A collection of Analog Devices drivers for the mbed platform
Embed:
(wiki syntax)
Show/hide line numbers
Lcd.h
00001 /** 00002 ****************************************************************************** 00003 * @file Lcd.c 00004 * @brief Header file for ST7565R LCD control. 00005 * @author ADI 00006 * @date March 2016 00007 * 00008 ******************************************************************************* 00009 * Copyright 2015(c) Analog Devices, Inc. 00010 * 00011 * All rights reserved. 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * - Redistributions of source code must retain the above copyright 00016 * notice, this list of conditions and the following disclaimer. 00017 * - Redistributions in binary form must reproduce the above copyright 00018 * notice, this list of conditions and the following disclaimer in 00019 * the documentation and/or other materials provided with the 00020 * distribution. 00021 * - Neither the name of Analog Devices, Inc. nor the names of its 00022 * contributors may be used to endorse or promote products derived 00023 * from this software without specific prior written permission. 00024 * - The use of this software may or may not infringe the patent rights 00025 * of one or more patent holders. This license does not release you 00026 * from the requirement that you obtain separate licenses from these 00027 * patent holders to use this software. 00028 * - Use of the software either in source or binary form, must be run 00029 * on or directly connected to an Analog Devices Inc. component. 00030 * 00031 * THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR IMPLIED 00032 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, MERCHANTABILITY 00033 * AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 * IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00035 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00036 * INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00037 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00038 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00039 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00040 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 * 00042 ******************************************************************************* 00043 **/ 00044 #ifndef LCD_H_ 00045 #define LCD_H_ 00046 00047 00048 /******************************************************************************/ 00049 /***************************** Include Files **********************************/ 00050 /******************************************************************************/ 00051 #include <stdio.h> 00052 #include "mbed.h" 00053 00054 00055 /** 00056 * @brief Lcd class 00057 */ 00058 class Lcd 00059 { 00060 public: 00061 00062 const static uint8_t pui8Rec8x8[8]; 00063 const static uint8_t pui8RecInv8x8[8]; 00064 const static uint8_t pui8font5x7[96][5]; ///< Symbol matrix structure: Font (8x14) 00065 00066 Lcd(PinName rst = D3, PinName a0 = D5, PinName bl = D8, PinName cs = D6, 00067 PinName MOSI = SPI_MOSI, PinName MISO = SPI_MISO, PinName SCK = 00068 SPI_SCK); 00069 void init(void); 00070 void display_string(uint8_t ui8row, uint8_t ui8col, int8_t *pi8str); 00071 void display_symbol(uint8_t ui8row, uint8_t ui8col, uint8_t ui8width, 00072 const uint8_t *pui8symbol); 00073 void fill_pages(uint8_t ui8start, uint8_t ui8num, uint8_t ui8Data); 00074 void set_line(uint8_t ui8line); 00075 void set_cursor(uint8_t ui8PA, uint8_t ui8CA); 00076 void bl_enable(); 00077 void bl_disable(); 00078 00079 void write_cmd(uint8_t cmd); 00080 void write_data(uint8_t data); 00081 00082 00083 typedef enum { 00084 CMD_DISPLAY_OFF = 0xAE, 00085 CMD_DISPLAY_ON = 0xAF, 00086 CMD_SET_DISP_START_LINE = 0x40, 00087 CMD_SET_PAGE = 0xB0, 00088 CMD_SET_COLUMN_UPPER = 0x10, 00089 CMD_SET_COLUMN_LOWER = 0x00, 00090 CMD_SET_ADC_NORMAL = 0xA0, 00091 CMD_SET_ADC_REVERSE = 0xA1, 00092 CMD_SET_DISP_NORMAL = 0xA6, 00093 CMD_SET_DISP_REVERSE = 0xA7, 00094 CMD_SET_ALLPTS_NORMAL = 0xA4, 00095 CMD_SET_ALLPTS_ON = 0xA5, 00096 CMD_SET_BIAS_9 = 0xA2, 00097 CMD_SET_BIAS_7 = 0xA3, 00098 CMD_RMW = 0xE0, 00099 CMD_RMW_CLEAR = 0xEE, 00100 CMD_INTERNAL_RESET = 0xE2, 00101 CMD_SET_COM_NORMAL = 0xC0, 00102 CMD_SET_COM_REVERSE = 0xC8, 00103 CMD_SET_POWER_CONTROL = 0x28, 00104 CMD_SET_RESISTOR_RATIO = 0x20, 00105 CMD_SET_VOLUME_FIRST = 0x81, 00106 CMD_SET_VOLUME_SECOND = 0, 00107 CMD_SET_STATIC_OFF = 0xAC, 00108 CMD_SET_STATIC_ON = 0xAD, 00109 CMD_SET_STATIC_REG = 0x0, 00110 CMD_SET_BOOSTER_FIRST = 0xF8, 00111 CMD_SET_BOOSTER_234 = 0, 00112 CMD_SET_BOOSTER_5 = 1, 00113 CMD_SET_BOOSTER_6 = 3, 00114 CMD_NOP = 0xE3, 00115 CMD_TEST = 0xF0, 00116 } lcd_commands_t; 00117 00118 static const uint8_t LCD_COLUMNS = 128u; 00119 static const uint8_t LCD_PAGES = 4u; 00120 static const uint8_t LCD_LINES = 64u; 00121 static const uint8_t UP_X = 112; 00122 static const uint8_t LEFT_X = 104; 00123 static const uint8_t RIGHT_X = 120; 00124 static const uint8_t DOWN_X = 112; 00125 static const uint8_t CENTER_X = 112; 00126 static const uint8_t ACC_LIMIT = 80; 00127 static const uint8_t FONT_Y_SIZE = 8; ///< Font size for Y 00128 static const uint8_t OFFS_ASCII = 32; ///< ASCII offset 00129 00130 static const float ACC_TEMP_BIAS = 350 ; ///< Accelerometer temperature bias(in ADC codes) at 25 Deg C 00131 static const float ACC_TEMP_SENSITIVITY = 0.065; ///< Accelerometer temperature sensitivity from datasheet (DegC per Code) 00132 00133 private: 00134 00135 DigitalOut rst, a0, bl, cs; 00136 SPI lcd_spi; 00137 00138 00139 00140 }; 00141 00142 #endif /* LCD_H_ */
Generated on Tue Jul 12 2022 17:59:52 by
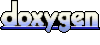