 00157 } 00158 } 00159 00160 err_gpio_input: 00161 // Nothing to free 00162 00163 return FAILURE; 00164 } 00165 00166 00167 /** 00168 * @brief Enable the output direction of the specified GPIO. 00169 * @param desc - The GPIO descriptor. 00170 * @param value - The value. 00171 * Example: GPIO_HIGH 00172 * GPIO_LOW 00173 * @return SUCCESS in case of success, FAILURE otherwise. 00174 * @note does not support reconfiguration of already set pin direction 00175 */ 00176 int32_t gpio_direction_output(struct gpio_desc *desc, uint8_t value) 00177 { 00178 DigitalOut *gpio_output; // pointer to gpio output object 00179 mbed_gpio_desc *gpio_desc_extra; // pointer to gpio desc extra parameters 00180 00181 if(desc && desc->extra) { 00182 gpio_desc_extra = (mbed_gpio_desc *)(desc->extra); 00183 if (gpio_desc_extra->gpio_pin == NULL) { 00184 00185 // Configure and instantiate GPIO pin as output 00186 gpio_output = new DigitalOut((PinName)desc->number); 00187 00188 if (!gpio_output) { 00189 goto err_gpio_output; 00190 } 00191 00192 gpio_desc_extra->gpio_pin = (mbed_gpio_desc *)gpio_output; 00193 gpio_desc_extra->direction = GPIO_OUT; 00194 00195 // Set the GPIO value 00196 if(gpio_set_value(desc, value) == SUCCESS) { 00197 return SUCCESS; 00198 } 00199 } 00200 } 00201 00202 err_gpio_output: 00203 // Nothing to free 00204 00205 return FAILURE; 00206 } 00207 00208 00209 /** 00210 * @brief Get the direction of the specified GPIO. 00211 * @param desc - The GPIO descriptor. 00212 * @param direction - The direction. 00213 * Example: GPIO_OUT 00214 * GPIO_IN 00215 * @return SUCCESS in case of success, FAILURE otherwise. 00216 */ 00217 int32_t gpio_get_direction(struct gpio_desc *desc, uint8_t *direction) 00218 { 00219 mbed_gpio_desc *gpio_desc_extra; // pointer to gpio desc extra parameters 00220 00221 if(desc && desc->extra) { 00222 gpio_desc_extra = (mbed_gpio_desc *)(desc->extra); 00223 00224 if (gpio_desc_extra->gpio_pin) { 00225 *direction = gpio_desc_extra->direction; 00226 } 00227 00228 return SUCCESS; 00229 } 00230 00231 return FAILURE; 00232 } 00233 00234 00235 /** 00236 * @brief Set the value of the specified GPIO. 00237 * @param desc - The GPIO descriptor. 00238 * @param value - The value. 00239 * Example: GPIO_HIGH 00240 * GPIO_LOW 00241 * @return SUCCESS in case of success, FAILURE otherwise. 00242 */ 00243 int32_t gpio_set_value(struct gpio_desc *desc, uint8_t value) 00244 { 00245 DigitalOut *gpio_output; // pointer to gpio output object 00246 mbed_gpio_desc *gpio_desc_extra; // pointer to gpio desc extra parameters 00247 00248 if(desc && desc->extra) { 00249 gpio_desc_extra = (mbed_gpio_desc *)(desc->extra); 00250 00251 if (gpio_desc_extra->gpio_pin) { 00252 gpio_output = (DigitalOut *)((mbed_gpio_desc *)desc->extra)->gpio_pin; 00253 gpio_output->write(value); 00254 } 00255 00256 return SUCCESS; 00257 } 00258 00259 return FAILURE; 00260 } 00261 00262 00263 /** 00264 * @brief Get the value of the specified GPIO. 00265 * @param desc - The GPIO descriptor. 00266 * @param value - The value. 00267 * Example: GPIO_HIGH 00268 * GPIO_LOW 00269 * @return SUCCESS in case of success, FAILURE otherwise. 00270 */ 00271 int32_t gpio_get_value(struct gpio_desc *desc, uint8_t *value) 00272 { 00273 DigitalIn *gpio_input; // pointer to gpio input object 00274 uint8_t returnVal = FAILURE; 00275 00276 if (desc && desc->extra) { 00277 gpio_input = (DigitalIn *)((mbed_gpio_desc *)desc->extra)->gpio_pin; 00278 *value = (uint8_t)gpio_input->read(); 00279 returnVal = gpio_input->is_connected() ? SUCCESS : FAILURE; 00280 00281 return returnVal; 00282 } 00283 00284 return FAILURE; 00285 } 00286 00287 #ifdef __cplusplus 00288 } 00289 #endif // _cplusplus
Generated on Wed Jul 13 2022 14:37:51 by
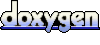